C#之入门总结_网络编程_18
2017-08-10 17:23
435 查看
ISO/OSI模型简介
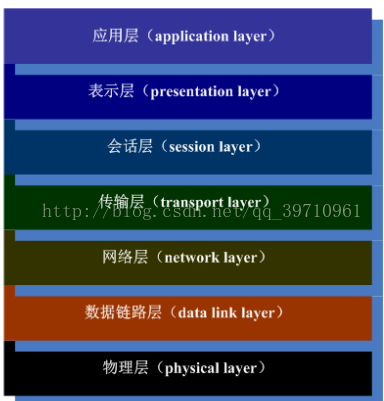
ISO:国际标准化组织
OSI:开放系统互联结构模型
ISO/OSI模型把网络分成了若干层,每层都实现特定的功能。
ISO/OSI模型把网络表示成竖直的线,模型中的每一层次至少包含有一个协议,所以也可以说是模型中的协议是逐个叠放的。协议栈是个由竖直的层和对方的协议抽象而来。
OSI不是一个实际的物理模型,而是一个将网络协议规范化了的逻辑参考模型
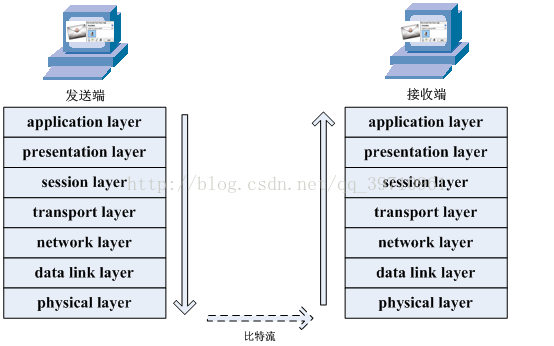
常见的三种协议:
IP协议:网际协议(Internet Protocol)
TCP协议: 传输控制协议(Transmission Control Protocol)
UDP协议:用户数据协议(User Datagram Protocol)
Microsoft.Net Framework为应用程序访问Internet提供了分层的、可扩展的以及受管辖的网络服务,其名字空间System.Net和System.Net.Sockets包含丰富的类可以开发多种网络应用程序。.Net类采用的分层结构允许应用程序在不同的控制级别上访问网络,开发人员可以根据需要选择针对不同的级别编制程序,这些级别几乎囊括了Internet的所有需要--从socket套接字到普通的请求/响应,更重要的是,这种分层是可以扩展的,能够适应Internet不断扩展的需要。
抛开ISO/OSI模型的7层构架,单从TCP/IP模型上的逻辑层面上看,.Net类可以视为包含3个层次:请求/响应层、应用协议层、传输层。WebReqeust和WebResponse 代表了请求/响应层,支持Http、Tcp和Udp的类组成了应用协议层,而Socket类处于传输层。可以如下图示意:
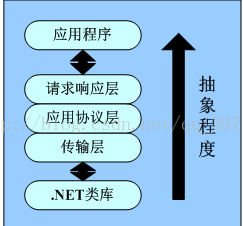
可见,传输层位于这个结构的最底层,当其上面的应用协议层和请求/响应层不能满足应用程序的特殊需要时,就需要使用这一层进行Socket套接字编程。
在.Net中,System.Net.Sockets 命名空间为需要严密控制网络访问的开发人员提供了 Windows Sockets (Winsock) 接口的托管实现。System.Net 命名空间中的所有其他网络访问类都建立在该套接字Socket实现之上,如TCPClient、TCPListener 和 UDPClient 类封装有关创建到 Internet 的 TCP 和 UDP 连接的详细信息;NetworkStream类则提供用于网络访问的基础数据流等,常见的许多Internet服务都可以见到Socket的踪影,如Telnet、Http、Email、Ftp等,这些服务尽管通讯协议Protocol的定义不同,但是其基础的传输都是采用的Socket。
Scoket TCP编程的流程图
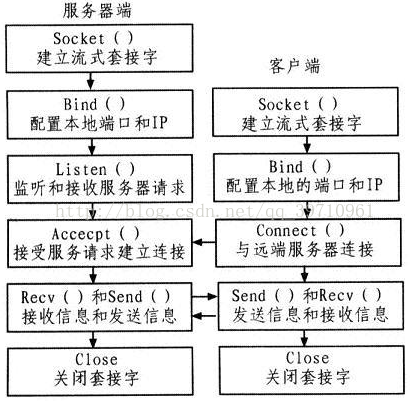
TCP的三次握手
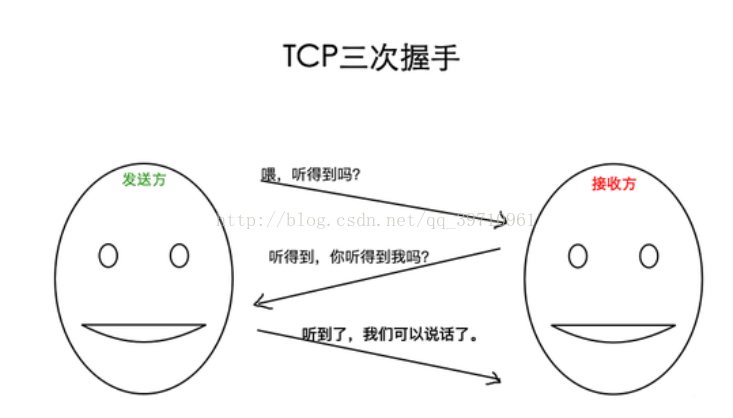
TCP的四次挥手
