使用POI实现Excel导入与导出
2017-10-08 19:36
736 查看
一、数据准备:
1、jar包:poi.jar
2、Excel模板存放路径:F:\ExcelsPath,在该目录下同时包含有文件和子目录
3、Excel格式:
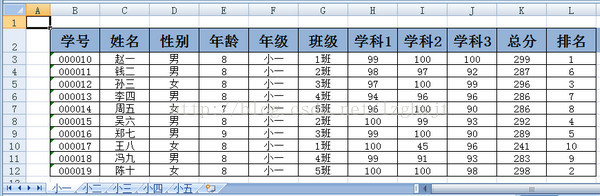
4、建表语句:
5、实体bean:
二、将Excel数据导入数据库:
1、读取Excel内容的工具类ExcelUtil:
3、主入口:
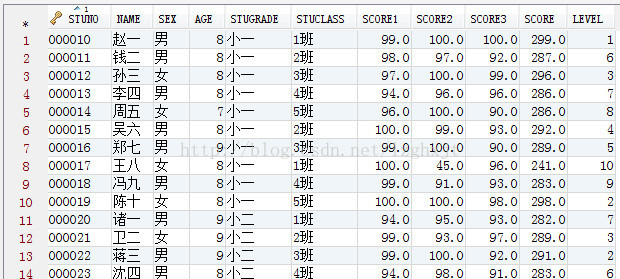
二、将数据库中数据导成Excel:
1、查询数据库中数据的工具类DBUtil:
2、写成Excel文件的工具类ExcelUtil:
最终导出的Excel内容为:
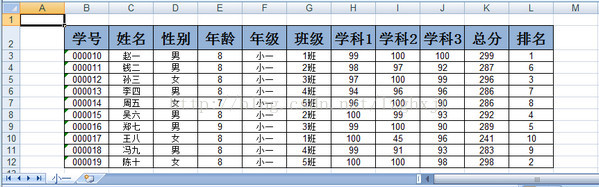
1、jar包:poi.jar
2、Excel模板存放路径:F:\ExcelsPath,在该目录下同时包含有文件和子目录
3、Excel格式:
4、建表语句:
CREATE TABLE STUDENT ( STUNO CHAR(6) NOT NULL, NAME VARCHAR(20) NOT NULL, SEX CHAR(1) NOT NULL, AGE INT NOT NULL, STUGRADE VARCHAR(10) NOT NULL, STUCLASS VARCHAR(10) NOT NULL, SCORE1 DOUBLE, SCORE2 DOUBLE, SCORE3 DOUBLE, SCORE DOUBLE, LEVEL INT )
5、实体bean:
public class Student { private String stuNo; private String name; private String sex; private int age; private String stuGrade; private String stuClass; private double score1; private double score2; private double score3; private double score; private int level; public String getStuNo() { return stuNo; } public void setStuNo(String stuNo) { this.stuNo = stuNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getStuGrade() { return stuGrade; } public void setStuGrade(String stuGrade) { this.stuGrade = stuGrade; } public String getStuClass() { return stuClass; } public void setStuClass(String stuClass) { this.stuClass = stuClass; } public double getScore1() { return score1; } public void setScore1(double score1) { this.score1 = score1; } public double getScore2() { return score2; } public void setScore2(double score2) { this.score2 = score2; } public double getScore3() { return score3; } public void setScore3(double score3) { this.score3 = score3; } public double getScore() { return score; } public void setScore(double score) { this.score = score; } public int getLevel() { return level; } public void setLevel(int level) { this.level = level; } }
二、将Excel数据导入数据库:
1、读取Excel内容的工具类ExcelUtil:
import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import org.apache.poi.hssf.usermodel.HSSFCell; import org.apache.poi.hssf.usermodel.HSSFRow; import org.apache.poi.hssf.usermodel.HSSFSheet; import org.apache.poi.hssf.usermodel.HSSFWorkbook; public class ExcelUtil { //要读取的excel文件所在的目录 private static String FilePath = "F:/ExcelsPath/"; //读取到的.xls文件列表 public static List<File> Files = null; //从excel读取到的数据 public static List<Map<String,Object>> ResultList = null; //读取根目录下的文件 public static void queryFiles(){ System.out.println("查询路径:"+FilePath+"下的所有文件"); Files = new ArrayList<File>(); File f = new File(FilePath); for(File tmp:f.listFiles()){ if(tmp.isFile()){ Files.add(tmp); }else{ List<File> subFiles = querysubFiles(tmp); if(subFiles!=null) Files.addAll(subFiles); } } if(Files.size()<1) Files=null; } //读取子目录及子目录下的文件 public static List<File> querysubFiles(File f){ List<File> subFiles = new ArrayList<File>(); if(f.isFile()){ subFiles.add(f); }else{ for(File tmp:f.listFiles()){ if(tmp.isFile()){ subFiles.add(tmp); }else{ List<File> tmpSubFiles = querysubFiles(tmp); if(tmpSubFiles!