python一行代码实现人脸识别
2017-11-08 10:21
1131 查看
我是在鸟哥私房菜的网站中看到这篇文章,觉得很有趣,所以和大家一起分享:
http://www.linuxidc.com/Linux/2017-10/148126.htm
实现人脸识别之前,我们首先要搭建环境:
1.安装Ubuntu17.10 ,因为我的电脑上本来有了Ubuntu,和这个版本不同,后面搭建环境的时候出现了一些问题
2.安装Python2.7.14(如果安装了Ubuntu17.10,就会有这个Python版本)
3.安装git,cmake,python-pip
(1)安装git的命令
sudo apt-get install -y git
(2)安装cmake的命令
sudo apt-get install -y cmake
(3)安装python-pip
sudo apt-get install -y python-pip
4.安装编译dlib
安装face_recongnition这个之前需要先安装编译dlib
编译dlib前先安装boost
sudo apt-get install libboost-all-dev
开始编译dlib,克隆dlib源代码
git clone https://github.com/davisking/dlib.git
cd dlib
mkdir build
cd build
cmake .. -DDLIB_USE_CUDA=0 -DUSE_AVX_INSTRUCTIONS=1
cmake --build .
cd ..
python setup.py install --yes USE_AVX_INSTRUCTIONS --no DLIB_USE_CUDA
5.安装face_recognition
pip install face_recongnition
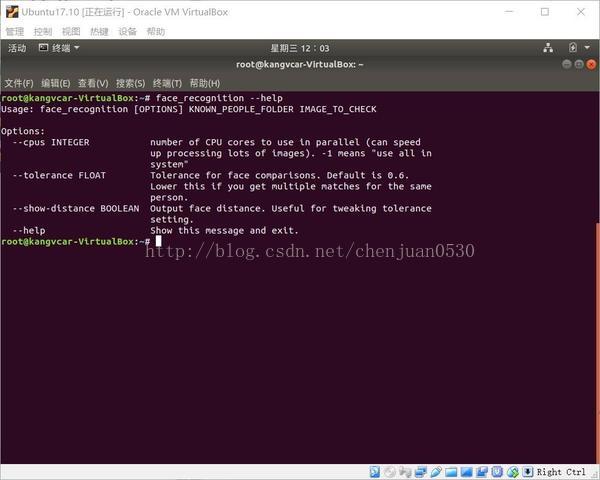
查看环境是否搭建完成,在终端输入face_recognition命令查看是否成功
实现人脸识别
实例1:
1、 首先你需要提供一个文件夹,里面是所有你希望系统认识的人的图片。其中每个人一张图片,图片以人的名字命名:
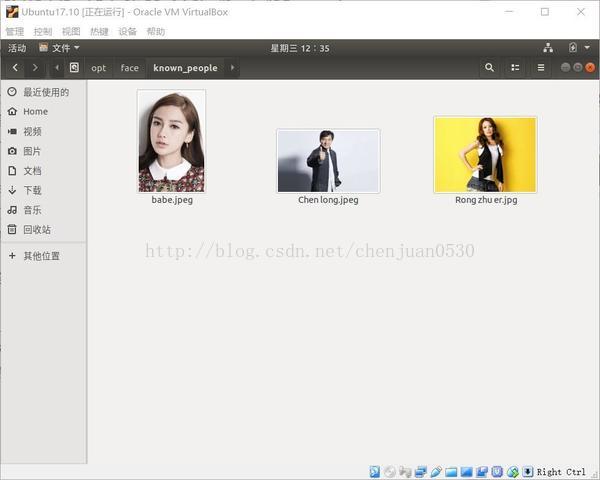
known_people 文件夹下有 babe、成龙、容祖儿的照片
2、 接下来,你需要准备另一个文件夹,里面是你要识别的图片:
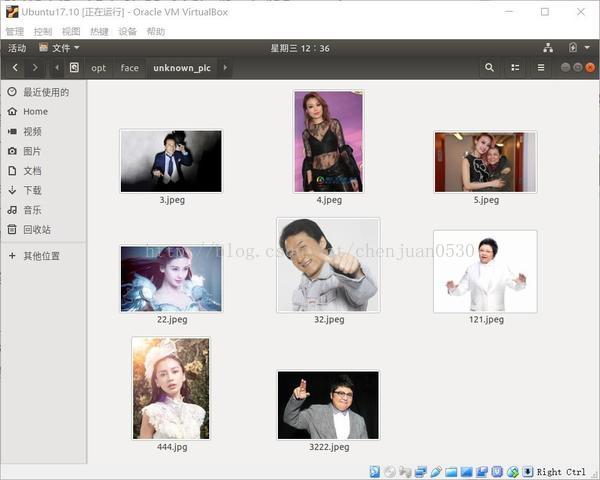
unknown_pic 文件夹下是要识别的图片,其中韩红是机器不认识的
3、 然后你就可以运行 face_recognition 命令了,把刚刚准备的两个文件夹作为参数传入,命令就会返回需要识别的图片中都出现了谁:
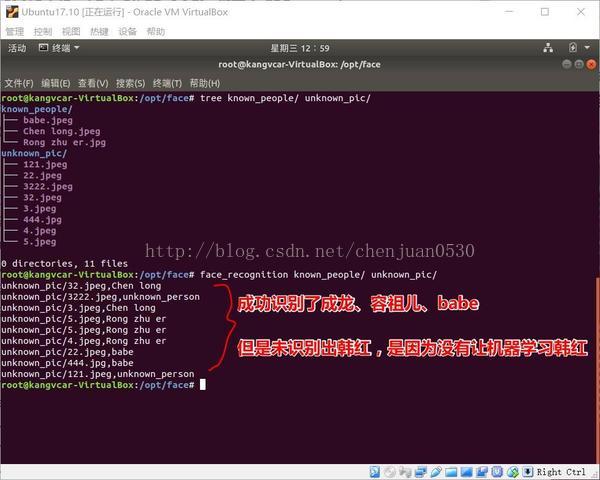
识别成功!
实例2:
识别一张图像上有多张人脸的图片,并将识别到的图像打印出来
新建一个*.py的文件,在文件中编写代码:# filename : find_faces_in_picture.py
# -*- coding: utf-8 -*-
# 导入pil模块 ,可用命令安装 apt-get install python-Imaging
from PIL import Image