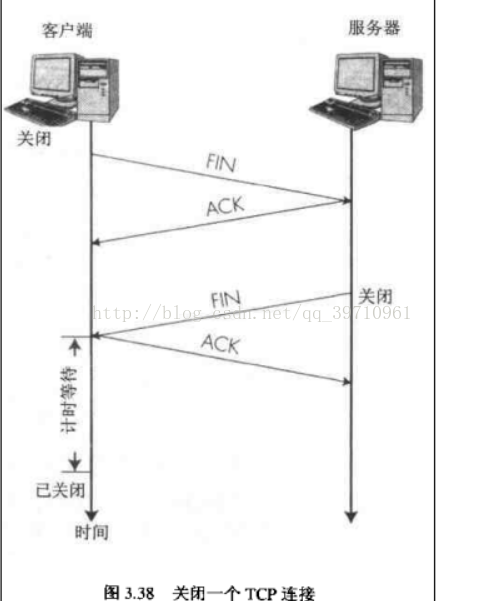
namespace TcpServer
{
class Program
{
static TcpListener server;
static void Main(string[] args)
{
IPAddress ip = IPAddress.Parse("127.0.0.1");
IPEndPoint ipendPoint = new IPEndPoint(ip, 9999);
server = new TcpListener(ipendPoint);
server.Start();
Thread th = new Thread(Liseten);
th.Start();
}
static void Liseten()
{
TcpClient client = null ;
try
{
while (true)
{
client = server.AcceptTcpClient();
Console.WriteLine("连接成功");
NetworkStream netWorkStream = client.GetStream();
Console.WriteLine(client.Connected);
byte[] buffer = new byte[4096];
int len = 0;
try
{
while ((len = netWorkStream.Read(buffer, 0, buffer.Length)) != 0)
{
Console.WriteLine(Encoding.Default.GetString(buffer, 0, len));
}
}
catch (Exception e)
{
//不做任何处理即可 这里不影响服务器和客户端
}
}
}
catch (SocketException e)
{
if (client.Connected)
{
client.Close();
}
Console.WriteLine("***************");
}
catch (Exception e)
{
Console.WriteLine(e);
}
}
}
}
namespace TcpClientDemo
{
class Program
{
static void Main(string[] args)
{
TcpClient client = new TcpClient();
client.Connect("127.0.0.1", 9999);
while (true)
{
string str = Console.ReadLine();
byte[] buffer = Encoding.Default.GetBytes(str);
NetworkStream networkStream = new NetworkStream(client.Client);
networkStream.Write(buffer, 0, buffer.Length);
}
4000
}
}
}
Scoket UDP编程的流程图
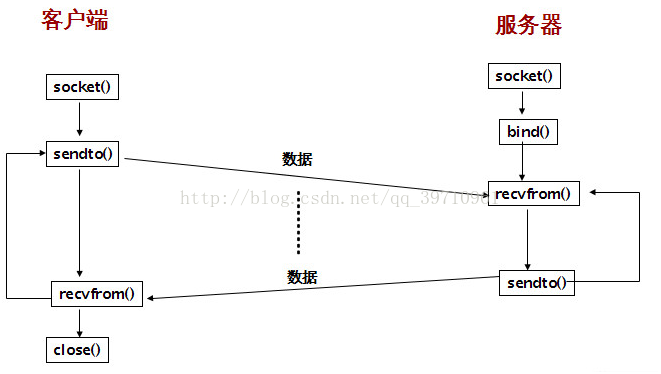
UDP套接口是无连接的、不可靠的数据报协议;
为什么要使用这种不可靠的协议呢?
