TensorFlow简单实例(三):nearest_neighbor
2017-12-12 11:17
357 查看
http://www.longxyun.com/blog.html [原文地址]
k-NN算法:有那么一堆你已经知道分类的数据,然后当一个新数据进入的时候,就开始跟训练数据里的每个点求距离,然后挑离这个训练数据最近的K个点看看这几个点属于什么类型,然后用少数服从多数的原则,给新数据归类。
k-NN算法步骤:
初始化距离为最大值
计算未知样本和每个训练样本的距离dist
得到目前K个最临近样本中的最大距离maxdist
如果dist小于maxdist,则将该训练样本作为K-最近邻样本
重复步骤2、3、4,直到未知样本和所有训练样本的距离都算完
统计K-最近邻样本中每个类标号出现的次数
选择出现频率最大的类标号作为未知样本的类标号
k-NN算法:有那么一堆你已经知道分类的数据,然后当一个新数据进入的时候,就开始跟训练数据里的每个点求距离,然后挑离这个训练数据最近的K个点看看这几个点属于什么类型,然后用少数服从多数的原则,给新数据归类。
k-NN算法步骤:
初始化距离为最大值
计算未知样本和每个训练样本的距离dist
得到目前K个最临近样本中的最大距离maxdist
如果dist小于maxdist,则将该训练样本作为K-最近邻样本
重复步骤2、3、4,直到未知样本和所有训练样本的距离都算完
统计K-最近邻样本中每个类标号出现的次数
选择出现频率最大的类标号作为未知样本的类标号
''' A nearest neighbor learning algorithm example using TensorFlow library. This example is using the MNIST database of handwritten digits (http://yann.lecun.com/exdb/mnist/) Author: Aymeric Damien Project: https://github.com/aymericdamien/TensorFlow-Examples/ ''' from __future__ import print_function import numpy as np import tensorflow as tf # Import MNIST data from tensorflow.examples.tutorials.mnist import input_data mnist = input_data.read_data_sets("/tmp/data/", one_hot=True) #这里主要是导入数据,数据通过input_data.py已经下载到/tmp/data/目录之下了,这里下载数据的时候,需要提前用浏览器尝试是否可以打开 #http://yann.lecun.com/exdb/mnist/,如果打不开,下载数据阶段会报错。而且一旦数据下载中断,需要将之前下载的未完成的数据清空,重新 #进行下载,否则会出现CRC Check错误。read_data_sets是input_data.py里面的一个函数,主要是将数据解压之后,放到对应的位置。 # In this example, we limit mnist data Xtr, Ytr = mnist.train.next_batch(5000) #5000 for training (nn candidates) Xte, Yte = mnist.test.next_batch(200) #200 for testing #mnist.train.next_batch,其中train和next_batch都是在input_data.py里定义好的数据项和函数。此处主要是取得一定数量的数据。 # tf Graph Input xtr = tf.placeholder("float", [None, 784]) xte = tf.placeholder("float", [784]) #设立两个空的类型,并没有给具体的数据。这也是为了基于这两个类型,去实现部分的graph。 # Nearest Neighbor calculation using L1 Distance # Calculate L1 Distance distance = tf.reduce_sum(tf.abs(tf.add(xtr, tf.negative(xte))), reduction_indices=1) # Prediction: Get min distance index (Nearest neighbor) pred = tf.arg_min(distance, 0) #最近邻居算法,算最近的距离的邻居,并且获取该邻居的下标,这里只是基于空的类型,实现的graph,并未进行真实的计算。 accuracy = 0. # Initializing the variables init = tf.global_variables_initializer() #初始化所有的变量和未分配数值的占位符,这个过程是所有程序中必须做的,否则可能会读出随机数值。 # Launch the graph with tf.Session() as sess: sess.run(init) # loop over test data for i in range(len(Xte)): # Get nearest neighbor nn_index = sess.run(pred, feed_dict={xtr: Xtr, xte: Xte[i, :]}) # Get nearest neighbor class label and compare it to its true label print("Test", i, "Prediction:", np.argmax(Ytr[nn_index]), \ "True Class:", np.argmax(Yte[i])) # Calculate accuracy if np.argmax(Ytr[nn_index]) == np.argmax(Yte[i]): accuracy += 1./len(Xte) print("Done!") print("Accuracy:", accuracy) #for循环迭代计算每一个测试数据的预测值,并且和真正的值进行对比,并计算精确度。该算法比较经典的是不需要提前训练,直接在测试阶段进行识别。
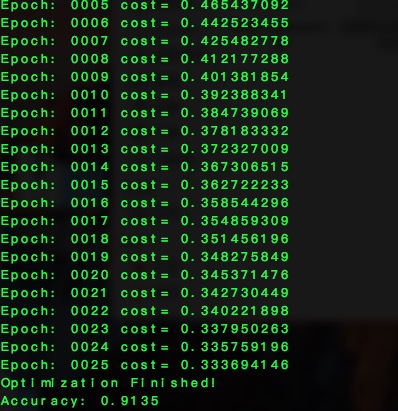
相关文章推荐
- tensorflow学习笔记四:mnist实例--用简单的神经网络来训练和测试
- TensorFlow简单实例(一):linear_regression
- Tensorflow学习笔记:模型训练数据的保存和恢复的简单实例
- Tensorflow笔记(四)——Nearest Neighbor Example
- TensorFlow学习笔记之源码分析----最近算法nearest_neighbor.py
- TensorFlow下进行MNIST手写数字识别实例,从最简单的两层到LeNet5
- Tensorflow的一个简单实例,线性回归
- 基于tensorflow的简单线性回归实例
- tensorflow 学习专栏(五):在mnist数据集上使用tensorflow实现临近算法(Nearest-Neighbor)进行手写数字识别
- TensorFlow学习笔记之源码分析(1)----最近算法nearest_neighbor
- Tensorflow实例:实现简单的卷积神经网络
- TensorFlow简单实例(二):logistic regression
- 基于tensorflow的神经网络简单实例
- 最近邻检索(Nearest Neighbor Search)的简单综述
- tensorflow学习笔记四:mnist实例--用简单的神经网络来训练和测试
- tensorflow-简单实例1
- TensorFlow----最近算法nearest_neighbor
- tensorflow 学习笔记(四) - mnist实例--用简单的神经网络来训练和测试
- tensorflow学习笔记四:mnist实例--用简单的神经网络来训练和测试(MNIST For ML Beginners)
- K最近邻结点算法(k-Nearest Neighbor algorithm)KNN——python简单实现