求一个二叉树中两个结点的最大距离
2017-05-19 17:30
381 查看
首先说一下距离这个词,在二叉树中距离就是从一个节点到另一个结点的边的条数,为了更为清晰的描述这个概念,下面来看一张图:
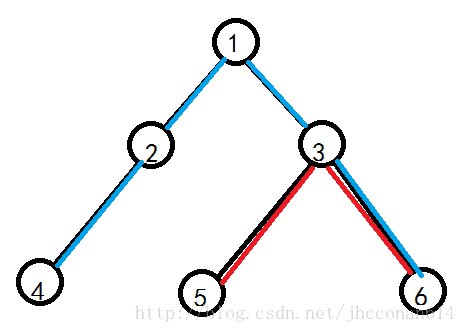
看上面的图,蓝色线表示的是结点4到6的距离,红色线表示的是从结点5到6的距离,从图中我们可以看到,不是所有的距离都经过根结点的,这一点很重要。
那么怎么求最远距离呢?
上面的那个图中,我们可以很容易看出最远的距离就是从4到6,或者是从4到5,都是一样的。而且我们还发现,他们分别是左子树的最底层的结点和右子树最底层的结点。那么我们可不可以这样算呢,分别求出左子树和右子树的高度,然后让他们相加再加上1(根结点)。
这是最远的吗?
显然不是,下面我们再来看一个例子:
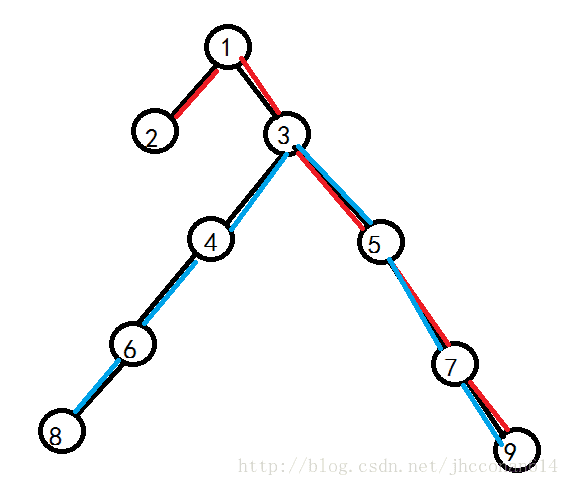
如果按照上面我们算的,最远路径应该是从2到9,也就是红色线,但是我们发现,从8到9更长。那么这里又有什么规律呢?
我们在自习看一下这个图,我们发现不管是哪一条,他们都分别在一个结点的左右子树中,所以,我们可以将所有的结点的左右子树的高度和计算一下,然后取出最大值,就是最远的距离。
接下来看一下这个问题的实现过程:
看上面的图,蓝色线表示的是结点4到6的距离,红色线表示的是从结点5到6的距离,从图中我们可以看到,不是所有的距离都经过根结点的,这一点很重要。
那么怎么求最远距离呢?
上面的那个图中,我们可以很容易看出最远的距离就是从4到6,或者是从4到5,都是一样的。而且我们还发现,他们分别是左子树的最底层的结点和右子树最底层的结点。那么我们可不可以这样算呢,分别求出左子树和右子树的高度,然后让他们相加再加上1(根结点)。
这是最远的吗?
显然不是,下面我们再来看一个例子:
如果按照上面我们算的,最远路径应该是从2到9,也就是红色线,但是我们发现,从8到9更长。那么这里又有什么规律呢?