# 导入face_recogntion模块,可用命令安装 pip install face_recognition
import face_recognition
# 将jpg文件加载到numpy 数组中
image = face_recognition.load_image_file("/opt/face/unknown_pic/all_star.jpg")
# 使用默认的给予HOG模型查找图像中所有人脸
# 这个方法已经相当准确了,但还是不如CNN模型那么准确,因为没有使用GPU加速
# 另请参见: find_faces_in_picture_cnn.py
face_locations = face_recognition.face_locations(image)
# 使用CNN模型
# face_locations = face_recognition.face_locations(image, number_of_times_to_upsample=0, model="cnn")
# 打印:我从图片中找到了 多少 张人脸
print("I found {} face(s) in this photograph.".format(len(face_locations)))
# 循环找到的所有人脸
for face_location in face_locations:
# 打印每张脸的位置信息
top, right, bottom, left = face_location
print("A face is located at pixel location Top: {}, Left: {}, Bottom: {}, Right: {}".format(top, left, bottom, right))
# 指定人脸的位置信息,然后显示人脸图片
face_image = image[top:bottom, left:right]
pil_image = Image.fromarray(face_image)
pil_image.show()
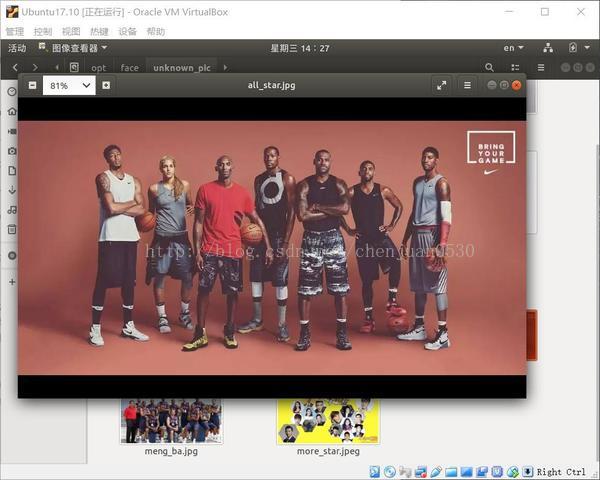
用于识别的图片,执行python文件得到的结果为:
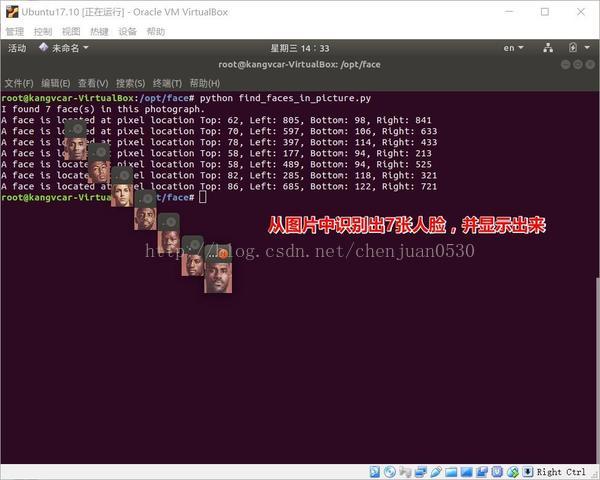
实例三:
# filename : find_facial_features_in_picture.py
# -*- coding: utf-8 -*-
# 导入pil模块 ,可用命令安装 apt-get install python-Imaging
from PIL import Image, ImageDraw
# 导入face_recogntion模块,可用命令安装 pip install face_recognition
import face_recognition
# 将jpg文件加载到numpy 数组中
image = face_recognition.load_image_file("biden.jpg")
#查找图像中所有面部的所有面部特征
face_landmarks_list = face_recognition.face_landmarks(image)
