Netty 4.0 源码分析(四):ByteBuf
2017-07-03 13:05
357 查看
NIO框架中的缓冲区。ByteBuf接口可以理解为一般的Byte数组,不过Netty对Byte进行了封装,增加了一些实用的方法。
ChannelBuf接口
package io.netty.buffer;
public interface ChannelBuf {
ChannelBufType type();
boolean isPooled();
}
ByteBuf接口
package io.netty.buffer;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.nio.channels.GatheringByteChannel;
import java.nio.channels.ScatteringByteChannel;
import java.nio.charset.Charset;
import java.nio.charset.UnsupportedCharsetException;
public interface ByteBuf extends ChannelBuf, Comparable<ByteBuf> {
int capacity();
ByteBuf capacity(int newCapacity);
int maxCapacity();
ByteOrder order();
ByteBuf order(ByteOrder endianness);
boolean isDirect();
int readerIndex();
ByteBuf readerIndex(int readerIndex);
int writerIndex();
ByteBuf writerIndex(int writerIndex);
ByteBuf setIndex(int readerIndex, int writerIndex);
int readableBytes();
int writableBytes();
boolean readable();
boolean writable();
ByteBuf clear();
ByteBuf markReaderIndex();
ByteBuf resetReaderIndex();
ByteBuf markWriterIndex();
ByteBuf resetWriterIndex();
ByteBuf discardReadBytes();
ByteBuf ensureWritableBytes(int minWritableBytes);
int ensureWritableBytes(int minWritableBytes, boolean force);
boolean getBoolean(int index);
byte getByte(int index);
short getUnsignedByte(int index);
short getShort(int index);
int getUnsignedShort(int index);
int getMedium(int index);
int getUnsignedMedium(int index);
int getIn
102e4
t(int index);
long getUnsignedInt(int index);
long getLong(int index);
char getChar(int index);
float getFloat(int index);
double getDouble(int index);
ByteBuf getBytes(int index, ByteBuf dst);
ByteBuf getBytes(int index, ByteBuf dst, int length);
ByteBuf getBytes(int index, ByteBuf dst, int dstIndex, int length);
ByteBuf getBytes(int index, byte[] dst);
ByteBuf getBytes(int index, byte[] dst, int dstIndex, int length);
ByteBuf getBytes(int index, ByteBuffer dst);
ByteBuf getBytes(int index, OutputStream out, int length) throws IOException;
int getBytes(int index, GatheringByteChannel out, int length) throws IOException;
ByteBuf setBoolean(int index, boolean value);
ByteBuf setByte(int index, int value);
ByteBuf setShort(int index, int value);
ByteBuf setMedium(int index, int value);
ByteBuf setInt(int index, int value);
ByteBuf setLong(int index, long value);
ByteBuf setChar(int index, int value);
ByteBuf setFloat(int index, float value);
ByteBuf setDouble(int index, double value);
ByteBuf setBytes(int index, ByteBuf src);
ByteBuf setBytes(int index, ByteBuf src, int length);
ByteBuf setBytes(int index, ByteBuf src, int srcIndex, int length);
ByteBuf setBytes(int index, byte[] src);
ByteBuf setBytes(int index, byte[] src, int srcIndex, int length);
ByteBuf setBytes(int index, ByteBuffer src);
int setBytes(int index, InputStream in, int length) throws IOException;
int setBytes(int index, ScatteringByteChannel in, int length) throws IOException;
ByteBuf setZero(int index, int length);
boolean readBoolean();
byte readByte();
short readUnsignedByte();
short readShort();
int readUnsignedShort();
int readMedium();
int readUnsignedMedium();
int readInt();
long readUnsignedInt();
long readLong();
char readChar();
float readFloat();
double readDouble();
ByteBuf readBytes(int length);
ByteBuf readSlice(int length);
ByteBuf readBytes(ByteBuf dst);
ByteBuf readBytes(ByteBuf dst, int length);
ByteBuf readBytes(ByteBuf dst, int dstIndex, int length);
ByteBuf readBytes(byte[] dst);
ByteBuf readBytes(byte[] dst, int dstIndex, int length);
ByteBuf readBytes(ByteBuffer dst);
