ReactJS组件的生命周期
2017-08-23 10:46
676 查看
先来看看官方给出的示例图,然后我们在通过代码来实际感受一下:
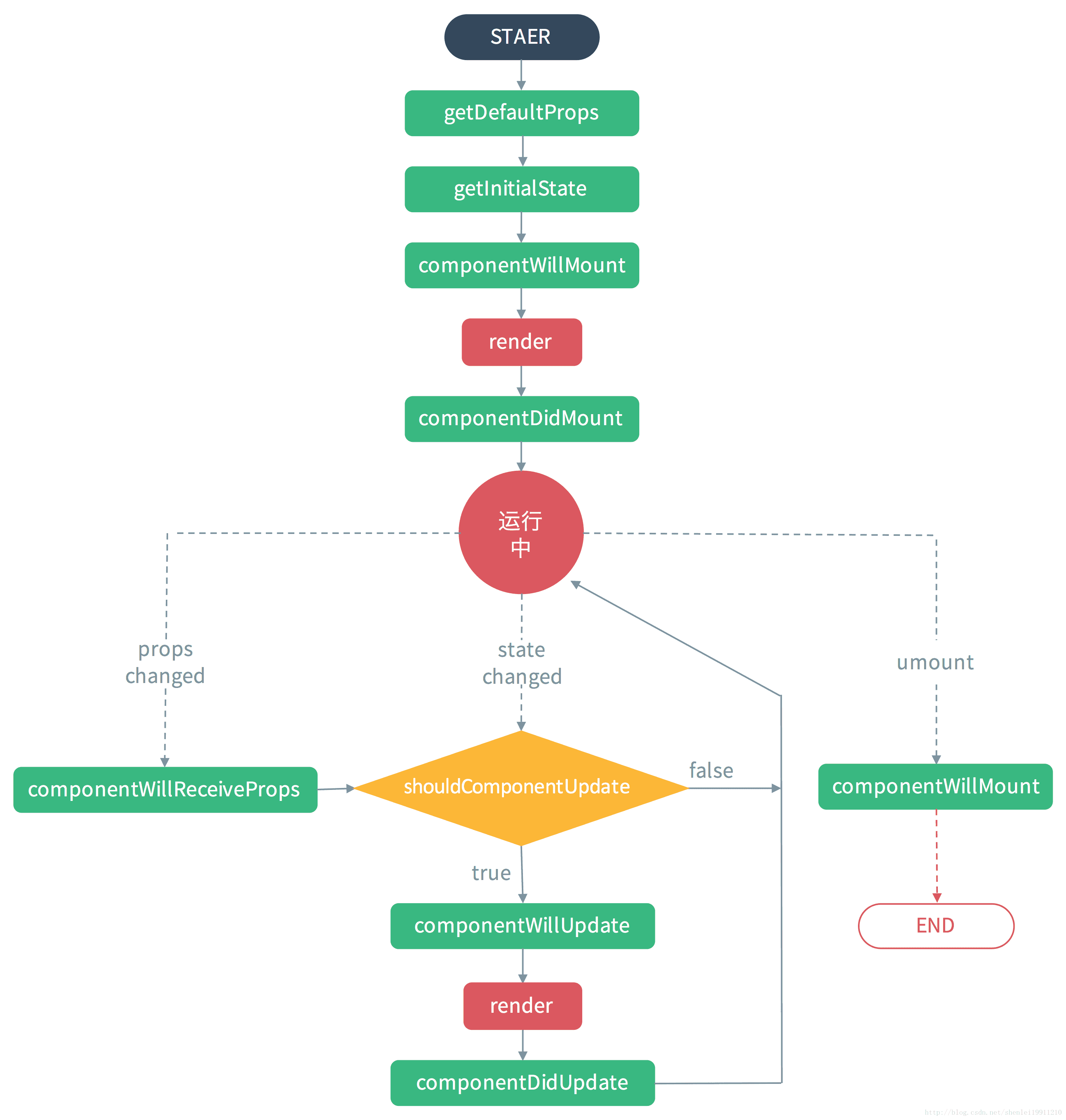
为了理解ReactJS中的组件的生命周期,我们通过下面的示例代码来直观的感受一下,当我们的React组件的整个生命周期都发生了哪些事件。直接上代码和运行结果吧!
