[js高手之路]设计模式系列课程-设计一个模块化扩展功能(define)和使用(use)库
2017-09-06 10:05
791 查看
模块化的诞生标志着javascript开发进入工业时代,近几年随着es6, require js( sea js ), node js崛起,特别是es6和node js自带模块加载功能,给大型程序开发带来了极大的便利。这几个东西没有出来之前,最原始的开发全部是利用全局函数进行封装,如:
这种开发方式,非常容易造成一个问题,全局变量污染(覆盖)
如:有个页面需要引入3个js文件( a.js, b.js, c.js ), A程序员在a.js文件中定义了一个函数叫show, B程序员在b.js中也定义了一个函数叫show, 那么引入同一个页面之后,后面的函数会把前面的覆盖,那C程序员引入之后,本来想调用A程序员的show方法,结果被B程序员写的文件给覆盖了,这就很恐怖了,如果这是一个公共方法(核心方法),可能造成系统完全挂掉,那怎么解决呢?早期程序员一般用命名空间,如:
这样就有可能大大降低重名的可能性,但是还是会有小部分覆盖的可能性,当然可以在程序中判断,如果存在这个命名空间,就不让定义,但是这样子写,就没有封装性可言,只要引入了js文件,谁都能够操作这些命名空间,也很危险,对于公共接口( 比如获取数据,可以暴露给外部使用),对于一些核心,比较重要的,就要封装起来,不要暴露给外部使用,所以可以通过闭包( 立即表达式)把命名空间中的方法选择性的暴露给外部
改进之后,封装性和团队协作开发又得到了进一步的提高,但是依然存在另一个问题,后来的开发者如果要为这个框架新增一个功能模块,而且也能选择性的暴露一些接口怎么办呢?
我们可以封装一个模块扩展功能和模块使用功能,以后要做扩展就非常方便了.
我们封装了一个define函数,这个函数用来给G模块扩展功能,这里我扩展了一个字符串处理对象,包含了3个方法: trim, trimLeft, trimRight
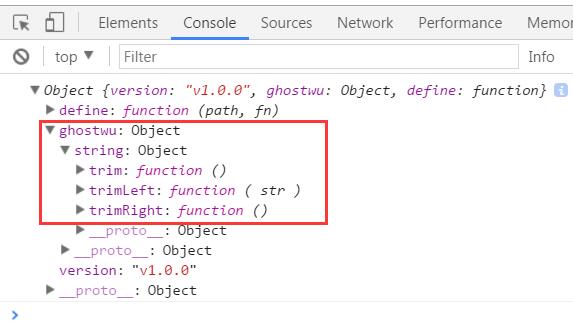
使用的时候,按照命名空间的方式使用即可,我们也可以加一个使用模块的方法use
1 function checkEmail(){} 2 function checkName(){} 3 function checkPwd(){}
这种开发方式,非常容易造成一个问题,全局变量污染(覆盖)
如:有个页面需要引入3个js文件( a.js, b.js, c.js ), A程序员在a.js文件中定义了一个函数叫show, B程序员在b.js中也定义了一个函数叫show, 那么引入同一个页面之后,后面的函数会把前面的覆盖,那C程序员引入之后,本来想调用A程序员的show方法,结果被B程序员写的文件给覆盖了,这就很恐怖了,如果这是一个公共方法(核心方法),可能造成系统完全挂掉,那怎么解决呢?早期程序员一般用命名空间,如:
1 var ghostwu = ghostwu || {}; 2 ghostwu.tools = { 3 checkEmail: function () { 4 }, 5 checkName: function () { 6 }, 7 checkPwd: function () { 8 } 9 } 10 var zhangsan = zhangsan || {}; 11 zhangsan.tools = { 12 checkEmail: function () { 13 }, 14 checkName: function () { 15 }, 16 checkPwd: function () { 17 } 18 } 19 ghostwu.tools.checkPwd(); 20 zhangsan.tools.checkPwd();
这样就有可能大大降低重名的可能性,但是还是会有小部分覆盖的可能性,当然可以在程序中判断,如果存在这个命名空间,就不让定义,但是这样子写,就没有封装性可言,只要引入了js文件,谁都能够操作这些命名空间,也很危险,对于公共接口( 比如获取数据,可以暴露给外部使用),对于一些核心,比较重要的,就要封装起来,不要暴露给外部使用,所以可以通过闭包( 立即表达式)把命名空间中的方法选择性的暴露给外部
1 (function ( window, undefined ) { 2 var ghostwu = ghostwu || {}; 3 ghostwu.tools = { 4 checkEmail: function () { 5 console.log('ghostwu给你暴露一个公共的checkEmail方法'); 6 }, 7 checkName: function () { 8 console.log('checkName'); 9 }, 10 checkPwd: function () { 11 console.log('checkPwd'); 12 } 13 }, 14 public = { 15 checkEmail : ghostwu.tools.checkEmail, 16 } 17 window.G = public; 18 })( window ); 19 G.checkEmail(); 20 G.checkName(); //报错, checkName没有暴露出来