print("I found {} face(s) in this photograph.".format(len(face_landmarks_list)))
for face_landmarks in face_landmarks_list:
#打印此图像中每个面部特征的位置
facial_features = [
'chin',
'left_eyebrow',
'right_eyebrow',
'nose_bridge',
'nose_tip',
'left_eye',
'right_eye',
'top_lip',
'bottom_lip'
]
for facial_feature in facial_features:
print("The {} in this face has the following points: {}".format(facial_feature, face_landmarks[facial_feature]))
#让我们在图像中描绘出每个人脸特征!
pil_image = Image.fromarray(image)
d = ImageDraw.Draw(pil_image)
for facial_feature in facial_features:
d.line(face_landmarks[facial_feature], width=5)
pil_image.show()
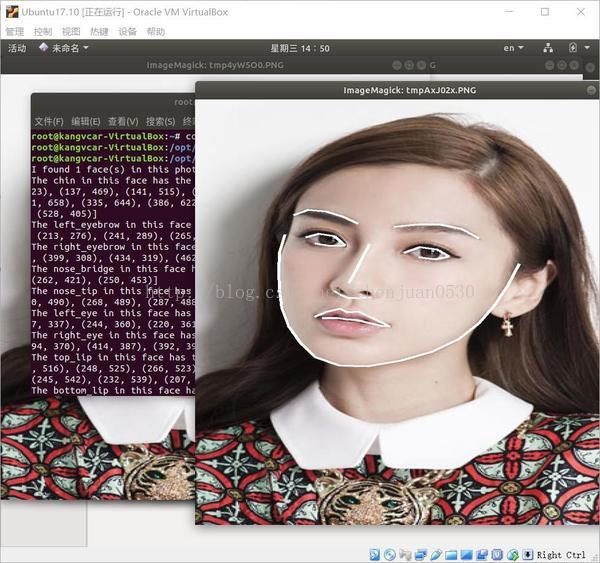
自动识别出人脸特征
实例四(通过多张图片对比,识别出是哪一个人):
# filename : recognize_faces_in_pictures.py
# -*- conding: utf-8 -*-
# 导入face_recogntion模块,可用命令安装 pip install face_recognition
import face_recognition
#将jpg文件加载到numpy数组中
babe_image = face_recognition.load_image_file("/opt/face/known_people/babe.jpeg")
Rong_zhu_er_image = face_recognition.load_image_file("/opt/face/known_people/Rong zhu er.jpg")
unknown_image = face_recognition.load_image_file("/opt/face/unknown_pic/babe2.jpg")
#获取每个图像文件中每个面部的面部编码
#由于每个图像中可能有多个面,所以返回一个编码列表。
#但是由于我知道每个图像只有一个脸,我只关心每个图像中的第一个编码,所以我取索引0。
babe_face_encoding = face_recognition.face_encodings(babe_image)[0]
Rong_zhu_er_face_encoding = face_recognition.face_encodings(Rong_zhu_er_image)[0]
unknown_face_encoding = face_recognition.face_encodings(unknown_image)[0]