ByteBuf readBytes(OutputStream out, int length) throws IOException;
int readBytes(GatheringByteChannel out, int length) throws IOException;
ByteBuf skipBytes(int length);
ByteBuf writeBoolean(boolean value);
ByteBuf writeByte(int value);
ByteBuf writeShort(int value);
ByteBuf writeMedium(int value);
ByteBuf writeInt(int value);
ByteBuf writeLong(long value);
ByteBuf writeChar(int value);
ByteBuf writeFloat(float value);
ByteBuf writeDouble(double value);
ByteBuf writeBytes(ByteBuf src);
ByteBuf writeBytes(ByteBuf src, int length);
ByteBuf writeBytes(ByteBuf src, int srcIndex, int length);
ByteBuf writeBytes(byte[] src);
ByteBuf writeBytes(byte[] src, int srcIndex, int length);
ByteBuf writeBytes(ByteBuffer src);
int writeBytes(InputStream in, int length) throws IOException;
int writeBytes(ScatteringByteChannel in, int length) throws IOException;
ByteBuf writeZero(int length);
int indexOf(int fromIndex, int toIndex, byte value);
int indexOf(int fromIndex, int toIndex, ByteBufIndexFinder indexFinder);
int bytesBefore(byte value);
int bytesBefore(ByteBufIndexFinder indexFinder);
int bytesBefore(int length, byte value);
int bytesBefore(int length, ByteBufIndexFinder indexFinder);
int bytesBefore(int index, int length, byte value);
int bytesBefore(int index, int length, ByteBufIndexFinder indexFinder);
ByteBuf copy();
ByteBuf copy(int index, int length);
ByteBuf slice();
ByteBuf slice(int index, int length);
ByteBuf duplicate();
boolean hasNioBuffer();
ByteBuffer nioBuffer();
ByteBuffer nioBuffer(int index, int length);
boolean hasNioBuffers();
ByteBuffer[] nioBuffers();
ByteBuffer[] nioBuffers(int offset, int length);
boolean hasArray();
byte[] array();
int arrayOffset();
String toString(Charset charset);
String toString(int index, int length, Charset charset);
@Override
int hashCode();
@Override
boolean equals(Object obj);
@Override
int compareTo(ByteBuf buffer);
@Override
String toString();
Unsafe unsafe();
interface Unsafe {
ByteBuffer nioBuffer();
ByteBuffer[] nioBuffers();
ByteBuf newBuffer(int initialCapacity);
void discardSomeReadBytes();
void acquire();
void release();
}
}
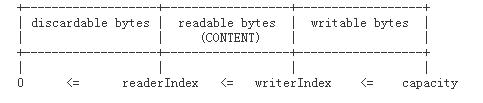
ByteBuf Index
ByteBuf通过两个指针来协助I/O的读写操作,读操作的readIndex和写操作的writeIndex
readerIndex和writerIndex都是一开始都是0,随着数据的写入writerIndex会增加,读取数据会使readerIndex增加,但是他不会超过writerIndx,在读取之后,0-readerIndex的就被视为discard的.调用discardReadBytes方法,可以释放这部分空间,他的作用类似ByeBuffer的compact方法;
读和写的时候Index是分开的,因此也就没必要再每次读完以后调用flip方法,另外还有indexOf、bytesBefore等一些方便的方法;
ByteBuf的几个重要方法
discardReadBytes()
丢弃已读的内容。其执行过程如下:
调用discardReadBytes()之前:
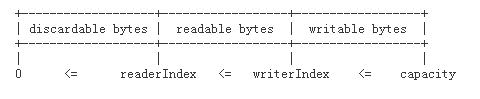
调用 discardReadBytes()方法后
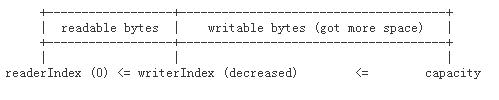
clear()
丢弃所有的数据,并将readerIndex和writerIndex重置为0。
调用clear()之前
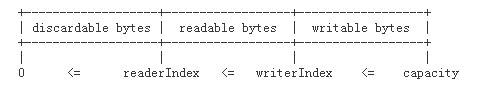
调用clear()之后
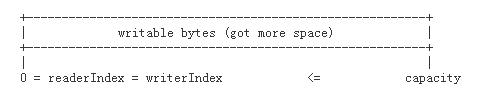
相关文章推荐
- Netty 4.0 源码分析(四):ByteBuf
- Netty 4.0 源码分析(三):Channel和ChannelPipeline
- netty源码分析 之十一 ByteBuf
- Netty 4.0源码分析1:服务端启动过程中的Channel与EventLoopGroup的注册
- Android 4.0 Launcher2源码分析——Launcher内容加载详细过程
- Android 4.0 Launcher2源码分析——目录
- Android 4.0 Launcher源码分析系列(二)
- Android 4.0 Launcher源码分析系列(二)
- Android 4.0 Launcher源码分析系列(三)
- Android 4.0 Launcher2源码分析——主布局文件
- Android 4.0 Launcher2源码分析——Workspace滑动
- Android 4.0 Launcher源码分析系列(二)
- Android 4.0 Launcher2源码分析——导入eclipse进行调试
- Android 4.0 Launcher源码分析系列(一)
- Android 4.0 Launcher2源码分析——启动过程分析
- VNC源码研究(二十四)vnc-4.0-winsrc版本之winvnc工程分析
- Android 4.0 Launcher源码分析系列(一)
- Android 4.0 Launcher源码分析系列(一)
- Android 4.0 Launcher源码分析系列(三)
- Android 4.0 Launcher源码分析系列(三)