改进之后,封装性和团队协作开发又得到了进一步的提高,但是依然存在另一个问题,后来的开发者如果要为这个框架新增一个功能模块,而且也能选择性的暴露一些接口怎么办呢?
我们可以封装一个模块扩展功能和模块使用功能,以后要做扩展就非常方便了.
1 (function (window, undefined) { 2 var G = G || {}; 3 G.version = 'v1.0.0'; 4 G.define = function (path, fn) { 5 var args = path.split('.'), 6 parent = old = this; 7 if (args[0] === 'G') { 8 args = args.slice(1); 9 } 10 if (args[0] === 'define' || args[0] === 'module') { 11 return; 12 } 13 for (var i = 0, len = args.length; i < len; i++) { 14 if (typeof parent[args[i]] === 'undefined') { 15 parent[args[i]] = {}; 16 } 17 old = parent; 18 parent = parent[args[i]]; 19 } 20 if (fn) old[args[--i]] = fn(); 21 return this; 22 }; 23 window.G = G; 24 })(window); 25 26 G.define( "ghostwu.string", function(){ 27 return { 28 trimLeft : function( str ){ 29 return str.replace( /^\s+/, '' ); 30 }, 31 trimRight : function(){ 32 return str.replace( /\s+$/, '' ); 33 }, 34 trim : function(){ 35 return str.replace( /^\s+|\s+$/g, '' ); 36 } 37 } 38 } ); 39 40 var str = ' 跟着ghostwu学习设计模式 '; 41 alert( '(' + str + ')' ); 42 alert( '(' + G.ghostwu.string.trimLeft( str ) + ')' ); 43 alert( '(' + G.ghostwu.string.trimRight( str ) + ')' ); 44 alert( '(' + G.ghostwu.string.trim( str ) + ')' );
我们封装了一个define函数,这个函数用来给G模块扩展功能,这里我扩展了一个字符串处理对象,包含了3个方法: trim, trimLeft, trimRight
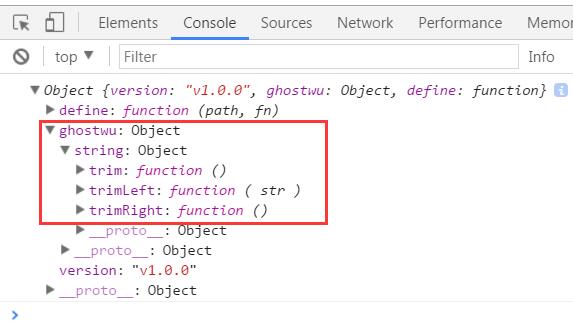
使用的时候,按照命名空间的方式使用即可,我们也可以加一个使用模块的方法use
1 (function (window, undefined) { 2 var G = G || {}; 3 G.version = 'v1.0.0'; 4 G.define = function (path, fn) { 5 var args = path.split('.'), 6 parent = old = this; 7 if (args[0] === 'G') { 8 args = args.slice(1); 9 } 10 if (args[0] === 'define' || args[0] === 'module') { 11 return; 12 } 13 for (var i = 0, len = args.length; i < len; i++) { 14 if (typeof parent[args[i]] === 'undefined') { 15 parent[args[i]] = {}; 16 } 17 old = parent; 18 parent = parent[args[i]]; 19 } 20 if (fn) old[args[--i]] = fn(); 21 return this; 22 }; 23 G.use = function(){ 24 var args = Array.prototype.slice.call( arguments ), 25 fn = args.pop(), 26 path = args[0] && args[0] instanceof Array ? args[0] : args, 27 relys = [], 28 mId = '', 29 i = 0, 30 len = path.length, 31 parent, j, jLen; 32 while ( i < len ) { 33 if( typeof path[i] === 'string' ){ 34 parent = this; 35 mId = path[i].replace( /^G\./, '' ).split('.'); 36 for( var j = 0, jLen = mId.length; j < jLen; j++ ){ 37 parent = parent[mId[j]] || false; 38 } 39 relys.push( parent ); 40 }else { 41 relys.push( path[i] ); 42 } 43 i++; 44 } 45 fn.apply( null, relys ); 46 }; 47 window.G = G; 48 })(window); 49 50 G.define( "ghostwu.string", function(){ 51 return { 52 trimLeft : function( str ){ 53 return str.replace( /^\s+/, '' ); 54 }, 55 trimRight : function(){ 56 return str.replace( /\s+$/, '' ); 57 }, 58 trim : function(){ 59 return str.replace( /^\s+|\s+$/g, '' ); 60 } 61 } 62 } ); 63 64 var str = ' 跟着ghostwu学习设计模式 '; 65 // G.use( ['ghostwu.string'], function( s ){ 66 // console.log( '(' + s.trim( str ) + ')' ); 67 // } ); 68 // G.use( ['ghostwu.string', document], function( s, doc ){ 69 // console.log( doc ); 70 // console.log( '(' + s.trim( str ) + ')' ); 71 // } ); 72 G.use( 'ghostwu.string', function( s ){ 73 console.log( s ); 74 alert( '(' + s.trim( str ) + ')' ); 75 } );
相关文章推荐
- [js高手之路]设计模式系列课程-设计一个模块化扩展功能(define)和使用(use)库
- [js高手之路]设计模式系列课程-设计一个模块化扩展功能(define)和使用(use)库
- [js高手之路]设计模式系列课程-设计一个模块化扩展功能(define)和使用(use)库
- [js高手之路]设计模式系列课程-委托模式实战微博发布功能
- [js高手之路] 设计模式系列课程 - DOM迭代器(2)
- [js高手之路]设计模式系列课程-发布者,订阅者重构购物车
- [js高手之路]设计模式系列课程-组合模式+寄生组合继承实战新闻列表
- [js高手之路]设计模式系列课程-发布者,订阅者重构购物车
- [js高手之路]设计模式系列课程-发布者,订阅者重构购物车
- [js高手之路] 设计模式系列课程 - jQuery的链式调用与灵活的构造函数
- [js高手之路]设计模式系列课程-发布者,订阅者重构购物车的实例
- [js高手之路] 设计模式系列课程 - 迭代器(1)
- [js高手之路] 设计模式系列课程 - jQuery的extend插件机制
- [js高手之路]设计模式系列课程-组合模式+寄生组合继承实战新闻列表
- [js高手之路]设计模式系列课程-组合模式+寄生组合继承实战新闻列表
- [js高手之路]设计模式系列课程-单例模式实现模态框
- [js高手之路]设计模式系列课程-组合模式+寄生组合继承实战新闻列表
- [js高手之路]设计模式系列课程-单例模式实现模态框
- [js高手之路] es6系列教程 - 对象功能扩展详解
- [js高手之路] es6系列教程 - 对象功能扩展详解