Leetcode 题解系列(十)
2017-12-05 00:59
337 查看
62. Unique Path
题目要求
A robot is located at the top-left corner of a m∗n grid (marked ‘Start’ in the diagram below).The robot can only move either down or right at any point in time. The robot is trying to reach the bottom-right corner of the grid (marked ‘Finish’ in the diagram below).
How many possible unique paths are there?
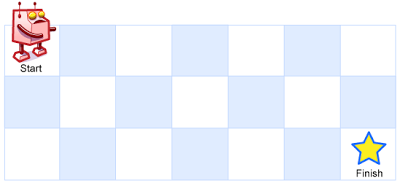
Above is a 3 x 7 grid. How many possible unique paths are there?
Note: m and n will be at most 100.
题目分析
简析这道题使用动态规划即可求解,其中到达任意一点的路径是这一点上方的一点以及右边一点的路径之和。其状态转换方程为:
dp(i,j)={1,dp(i−1,j)+dp(i,j−1),i = 0 or j = 0i > 0 and j > 0
其中dp(i,j)为到达点(i,j)的唯一路径,dp(m−1,n−1)即为所求。时间复杂度为O(m∗n),空间复杂度为O(m∗n)
写成代码有
class Solution { public: int uniquePaths(int m, int n) { int dp[101][101] = {}; for (auto &i : dp) i[0] = 1; for (auto &i : dp[0]) i = 1; for (int i = 1; i < m; ++i) { for (int j = 1; j < n; ++j) { dp[i][j] = dp[i - 1][j] + dp[i][j - 1]; } } return dp[m - 1][n - 1]; } };
简化
由于计算第
i行的时候,只需要第
i - 1的值,所以dp的数组可以简化为一维数组,空间复杂度变为O(m)
class Solution { public: int uniquePaths(int m, int n) { int dp[101] = {}; for (auto &i : dp) i = 1; for (int i = 1; i < n; ++i) { for (int j = 1; j < m; ++j) { dp[j] += dp[j - 1]; } } return dp[m - 1]; } };
63. Unique Path II
题目要求
Follow up for “Unique Paths”:Now consider if some obstacles are added to the grids. How many unique paths would there be?
An obstacle and empty space is marked as 1 and 0 respectively in the grid.
For example,
There is one obstacle in the middle of a 3x3 grid as illustrated below.
[ [0,0,0], [0,1,0], [0,0,0] ]
The total number of unique paths is
2.
Note: m and n will be at most 100.
题目分析
此题与上题类似,但有所不同的是多了有障碍物的条件。如果一个格子有障碍物,那么通向那个格子的路径数量为0。转移方程为:
dp(i,j)=⎧⎩⎨⎪⎪⎪⎪⎪⎪0,dp(i,j−1),dp(i−1,j),dp(i−1,j)+dp(i,j−1)obstacleGrid[i][j]=0i=0j=0
时间复杂度为O(m∗n),空间复杂度为O(m∗n),借用上题的优化方法,空间复杂度可以变为O(m)。代码如下:
class Solution { public: int uniquePathsWithObstacles(vector<vector<int>>& obstacleGrid) { int dp[101] = {}; const int m = obstacleGrid[0].size(), n = obstacleGrid.size(); for (int i = 0; i < m; ++i) { if (obstacleGrid[0][i] == 1) break; dp[i] = 1; } for (int i = 1; i < n; ++i) { dp[0] = obstacleGrid[i][0] == 1 ? 0 : dp[0]; for (int j = 1; j < m; ++j) { dp[j] = obstacleGrid[i][j] == 0 ? dp[j] + dp[j - 1] : 0; } } return dp[m - 1]; } };
相关文章推荐
- Leetcode 题解系列(三)
- LeetCode题解系列--684. Redundant Connection
- Leetcode 题解系列(十六)
- LeetCode题解系列--1. Two Sum
- Leetcode 题解系列(十一)
- Leetcode 题解系列(十七)
- LeetCode题解系列--685. Redundant Connection II
- Leetcode 题解系列(十二)
- LeetCode题解系列--5. Longest Palindromic Substring
- Leetcode 题解系列(四)
- Leetcode 题解系列(六)
- Leetcode 题解系列(十四)
- LeetCode题解系列--123. Best Time to Buy and Sell Stock III
- LeetCode题解系列--763. Partition Labels
- LeetCode题解系列--712. Minimum ASCII Delete Sum for Two Strings
- Leetcode 系列题解(十八)
- Leetcode 题解系列(七)
- LeetCode题解系列--718. Maximum Length of Repeated Subarray
- LeetCode题解系列--215. Kth Largest Element in an Array
- Leetcode 题解系列(十三)