observer, pull or push?
2011-10-25 10:33
134 查看
1. 解决的问题:
假如现在要编写一个天气预报的公布栏, 公布栏有两种显示方式, 一种是图像方式显示, 一种是表格形式显示.
2. 问题分析:
应该根据数据与现实分离的原则将天气预报数据和现实形式分别封装起来,
今后可能增加其他的显示形式;
天气预报数据发生变化后,需要对所有的显示形式进行更新.
3. UML图与代码实现:
1)用Push的方式更新Observer数据, 通过Subject对Observer进行注册:
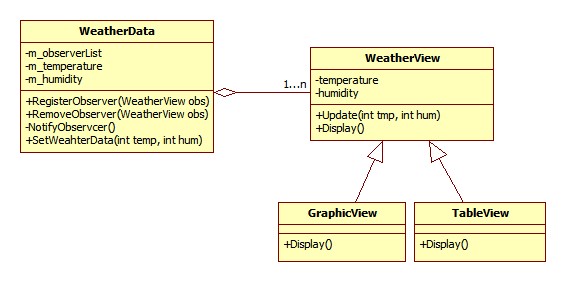
view plaincopy to clipboardprint?
//这个例子中WeatherData就是Subject, 而WeatherView则是Observer,
//这里WeatherView中没有包含到WeatherData的引用,
//因此这里是Subject用push的方法向Observer发送数据;
//并且注册和反注册Observer的时候都是由Subject(WeatherData)执行的
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
class WeatherData;
class WeatherView
{
public:
void Update(int temp, int hum)
{
temperature = temp;
humidity = hum;
};
virtual void Display()
{
cout << "temperature = " << temperature << ", humidity = " << humidity << endl;
}
private:
int temperature;
int humidity;
};
class GraphicView: public WeatherView
{
public:
void Display()
{
cout << "====--Weather Report With Graphic Format--===="<< endl;
WeatherView::Display();
}
};
class TableView: public WeatherView
{
public:
void Display()
{
cout << "====--Weather Report With Table Format--===="<< endl;
WeatherView::Display();
}
};
class WeatherData
{
public:
void SetWeahterData(int temp, int hum)
{
temperature = temp;
humidity = hum;
NotifyObservcer();
}
void RegisterObserver(WeatherView* obs)
{
obsList.push_back(obs);
}
void RemoveObserver(WeatherView* obs)
{
vector<WeatherView*>::iterator it;
it = find(obsList.begin(), obsList.end(), obs);
if (it != obsList.end())
obsList.erase(it);
}
private:
vector<WeatherView*> obsList;
int temperature;
int humidity;
void NotifyObservcer()
{
vector<WeatherView*>::iterator it;
for(it = obsList.begin(); it < obsList.end(); it++)
{
(*it)->Update(temperature, humidity);
}
}
};
int main()
{
WeatherData *wd = new WeatherData();
GraphicView *gv = new GraphicView();
TableView *tv = new TableView();
wd->RegisterObserver(gv);
wd->RegisterObserver(tv);
wd->SetWeahterData(23,45);
gv->Display();
tv->Display();
wd->RemoveObserver(gv);
wd->SetWeahterData(67,89);
gv->Display();
tv->Display();
return 0;
}
view plaincopy to clipboardprint?
#ifndef WEATHERVIEW_HPP_INCLUDED
#define WEATHERVIEW_HPP_INCLUDED
#include <iostream>
#include <vector>
#include <algorithm>
class WeatherData;
using namespace std;
class WeatherView
{
public:
WeatherView(WeatherData* wd);
void Update();
void Register();
void Unregister();
virtual void Display()
{
cout << "temperature = " << temperature << ", humidity = " << humidity << endl;
}
private:
WeatherData* wd;
int temperature;
int humidity;
};
class GraphicView: public WeatherView
{
public:
GraphicView(WeatherData* wd);
void Display()
{
cout << "====--Weather Report With Graphic Format--===="<< endl;
WeatherView::Display();
}
};
class TableView: public WeatherView
{
public:
TableView(WeatherData* wd);
void Display()
{
cout << "====--Weather Report With Table Format--===="<< endl;
WeatherView::Display();
}
};
#endif
上面两种实现的执行结果如下:
====--Weather Report With Graphic Format--====
temperature = 23, humidity = 45
====--Weather Report With Table Format--====
temperature = 23, humidity = 45
====--Weather Report With Graphic Format--====
temperature = 23, humidity = 45
====--Weather Report With Table Format--====
temperature = 67, humidity = 89
====--Weather Report With Graphic Format--====
temperature = 23, humidity = 45
====--Weather Report With Table Format--====
temperature = 23, humidity = 45
====--Weather Report With Graphic Format--====
temperature = 23, humidity = 45
====--Weather Report With Table Format--====
temperature = 67, humidity = 89
4. Push还是Pull?
对于上面的例子, Observer中的数据是从Subject中一次性全部更新的(temperature 和 humidity), 这种更新数据的方式便是push;然而如果WeatherData中的数据量非常大, 而有些Observer并不需要所有的数据, 比如现在要新增两个显示方式,一个是只显示温度,而另一个则只显示湿度, 这样的话就没有必要让所有的Observer都得到所有的数据. 最好的方式是Observer能根据自己的需要从Subject中去取得数据,这种更新数据的方式便是Pull. Observer模式中Push和Pull两种设计方法体现在具体的程序中就是Observer中的Update()接口参数不同,
对于Push模式, Update()接口的参数通常就是需要Push的那些数据,比如这里的温度和湿度; 对于Pull模式, Update()的参数是Subject的一个引用, 然后Subject提供一些数据接口,由Observer通过这些接口自己取得所需要的数据.
5. 总结:
1. Strategy 模式定义:
定义对象间的一种一对多的依赖关系,当一个对象的状态发生改变时,所有依赖于它的对象都得到通知并被自动更新.
2. 体现的设计原则:
将数据与现实分别封装;
多使用组合,少使用继承;
面向接口编程,而不面向实现编程;
3. UML图:
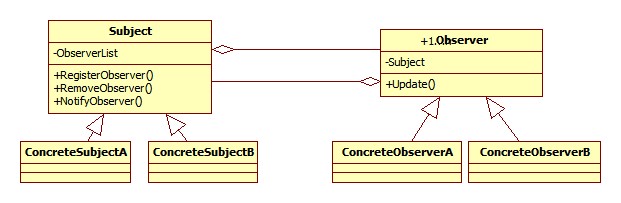
4. 要点:
Strategy 基类需要定义出可供Client使用的一些算法接口;
可以随时根据需要增加 Strategy 而不会影响到Client;
Client 里面需要包含对 Strategy 的引用;
Client 可以随时更换 Strategy;
6. 理解:
Observer模式是解决对象之间数据传递问题的一种模式;
Observer的注册可以由Subject执行也可以由Observer自己执行;
和Strategy模式的比较:
1) Observer模式中Observer 中定义了 Update()接口供 Subject调用; 而Strategy模式中,Strategy定义了AlgrithmInterface()供Client调用;
2) Observer模式中Subject和Observer是一对多的关系, 因此Subject是一次调用n个Observer的Update()接口;而Strategy模式中Client与Strategy之间是一对一的关系, Client 就是调用指定的那个Strategy的AlgrithmInterface();
3) 也正因为这种对应关系的不同, 在Observer模式中, Subject可以Register或者Remove某个Observer, 而Strategy模式中通常只是set某个Strategy
假如现在要编写一个天气预报的公布栏, 公布栏有两种显示方式, 一种是图像方式显示, 一种是表格形式显示.
2. 问题分析:
应该根据数据与现实分离的原则将天气预报数据和现实形式分别封装起来,
今后可能增加其他的显示形式;
天气预报数据发生变化后,需要对所有的显示形式进行更新.
