您的位置:首页
『TensorFlow』第九弹_图像预处理_不爱红妆爱武装
2017-05-27 12:18
211 查看
部分代码单独测试:
这里实践了图像大小调整的代码,值得注意的是格式问题:输入输出图像时一定要使用uint8编码,
但是数据处理过程中TF会自动把编码方式调整为float32,所以输入时没问题,输出时要手动转换回来!使用numpy.asarray(dtype)或者tf.image.convert_image_dtype(dtype)都行
都行 1 import numpy as np import tensorflow as tf import matplotlib.pyplot as plt # 使用'r'会出错,无法解码,只能以2进制形式读取 # img_raw = tf.gfile.FastGFile('./123.png','rb').read() img_raw = open('./123.png','rb').read() # 把二进制文件解码为uint8 img_0 = tf.image.decode_png(img_raw) # 不太必要了,可以用np直接转换了 # img_1 = tf.image.convert_image_dtype(img_0,dtype=tf.uint8) sess = tf.Session() print(sess.run(img_0).shape) # plt.imshow(sess.run(img_0)) # plt.show() def show_pho(img,sess=sess): ''' TF处理过的图片自动转换了类型,需要调整回uint8才能正常显示 :param sess: :param img: :return: ''' cat = np.asarray(sess.run(img),dtype='uint8') print(cat.shape) plt.imshow(cat) plt.show() '''调整图像大小''' # 插值尽量保存原图信息 img_1 = tf.image.resize_images(img_0,[500,500],method=3) # show_pho(img_1) # 裁剪或填充 # 自动中央截取 img_2 = tf.image.resize_image_with_crop_or_pad(img_0,500,500) # show_pho(img_2) # 自动四周填充[0,0,0] img_3 = tf.image.resize_image_with_crop_or_pad(img_0,2500,2500) # show_pho(sess,img_3) # 比例中央裁剪 img_4 = tf.image.central_crop(img_0,0.5) # show_pho(img_4) # 画框裁剪 # {起点高度,起点宽度,框高,框宽} img_5 = tf.image.crop_to_bounding_box(img_0,700,300,500,500) show_pho(img_5)
完整的图像预处理函数:
处理单张图片import tensorflow as tf import numpy as np # import matplotlib.pyplot as plt def distort_color(image, color_ordering=0): ''' 随机调整图片的色彩,定义两种处理顺序。 注意,对3通道图像正常,4通道图像会出错,自行先reshape之 :param image: :param color_ordering: :return: ''' if color_ordering == 0: image = tf.image.random_brightness(image, max_delta=32./255.) image = tf.image.random_saturation(image, lower=0.5, upper=1.5) image = tf.image.random_hue(image, max_delta=0.2) image = tf.image.random_contrast(image, lower=0.5, upper=1.5) else: image = tf.image.random_saturation(image, lower=0.5, upper=1.5) image = tf.image.random_brightness(image, max_delta=32./255.) image = tf.image.random_contrast(image, lower=0.5, upper=1.5) image = tf.image.random_hue(image, max_delta=0.2) return tf.clip_by_value(image, 0.0, 1.0) def preprocess_for_train(image, height, width, bbox): ''' 对图片进行预处理,将图片转化成神经网络的输入层数据。 :param image: :param height: :param width: :param bbox: :return: ''' # 查看是否存在标注框。 if image.dtype != tf.float32: image = tf.image.convert_image_dtype(image, dtype=tf.float32) # 随机的截取图片中一个块。 bbox_begin, bbox_size, _ = tf.image.sample_distorted_bounding_box( tf.shape(image), bounding_boxes=bbox) bbox_begin, bbox_size, _ = tf.image.sample_distorted_bounding_box( tf.shape(image), bounding_boxes=bbox) distorted_image = tf.slice(image, bbox_begin, bbox_size) # 将随机截取的图片调整为神经网络输入层的大小。 distorted_image = tf.image.resize_images(distorted_image, [height, width], method=np.random.randint(4)) distorted_image = tf.image.random_flip_left_right(distorted_image) distorted_image = distort_color(distorted_image, np.random.randint(2)) return distorted_image def pre_main(img,bbox=None): if bbox is None: bbox = tf.constant([0.0, 0.0, 1.0, 1.0], dtype=tf.float32, shape=[1, 1, 4]) with tf.gfile.FastGFile(img, "rb") as f: image_raw_data = f.read() with tf.Session() as sess: img_data = tf.image.decode_jpeg(image_raw_data) for i in range(9): result = preprocess_for_train(img_data, 299, 299, bbox) # {wb打开文件{矩阵编码为jpeg{格式转换为uint8}}.eval()} with tf.gfile.FastGFile('./代号{}.jpeg'.format(i),'wb') as f: f.write(sess.run(tf.image.encode_jpeg(tf.image.convert_image_dtype(result,dtype=tf.uint8)))) # plt.imshow(result.eval()) # plt.axis('off') # plt.savefig('代号{}'.format(i)) if __name__=='__main__': pre_main("./123123.jpeg",bbox=None) exit()
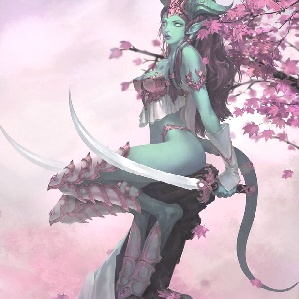

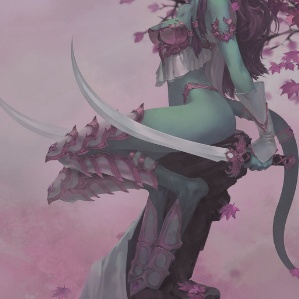


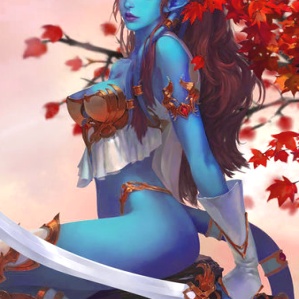


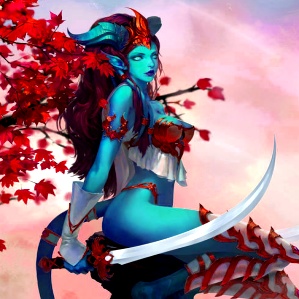
相关文章推荐
- TensorFlow图像数据预处理
- tensorflow图像数据预处理
- TensorFlow 图像数据预处理及可视化
- TensorFlow 图像预处理(二) 图像翻转,图像色彩调整
- Tensorflow中图像的预处理
- 《慢慢学TensorFlow》微信公众号之TensorFlow 图像数据预处理及可视化
- TensorFlow 图像数据预处理及可视化
- TensorFlow 图像数据预处理及可视化
- TensorFlow 图像数据预处理及可视化
- TensorFlow 图像数据预处理及可视化
- TensorFlow 图像预处理(一) 图像编解码,图像尺寸调整
- 图像的预处理——tensorflow实践
- tensorflow图像分类实战解析(下)
- Tensorflow 图像处理函数
- 图像预处理——二值化(大律法)
- 使用TensorFlow-Slim进行图像分类
- 简单图像分类与识别CNN,Tensorflow,Cifar10(吴恩达Deep Learning)
- 人脸图像的预处理
- Tensorflow之构建自己的图像分类器
- ML 图像预处理工具