api 支付宝接口 支付接口 退款接口
2017-11-01 10:09
477 查看
首先在支付宝开放平台上新建一个应用 具体操作请上 https://open.alipay.com/developmentAccess/developmentAccess.htm
从支付宝官网上下载支付宝服务端支付接口 地址:https://docs.open.alipay.com/54/103419/
我用的框架是TP5 把sdk 放在了 vendor 文件下 这个sdk 包文件 你们可以放在自己引用第三方文件的文件夹下
下面来看具体操作
在此之前 我们需要知道 appid 支付宝公钥 应用私钥 应用私钥生成方式在 https://docs.open.alipay.com/291/106097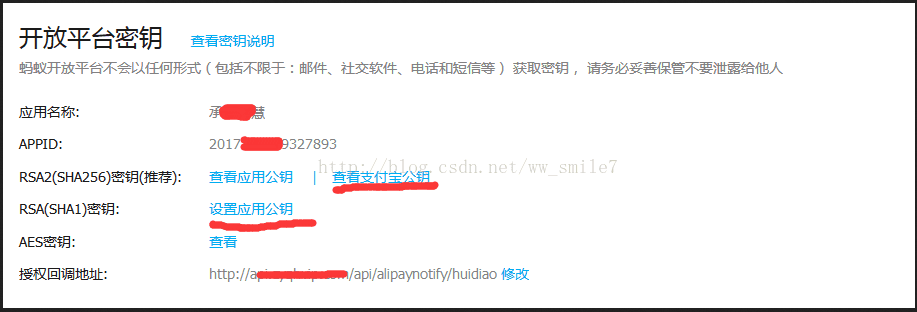
开始代码部分
从支付宝官网上下载支付宝服务端支付接口 地址:https://docs.open.alipay.com/54/103419/
我用的框架是TP5 把sdk 放在了 vendor 文件下 这个sdk 包文件 你们可以放在自己引用第三方文件的文件夹下
下面来看具体操作
在此之前 我们需要知道 appid 支付宝公钥 应用私钥 应用私钥生成方式在 https://docs.open.alipay.com/291/106097
开始代码部分
<?php namespace app\api\controller; use think\Controller; use think\Request; use think\Db; use think\cache\driver\Redis; // 本类由系统自动生成,仅供测试用途 class Alipay extends Sms{ // 支付宝 appid public $appid = ''; //支付宝公钥 public $alipayrsaPublicKey= ''; //支付宝私钥 public $rsaPrivateKey = ''; //支付宝同步通知 public function index(){ vendor('alipay.aop.AopClient'); vendor('alipay.aop.request.AlipayTradeAppPayRequest'); $aop = new \AopClient(); //异步回调地址 $aop->gatewayUrl = "https://openapi.alipay.com/gateway.do"; //appid $aop->appId = $this -> appid; //本地生成的私钥 $aop->rsaPrivateKey = $this -> rsaPrivateKey; $aop->format = "json"; $aop->charset = "UTF-8"; $aop->signType = "RSA2"; //支付宝公钥 $aop->alipayrsaPublicKey = $this -> alipayrsaPublicKey; $request = new \AlipayTradeAppPayRequest(); $order_id = input('post.order_sn'); //根据 order_id 可以知道 订单的业务参数 如:订单总金额 //订单总金额 $amount_payable=12; $bizcontent = "{\"body\":\"App支付\","//订单描述 . "\"subject\": \"购买的商品\","//订单标题 . "\"out_trade_no\": \"{$order_id}\","//商户网站唯一订单号 . "\"timeout_express\": \"30m\"," . "\"total_amount\": \"{$amount_payable}\","//订单总金额 . "\"product_code\":\"QUICK_MSECURITY_PAY\""//固定值 . "}"; //异步回调 $request->setNotifyUrl("http://api.***.com/api/alipaynotify/huidiao"); $request->setBizContent($bizcontent); $response = $aop->sdkExecute($request); //给客户端返回的 签名 和 一些订单信息 die( json_encode( array('status'=>strval( 1 ),'msg'=>'支付成功', 'data'=>$response)) ); } //支付宝回调地址 public function huidiao(){ vendor('alipay.aop.AopClient'); $aop = new \AopClient(); $aop->alipayrsaPublicKey = $this -> alipayrsaPublicKey; $flag = $aop->rsaCheckV1($_POST,NULL,"RSA2"); if($flag == true){ //进行业务参数预算 用$_post[''] 具体参数可访问支付宝客户端 https://docs.open.alipay.com/204/105301/ //原支付请求的商户订单号 只需要这一个就可以了 就可 查询订单表 echo 'success';exit; }else{ echo 'false'; } } /** 支付宝退款*/ public function tuikuan() { vendor('alipay.aop.AopClient'); vendor('alipay.aop.request.AlipayTradeRefundRequest'); if (request()->isPost()) { $token = input('post.token'); $redis = new \Redis; $redis->connect('127.0.0.1', 6379); $id = $redis->get($token); $uid = $id; if (!empty($uid)) { //一下全都是一些业务运算 可忽略 //订单号 $order_ns = input('post.order_sn'); //退款原因 $reason = input('post.reason'); //订单商品id $product_id = input('post.product_id'); if (!empty($order_ns) && !empty($reason)) { $str = db("order_info") ->join("tp_order_detail", "tp_order_info.order_info_id=tp_order_detail.order_info_id") ->where("tp_order_info.oid", $order_ns) ->where("tp_order_detail.goods_id", $product_id) ->find(); //退款金额 不能小于 0 $details = $str['sum_price']; if ($details >= 0) { return json(array( 'status' => -1, 'msg' => "退款失败1", 'data' => "", )); } else { // 退款 从这里正是开始 真正需要的值 是 “订单号 ” 之前支付时 使用的订单号 “退款金额 ” 不可超过支付过的金额 “退款原因” $aop = new \AopClient(); $aop->gatewayUrl = 'https://openapi.alipay.com/gateway.do'; $aop->appId = $this -> appid; $aop->rsaPrivateKey = $this -> rsaPrivateKey; $aop->alipayrsaPublicKey= $this -> alipayrsaPublicKey; $aop->apiVersion = '1.0'; $aop->signType = 'RSA2'; $aop->postCharset='UTF-8'; $aop->format='json'; $request = new \AlipayTradeRefundRequest(); $request->setBizContent("{" . "\"out_trade_no\":\"{$order_ns}\"," . "\"trade_no\":\"\"," . "\"refund_amount\":{$details}," . "\"refund_reason\":\"{$reason}\"," . "\"out_request_no\":\"HZ01RF001\"," . "\"operator_id\":\"OP001\"," . "\"store_id\":\"NJ_S_001\"," . "\"terminal_id\":\"NJ_T_001\"" . " }"); $result = $aop->execute($request); // print_r($result);die; $responseNode = str_replace(".", "_", $request->getApiMethodName()) . "_response"; $resultCode = $result->$responseNode->code; if(!empty($resultCode)&&$resultCode == 10000){ //退款成功 可以在这里进行 修改 状态 等等一系列操作 return json(array( 'status' => 1, 'msg' => "退款成功", 'data' => "", )); }else { return json(array( 'status' => -1, 'msg' => "退款失败", 'data' => "", )); } } } } } } }注意啊 回调地址 不能有任何的参数传递 !!!
相关文章推荐
- php调用支付宝PHP接口API实现在线即时支付功能
- Android IOS手机客户端支付接口API(支付宝)
- 支付宝支付-常用支付API详解(查询、退款、提现等)(转)
- 支付宝免签约 免手续费 支付接口API
- 调用支付宝PHP接口API实现在线即时支付功能(UTF-8编码)
- 支付宝支付-常用支付API详解(查询、退款、提现等)
- 调用支付宝PHP接口API实现在线即时支付功能(UTF-8编码)
- 微信沙箱退款查询接口demo实例,微信公众号支付退款查询api,微信沙盒1004用例-公众号/APP/扫码支付退款查询——微信支付沙箱开发11
- 支付宝微信免签约 免手续费 支付接口API
- C#版支付宝手机网站支付接口API之
- 支付宝支付-常用支付API详解(查询、退款、提现等)
- APP中微信,支付宝支付,退款接口从申请到开发过程
- 支付宝支付-常用支付API详解(查询、退款、提现等)-转
- 支付宝免签约 免手续费 支付接口API
- [接口]支付宝接口开发集成支付环境开发总结
- 支付宝退款接口(即时到帐有密退款)
- 支付宝老版本支付接口无法生成签名问题
- .NET C#支付宝条码支付接口详解
- 支付宝支付-手机网站支付接口(H5手机站)
- Spring boot--支付宝APP支付安卓老版接口实现(含代码)