3. UML图与代码实现:
1)用Push的方式更新Observer数据, 通过Subject对Observer进行注册:
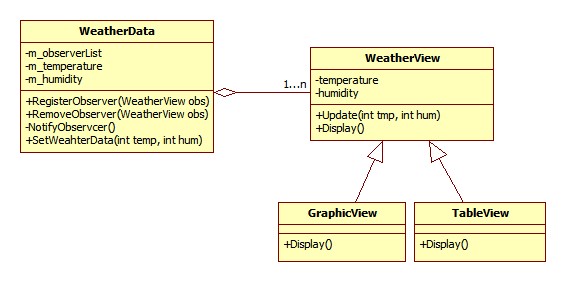
view plaincopy to clipboardprint?
//这个例子中WeatherData就是Subject, 而WeatherView则是Observer,
//这里WeatherView中没有包含到WeatherData的引用,
//因此这里是Subject用push的方法向Observer发送数据;
//并且注册和反注册Observer的时候都是由Subject(WeatherData)执行的
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
class WeatherData;
class WeatherView
{
public:
void Update(int temp, int hum)
{
temperature = temp;
humidity = hum;
};
virtual void Display()
{
cout << "temperature = " << temperature << ", humidity = " << humidity << endl;
}
private:
int temperature;
int humidity;
};
class GraphicView: public WeatherView
{
public:
void Display()
{
cout << "====--Weather Report With Graphic Format--===="<< endl;
WeatherView::Display();
}
};
class TableView: public WeatherView
{
public:
void Display()
{
cout << "====--Weather Report With Table Format--===="<< endl;
WeatherView::Display();
}
};
class WeatherData
{
public:
void SetWeahterData(int temp, int hum)
{
temperature = temp;
humidity = hum;
NotifyObservcer();
}
void RegisterObserver(WeatherView* obs)
{
obsList.push_back(obs);
}
void RemoveObserver(WeatherView* obs)
{
vector<WeatherView*>::iterator it;
it = find(obsList.begin(), obsList.end(), obs);
if (it != obsList.end())
obsList.erase(it);
}
private:
vector<WeatherView*> obsList;
int temperature;
int humidity;
void NotifyObservcer()
{
vector<WeatherView*>::iterator it;
for(it = obsList.begin(); it < obsList.end(); it++)
{
(*it)->Update(temperature, humidity);
}
}
};
int main()
{
WeatherData *wd = new WeatherData();
GraphicView *gv = new GraphicView();
TableView *tv = new TableView();
wd->RegisterObserver(gv);
wd->RegisterObserver(tv);
wd->SetWeahterData(23,45);
gv->Display();
tv->Display();
wd->RemoveObserver(gv);
wd->SetWeahterData(67,89);
gv->Display();
tv->Display();
return 0;
}
view plaincopy to clipboardprint? #ifndef WEATHERDATA_HPP_INCLUDED #define WEATHERDATA_HPP_INCLUDED #include <iostream> #include <vector> #include "WeatherView.hpp" class WeatherData { public: void SetWeahterData(int temp, int hum) { temperature = temp; humidity = hum; NotifyObservcer(); } int GetTemperature() { return temperature; } int GetHumidty() { return humidity; } void RegisterObserver(WeatherView* obs); void RemoveObserver(WeatherView* obs); private: vector<WeatherView*> obsList; int temperature; int humidity; void NotifyObservcer(); }; #endif #ifndef WEATHERDATA_HPP_INCLUDED #define WEATHERDATA_HPP_INCLUDED #include <iostream> #include <vector> #include "WeatherView.hpp" class WeatherData { public: void SetWeahterData(int temp, int hum) { temperature = temp; humidity = hum; NotifyObservcer(); } int GetTemperature() { return temperature; } int GetHumidty() { return humidity; } void RegisterObserver(WeatherView* obs); void RemoveObserver(WeatherView* obs); private: vector<WeatherView*> obsList; int temperature; int humidity; void NotifyObservcer(); }; #endif
view plaincopy to clipboardprint?
