base64之前端加密后端解密
2017-07-04 15:17
405 查看
前端引入base64.js
base64.js
/**
*
* Base64 encode / decode
*
* @author haitao.tu
* @date 2010-04-26
* @email tuhaitao@foxmail.com
*
*/
function Base64() {
// private property
_keyStr = “ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=”;
// public method for encoding
this.encode = function (input) {
var output = “”;
var chr1, chr2, chr3, enc1, enc2, enc3, enc4;
var i = 0;
input = _utf8_encode(input);
while (i < input.length) {
chr1 = input.charCodeAt(i++);
chr2 = input.charCodeAt(i++);
chr3 = input.charCodeAt(i++);
enc1 = chr1 >> 2;
enc2 = ((chr1 & 3) << 4) | (chr2 >> 4);
enc3 = ((chr2 & 15) << 2) | (chr3 >> 6);
enc4 = chr3 & 63;
if (isNaN(chr2)) {
enc3 = enc4 = 64;
} else if (isNaN(chr3)) {
enc4 = 64;
}
output = output +
_keyStr.charAt(enc1) + _keyStr.charAt(enc2) +
_keyStr.charAt(enc3) + _keyStr.charAt(enc4);
}
return output;
}
// public method for decoding
this.decode = function (input) {
var output = “”;
var chr1, chr2, chr3;
var enc1, enc2, enc3, enc4;
var i = 0;
input = input.replace(/[^A-Za-z0-9+\/\=]/g, “”);
while (i < input.length) {
enc1 = _keyStr.indexOf(input.charAt(i++));
enc2 = _keyStr.indexOf(input.charAt(i++));
enc3 = _keyStr.indexOf(input.charAt(i++));
enc4 = _keyStr.indexOf(input.charAt(i++));
chr1 = (enc1 << 2) | (enc2 >> 4);
chr2 = ((enc2 & 15) << 4) | (enc3 >> 2);
chr3 = ((enc3 & 3) << 6) | enc4;
output = output + String.fromCharCode(chr1);
if (enc3 != 64) {
output = output + String.fromCharCode(chr2);
}
if (enc4 != 64) {
output = output + String.fromCharCode(chr3);
}
}
output = _utf8_decode(output);
return output;
}
// private method for UTF-8 encoding
_utf8_encode = function (string) {
string = string.replace(/\r\n/g,”\n”);
var utftext = “”;
for (var n = 0; n < string.length; n++) {
var c = string.charCodeAt(n);
if (c < 128) {
utftext += String.fromCharCode(c);
} else if((c > 127) && (c < 2048)) {
utftext += String.fromCharCode((c >> 6) | 192);
utftext += String.fromCharCode((c & 63) | 128);
} else {
utftext += String.fromCharCode((c >> 12) | 224);
utftext += String.fromCharCode(((c >> 6) & 63) | 128);
utftext += String.fromCharCode((c & 63) | 128);
}
}
return utftext;
}
// private method for UTF-8 decoding
_utf8_decode = function (utftext) {
var string = “”;
var i = 0;
var c = c1 = c2 = 0;
while ( i < utftext.length ) {
c = utftext.charCodeAt(i);
if (c < 128) {
string += String.fromCharCode(c);
i++;
} else if((c > 191) && (c < 224)) {
c2 = utftext.charCodeAt(i+1);
string += String.fromCharCode(((c & 31) << 6) | (c2 & 63));
i += 2;
} else {
c2 = utftext.charCodeAt(i+1);
c3 = utftext.charCodeAt(i+2);
string += String.fromCharCode(((c & 15) << 12) | ((c2 & 63) << 6) | (c3 & 63));
i += 3;
}
}
return string;
}
}
直接在前端js代码里调用进行加密处理
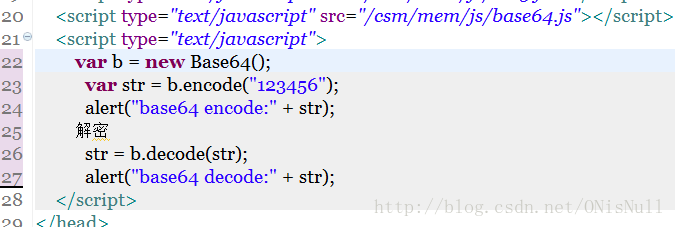
后端解密代码:
package com.base.util;
import java.io.IOException;
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
/**
* BASE64算法工具类,该算法封装了对字符串,
* 字节数组的加密和字符串解密的功能.