css代码
html代码
jsx代码
运行结果:
Initial
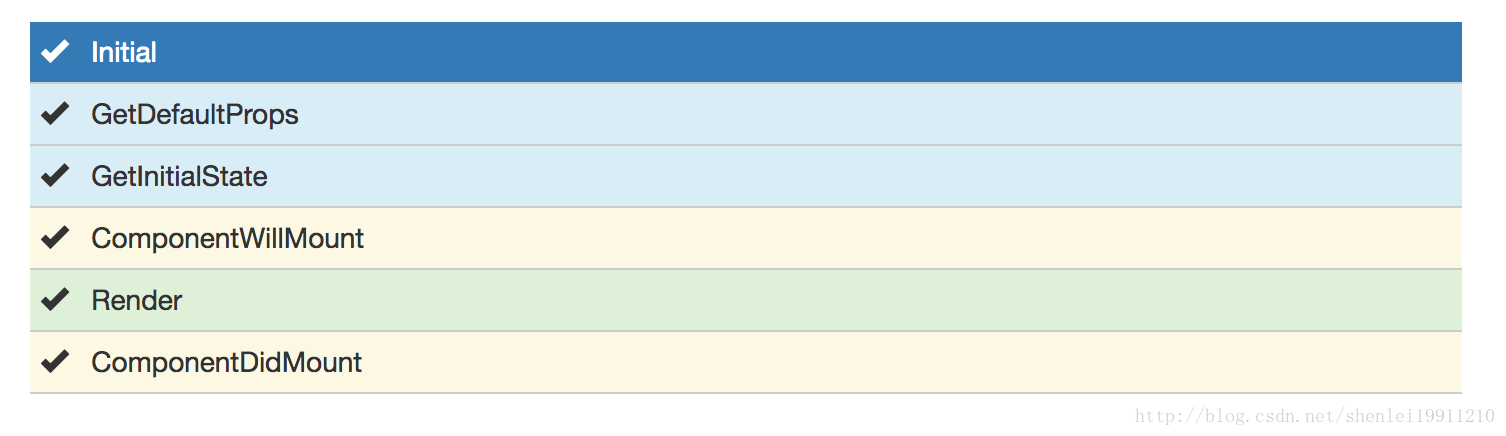
UpdateProps
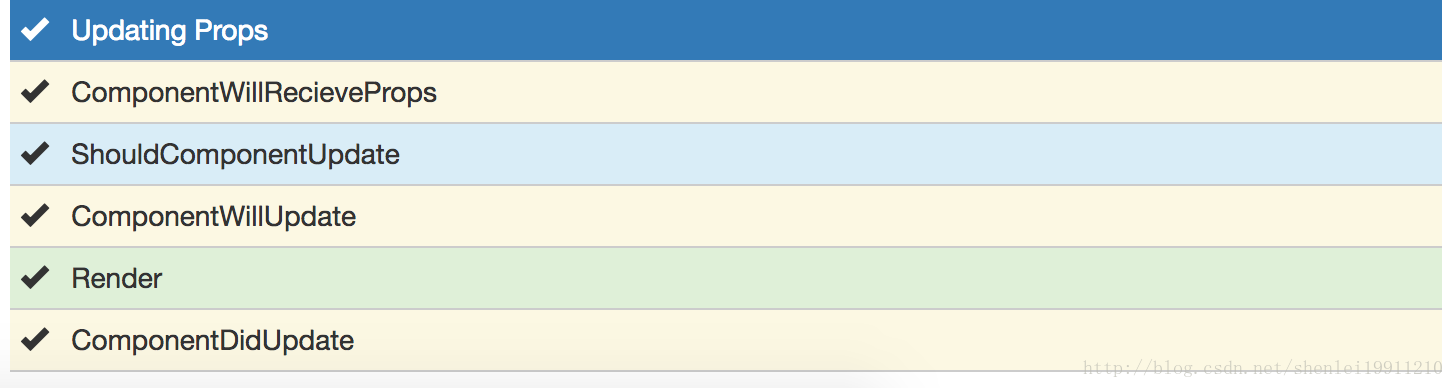
UpdateState
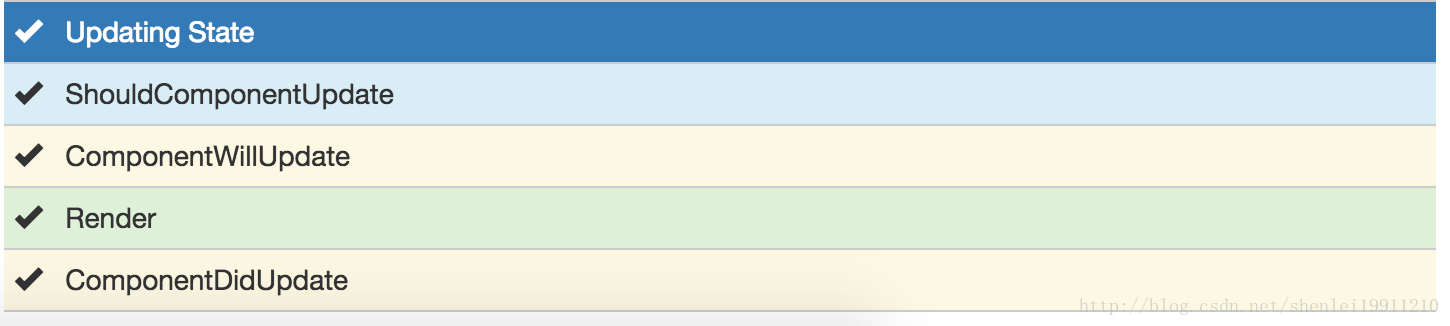
unmount

whole
为了理解ReactJS中的组件的生命周期,我们通过下面的示例代码来直观的感受一下,当我们的React组件的整个生命周期都发生了哪些事件。直接上代码和运行结果吧!