#ifndef WEATHERVIEW_HPP_INCLUDED
#define WEATHERVIEW_HPP_INCLUDED
#include <iostream>
#include <vector>
#include <algorithm>
class WeatherData;
using namespace std;
class WeatherView
{
public:
WeatherView(WeatherData* wd);
void Update();
void Register();
void Unregister();
virtual void Display()
{
cout << "temperature = " << temperature << ", humidity = " << humidity << endl;
}
private:
WeatherData* wd;
int temperature;
int humidity;
};
class GraphicView: public WeatherView
{
public:
GraphicView(WeatherData* wd);
void Display()
{
cout << "====--Weather Report With Graphic Format--===="<< endl;
WeatherView::Display();
}
};
class TableView: public WeatherView
{
public:
TableView(WeatherData* wd);
void Display()
{
cout << "====--Weather Report With Table Format--===="<< endl;
WeatherView::Display();
}
};
#endif
view plaincopy to clipboardprint? //这个例子中WeatherData就是Subject, 而WeatherView则是Observer, //这里WeatherView中有一个包含到WeatherData的指针, //因此这里是Observer用pull的方法主动向Observer索取数据; //并且注册和反注册都是Observer自己执行的 #include <iostream> #include <vector> #include <algorithm> #include "WeatherView.hpp" #include "WeatherData.hpp" void WeatherData::RegisterObserver(WeatherView* obs) { obsList.push_back(obs); } void WeatherData::RemoveObserver(WeatherView* obs) { vector<WeatherView*>::iterator it; it = find(obsList.begin(), obsList.end(), obs); if (it != obsList.end()) obsList.erase(it); } void WeatherData::NotifyObservcer() { vector<WeatherView*>::iterator it; for(it = obsList.begin(); it < obsList.end(); it++) { (*it)->Update(); } } WeatherView::WeatherView(WeatherData* pwd) { wd = pwd; } void WeatherView::Update() { temperature = wd->GetTemperature(); humidity = wd->GetHumidty(); }; void WeatherView::Register() { wd->RegisterObserver(this); }; void WeatherView::Unregister() { wd->RemoveObserver(this); }; GraphicView::GraphicView(WeatherData* pwd) : WeatherView(pwd) { } TableView::TableView(WeatherData* pwd) : WeatherView(pwd) { } int main() { WeatherData *wd = new WeatherData(); GraphicView *gv = new GraphicView(wd); gv->Register(); TableView *tv = new TableView(wd); tv->Register(); wd->SetWeahterData(23,45); gv->Display(); tv->Display(); gv->Unregister(); wd->SetWeahterData(67,89); gv->Display(); tv->Display(); return 0; } //这个例子中WeatherData就是Subject, 而WeatherView则是Observer, //这里WeatherView中有一个包含到WeatherData的指针, //因此这里是Observer用pull的方法主动向Observer索取数据; //并且注册和反注册都是Observer自己执行的 #include <iostream> #include <vector> #include <algorithm> #include "WeatherView.hpp" #include "WeatherData.hpp" void WeatherData::RegisterObserver(WeatherView* obs) { obsList.push_back(obs); } void WeatherData::RemoveObserver(WeatherView* obs) { vector<WeatherView*>::iterator it; it = find(obsList.begin(), obsList.end(), obs); if (it != obsList.end()) obsList.erase(it); } void WeatherData::NotifyObservcer() { vector<WeatherView*>::iterator it; for(it = obsList.begin(); it < obsList.end(); it++) { (*it)->Update(); } } WeatherView::WeatherView(WeatherData* pwd) { wd = pwd; } void WeatherView::Update() { temperature = wd->GetTemperature(); humidity = wd->GetHumidty(); }; void WeatherView::Register() { wd->RegisterObserver(this); }; void WeatherView::Unregister() { wd->RemoveObserver(this); }; GraphicView::GraphicView(WeatherData* pwd) : WeatherView(pwd) { } TableView::TableView(WeatherData* pwd) : WeatherView(pwd) { } int main() { WeatherData *wd = new WeatherData(); GraphicView *gv = new GraphicView(wd); gv->Register(); TableView *tv = new TableView(wd); tv->Register(); wd->SetWeahterData(23,45); gv->Display(); tv->Display(); gv->Unregister(); wd->SetWeahterData(67,89); gv->Display(); tv->Display(); return 0; }
上面两种实现的执行结果如下:
====--Weather Report With Graphic Format--====
temperature = 23, humidity = 45
====--Weather Report With Table Format--====
temperature = 23, humidity = 45
====--Weather Report With Graphic Format--====
temperature = 23, humidity = 45
====--Weather Report With Table Format--====
temperature = 67, humidity = 89
====--Weather Report With Graphic Format--====
temperature = 23, humidity = 45
====--Weather Report With Table Format--====
temperature = 23, humidity = 45
====--Weather Report With Graphic Format--====
temperature = 23, humidity = 45
====--Weather Report With Table Format--====
temperature = 67, humidity = 89
4. Push还是Pull?
对于上面的例子, Observer中的数据是从Subject中一次性全部更新的(temperature 和 humidity), 这种更新数据的方式便是push;然而如果WeatherData中的数据量非常大, 而有些Observer并不需要所有的数据, 比如现在要新增两个显示方式,一个是只显示温度,而另一个则只显示湿度, 这样的话就没有必要让所有的Observer都得到所有的数据. 最好的方式是Observer能根据自己的需要从Subject中去取得数据,这种更新数据的方式便是Pull. Observer模式中Push和Pull两种设计方法体现在具体的程序中就是Observer中的Update()接口参数不同,
对于Push模式, Update()接口的参数通常就是需要Push的那些数据,比如这里的温度和湿度; 对于Pull模式, Update()的参数是Subject的一个引用, 然后Subject提供一些数据接口,由Observer通过这些接口自己取得所需要的数据.
5. 总结:
1. Strategy 模式定义:
定义对象间的一种一对多的依赖关系,当一个对象的状态发生改变时,所有依赖于它的对象都得到通知并被自动更新.
2. 体现的设计原则:
将数据与现实分别封装;
多使用组合,少使用继承;
面向接口编程,而不面向实现编程;
3. UML图:
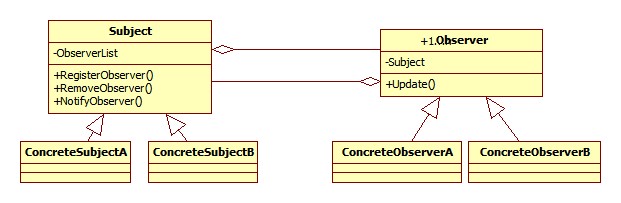
4. 要点:
Strategy 基类需要定义出可供Client使用的一些算法接口;
可以随时根据需要增加 Strategy 而不会影响到Client;
Client 里面需要包含对 Strategy 的引用;
Client 可以随时更换 Strategy;
6. 理解:
Observer模式是解决对象之间数据传递问题的一种模式;
Observer的注册可以由Subject执行也可以由Observer自己执行;
和Strategy模式的比较:
1) Observer模式中Observer 中定义了 Update()接口供 Subject调用; 而Strategy模式中,Strategy定义了AlgrithmInterface()供Client调用;
2) Observer模式中Subject和Observer是一对多的关系, 因此Subject是一次调用n个Observer的Update()接口;而Strategy模式中Client与Strategy之间是一对一的关系, Client 就是调用指定的那个Strategy的AlgrithmInterface();
3) 也正因为这种对应关系的不同, 在Observer模式中, Subject可以Register或者Remove某个Observer, 而Strategy模式中通常只是set某个Strategy
相关文章推荐
- Push or Pull
- Integrates Git with Sublime 3 to pull or push to Github by using Sublime plugin Git
- Push Or Pull?
- Push OR Pull
- push or pull 与hadoop 的机制
- Push or Pull?
- Refactoring--Pull Up /Push Down Method or Field
- Windows下Git push or pull免输入密码设置
- zeromq push-pull 模式
- I2C1已经改成了push-pull,为什么ref sch上这组外部还需上拉?
- 关于git的pull与push
- adb pull and adb push
- git pull 与 git push
- git的操作(拉代码到本地/commit到本地/pull/push到远程/新建分支/合并分支/)
- Git关于pull,commit,push的总结
- SourceTree怎样在每次 Pull、Push 时不用重复输入密码?
- Open-Drain与Push-Pull
- Git远程操作详解(clone、remote、fetch、pull、push)
- Git push与pull的默认行为
- MQ PUSH/PULL