php实现图形计算器
2018-01-30 17:56
162 查看
存档:
index.php
form.class.php
shape.class.php
result.class.php
rect.class.php
triangle.class.php
circle.class.php
结果如下:
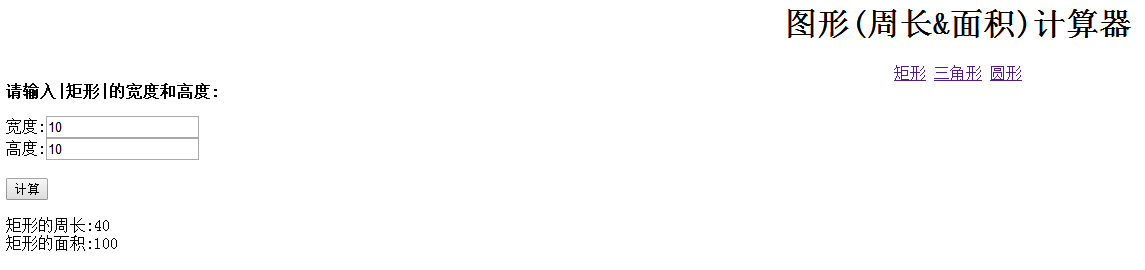
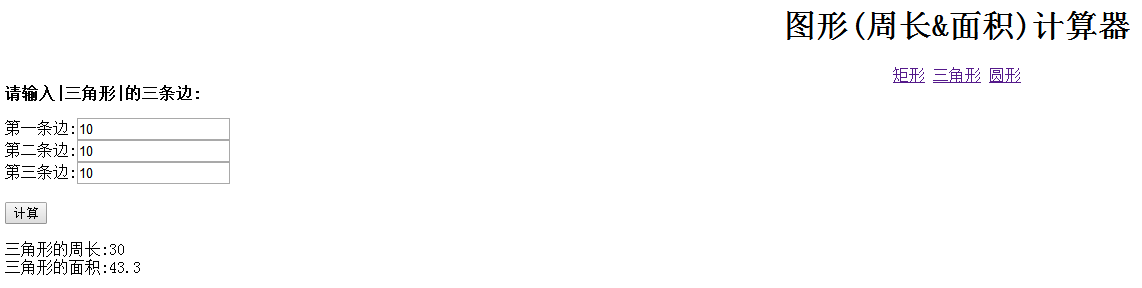
index.php
<html> <head> <title>图形计算器开发</title> <meta http-equiv="Content-type" content="text/html;charset=utf-8"> </head> <body> <center> <h1>图形(周长&面积)计算器</h1> <a href="index.php?action=rect">矩形</a> <a href="index.php?action=triangle">三角形</a> <a href="index.php?action=circle">圆形</a> </center> <?php error_reporting(E_ALL & ~E_NOTICE); function __autoload($className){ include strtolower($className).".class.php"; } echo new Form("index.php"); if(isset($_POST["sub"])){ echo new Result(); } ?> </body> </html>
form.class.php
<?php class Form{ private $action; private $shape; function __construct($action=""){ $this->action = $action; $this->shape = isset($_GET["action"])?$_GET["action"]:"rect"; } function __toString(){ $form='<form action="'.$this->action.'?action='.$this->shape.'" method="post">'; $shape="get".ucfirst($this->shape); $form .=$this->$shape(); $form .='<br><input type="submit" name="sub" value="计算"><br>'; $form .='</form>'; return $form; } private function getRect(){ $input = '<b>请输入|矩形|的宽度和高度:</b><p>'; $input .= '宽度:<input type="text" name="width" value="'.$_POST["width"].'"><br>'; $input .= '高度:<input type="text" name="height" value="'.$_POST["height"].'"><br>'; return $input; } private function getTriangle(){ $input = '<b>请输入|三角形|的三条边:</b><p>'; $input .= '第一条边:<input type="text" name="side1" value="'.$_POST["side1"].'"><br>'; $input .= '第二条边:<input type="text" name="side2" value="'.$_POST["side2"].'"><br>'; $input .= '第三条边:<input type="text" name="side3" value="'.$_POST["side3"].'"><br>'; return $input; } private function getCircle(){ $input = '<b>请输入|圆形|的半径:</b><p>'; $input .= '半径:<input type="text" name="radius" value="'.$_POST["radius"].'"><br>'; return $input; } } ?>
shape.class.php
<?php abstract class Shape{ public $shapeName; abstract function area(); abstract function perimeter(); protected function validate($value,$message = '输入的值'){ if($value=="" || !is_numeric($value) || $value<0){ $message=$this->shapeName.$message; echo '<font color="red">'.$message.'必须为非负值的数字,并且不能为空</font><br>'; return false; } else{ return true; } } } ?>
result.class.php