known_faces = [
babe_face_encoding,
Rong_zhu_er_face_encoding
]
#结果是True/false的数组,未知面孔known_faces阵列中的任何人相匹配的结果
results = face_recognition.compare_faces(known_faces, unknown_face_encoding)
print("这个未知面孔是 Babe 吗? {}".format(results[0]))
print("这个未知面孔是 容祖儿 吗? {}".format(results[1]))
print("这个未知面孔是 我们从未见过的新面孔吗? {}".format(not True in results))
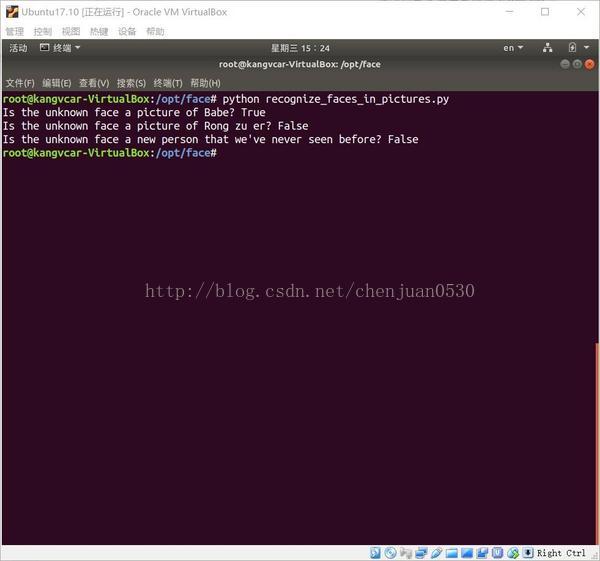
为true代表是相符的
实例五(识别人脸特征并美颜):
# filename : digital_makeup.py
#-*- coding: utf-8-*-
#导入pil模块,可用命令安装apt-get install python-Imaging
from PIL importImage,ImageDraw
#导入face_recogntion模块,可用命令安装 pip install face_recognition
import face_recognition
#将jpg文件加载到numpy数组中
image = face_recognition.load_image_file("biden.jpg")
#查找图像中所有面部的所有面部特征
face_landmarks_list = face_recognition.face_landmarks(image)
for face_landmarks in face_landmarks_list:
pil_image =Image.fromarray(image)
d =ImageDraw.Draw(pil_image,'RGBA')
#让眉毛变成了一场噩梦
d.polygon(face_landmarks['left_eyebrow'], fill=(68,54,39,128))
d.polygon(face_landmarks['right_eyebrow'], fill=(68,54,39,128))
d.line(face_landmarks['left_eyebrow'], fill=(68,54,39,150), width=5)
d.line(face_landmarks['right_eyebrow'], fill=(68,54,39,150), width=5)
#光泽的嘴唇
d.polygon(face_landmarks['top_lip'], fill=(150,0,0,128))
d.polygon(face_landmarks['bottom_lip'], fill=(150,0,0,128))
d.line(face_landmarks['top_lip'], fill=(150,0,0,64), width=8)
d.line(face_landmarks['bottom_lip'], fill=(150,0,0,64), width=8)
#闪耀眼睛
