Difference between ArrayList and LinkedList in Java
2017-07-10 14:02
936 查看
When practicing on Leetcode, I often utilize a common data structure: list, and there are two main types for list in java: ArrayList and LinkedList. So, what's the difference between them and how we choose between them when using list.
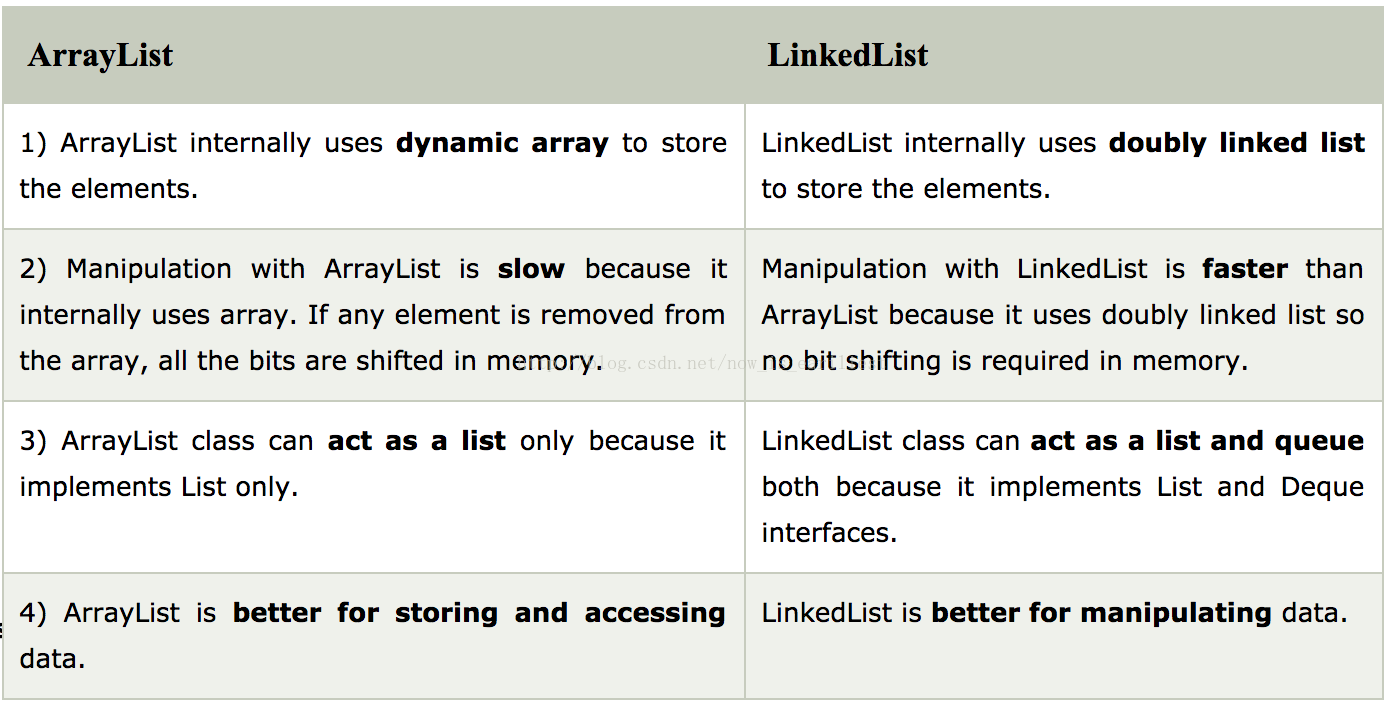
(from https://www.javatpoint.com/difference-between-arraylist-and-linkedlist)
From above picture, we learnt that these two implementations use different data structures internally. For ArrayList, it uses dynamic array, while LinkedList, double linked list. Based on this fact, it is easy to know, ArrayList is better for searching while
worse for inserting and removing; LinkedList is better for inserting and removing, while worse for searching.
From the java doc, it says both of the implementations are
synchronized.