其一:当应用程序使用广播或多播时只能使用UDP协议;
其二:由于他是无连接的,所以速度快。
UDP收发数据的流程:
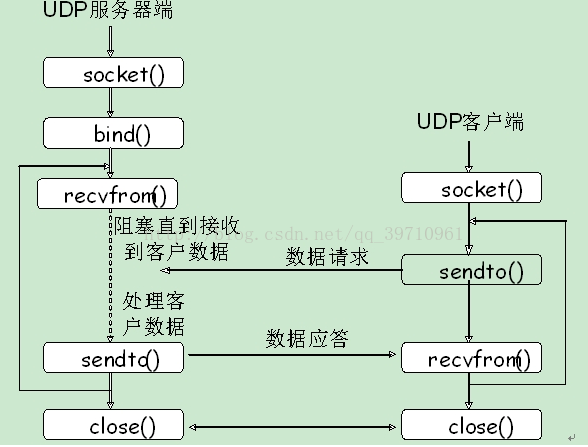
namespace UDPClientDemo
{
class Program
{
static void Main(string[] args)
{
UdpClient client = new UdpClient("127.0.0.1", 9999);
try
{
while (true)
{
string str = Console.ReadLine();
byte[] buffer = Encoding.Default.GetBytes(str);
client.Send(buffer, buffer.Length);
Console.WriteLine("发送完毕");
}
}
catch (Exception e)
{
Console.WriteLine(e);
}
}
}
}
class Program
{
static void Main(string[] args)
{
UdpClient udpServer = new UdpClient(9999);
try
{
while (true)
{
IPEndPoint ipendpoint = new IPEndPoint(IPAddress.Any, 0);
byte[] buffer = udpServer.Receive(ref ipendpoint);
string ret = Encoding.Default.GetString(buffer, 0, buffer.Length);
Console.WriteLine("来自{0}:{1}的信息是{2}", ipendpoint.Address, ipendpoint.Port, ret);
}
}
catch (Exception e)
{
Console.WriteLine(e);
}
TCP和UDP的区别!
1.基于连接与无连接;
2.对系统资源的要求(TCP较多,UDP少);
3.UDP程序结构较简单;
4.流模式与数据报模式 ;
5.TCP保证数据正确性,UDP可能丢包,TCP保证数据顺序,UDP不保证。
ISO:国际标准化组织
OSI:开放系统互联结构模型
ISO/OSI模型把网络分成了若干层,每层都实现特定的功能。
ISO/OSI模型把网络表示成竖直的线,模型中的每一层次至少包含有一个协议,所以也可以说是模型中的协议是逐个叠放的。协议栈是个由竖直的层和对方的协议抽象而来。
OSI不是一个实际的物理模型,而是一个将网络协议规范化了的逻辑参考模型
常见的三种协议:
IP协议:网际协议(Internet Protocol)
TCP协议: 传输控制协议(Transmission Control Protocol)
UDP协议:用户数据协议(User Datagram Protocol)
Microsoft.Net Framework为应用程序访问Internet提供了分层的、可扩展的以及受管辖的网络服务,其名字空间System.Net和System.Net.Sockets包含丰富的类可以开发多种网络应用程序。.Net类采用的分层结构允许应用程序在不同的控制级别上访问网络,开发人员可以根据需要选择针对不同的级别编制程序,这些级别几乎囊括了Internet的所有需要--从socket套接字到普通的请求/响应,更重要的是,这种分层是可以扩展的,能够适应Internet不断扩展的需要。
抛开ISO/OSI模型的7层构架,单从TCP/IP模型上的逻辑层面上看,.Net类可以视为包含3个层次:请求/响应层、应用协议层、传输层。WebReqeust和WebResponse 代表了请求/响应层,支持Http、Tcp和Udp的类组成了应用协议层,而Socket类处于传输层。可以如下图示意:
可见,传输层位于这个结构的最底层,当其上面的应用协议层和请求/响应层不能满足应用程序的特殊需要时,就需要使用这一层进行Socket套接字编程。
在.Net中,System.Net.Sockets 命名空间为需要严密控制网络访问的开发人员提供了 Windows Sockets (Winsock) 接口的托管实现。System.Net 命名空间中的所有其他网络访问类都建立在该套接字Socket实现之上,如TCPClient、TCPListener 和 UDPClient 类封装有关创建到 Internet 的 TCP 和 UDP 连接的详细信息;NetworkStream类则提供用于网络访问的基础数据流等,常见的许多Internet服务都可以见到Socket的踪影,如Telnet、Http、Email、Ftp等,这些服务尽管通讯协议Protocol的定义不同,但是其基础的传输都是采用的Socket。
Scoket TCP编程的流程图
TCP的三次握手
TCP的四次挥手
namespace TcpServer
{
class Program
{
static TcpListener server;
static void Main(string[] args)
{
IPAddress ip = IPAddress.Parse("127.0.0.1");
IPEndPoint ipendPoint = new IPEndPoint(ip, 9999);
server = new TcpListener(ipendPoint);
server.Start();
Thread th = new Thread(Liseten);
th.Start();