d.polygon(face_landmarks['left_eye'], fill=(255,255,255,30))
d.polygon(face_landmarks['right_eye'], fill=(255,255,255,30))
#涂一些眼线
d.line(face_landmarks['left_eye']+[face_landmarks['left_eye'][0]], fill=(0,0,0,110), width=6)
d.line(face_landmarks['right_eye']+[face_landmarks['right_eye'][0]], fill=(0,0,0,110), width=6)
pil_image.show()
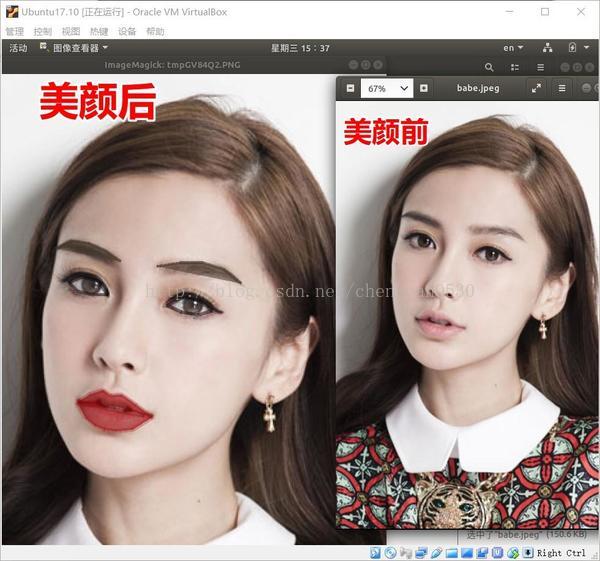
美颜前后的对比图
这个技术可以用到很多开发上面,所以还是有必要学习一下
http://www.linuxidc.com/Linux/2017-10/148126.htm
实现人脸识别之前,我们首先要搭建环境:
1.安装Ubuntu17.10 ,因为我的电脑上本来有了Ubuntu,和这个版本不同,后面搭建环境的时候出现了一些问题
2.安装Python2.7.14(如果安装了Ubuntu17.10,就会有这个Python版本)
3.安装git,cmake,python-pip
(1)安装git的命令
sudo apt-get install -y git
(2)安装cmake的命令
sudo apt-get install -y cmake
(3)安装python-pip
sudo apt-get install -y python-pip
4.安装编译dlib
安装face_recongnition这个之前需要先安装编译dlib
编译dlib前先安装boost
sudo apt-get install libboost-all-dev
开始编译dlib,克隆dlib源代码
git clone https://github.com/davisking/dlib.git
cd dlib
mkdir build
cd build
cmake .. -DDLIB_USE_CUDA=0 -DUSE_AVX_INSTRUCTIONS=1
cmake --build .
cd ..
python setup.py install --yes USE_AVX_INSTRUCTIONS --no DLIB_USE_CUDA
5.安装face_recognition
pip install face_recongnition
查看环境是否搭建完成,在终端输入face_recognition命令查看是否成功
实现人脸识别
实例1:
1、 首先你需要提供一个文件夹,里面是所有你希望系统认识的人的图片。其中每个人一张图片,图片以人的名字命名:
known_people 文件夹下有 babe、成龙、容祖儿的照片
2、 接下来,你需要准备另一个文件夹,里面是你要识别的图片:
unknown_pic 文件夹下是要识别的图片,其中韩红是机器不认识的
3、 然后你就可以运行 face_recognition 命令了,把刚刚准备的两个文件夹作为参数传入,命令就会返回需要识别的图片中都出现了谁:
识别成功!
