dubbo的简单应用
2017-06-21 17:12
113 查看
一. dubbo简介
dubbo是一个分布式服务框架,致力于提供高性能和透明化的RPC远程服务调用方案,是阿里巴巴SOA服务化治理方案的核心框架。
二. 架构
引用dubbo的架构图:
pom.xml
application.xml配置文件如下:
dubbo.xml配置文件如下:
项目启动配置文件web.xml:
index.jsp页面很简单,就是做一个提交form的请求:
UserServlet.java代码也很简单,仅仅用于接收请求并且调用暴露的服务:
在servlet的init()方法中,我使用了spring类获取暴露的服务接口,实际上获取到的是dubbo创建的代理对象。
四. 运行
因为dubbo是基于长连接发送数据的,所以消息订阅者在启动时就会去查找这个认为已经注册的服务,如果不存在这个服务,则会报错。所以应该先注册服务,在开启订阅者订阅。故应该先启动Provider项目再启动Consumer项目。
一切运行成功之后,打开Consumer的index.jsp页面:
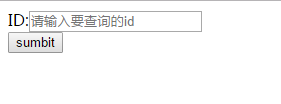
输入id点击提交之后:

说明服务调用成功,分布式作用起效了。
五. 总结
此代码仅作为抛砖引玉的作用,用来说明dubbo的基本应用。dubbo官方的文档写的很详细,从配置到说明示例。目前很多地方还没有探索到,比如启动是顺序其实可以设置服务启动时候是否检查,暴露服务的协议使用hessian还是dubbo,服务超时连接设置、服务暴露方式使用zookeeper等等。
dubbo是一个分布式服务框架,致力于提供高性能和透明化的RPC远程服务调用方案,是阿里巴巴SOA服务化治理方案的核心框架。
二. 架构
引用dubbo的架构图:
1 <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" 2 xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> 3 <modelVersion>4.0.0</modelVersion> 4 <groupId>com.bigbang</groupId> 5 <artifactId>consumer</artifactId> 6 <packaging>war</packaging> 7 <version>0.0.1-SNAPSHOT</version> 8 <name>consumer</name> 9 <properties> 10 <spring.version>3.2.4.RELEASE</spring.version> 11 </properties> 12 <dependencies> 13 <dependency> 14 <groupId>junit</groupId> 15 <artifactId>junit</artifactId> 16 <version>3.8.1</version> 17 <scope>test</scope> 18 </dependency> 19 <dependency> 20 <groupId>javax.servlet</groupId> 21 <artifactId>servlet-api</artifactId> 22 <version>2.3</version> 23 </dependency> 24 <dependency> 25 <groupId>org.springframework</groupId> 26 <artifactId>spring-context</artifactId> 27 <version>${spring.version}</version> 28 </dependency> 29 30 <dependency> 31 <groupId>org.springframework</groupId> 32 <artifactId>spring-core</artifactId> 33 <version>${spring.version}</version> 34 </dependency> 35 36 <dependency> 37 <groupId>org.springframework</groupId> 38 <artifactId>spring-beans</artifactId> 39 <version>${spring.version}</version> 40 </dependency> 41 42 <dependency> 43 <groupId>org.springframework</groupId> 44 <artifactId>spring-webmvc</artifactId> 45 <version>${spring.version}</version> 46 </dependency> 47 <dependency> 48 <groupId>org.springframework</groupId> 49 <artifactId>spring-orm</artifactId> 50 <version>${spring.version}</version> 51 </dependency> 52 53 <dependency> 54 <groupId>com.alibaba</groupId> 55 <artifactId>dubbo</artifactId> 56 <version>2.5.3</version> 57 </dependency> 58 59 <dependency> 60 <groupId>com.bigbang</groupId> 61 <artifactId>service</artifactId> 62 <version>0.0.1-SNAPSHOT</version> 63 </dependency> 64 </dependencies> 65 <build> 66 <plugins> 67 <plugin> 68 <groupId>org.apache.maven.plugins</groupId> 69 <artifactId>maven-compiler-plugin</artifactId> 70 <configuration> 71 <source>1.8</source> 72 <target>1.8</target> 73 </configuration> 74 </plugin> 75 </plugins> 76 <finalName>consumer</finalName> 77 </build> 78 </project>
pom.xml
application.xml配置文件如下:
1 <?xml version="1.0" encoding="UTF-8"?> 2 <beans xmlns="http://www.springframework.org/schema/beans" 3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" 4 xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> 5 6 <import resource="dubbo.xml" /> 7 </beans>
dubbo.xml配置文件如下:
1 <?xml version="1.0" encoding="UTF-8"?> 2 <beans xmlns="http://www.springframework.org/schema/beans" 3 xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo" 4 xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://code.alibabatech.com/schema/dubbo 5 http://code.alibabatech.com/schema/dubbo/dubbo.xsd"> 6 7 <!-- 消费方应用名,用于计算依赖关系,不是匹配条件,不要与提供方一样 --> 8 <dubbo:application name="consumer" /> 9 10 <!-- 使用multicast广播注册中心暴露发现服务地址 --> 11 <dubbo:registry address="multicast://224.5.6.7:1234" /> 12 13 <!-- 用dubbo协议在20880端口暴露服务 --> 14 <dubbo:protocol name="dubbo" port="20880" /> 15 16 <!-- 生成远程服务代理,可以和本地bean一样使用 --> 17 <dubbo:reference id="userService" interface="com.bigbang.service.UserService" /> 18 </beans>
项目启动配置文件web.xml:
1 <?xml version="1.0" encoding="UTF-8"?> 2 <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" 3 xmlns="http://java.sun.com/xml/ns/javaee" 4 xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" 5 id="WebApp_ID" version="2.5"> 6 <display-name>consumer</display-name> 7 <context-param> 8 <param-name>contextConfigLocation</param-name> 9 <param-value>classpath*:applicationContext.xml</param-value> 10 </context-param> 11 <listener> 12 <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> 13 </listener> 14 <welcome-file-list> 15 <welcome-file>index.jsp</welcome-file> 16 </welcome-file-list> 17 <servlet> 18 <description>用户查询servlet</description> 19 <display-name>UserQueryServlet</display-name> 20 <servlet-name>UserQueryServlet</servlet-name> 21 <servlet-class>com.bigbang.servlet.UserQueryServlet</servlet-class> 22 <load-on-startup>1</load-on-startup> 23 </servlet> 24 <servlet-mapping> 25 <servlet-name>UserQueryServlet</servlet-name> 26 <url-pattern>/userQueryServlet</url-pattern> 27 </servlet-mapping> 28 </web-app>
index.jsp页面很简单,就是做一个提交form的请求:
1 <form action="/userQueryServlet" method="post"> 2 ID:<input name="id" type="text" placeholder="请输入要查询的id" /><br/> 3 <input type="submit" value="sumbit"/> 4 </form>
UserServlet.java代码也很简单,仅仅用于接收请求并且调用暴露的服务:
1 package com.bigbang.servlet; 2 3 import java.io.IOException; 4 import javax.servlet.ServletException; 5 import javax.servlet.http.HttpServlet; 6 import javax.servlet.http.HttpServletRequest; 7 import javax.servlet.http.HttpServletResponse; 8 9 import org.springframework.web.context.ContextLoader; 10 import org.springframework.web.context.WebApplicationContext; 11 12 import com.bigbang.common.domain.User; 13 import com.bigbang.service.UserService; 14 15 public class UserQueryServlet extends HttpServlet { 16 private static final long serialVersionUID = 1L; 17 18 private UserService userService; 19 20 /** 21 * @see HttpServlet#HttpServlet() 22 */ 23 public UserQueryServlet() { 24 super(); 25 } 26 27 /** 28 * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse 29 * response) 30 */ 31 protected void doGet(HttpServletRequest request, HttpServletResponse response) 32 throws ServletException, IOException { 33 doPost(request, response); 34 } 35 36 /** 37 * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse 38 * response) 39 */ 40 protected void doPost(HttpServletRequest request, HttpServletResponse response) 41 throws ServletException, IOException { 42 String idStr = request.getParameter("id"); 43 Long id = Long.parseLong(idStr); 44 User user = userService.getUserById(id); 45 response.getWriter().write(user.toString()); 46 } 47 48 @Override 49 public void init() throws ServletException { 50 super.init(); 51 WebApplicationContext applicationContext = ContextLoader.getCurrentWebApplicationContext(); 52 UserService userService = (UserService) applicationContext.getBean("userService"); 53 this.userService = userService; 54 System.out.println("获取userService对象完成:" + userService); 55 } 56 57 }
在servlet的init()方法中,我使用了spring类获取暴露的服务接口,实际上获取到的是dubbo创建的代理对象。
四. 运行
因为dubbo是基于长连接发送数据的,所以消息订阅者在启动时就会去查找这个认为已经注册的服务,如果不存在这个服务,则会报错。所以应该先注册服务,在开启订阅者订阅。故应该先启动Provider项目再启动Consumer项目。
一切运行成功之后,打开Consumer的index.jsp页面:
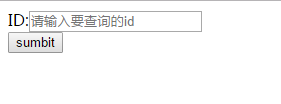
输入id点击提交之后:

说明服务调用成功,分布式作用起效了。
五. 总结
此代码仅作为抛砖引玉的作用,用来说明dubbo的基本应用。dubbo官方的文档写的很详细,从配置到说明示例。目前很多地方还没有探索到,比如启动是顺序其实可以设置服务启动时候是否检查,暴露服务的协议使用hessian还是dubbo,服务超时连接设置、服务暴露方式使用zookeeper等等。
相关文章推荐
- zookeeper+dubbo简单应用
- Dubbo 简单的应用Dubbo+Zookeeper+Spring整合
- HTA的简单应用
- BootStrap便签页的简单应用
- Tomcat4/5连接池的设置及简单应用示例
- Tomcat4/5连接池的设置及简单应用示例
- J2ME应用实例——一个简单的计算器实现(附源代码)
- 一个简单的定时器应用: VarTimer (java)
- Tomcat4/5连接池的设置及简单应用示例
- java(j2ee)应用-简单自定义标签开发全过程----之一(Inber)——JAVA夜未眠
- 有行统计项和列统计项的行列转换,以及EXCEL导入SQL的简单应用
- XML在Web中的简单应用
- EJB中JNDI的逻辑名的使用及部署_{EJB之无状态会话Bean简单应用-学习与实践}续(inber原作)
- SQLSERVER扩展存储过程XP_CMDSHELL的简单应用
- 使用C#开发一个简单的P2P应用
- [原创]用jar命令将Web应用打包成war文件的简单方法
- 使用C#开发一个简单的P2P应用
- 简单dll动态链接库的建立和应用
- Tomcat4/5连接池的设置及简单应用示例
- J2ME应用实例——一个简单的计算器实现(附源代码)