* @author mzllon
* @version 1.0,05/21/2012
*
*/
public final class Base64Util {
/**
* 采用BASE64算法对字符串进行加密
* @param base 原字符串
* @return 加密后的字符串
*/
public static final String encode(String base){
return Base64Util.encode(base.getBytes());
}
}
调用Base64Util进行解密,并用Md5加密存入数据库:

附加一个md5加密:
package com.base.util;
import java.security.MessageDigest;
import sun.misc.BASE64Encoder;
public class PasswordMD5{
}
base64.js
/**
*
* Base64 encode / decode
*
* @author haitao.tu
* @date 2010-04-26
* @email tuhaitao@foxmail.com
*
*/
function Base64() {
// private property
_keyStr = “ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=”;
// public method for encoding
this.encode = function (input) {
var output = “”;
var chr1, chr2, chr3, enc1, enc2, enc3, enc4;
var i = 0;
input = _utf8_encode(input);
while (i < input.length) {
chr1 = input.charCodeAt(i++);
chr2 = input.charCodeAt(i++);
chr3 = input.charCodeAt(i++);
enc1 = chr1 >> 2;
enc2 = ((chr1 & 3) << 4) | (chr2 >> 4);
enc3 = ((chr2 & 15) << 2) | (chr3 >> 6);
enc4 = chr3 & 63;
if (isNaN(chr2)) {
enc3 = enc4 = 64;
} else if (isNaN(chr3)) {
enc4 = 64;
}
output = output +
_keyStr.charAt(enc1) + _keyStr.charAt(enc2) +
_keyStr.charAt(enc3) + _keyStr.charAt(enc4);
}
return output;
}
// public method for decoding
this.decode = function (input) {
var output = “”;
var chr1, chr2, chr3;
var enc1, enc2, enc3, enc4;
var i = 0;
input = input.replace(/[^A-Za-z0-9+\/\=]/g, “”);
while (i < input.length) {
enc1 = _keyStr.indexOf(input.charAt(i++));
enc2 = _keyStr.indexOf(input.charAt(i++));
enc3 = _keyStr.indexOf(input.charAt(i++));
enc4 = _keyStr.indexOf(input.charAt(i++));
chr1 = (enc1 << 2) | (enc2 >> 4);
chr2 = ((enc2 & 15) << 4) | (enc3 >> 2);
chr3 = ((enc3 & 3) << 6) | enc4;
output = output + String.fromCharCode(chr1);
if (enc3 != 64) {
output = output + String.fromCharCode(chr2);
}
if (enc4 != 64) {
output = output + String.fromCharCode(chr3);
}
}
output = _utf8_decode(output);
return output;
}
// private method for UTF-8 encoding
_utf8_encode = function (string) {
string = string.replace(/\r\n/g,”\n”);
var utftext = “”;
for (var n = 0; n < string.length; n++) {
var c = string.charCodeAt(n);
if (c < 128) {
utftext += String.fromCharCode(c);
} else if((c > 127) && (c < 2048)) {
utftext += String.fromCharCode((c >> 6) | 192);
utftext += String.fromCharCode((c & 63) | 128);
} else {
utftext += String.fromCharCode((c >> 12) | 224);
utftext += String.fromCharCode(((c >> 6) & 63) | 128);
utftext += String.fromCharCode((c & 63) | 128);
}
}
return utftext;
}
// private method for UTF-8 decoding
_utf8_decode = function (utftext) {
var string = “”;
var i = 0;
var c = c1 = c2 = 0;
while ( i < utftext.length ) {
c = utftext.charCodeAt(i);
if (c < 128) {
string += String.fromCharCode(c);
i++;
} else if((c > 191) && (c < 224)) {
c2 = utftext.charCodeAt(i+1);
string += String.fromCharCode(((c & 31) << 6) | (c2 & 63));
i += 2;
} else {
c2 = utftext.charCodeAt(i+1);
c3 = utftext.charCodeAt(i+2);
string += String.fromCharCode(((c & 15) << 12) | ((c2 & 63) << 6) | (c3 & 63));
i += 3;
}
}
return string;
}
}
直接在前端js代码里调用进行加密处理
后端解密代码:
package com.base.util;
import java.io.IOException;
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
/**
* BASE64算法工具类,该算法封装了对字符串,
* 字节数组的加密和字符串解密的功能.
* @author mzllon
* @version 1.0,05/21/2012
*
*/
public final class Base64Util {
/**
* 采用BASE64算法对字符串进行加密
* @param base 原字符串
* @return 加密后的字符串
*/
public static final String encode(String base){
return Base64Util.encode(base.getBytes());
}
/** * 采用BASE64算法对字节数组进行加密 * @param baseBuff 原字节数组 * @return 加密后的字符串 */ public static final String encode(byte[] baseBuff){ return new BASE64Encoder().encode(baseBuff); } /** * 字符串解密,采用BASE64的算法 * @param encoder 需要解密的字符串 * @return 解密后的字符串 */ public static final String decode(String encoder){ try { BASE64Decoder decoder = new BASE64Decoder(); byte[] buf = decoder.decodeBuffer(encoder); return new String(buf); } catch (IOException e) { e.printStackTrace(); return null; } }
}
调用Base64Util进行解密,并用Md5加密存入数据库:
附加一个md5加密:
package com.base.util;
import java.security.MessageDigest;
import sun.misc.BASE64Encoder;
public class PasswordMD5{
public static String createEncryptPSW(String psw) { MessageDigest messagedigest = null; try { messagedigest = MessageDigest.getInstance("MD5"); messagedigest.update(psw.getBytes("UTF8")); byte abyte0[] = messagedigest.digest(); psw=(new BASE64Encoder()).encode(abyte0); } catch (Exception e) { e.getMessage(); } return psw; } public static void main(String[] args) { String pwd="123"; String password= PasswordMD5.createEncryptPSW(pwd); System.out.println(password); }
}
相关文章推荐
- 关于base64前端加密,后端解密
- [置顶] 前端加密后端解密之Base64通用加密处理
- Base64的加密解密
- BASE64加密 解密
- javaScript base64算法的实现 与 java中的base64 加密 解密
- AES加密解密 附赠 base64
- php中base64_decode与base64_encode加密解密函数实例
- jQuery实现base64前台加密解密功能详解
- Oracle杂文:base64加密解密
- base64 前端加解密与后端解密
- C# DES 加密/解密,支持文件和中文/UNICODE字符,返回BASE64编码字符串
- 加密解密算法java实现(1)—BASE64
- MD5加密,Base64加密/解密,AES加密/解密
- C#中base64之加密解密
- base64加密 解密
- python中base64加密解密方法实例分析
- php实现base64加密解密的代码
- @Java Base64 加密解密
- C# 加密解密(DES,3DES,MD5,Base64) 类
- js加密解密之base64encode,base64decode