实例2:
识别一张图像上有多张人脸的图片,并将识别到的图像打印出来
新建一个*.py的文件,在文件中编写代码:# filename : find_faces_in_picture.py
# -*- coding: utf-8 -*-
# 导入pil模块 ,可用命令安装 apt-get install python-Imaging
from PIL import Image
# 导入face_recogntion模块,可用命令安装 pip install face_recognition
import face_recognition
# 将jpg文件加载到numpy 数组中
image = face_recognition.load_image_file("/opt/face/unknown_pic/all_star.jpg")
# 使用默认的给予HOG模型查找图像中所有人脸
# 这个方法已经相当准确了,但还是不如CNN模型那么准确,因为没有使用GPU加速
# 另请参见: find_faces_in_picture_cnn.py
face_locations = face_recognition.face_locations(image)
# 使用CNN模型
# face_locations = face_recognition.face_locations(image, number_of_times_to_upsample=0, model="cnn")
# 打印:我从图片中找到了 多少 张人脸
print("I found {} face(s) in this photograph.".format(len(face_locations)))
# 循环找到的所有人脸
for face_location in face_locations:
# 打印每张脸的位置信息
top, right, bottom, left = face_location
print("A face is located at pixel location Top: {}, Left: {}, Bottom: {}, Right: {}".format(top, left, bottom, right))
# 指定人脸的位置信息,然后显示人脸图片
face_image = image[top:bottom, left:right]
pil_image = Image.fromarray(face_image)
pil_image.show()
用于识别的图片,执行python文件得到的结果为:
实例三:
# filename : find_facial_features_in_picture.py
# -*- coding: utf-8 -*-
# 导入pil模块 ,可用命令安装 apt-get install python-Imaging
from PIL import Image, ImageDraw
# 导入face_recogntion模块,可用命令安装 pip install face_recognition
import face_recognition
# 将jpg文件加载到numpy 数组中
image = face_recognition.load_image_file("biden.jpg")
#查找图像中所有面部的所有面部特征
face_landmarks_list = face_recognition.face_landmarks(image)
print("I found {} face(s) in this photograph.".format(len(face_landmarks_list)))
for face_landmarks in face_landmarks_list:
#打印此图像中每个面部特征的位置
facial_features = [
'chin',
'left_eyebrow',
'right_eyebrow',
'nose_bridge',
'nose_tip',
'left_eye',
'right_eye',
'top_lip',
'bottom_lip'
]
for facial_feature in facial_features:
print("The {} in this face has the following points: {}".format(facial_feature, face_landmarks[facial_feature]))
#让我们在图像中描绘出每个人脸特征!
pil_image = Image.fromarray(image)
d = ImageDraw.Draw(pil_image)
for facial_feature in facial_features:
d.line(face_landmarks[facial_feature], width=5)
pil_image.show()
自动识别出人脸特征
实例四(通过多张图片对比,识别出是哪一个人):
# filename : recognize_faces_in_pictures.py
# -*- conding: utf-8 -*-
# 导入face_recogntion模块,可用命令安装 pip install face_recognition
import face_recognition
#将jpg文件加载到numpy数组中
babe_image = face_recognition.load_image_file("/opt/face/known_people/babe.jpeg")
Rong_zhu_er_image = face_recognition.load_image_file("/opt/face/known_people/Rong zhu er.jpg")
unknown_image = face_recognition.load_image_file("/opt/face/unknown_pic/babe2.jpg")
#获取每个图像文件中每个面部的面部编码
#由于每个图像中可能有多个面,所以返回一个编码列表。
#但是由于我知道每个图像只有一个脸,我只关心每个图像中的第一个编码,所以我取索引0。