我们在自习看一下这个图,我们发现不管是哪一条,他们都分别在一个结点的左右子树中,所以,我们可以将所有的结点的左右子树的高度和计算一下,然后取出最大值,就是最远的距离。
接下来看一下这个问题的实现过程:
#include<iostream> using namespace std; #include<string> // 孩子表示法 template<class T> struct BinaryTreeNode { BinaryTreeNode(const T& value) : _value(value) , _pLeft(NULL) , _pRight(NULL) {} T _value; BinaryTreeNode<T>* _pLeft; // 左孩子 BinaryTreeNode<T>* _pRight; // 右孩子 }; template<class T> class BinaryTree1 { typedef BinaryTreeNode<T> Node; public: BinaryTree1() :_pRoot(NULL) {} BinaryTree1(const T array[], size_t size, const T& invalid) { size_t index = 0; _CreateBinaryTree(_pRoot, array, size, index, invalid); } //求一个二叉树中两个结点的最大距离 void MaxDistance() { int _maxlenth = 0; //左右子树中最大深度的和 cout << _MaxDistance(_pRoot, _maxlenth) << endl; cout << _maxlenth << endl; } protected: //创建二叉树 void _CreateBinaryTree(Node* &pRoot, const T array[], size_t size, size_t& index, const T& invalid) { if (index < size&&array[index] != invalid) { pRoot = new Node(invalid);//注意new的时候要和结构体中写的函数参数对应 pRoot->_value = array[index]; _CreateBinaryTree(pRoot->_pLeft, array, size, ++index, invalid);//注意:++index _CreateBinaryTree(pRoot->_pRight, array, size, ++index, invalid); } } size_t _MaxDistance(Node* pRoot, int &maxlenth) { if (pRoot == NULL) return 0; //左右子树的长度 int leftlenth = _MaxDistance(pRoot->_pLeft, maxlenth); int rightlenth = _MaxDistance(pRoot->_pRight, maxlenth); //用一个临时变量保存当前的最远距离,如果大于原来的,那么交换 int temp = leftlenth + rightlenth; if (temp > maxlenth) maxlenth = temp; return leftlenth > rightlenth ? leftlenth + 1 : rightlenth + 1; } private: Node* _pRoot; }; void FunTest() { char array[] = "12##3468####5#7#9"; //char array[] = "124###35##6"; BinaryTree1<char> bt(array, strlen(array), '#'); bt.MaxDistance(); } int main() { FunTest(); return 0; }
相关文章推荐
- 求一个二叉树中任意两个节点间的最大距离,两个节点的距离的定义是这两个节点间边的个数,比如某个孩子节点和父节点间的距离是1,和相邻兄弟节点间的距离是2,
- 求一个二叉树中任意两个节点间的最大距离
- 每天学习一算法系列(34)(求一个二叉树中任意两个节点间的最大距离,两个节点的距离的定义是这两个节点间边的个数)
- 求一个二叉树中任意两个节点间的最大距离
- 利用栈结构实现二叉树的非递归遍历,求二叉树深度、叶子节点数、两个结点的最近公共祖先及二叉树结点的最大距离
- 【每日面试题】求一个二叉树中任意两个节点间的最大距离
- 求一个二叉树中任意两个节点间的最大距离
- 利用栈结构实现二叉树的非递归遍历,求二叉树深度、叶子节点数、两个结点的最近公共祖先及二叉树结点的最大距离
- 利用栈结构实现二叉树的非递归遍历,求二叉树深度、叶子节点数、两个结点的最近公共祖先及二叉树结点的最大距离
- 写一个函数,输入一个二叉树,树中每一个节点存放了一个整数值,函数返回这棵二叉树中相差最大的两个节点间的差值绝对值。请注意程序效率。
- 二叉排序树中,令f = (最大值+最小值) / 2,设计一个算法, 找出距离f值最近、大于f值的结点。复杂度不能为O(n2)。
- 求二叉树中结点的最大距离
- 14、一棵排序二叉树,令 f=(最大值+最小值)/2, 设计一个算法,找出距离f值最近、大于f值的结点。
- 求二叉树结点最大距离
- 一棵排序二叉树(即二叉搜索树BST),令 f=(最大值+最小值)/2,设计一个算 //法,找出距离f值最近、大于f值的结点。复杂度如果是O(n2)则不得分。
- 【100题】第三十九题 二叉树任意两个节点间最大距离和有向图割点
- 3.8——求二叉树中结点的最大距离(树,递归,动态规划)
- 二叉树中任意两个节点之间的最大距离
- 二叉树中结点的最大距离
- 树(2)求二叉树结点最大距离