}
static void Liseten()
{
TcpClient client = null ;
try
{
while (true)
{
client = server.AcceptTcpClient();
Console.WriteLine("连接成功");
NetworkStream netWorkStream = client.GetStream();
Console.WriteLine(client.Connected);
byte[] buffer = new byte[4096];
int len = 0;
try
{
while ((len = netWorkStream.Read(buffer, 0, buffer.Length)) != 0)
{
Console.WriteLine(Encoding.Default.GetString(buffer, 0, len));
}
}
catch (Exception e)
{
//不做任何处理即可 这里不影响服务器和客户端
}
}
}
catch (SocketException e)
{
if (client.Connected)
{
client.Close();
}
Console.WriteLine("***************");
}
catch (Exception e)
{
Console.WriteLine(e);
}
}
}
}
namespace TcpClientDemo
{
class Program
{
static void Main(string[] args)
{
TcpClient client = new TcpClient();
client.Connect("127.0.0.1", 9999);
while (true)
{
string str = Console.ReadLine();
byte[] buffer = Encoding.Default.GetBytes(str);
NetworkStream networkStream = new NetworkStream(client.Client);
networkStream.Write(buffer, 0, buffer.Length);
}
4000
}
}
}
Scoket UDP编程的流程图
UDP套接口是无连接的、不可靠的数据报协议;
为什么要使用这种不可靠的协议呢?
其一:当应用程序使用广播或多播时只能使用UDP协议;
其二:由于他是无连接的,所以速度快。
UDP收发数据的流程:
namespace UDPClientDemo
{
class Program
{
static void Main(string[] args)
{
UdpClient client = new UdpClient("127.0.0.1", 9999);
try
{
while (true)
{
string str = Console.ReadLine();
byte[] buffer = Encoding.Default.GetBytes(str);
client.Send(buffer, buffer.Length);
Console.WriteLine("发送完毕");
}
}
catch (Exception e)
{
Console.WriteLine(e);
}
}
}
}
class Program
{
static void Main(string[] args)
{
UdpClient udpServer = new UdpClient(9999);
try
{
while (true)
{
IPEndPoint ipendpoint = new IPEndPoint(IPAddress.Any, 0);
byte[] buffer = udpServer.Receive(ref ipendpoint);
string ret = Encoding.Default.GetString(buffer, 0, buffer.Length);
Console.WriteLine("来自{0}:{1}的信息是{2}", ipendpoint.Address, ipendpoint.Port, ret);
}
}
catch (Exception e)
{
Console.WriteLine(e);
}
TCP和UDP的区别!
1.基于连接与无连接;
2.对系统资源的要求(TCP较多,UDP少);
3.UDP程序结构较简单;
4.流模式与数据报模式 ;
5.TCP保证数据正确性,UDP可能丢包,TCP保证数据顺序,UDP不保证。
相关文章推荐
- C#精通C#编程:玩转开发实例...C#编程入门、C#编程实例、C#开发实例与网络编程等....
- C#编程入门18_进程和线程
- C#网络编程入门3
- C#网络编程入门4
- [C#]网络编程入门的几个介绍
- 黑马程序员-java网络编程入门总结
- C#编程入门21_网络编程
- [C#]网络编程入门的几个介绍2
- C#编程入门_进程和线程_18
- Java基础学习总结(18)——网络编程
- Java基础学习总结(18)——网络编程
- C#编程入门_网络编程_21
- C#网络编程入门1
- Java基础学习总结(18)——网络编程
- JAVA知识点总结-18 网络编程
- C#网络编程socket使用总结
- 总结C#网络编程中对于Cookie的设定要点
- C#网络编程入门2
- C#编程总结(三)线程同步
- 【Linux网络编程】 网络协议入门(很透彻,跟风学习)