<?php class Result{ private $shape = null; function __construct(){ $this->shape = new $_GET['action'](); } function __toString(){ $result = $this->shape->shapeName.'的周长:'.round($this->shape->perimeter(),2).'<br>'; $result .= $this->shape->shapeName.'的面积:'.round($this->shape->area(),2).'<br>'; return $result; } } ?>
rect.class.php
<?php class Rect extends Shape{ private $width = 0; private $height = 0; function __construct(){ $this->shapeName = "矩形"; if($this->validate($_POST["width"],"宽度") & $this->validate($_POST["height"],"高度")){ $this->width = $_POST["width"]; $this->height = $_POST["height"]; } } function area(){ return $this->width*$this->height; } function perimeter(){ return 2*($this->width+$this->height); } } ?>
triangle.class.php
<?php class Triangle extends Shape{ private $side1 = 0; private $side2 = 0; private $side3 = 0; function __construct(){ $this->shapeName = "三角形"; if($this->validate($_POST["side1"],"第一条边") & $this->validate($_POST["side2"],"第二条边") & $this->validate($_POST["side3"],"第三条边")){ if($this->validateSum($_POST["side1"],$_POST["side2"],$_POST["side3"])){ $this->side1 = $_POST["side1"]; $this->side2 = $_POST["side2"]; $this->side3 = $_POST["side3"]; } else{ echo '<font color="red">三角形的两边之和要大于第三边</font><br>'; } } } function area(){ $s = ($this->side1+$this->side2+$this->side3)/2; return sqrt($s*($s-$this->side1)*($s-$this->side2)*($s-$this->side3)); } function perimeter(){ return $this->side1+$this->side2+$this->side3; } private function validateSum($s1,$s2,$s3){ if((($s1+$s2)>$s3) && (($s1+$s3)>$s2) && (($s2+$s3)>$s1)){ return true; } else{ return false; } } } ?>
circle.class.php
<?php class Circle extends Shape{ private $radius = 0; function __construct(){ $this->shapeName = "图形"; if($this->validate($_POST['radius'],'半径')){ $this->radius = $_POST['radius']; } } function area(){ return pi()*$this->radius*$this->radius; } function perimeter(){ return 2*pi()*$this->radius; } } ?>
结果如下:
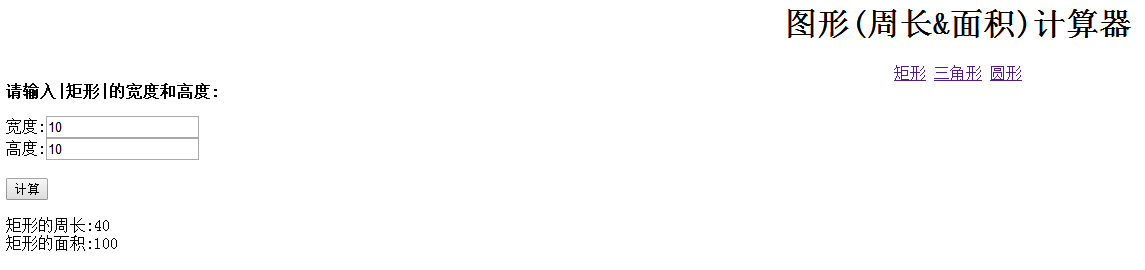
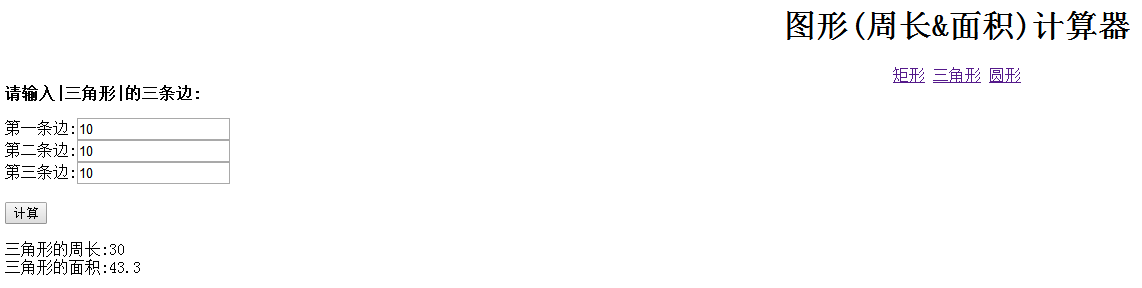
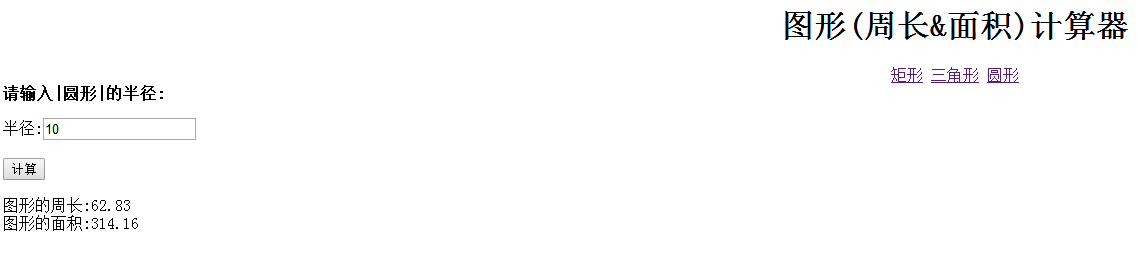
相关文章推荐
- PHP使用原生函数实现文件上传及下载
- php实现数组按指定KEY排序的方法
- jQuery+php+MySQL一张表实现三级联动菜单
- PHP实现简单顺序栈
- php学习之流程控制实现代码
- 关于PHP如何用实现防止用户在浏览器上使用后退功能重复提交输入
- PHP进制转换[实现2、8、16、36、64进制至10进制相互转换]
- round robin权重轮循算法php实现代码
- PHP+FFMPEG实现将视频自动转码成H264标准Mp4文件
- php图片缩放实现代码
- 用php实现Google /Baidu Ping服务快速收录
- PHP实现分页:文本分页和数字分页
- PHP内核探索 —— 常量的实现
- PHP实现的登录,注册及密码修改功能分析
- PHP AES 加密 解密 实现实例
- 用PHP实现的四则运算表达式计算
- php动态实现表格跨行跨列实现代码
- php源码之路第七章第二节 (语法的实现之语法解析)
- PHP 抽象类实现接口注意事项
- PHP缓存的实现