=null) subFiles.addAll(tmpSubFiles); } } } if(subFiles.size()<1) subFiles=null; return subFiles; } //读取Excel文件中的数据 public static void getCellDataFromExcel(List<File> files){ ResultList = new ArrayList<Map<String,Object>>(); FileInputStream fis = null; HSSFWorkbook wk = null; HSSFSheet sheet = null; HSSFRow row = null; HSSFCell cell = null; System.out.println("即将导入excel文件"+files.size()+"个"); for(File file:files){ try { String fileName = file.getName(); fis = new FileInputStream(file); wk = new HSSFWorkbook(fis); int sheetNum = wk.getNumberOfSheets(); System.out.println(fileName+"共有"+sheetNum+"个工作薄"); for(int i=0;i<sheetNum;i++){ sheet=wk.getSheetAt(i); String sheetName=sheet.getSheetName(); //从0开始数,最后一行的行号 int lastRowNum = sheet.getLastRowNum(); int count = lastRowNum-2+1; if(count>0){ System.out.println(fileName+"中["+sheetName+"]共有"+count+"行数据"); Map<String,Object> rowData = null; for(int j=2;j<=lastRowNum;j++){ rowData=new HashMap<String,Object>(); //获取第j行 row=sheet.getRow(j); //从0开始数,最后一列的列号+1 int cellNum=row.getLastCellNum(); for(int k=1;k<cellNum;k++){ //获取第j行的第k列 cell=row.getCell(k); //检查单元格中的数据格式 boolean boo = checkAndSetCellType(cell); if(boo){ if(k==1){ rowData.put("stuNo", cell.getStringCellValue()); }else if(k==2){ rowData.put("name", cell.getStringCellValue()); }else if(k==3){ rowData.put("sex", cell.getStringCellValue()); }else if(k==4){ rowData.put("age", (int)cell.getNumericCellValue()); }else if(k==5){ rowData.put("stuGrade", cell.getStringCellValue()); }else if(k==6){ rowData.put("stuClass", cell.getStringCellValue()); }else if(k==7){ rowData.put("score1", cell.getNumericCellValue()); }else if(k==8){ rowData.put("score2", cell.getNumericCellValue()); }else if(k==9){ rowData.put("score3", cell.getNumericCellValue()); }else if(k==10){ rowData.put("score", cell.getNumericCellValue()); }else if(k==11){ rowData.put("level", (int)cell.getNumericCellValue()); } }else{ continue; } } System.out.println("第"+j+"行数据读取成功"); ResultList.add(rowData); } System.out.println(fileName+"中["+sheetName+"]共有"+count+"行数据读取成功"); }else{ System.out.println(fileName+"中["+sheetName+"]无数据需导入"); } } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } } //检查Excel数据的格式 public static boolean checkAndSetCellType(HSSFCell cell){ boolean result = true; int rowIndex = cell.getRowIndex(); int cellIndex = cell.getColumnIndex(); if(cellIndex==1 || cellIndex==2 || cellIndex==3 || cellIndex==5 || cellIndex==6){ if(cell.getCellType()!=HSSFCell.CELL_TYPE_STRING){ System.out.println("第"+rowIndex+"行第"+cellIndex+"列数据格式不是文本"); result = false; } }else if(cellIndex==4 || cellIndex==7 || cellIndex==8 || cellIndex==9 || cellIndex==11){ if(cell.getCellType()!=HSSFCell.CELL_TYPE_NUMERIC){ System.out.println("第"+rowIndex+"行第"+cellIndex+"列数据格式不是数字"); result = false; } }else{ if(cell.getCellType()!=HSSFCell.CELL_TYPE_FORMULA){ System.out.println("第"+rowIndex+"行第"+cellIndex+"列数据格式不是公式"); result = false; } } if(cellIndex==1 || cellIndex==2 || cellIndex==3 || cellIndex==5 || cellIndex==6){ cell.setCellType(HSSFCell.CELL_TYPE_STRING); }else if(cellIndex==4 || cellIndex==7 || cellIndex==8 || cellIndex==9 || cellIndex==11){ cell.setCellType(HSSFCell.CELL_TYPE_NUMERIC); }else{ cell.setCellType(HSSFCell.CELL_TYPE_NUMERIC); } return result; } }2、插入数据库的工具类DBUtil:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; import java.util.List; import