React -- 组件生命周期
2017-05-03 15:03
435 查看
React的生命周期分为挂载、渲染和卸载三个阶段。
这三个阶段分为两类:
1、组件在挂载或卸载时
2、组件接受新的数据时,即组件更新渲染时
propTypes和defaultProps代表props类型检查和默认类型,并且被声明为静态属性,我们可以使用APP.propTypes这样的方式访问它们。
声明周期方法componentWillMount将在render方法前执行,而componentDidMount则会在render方法后执行。
如果我们在componentWillMount中执行setState: 组件会更新state,但是只渲染一次。因此,不必要在componentWillMount中再次执行setState, 直接在constructor中将state设置稳妥即可。
但是如果在componentDidMount中执行了setState,组件就会再次更新,这样,初始化的过程中组件就渲染了两次,这样效率不高,不是推荐的做法。
2、组件的卸载
组件的卸载很简单,涉及的生命周期方法为componentWillUnmount。在这个方法中,通常执行一些清理方法,例如事件回收和清除定时器等。
如果组件自身的state更新了,那么就会依次执行shouldComponentUpdate、componentWillUpdate、render、componentDidUpdate。
shouldComponentUpdate:它接收需要更新的props和state,开发者可以根据需要,判断是否需要更新,如果不需要更新,将方法返回false,组件将不会再向下执行生命周期的方法。
shouldComponentUpdate的本质是用来进行正确的组件渲染。当父节点的props改变的时候,在理想的情况下, 应该只渲染某DOM树上某一条链路上的节点。而在默认情况下,React会渲染此父节点下所有的跟节点(shouldComponentUpdate默认返回true),因此,利用shouldComponentUpdate方法砍去没必要的渲染,是性能优化的手段之一。
另外,关于componentWillUpdate和componentDidUpdate,前者提供需要更新的props和state,后者提供更新前的props和state。需要注意的是,不能在componentWillUpdate中执行setState。
如果组件是由于父组件更新了它的props而更新的,那么在shouldComponentUpdate方法之前,会先执行componentWillReceiveProps方法。此方法可以作为React在props传入后,渲染之前setState的机会,在此方法中调用setState是不会二次渲染的。
整体流程图:
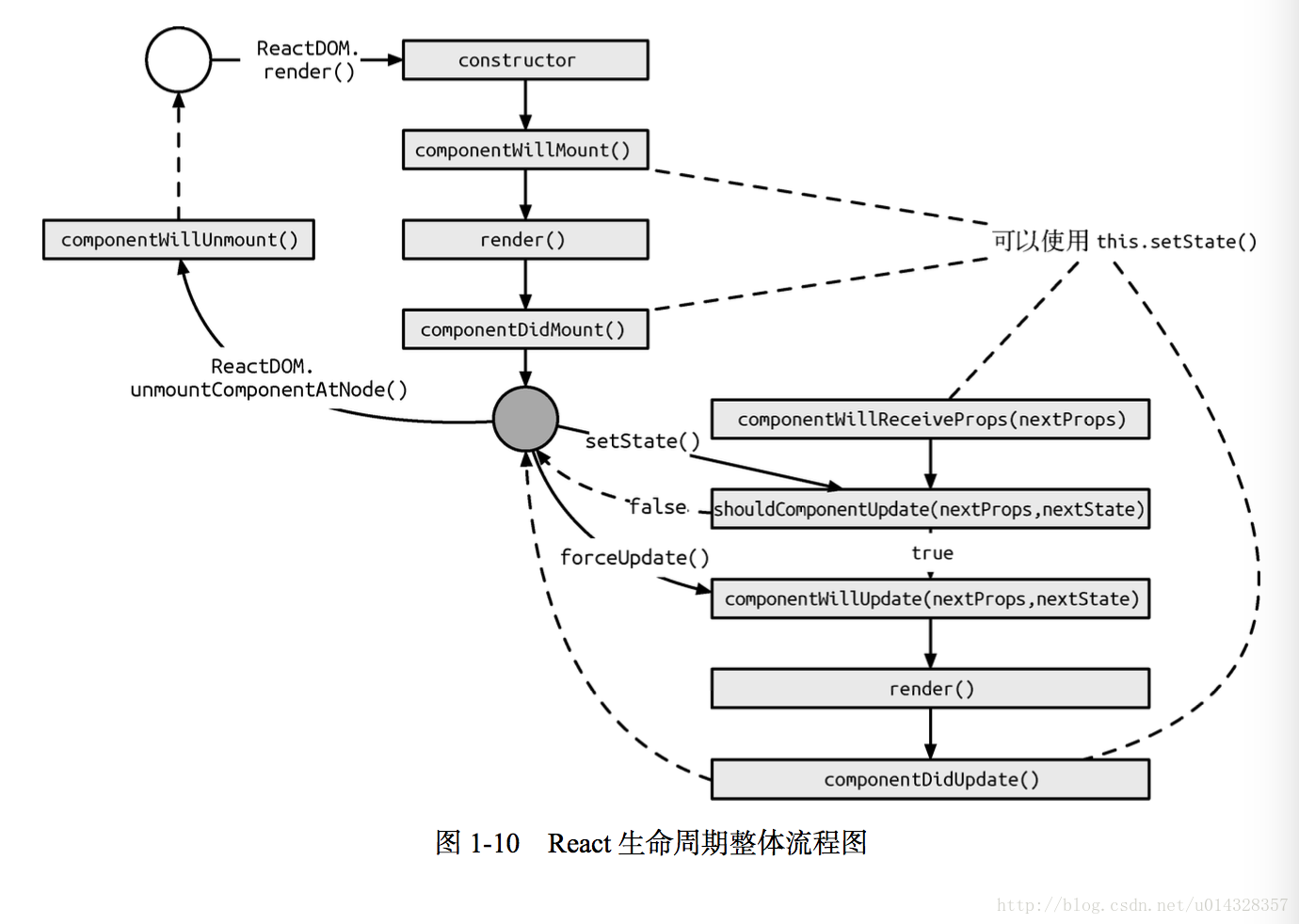
使用ES6 class与creatClass的异同:
这三个阶段分为两类:
1、组件在挂载或卸载时
2、组件接受新的数据时,即组件更新渲染时
卸载和挂载
1、组件的挂载class App extends Component { static propTypes = { // ... }; static defaultProps = { // ... }; constructor(props) { super(props); this.state = { // ... }; } componentWillMount() { // ... } componentDidMount() { // ... } render() { return <div>This is a demo.</div>; } }