In the leetcode 380, one of the solution uses the ArrayList and wisely avoid its defect, it tries to insert and remove the element at the ending of the list.
public class RandomizedSet {
/** Initialize your data structure here. */
Mapmap;//val -> index
Listlist;
public RandomizedSet() {
map = new HashMap<>();
list = new ArrayList<>();
}
/** Inserts a value to the set. Returns true if the set did not already contain the specified element. */
public boolean insert(int val) {
if(!map.containsKey(val)){
list.add(val);
map.put(val, list.size() - 1);
return true;
}
else return false;
}
/** Removes a value from the set. Returns true if the set contained the specified element. */
public boolean remove(int val) {
if(!map.containsKey(val))
return false;
else{
int index = map.get(val), ending = list.get(list.size() - 1);
list.set(index, ending);
list.remove(list.size() - 1);
map.put(ending, index);
map.remove(val);
return true;
}
}
/** Get a random element from the set. */
public int getRandom() {
if(list.size() <= 0)
return -1;
return list.get(new Random().nextInt(list.size()));
}
}
/**
* Your RandomizedSet object will be instantiated and called as such:
* RandomizedSet obj = new RandomizedSet();
* boolean param_1 = obj.insert(val);
* boolean param_2 = obj.remove(val);
* int param_3 = obj.getRandom();
*/
(from https://www.javatpoint.com/difference-between-arraylist-and-linkedlist)
From above picture, we learnt that these two implementations use different data structures internally. For ArrayList, it uses dynamic array, while LinkedList, double linked list. Based on this fact, it is easy to know, ArrayList is better for searching while
worse for inserting and removing; LinkedList is better for inserting and removing, while worse for searching.
From the java doc, it says both of the implementations are
synchronized.
In the leetcode 380, one of the solution uses the ArrayList and wisely avoid its defect, it tries to insert and remove the element at the ending of the list.
public class RandomizedSet {
/** Initialize your data structure here. */
Mapmap;//val -> index
Listlist;
public RandomizedSet() {
map = new HashMap<>();
list = new ArrayList<>();
}
/** Inserts a value to the set. Returns true if the set did not already contain the specified element. */
public boolean insert(int val) {
if(!map.containsKey(val)){
list.add(val);
map.put(val, list.size() - 1);
return true;
}
else return false;
}
/** Removes a value from the set. Returns true if the set contained the specified element. */
public boolean remove(int val) {
if(!map.containsKey(val))
return false;
else{
int index = map.get(val), ending = list.get(list.size() - 1);
list.set(index, ending);
list.remove(list.size() - 1);
map.put(ending, index);
map.remove(val);
return true;
}
}
/** Get a random element from the set. */
public int getRandom() {
if(list.size() <= 0)
return -1;
return list.get(new Random().nextInt(list.size()));
}
}
/**
* Your RandomizedSet object will be instantiated and called as such:
* RandomizedSet obj = new RandomizedSet();
* boolean param_1 = obj.insert(val);
* boolean param_2 = obj.remove(val);
* int param_3 = obj.getRandom();
*/
相关文章推荐
- Difference between LinkedList vs ArrayList in Java
- Java - Difference between LinkedList and ArrayList
- Java: Difference between ArrayList and LinkedList
- Java: Difference between ArrayList and LinkedList
- Difference between Set, List and Map in Java - Interview question
- Difference between List and Set in Java Collection
- Difference between ArrayList and Vector in Java
- Difference between List and Set in Java Collection
- Difference between ArrayList and Vector In java
- Difference between Vector and ArrayList in java?
- Difference between List and Set in Java Collection
- Java - Difference between ArrayList and Vector in Java
- Difference between HashMap, LinkedHashMap and TreeMap in Java
- differences between List and Set interface in point format
- Difference between List View and DataGrid in WPF
- Difference between ConcurrentHashMap and Collections.synchronizedMap and Hashtable in Java
- Difference Between Class.ForName() And ClassLoader.LoadClass() Methods In Java
- Difference between Stack and Heap in Java
- Difference between getmessage and getlocalizedmessage in exceptions Java?
- Difference between RegularEnumSet and JumboEnumSet in Java