java.util.Map; public class DBUtil { private static String DriverName = "com.mysql.jdbc.Driver"; private static String Url="jdbc:mysql://localhost:3306/mysql"; private static String UserName = "root"; private static String PassWord = "root"; private static Connection Con; public static Student[] Students = null; //获取数据库连接 public static Connection getConnection(){ try { Class.forName(DriverName); try { Con = DriverManager.getConnection(Url,UserName,PassWord); Con.setAutoCommit(false); } catch (SQLException e) { e.printStackTrace(); } } catch (ClassNotFoundException e) { e.printStackTrace(); } return Con; } //读到的数据插入数据库 public static boolean insertDbList(Student[] students){ boolean boo = true; String sql = "insert into Student(stuNo,name,sex,age,stuGrade,stuClass,score1,score2,score3,score,level) values (?,?,?,?,?,?,?,?,?,?,?)"; try { PreparedStatement ps = Con.prepareStatement(sql); for(Student student:students){ ps.setString(1, student.getStuNo()); ps.setString(2, student.getName()); ps.setString(3, student.getSex()); ps.setInt(4, student.getAge()); ps.setString(5, student.getStuGrade()); 1080d ps.setString(6, student.getStuClass()); ps.setDouble(7, student.getScore1()); ps.setDouble(8, student.getScore2()); ps.setDouble(9, student.getScore3()); ps.setDouble(10, student.getScore()); ps.setInt(11, student.getLevel()); ps.addBatch(); } int[] results = ps.executeBatch(); for(int i=0;i<results.length;i++){ if(results[i]==0){ System.out.println("学号:"+students[i].getStuNo()+"的信息插库失败"); boo=false; Con.rollback(); break; } } if(boo){ Con.commit(); System.out.println("共成功导入"+students.length+"条数据"); } } catch (SQLException e) { e.printStackTrace(); } finally{ try { if(!Con.isClosed()){ Con.close(); } } catch (SQLException e) { e.printStackTrace(); } } return boo; } //Map转化为实体类 public static void convertMap2Entity(List<Map<String,Object>> list){ if(list!=null && list.size()>0){ Students = new Student[list.size()]; for(int i=0;i<list.size();i++){ Map<String,Object> tmpMap=list.get(i); Students[i] = new Student(); if(tmpMap.containsKey("stuNo")){ Students[i].setStuNo(tmpMap.get("stuNo").toString()); } if(tmpMap.containsKey("name")){ Students[i].setName(tmpMap.get("name").toString()); } if(tmpMap.containsKey("sex")){ Students[i].setSex(tmpMap.get("sex").toString()); } if(tmpMap.containsKey("age")){ Students[i].setAge(Integer.parseInt(tmpMap.get("age").toString())); } if(tmpMap.containsKey("stuGrade")){ Students[i].setStuGrade(tmpMap.get("stuGrade").toString()); } if(tmpMap.containsKey("stuClass")){ Students[i].setStuClass(tmpMap.get("stuClass").toString()); } if(tmpMap.containsKey("score1")){ Students[i].setScore1(Double.parseDouble(tmpMap.get("score1").toString())); } if(tmpMap.containsKey("score2")){ Students[i].setScore2(Double.parseDouble(tmpMap.get("score2").toString())); } if(tmpMap.containsKey("score3")){ Students[i].setScore3(Double.parseDouble(tmpMap.get("score3").toString())); } if(tmpMap.containsKey("score")){ Students[i].setScore(Double.parseDouble(tmpMap.get("score").toString())); } if(tmpMap.containsKey("level")){ Students[i].setLevel(Integer.parseInt(tmpMap.get("level").toString())); } } } } }
3、主入口:
public class Import { public static void main(String[] args) { ExcelUtil.queryFiles(); ExcelUtil.getCellDataFromExcel(ExcelUtil.Files); DBUtil.getConnection() DBUtil.convertMap2Entity(ExcelUtil.ResultList); boolean b = DBUtil.insertDbList(DBUtil.Students); } }最终导入的数据库中为:
二、将数据库中数据导成Excel:
1、查询数据库中数据的工具类DBUtil:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; public class DBUtil { private static String DriverName = "com.mysql.jdbc.Driver"; private static String Url="jdbc:mysql://localhost:3306/mysql"; private static String UserName = "root"; private static String PassWord = "root"; private static Connection Con; public static Student[] Students = null; //查询条件 public static Student QueryCon = null; //从数据库读取到的数据 public static List<Map<String,Object>> DataList = null; //获取数据库连接 public static Connection getConnection(){ try { Class.forName(DriverName); try { Con = DriverManager.getConnection(Url,UserName,PassWord); Con.setAutoCommit(false); } catch (SQLException e) { e.printStackTrace(); } } catch (ClassNotFoundException e) { e.printStackTrace(); } return Con; } //设置查询条件 public static void setQueryCondition(){ QueryCon = new Student(); QueryCon.setStuGrade("小一"); } //根据查询条件查询符合条件的记录 public static void queryDbData(){ DataList = new ArrayList<Map<String,Object>>(); StringBuffer sqlStr = new StringBuffer("select stuNo,name,sex,age,stuGrade,stuClass,score1,score2,score3,score,level from Student where 1=1"); if(QueryCon.getStuGrade()!=null && !"".equals(QueryCon.getStuGrade())) sqlStr.append(" and stuGrade='"+QueryCon.getStuGrade()+"'"); Statement sta=null; try { sta = Con.createStatement(); ResultSet tmpResult = sta.executeQuery(sqlStr.toString()); while(tmpResult.next()){ Map<String,Object> rowMap = new HashMap<String,Object>(); rowMap.put("stuNo", tmpResult.getString(1)); rowMap.put("name", tmpResult.getString(2)); rowMap.put("sex", tmpResult.getString(3)); rowMap.put("age", tmpResult.getInt(4)); rowMap.put("stuGrade", tmpResult.getString(5)); rowMap.put("stuClass", tmpResult.getString(6)); rowMap.put("score1", tmpResult.getDouble(7)); rowMap.put("score2", tmpResult.getDouble(8)); rowMap.put("score3", tmpResult.getDouble(9)); rowMap.put("score", tmpResult.getDouble(10)); rowMap.put("level", tmpResult.getInt(11)); DataList.add(rowMap); } } catch (SQLException e) { e.printStackTrace(); } if(DataList.size()<1) DataList=null; } //Map转化为实体 public static void convertMap2Entity(List<Map<String,Object>> list){ if(list!=null && list.size()>0){ Students = new Student[list.size()]; for(int i=0;i<list.size();i++){ Map<String,Object> tmpMap=list.get(i); Students[i] = new Student(); if(tmpMap.containsKey("stuNo")){ Students[i].setStuNo(tmpMap.get("stuNo").toString()); } if(tmpMap.containsKey("name")){ Students[i].setName(tmpMap.get("name").toString()); } if(tmpMap.containsKey("sex")){ Students[i].setSex(tmpMap.get("sex").toString()); } if(tmpMap.containsKey("age")){ Students[i].setAge(Integer.parseInt(tmpMap.get("age").toString())); } if(tmpMap.containsKey("stuGrade")){ Students[i].setStuGrade(tmpMap.get("stuGrade").toString()); } if(tmpMap.containsKey("stuClass")){ Students[i].setStuClass(tmpMap.get("stuClass").toString()); } if(tmpMap.containsKey("score1")){ Students[i].setScore1(Double.parseDouble(tmpMap.get("score1").toString())); } if(tmpMap.containsKey("score2")){ Students[i].setScore2(Double.parseDouble(tmpMap.get("score2").toString())); } if(tmpMap.containsKey("score3")){ Students[i].setScore3(Double.parseDouble(tmpMap.get("score3").toString())); } if(tmpMap.containsKey("score")){ Students[i].setScore(Double.parseDouble(tmpMap.get("score").toString())); } if(tmpMap.containsKey("level")){ Students[i].setLevel(Integer.parseInt(tmpMap.get("level").toString())); } } } } }
2、写成Excel文件的工具类ExcelUtil:
import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import org.apache.poi.hssf.usermodel.HSSFCell; import org.apache.poi.hssf.usermodel.HSSFCellStyle; import org.apache.poi.hssf.usermodel.HSSFFont; import org.apache.poi.hssf.usermodel.HSSFPalette; import org.apache.poi.hssf.usermodel.HSSFRow; import org.apache.poi.hssf.usermodel.HSSFSheet; import org.apache.poi.hssf.usermodel.HSSFWorkbook; import org.apache.poi.hssf.util.HSSFColor; public class ExcelUtil { public static boolean writeExcel(Student[] students){ boolean result = false; HSSFWorkbook wk = new HSSFWorkbook(); //表头的样式 HSSFCellStyle headStyle = wk.createCellStyle(); //表头内容水平居中 headStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER); //表头内容垂直居中 headStyle.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER); //表头中字体 HSSFFont headFont = wk.createFont(); //表头字体为宋体 headFont.setFontName("宋体"); //表头字体大小为16 headFont.setFontHeightInPoints((short)16); //表头字体加粗 headFont.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD); //字体样式放入表头样式 headStyle.setFont(headFont); //填充背景色 headStyle.setFillForegroundColor(HSSFColor.LIME.index); headStyle.setFillPattern(HSSFCellStyle.SOLID_FOREGROUND); //自定义颜色 HSSFPalette palette = wk.getCustomPalette(); //设置RGB颜色 palette.setColorAtIndex(HSSFColor.LIME.index, (byte) 149, (byte) 179, (byte) 215); //设置表头下边框 headStyle.setBorderBottom(HSSFCellStyle.BORDER_THIN); //设置表头左边框 headStyle.setBorderLeft(HSSFCellStyle.BORDER_THIN); //设置表头上边框 headStyle.setBorderTop(HSSFCellStyle.BORDER_THIN); //设置表头右边框 headStyle.setBorderRight(HSSFCellStyle.BORDER_THIN); //数据内容的样式 HSSFCellStyle bodyStyle = wk.createCellStyle(); //数据内容水平居中 bodyStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER); //数据内容垂直居中 bodyStyle.setVerticalAlignment(HSSFCellStyle.VERTICAL_CENTER); //设置数据内容下边框 bodyStyle.setBorderBottom(HSSFCellStyle.BORDER_THIN); //设置数据内容左边框 bodyStyle.setBorderLeft(HSSFCellStyle.BORDER_THIN); //设置数据内容上边框 bodyStyle.setBorderTop(HSSFCellStyle.BORDER_THIN); //设置数据内容右边框 bodyStyle.setBorderRight(HSSFCellStyle.BORDER_THIN); //设置sheet页名为查询条件的内容 HSSFSheet sheet = wk.createSheet(DBUtil.QueryCon.getStuGrade()); //设置表头高度为525 HSSFRow row = sheet.createRow(1); row.setHeight((short)525); //每个表头的单元格设置样式 HSSFCell cell1 = row.createCell(1); cell1.setCellStyle(headStyle); cell1.setCellValue("学号"); HSSFCell cell2 = row.createCell(2); cell2.setCellStyle(headStyle); cell2.setCellValue("姓名"); HSSFCell cell3 = row.createCell(3); cell3.setCellStyle(headStyle); cell3.setCellValue("性别"); HSSFCell cell4 = row.createCell(4); cell4.setCellStyle(headStyle); cell4.setCellValue("年龄"); HSSFCell cell5 = row.createCell(5); cell5.setCellStyle(headStyle); cell5.setCellValue("年级"); HSSFCell