propTypes和defaultProps代表props类型检查和默认类型,并且被声明为静态属性,我们可以使用APP.propTypes这样的方式访问它们。
声明周期方法componentWillMount将在render方法前执行,而componentDidMount则会在render方法后执行。
如果我们在componentWillMount中执行setState: 组件会更新state,但是只渲染一次。因此,不必要在componentWillMount中再次执行setState, 直接在constructor中将state设置稳妥即可。
但是如果在componentDidMount中执行了setState,组件就会再次更新,这样,初始化的过程中组件就渲染了两次,这样效率不高,不是推荐的做法。
2、组件的卸载
组件的卸载很简单,涉及的生命周期方法为componentWillUnmount。在这个方法中,通常执行一些清理方法,例如事件回收和清除定时器等。
数据的更新过程
更新过程指的是父组件向下传递props活着组件自身执行setState方法时发生的一系列更新动作。如果组件自身的state更新了,那么就会依次执行shouldComponentUpdate、componentWillUpdate、render、componentDidUpdate。
shouldComponentUpdate(nextProps, nextState) { // return true; }
shouldComponentUpdate:它接收需要更新的props和state,开发者可以根据需要,判断是否需要更新,如果不需要更新,将方法返回false,组件将不会再向下执行生命周期的方法。
shouldComponentUpdate的本质是用来进行正确的组件渲染。当父节点的props改变的时候,在理想的情况下, 应该只渲染某DOM树上某一条链路上的节点。而在默认情况下,React会渲染此父节点下所有的跟节点(shouldComponentUpdate默认返回true),因此,利用shouldComponentUpdate方法砍去没必要的渲染,是性能优化的手段之一。
另外,关于componentWillUpdate和componentDidUpdate,前者提供需要更新的props和state,后者提供更新前的props和state。需要注意的是,不能在componentWillUpdate中执行setState。
如果组件是由于父组件更新了它的props而更新的,那么在shouldComponentUpdate方法之前,会先执行componentWillReceiveProps方法。此方法可以作为React在props传入后,渲染之前setState的机会,在此方法中调用setState是不会二次渲染的。
整体流程图:
使用ES6 class与creatClass的异同:
相关文章推荐
- react组件生命周期
- react-native组件的生命周期
- React组件生命周期详解
- React——组件的生命周期函数
- React:组件的生命周期
- React组件的生命周期及执行顺序
- React组件生命周期
- React组件生命周期
- React组件生命周期
- react 初探:类组件、状态和生命周期
- 简述 React 组件生命周期
- Android React Native组件的生命周期及回调函数
- react-组件生命周期
- 【JAVASCRIPT】React学习-组件生命周期
- 老生常谈js-react组件生命周期
- 第八章 React组件的生命周期
- React 组件的生命周期API和事件处理
- React组件生命周期过程说明
- React入门教程 - 组件生命周期
- react组件的生命周期