babe_face_encoding = face_recognition.face_encodings(babe_image)[0]
Rong_zhu_er_face_encoding = face_recognition.face_encodings(Rong_zhu_er_image)[0]
unknown_face_encoding = face_recognition.face_encodings(unknown_image)[0]
known_faces = [
babe_face_encoding,
Rong_zhu_er_face_encoding
]
#结果是True/false的数组,未知面孔known_faces阵列中的任何人相匹配的结果
results = face_recognition.compare_faces(known_faces, unknown_face_encoding)
print("这个未知面孔是 Babe 吗? {}".format(results[0]))
print("这个未知面孔是 容祖儿 吗? {}".format(results[1]))
print("这个未知面孔是 我们从未见过的新面孔吗? {}".format(not True in results))
为true代表是相符的
实例五(识别人脸特征并美颜):
# filename : digital_makeup.py
#-*- coding: utf-8-*-
#导入pil模块,可用命令安装apt-get install python-Imaging
from PIL importImage,ImageDraw
#导入face_recogntion模块,可用命令安装 pip install face_recognition
import face_recognition
#将jpg文件加载到numpy数组中
image = face_recognition.load_image_file("biden.jpg")
#查找图像中所有面部的所有面部特征
face_landmarks_list = face_recognition.face_landmarks(image)
for face_landmarks in face_landmarks_list:
pil_image =Image.fromarray(image)
d =ImageDraw.Draw(pil_image,'RGBA')
#让眉毛变成了一场噩梦
d.polygon(face_landmarks['left_eyebrow'], fill=(68,54,39,128))
d.polygon(face_landmarks['right_eyebrow'], fill=(68,54,39,128))
d.line(face_landmarks['left_eyebrow'], fill=(68,54,39,150), width=5)
d.line(face_landmarks['right_eyebrow'], fill=(68,54,39,150), width=5)
#光泽的嘴唇
d.polygon(face_landmarks['top_lip'], fill=(150,0,0,128))
d.polygon(face_landmarks['bottom_lip'], fill=(150,0,0,128))
d.line(face_landmarks['top_lip'], fill=(150,0,0,64), width=8)
d.line(face_landmarks['bottom_lip'], fill=(150,0,0,64), width=8)
#闪耀眼睛
d.polygon(face_landmarks['left_eye'], fill=(255,255,255,30))
d.polygon(face_landmarks['right_eye'], fill=(255,255,255,30))
#涂一些眼线
d.line(face_landmarks['left_eye']+[face_landmarks['left_eye'][0]], fill=(0,0,0,110), width=6)
d.line(face_landmarks['right_eye']+[face_landmarks['right_eye'][0]], fill=(0,0,0,110), width=6)
pil_image.show()
美颜前后的对比图
这个技术可以用到很多开发上面,所以还是有必要学习一下
相关文章推荐
- 手把手教你用1行代码实现人脸识别 -- Python Face_recognition
- Python最短代码实现人脸识别,打造自己专用人脸识别!
- 手把手教你用1行代码实现人脸识别 -- Python Face_recognition
- Python 40行代码实现人脸识别功能
- python+opencv实现的简单人脸识别代码示例
- 手把手教你用1行代码实现人脸识别 -- Python Face_recognition
- 手把手教你用1行代码实现人脸识别 --Python Face_recognition
- 手把手教你用1行代码实现人脸识别 -- Python Face_recognition
- 手把手教你用1行代码实现人脸识别 -- Python Face_recognition
- 手把手教你用1行代码实现人脸识别 -- Python Face_recognition
- python实现人脸识别代码
- 25 行 Python 代码实现人脸识别——OpenCV
- 人脸检测及识别python实现系列(2)——识别出人脸
- 【人脸识别】人脸验证算法Joint Bayesian详解及实现(Python版)
- Python调用OpenCV实现人脸识别
- DeepLearning tutorial(5)CNN卷积神经网络应用于人脸识别(详细流程+代码实现)
- Python 一行代码实现并行
- 教你用一行Python代码实现并行(转)
- 人脸检测和识别及python实现系列(1)-- 环境配置和相关类库安装
- 用40行Python代码 实践高大上的人脸识别