cell6 = row.createCell(6); cell6.setCellStyle(headStyle); cell6.setCellValue("班级"); HSSFCell cell7 = row.createCell(7); cell7.setCellStyle(headStyle); cell7.setCellValue("学科1"); HSSFCell cell8 = row.createCell(8); cell8.setCellStyle(headStyle); cell8.setCellValue("学科2"); HSSFCell cell9 = row.createCell(9); cell9.setCellStyle(headStyle); cell9.setCellValue("学科3"); HSSFCell cell10 = row.createCell(10); cell10.setCellStyle(headStyle); cell10.setCellValue("总分"); HSSFCell cell11 = row.createCell(11); cell11.setCellStyle(headStyle); cell11.setCellValue("排名"); for(int i=0;i<students.length;i++){ Student student = students[i]; //每行的每个数据单元格设置样式 HSSFRow dataRow = sheet.createRow(i+2); HSSFCell dataCell1 = dataRow.createCell(1); dataCell1.setCellStyle(bodyStyle); dataCell1.setCellValue(student.getStuNo()); HSSFCell dataCell2 = dataRow.createCell(2); dataCell2.setCellStyle(bodyStyle); dataCell2.setCellValue(student.getName()); HSSFCell dataCell3 = dataRow.createCell(3); dataCell3.setCellStyle(bodyStyle); dataCell3.setCellValue(student.getSex()); HSSFCell dataCell4 = dataRow.createCell(4); dataCell4.setCellStyle(bodyStyle); dataCell4.setCellValue(student.getAge()); HSSFCell dataCell5 = dataRow.createCell(5); dataCell5.setCellStyle(bodyStyle); dataCell5.setCellValue(student.getStuGrade()); HSSFCell dataCell6 = dataRow.createCell(6); dataCell6.setCellStyle(bodyStyle); dataCell6.setCellValue(student.getStuClass()); HSSFCell dataCell7 = dataRow.createCell(7); dataCell7.setCellStyle(bodyStyle); dataCell7.setCellValue(student.getScore1()); HSSFCell dataCell8 = dataRow.createCell(8); dataCell8.setCellStyle(bodyStyle); dataCell8.setCellValue(student.getScore2()); HSSFCell dataCell9 = dataRow.createCell(9); dataCell9.setCellStyle(bodyStyle); dataCell9.setCellValue(student.getScore3()); HSSFCell dataCell10 = dataRow.createCell(10); dataCell10.setCellStyle(bodyStyle); dataCell10.setCellValue(student.getScore()); HSSFCell dataCell11 = dataRow.createCell(11); dataCell11.setCellStyle(bodyStyle); dataCell11.setCellValue(student.getLevel()); }; FileOutputStream fs = null; try { //导出的Excel文件存放路径 fs = new FileOutputStream("F:\\成绩表.xls"); wk.write(fs); result = true; } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } finally{ try { fs.close(); } catch (IOException e) { e.printStackTrace(); } } return result; } }3、主入口:
public class Exprot { public static void main(String[] args) { DBUtil.setQueryCondition(); DBUtil.getConnection(); DBUtil.queryDbData(); DBUtil.convertMap2Entity(DBUtil.DataList); ExcelUtil.writeExcel(DBUtil.Students); } }
最终导出的Excel内容为:
相关文章推荐
- SpringBoot中使用POI,快速实现Excel导入导出
- Jenke 使用apache的poi实现导入导出excel
- 使用apache的poi实现导入导出excel
- Java-Maven-POI 简单导入导出Excel通用工具,默认使用基于poi实现
- Spring使用POI实现Excel导入导出
- Spring使用POI实现Excel导入导出
- 使用apache的poi实现导入导出excel
- SpringBoot中使用POI,快速实现Excel导入导出
- 使用apache的poi实现导入导出excel
- 在SSM下使用POI实现Excel表的导入/导出
- 使用POI实现在java程序中导入导出Excel文件数据
- 【JavaWeb开发】使用java实现简单的Excel文件的导入与导出(POI)
- Spring使用POI实现Excel导入导出
- 在SSM下使用POI实现Excel表的导入/导出
- 在SSM下使用POI实现Excel表的导入/导出
- 使用POI 实现 Excel 导入导出
- 记 springmvc使用POI,快速实现Excel导入导出
- ThinkPHP使用phpExcel实现Excel数据的导入导出
- JAVA实现数据库数据导入/导出到Excel(POI)
- ThinkPHP使用PHPExcel实现Excel数据导入导出完整实例