css代码
.main { padding: 10px 50px; } .log { padding: 5px; border-bottom: 1px solid #ccc; }
html代码
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.2/css/bootstrap.min.css" /> <script src="http://libs.cdnjs.net/react/0.12.0/react.min.js"></script> <script src="http://code.jquery.com/jquery-2.1.3.min.js"></script> <link rel="stylesheet" href="style.css" /> </head> <body> <div class="main"> <h1>Understanding the React Component Lifecycle</h1> <div id="app"></div> <hr /> <button type="button" id="unmount" class="btn btn-danger">Unmount</button> <hr /> <div id="screen"></div> </div> <script src="script.js"></script> </body> </html>
jsx代码
writeToScreen('Initial', 'primary'); var Welcome = React.createClass({ getInitialState: function() { writeToScreen('GetInitialState', 'info'); return {foo : 1}; }, getDefaultProps: function() { writeToScreen('GetDefaultProps', 'info'); return {bar: 2}; }, update: function() { writeToScreen('Updating State', 'primary'); this.setState({foo: 2}); }, render: function() { writeToScreen('Render', 'success'); return (<div> This.state.foo: {this.state.foo} <br /> This.state.bar: {this.props.bar} <br/> <hr/> <button className="btn btn-success" onClick={this.update}> Update State </button> </div>); }, componentWillMount: function() { writeToScreen('ComponentWillMount', 'warning'); }, componentDidMount: function() { writeToScreen('ComponentDidMount', 'warning'); }, shouldComponentUpdate: function() { writeToScreen('ShouldComponentUpdate', 'info'); return true; }, componentWillReceiveProps: function(nextProps) { writeToScreen('ComponentWillRecieveProps', 'warning'); }, componentWillUpdate: function() { writeToScreen('ComponentWillUpdate', 'warning'); }, componentDidUpdate: function() { writeToScreen('ComponentDidUpdate', 'warning'); }, componentWillUnmount: function() { writeToScreen('componentWillUnmount', 'danger'); } }); var App = React.createClass({ getInitialState: function() { return {id: 1}; }, update: function() { writeToScreen('Updating Props', 'primary'); this.setState({id: 2}); }, render: function() { return ( <div> <hr/> <Welcome bar={this.state.id} /> <hr /> <button type="button" className="btn btn-primary" onClick={this.update}> Update Props </button> </div> ) } }); React.render( <App />, document.getElementById('app') ); var unmountBtn = document.getElementById('unmount'); unmountBtn.addEventListener('click', unmount); function unmount() { writeToScreen('Unmounting', 'primary'); React.unmountComponentAtNode(document.getElementById('app')); unmountBtn.remove(); } function writeToScreen(msg, level) { var elem = document.getElementById('screen'); elem.innerHTML += '<div class="log bg-' + level + '">' + '<span class="glyphicon glyphicon-ok"></span> ' + msg + '</div>'; }
运行结果:
Initial
UpdateProps
UpdateState
unmount
whole
相关文章推荐
- ReactJS组件的生命周期详解
- React Native实战之ReactJS组件生命周期
- ReactJS组件生命周期详述
- reactJS -- 8 组件生命周期
- reactjs入门到实战(七)---- React的组件的生命周期
- js-react组件生命周期
- ReactJS组件生命周期详述
- reactjs,组件的生命周期
- 老生常谈js-react组件生命周期
- 【ReactJS】通过一个例子学习React组件的生命周期
- React.js组件的生命周期
- react.js 从零开始(二)组件的生命周期
- ReactJS组件生命周期(ES5为例)
- ReactNative 第六节 实战之ReactJS 组件和生命周期
- React组件生命周期
- 关于在reactjs项目中如何用webpack配置组件按需加载
- React Native 中组件的生命周期
- React Native 中组件的生命周期
- React:组件的生命周期
- React.js之组件通信(基础)