剑指offer-面试题5.从尾到头打印链表
2016-04-07 19:31
861 查看
题目:输入一个链表的头结点,从尾到头反过来打印出每个结点的值。
刚看到这道题的小伙伴可能就会想,这还不简单,将链表反转输出。但是这种情况破坏了链表的结构。如果面试官要求不破坏链表结构呢,这时候我们就想到了一种数据结构---栈
当我们从前往后遍历链表逐个压栈 然后遍历结束后再逐个出栈。
首先我们先来用第一种方式实现:
截图:

注意:这种情况下是破坏了链表的结构了。
下面我们用栈结构来实现不破链表本身结构的逆序输出:
截图:
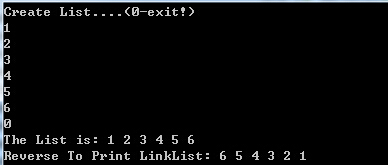
哎 好困。
刚看到这道题的小伙伴可能就会想,这还不简单,将链表反转输出。但是这种情况破坏了链表的结构。如果面试官要求不破坏链表结构呢,这时候我们就想到了一种数据结构---栈
当我们从前往后遍历链表逐个压栈 然后遍历结束后再逐个出栈。
首先我们先来用第一种方式实现:
1 #include <iostream> 2 using namespace std; 3 4 struct ListNode 5 { 6 int data; 7 struct ListNode *next; 8 }; 9 10 struct ListNode* CreateList() 11 { 12 struct ListNode* Head,*p; 13 Head=(struct ListNode*)malloc(sizeof(ListNode)); 14 Head->data=0; 15 Head->next=NULL; 16 p=Head; 17 18 cout<<"Create List....(0-exit!)"<<endl; 19 while(true) 20 { 21 int Data; 22 cin>>Data; 23 if(Data!=0) 24 { 25 struct ListNode* NewNode; 26 NewNode=(struct ListNode*)malloc(sizeof(ListNode)); 27 NewNode->data=Data; 28 NewNode->next=NULL; 29 p->next=NewNode; 30 p=p->next; 31 } 32 else 33 { 34 break; 35 } 36 } 37 38 return Head->next; 39 } 40 41 void PrintList(struct ListNode* Head) 42 { 43 cout<<"The List is: "; 44 45 struct ListNode *p; 46 p=Head; 47 while(p!=NULL) 48 { 49 cout<<p->data<<" "; 50 p=p->next; 51 } 52 cout<<endl; 53 } 54 55 struct ListNode* ReversePrint(struct ListNode* Head) 56 { 57 struct ListNode *p1,*p2,*p3; 58 59 p1=Head; 60 p2=p1->next; 61 62 while(p2!=NULL) 63 { 64 p3=p2->next; 65 p2->next=p1; 66 p1=p2; 67 p2=p3; 68 } 69 70 Head->next=NULL; 71 return p1; 72 } 73 74 int main() 75 { 76 ListNode *Head,*NewHead; 77 Head=CreateList(); 78 PrintList(Head); 79 NewHead=ReversePrint(Head); 80 81 cout<<endl<<"The Reverse List is:"<<endl; 82 PrintList(NewHead); 83 return 0; 84 }
截图:

注意:这种情况下是破坏了链表的结构了。
下面我们用栈结构来实现不破链表本身结构的逆序输出:
1 #include <iostream> 2 #include <stack> 3 using namespace std; 4 5 struct ListNode 6 { 7 int data; 8 struct ListNode *next; 9 }; 10 11 struct ListNode* CreateList() 12 { 13 struct ListNode* Head,*p; 14 Head=(struct ListNode*)malloc(sizeof(ListNode)); 15 Head->data=0; 16 Head->next=NULL; 17 p=Head; 18 19 cout<<"Create List....(0-exit!)"<<endl; 20 while(true) 21 { 22 int Data; 23 cin>>Data; 24 if(Data!=0) 25 { 26 struct ListNode* NewNode; 27 NewNode=(struct ListNode*)malloc(sizeof(ListNode)); 28 NewNode->data=Data; 29 NewNode->next=NULL; 30 p->next=NewNode; 31 p=p->next; 32 } 33 else 34 { 35 break; 36 } 37 } 38 39 return Head->next; 40 } 41 42 void PrintList(struct ListNode* Head) 43 { 44 cout<<"The List is: "; 45 46 struct ListNode *p; 47 p=Head; 48 while(p!=NULL) 49 { 50 cout<<p->data<<" "; 51 p=p->next; 52 } 53 cout<<endl; 54 } 55 56 void ReversePrintByStack(struct ListNode* Head) 57 { 58 struct ListNode* p; 59 p=Head; 60 61 stack<int> S; 62 63 while(p!=NULL) 64 { 65 S.push(p->data); 66 p=p->next; 67 } 68 69 cout<<"Reverse To Print LinkList: "; 70 while(!S.empty()) 71 { 72 cout<<S.top()<<" "; 73 S.pop(); 74 } 75 76 cout<<endl; 77 } 78 79 int main() 80 { 81 ListNode *Head,*NewHead; 82 Head=CreateList(); 83 PrintList(Head); 84 ReversePrintByStack(Head); 85 return 0; 86 }
截图:
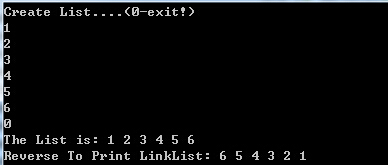
哎 好困。
相关文章推荐
- 剑指offer-面试题4.替换空格
- 剑指offer-面试题3.二维数组中的查找
- 剑指offer-面试题2.实例Singleton模式
- 剑指offer面试题 圆圈中最后剩下的数字(约瑟夫环问题)
- 剑指offer面试题 扑克牌的顺子
- 剑指offer-面试题1:赋值运算符函数
- 百度面试题:宏的概念,与函数的区别,优缺点的比较
- IT职业发展路线图
- Java程序员的日常——经验贴(纯干货)二
- 面试题53 正则表达式匹配
- 面试题52 构建乘积数组
- 面试题51 数组中重复的数字
- Android面试常问的技术问题
- LeetCodet题解--17. Letter Combinations of a Phone Number(所有数字键盘组合所对应的所有字符集合)
- 【面试/笔试】—— 数学
- php高级研发或架构师必了解---面试题系列
- 前端开发面试题--html
- 实用的SQL语句大全,面试或者工作都会用得着
- 数据库语言面试题
- 每个程序员都必读的11篇文章