LeetCode 题解 169. Majority Element(查找多数元素)
2017-04-28 16:23
656 查看
(尊重劳动成果,转载请注明出处:http://blog.csdn.net/qq_25827845/article/details/70917827冷血之心的博客)
开始在LeetCode上多学习别人的算法,加油~~~
题目链接地址:https://leetcode.com/problems/majority-element/#/description
题目描述:
Given an array of size n, find the majority element. The majority element is the element that appears more than
解析: 本题的意思是让你找出一个数组中占50%以上的元素,即寻找多数元素,并且多数元素是一定存在的假设。
思路1:将数组排序,则中间的那个元素一定是多数元素
public int majorityElement(int[] nums) {
Arrays.sort(nums);
return nums[nums.length/2];
}
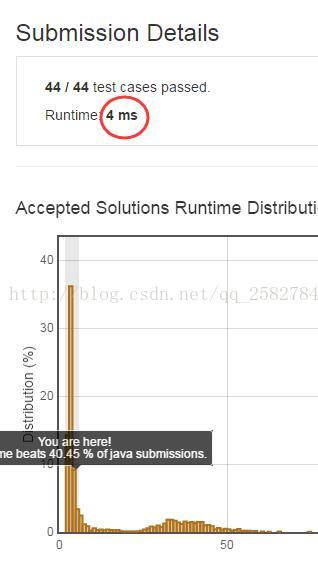
思路2:利用HashMap来记录每个元素的出现次数
public int majorityElement(int[] nums) {
HashMap<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
if(!map.containsKey(nums[i])){
map.put(nums[i], 1);
}else {
int values = map.get(nums[i]);
map.put(nums[i], ++values);
}
}
int n = nums.length/2;
Set<Integer> keySet = map.keySet();
Iterator<Integer> iterator = keySet.iterator();
while(iterator.hasNext()){
int key = iterator.next();
int value = map.get(key);
if (value>n) {
return key;
}
}
return 0;
}
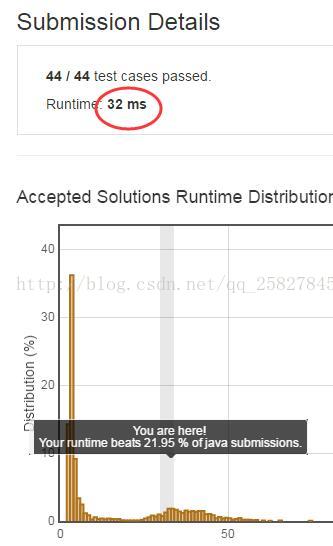
思路3:Moore voting algorithm--每找出两个不同的element,就成对删除即count--,最终剩下的一定就是所求的。时间复杂度:O(n)
public int majorityElement(int[] nums) {
int elem = 0;
int count = 0;
for(int i = 0; i < nums.length; i++) {
if(count == 0) {
elem = nums[i];
count = 1;
}
else {
if(elem == nums[i])
count++;
else
count--;
}
}
return elem;
}
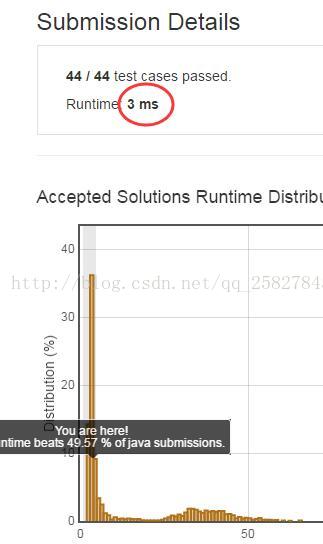
思路4:位运算
public int majorityElement(int[] nums) {
int[] bit = new int[32];
for (int num: nums)
for (int i=0; i<32; i++)
if ((num>>(31-i) & 1) == 1)
bit[i]++;
int ret=0;
for (int i=0; i<32; i++) {
bit[i]=bit[i]>nums.length/2?1:0;
ret += bit[i]*(1<<(31-i));
}
return ret;
}
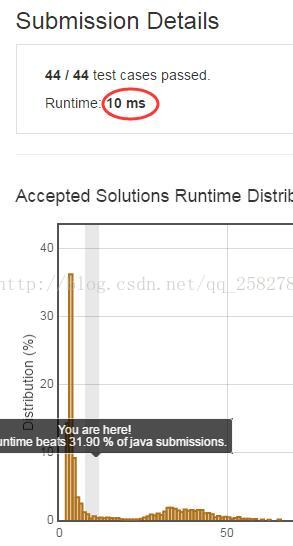
以下是leetcode解题思路:
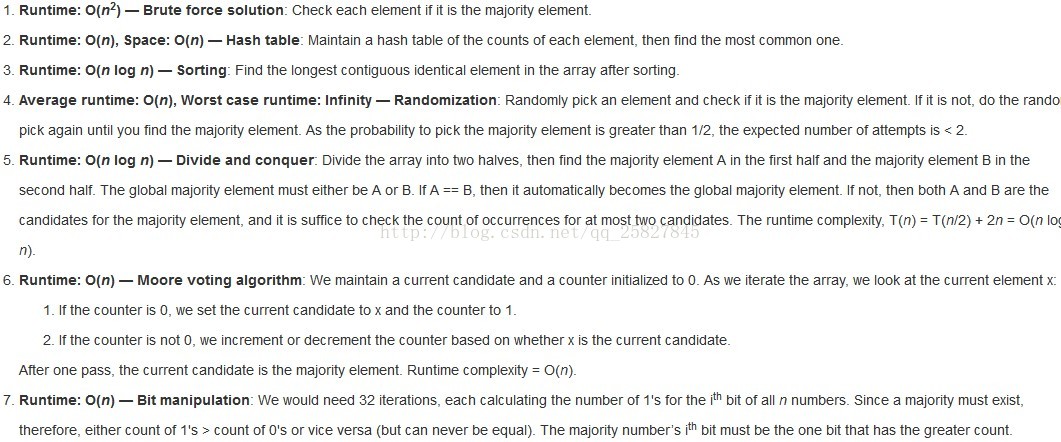
可以看到每个算法的运行时间,而我设计的算法往往是运行时间贼大的那个(HashMap解法),唉,以后多学学别人的高明解法,别动不动就用各种集合,复杂度太高了。不好使不好使

如果对你有帮助,记得点赞哦~欢迎大家关注我的博客,可以进群366533258一起交流学习哦~
开始在LeetCode上多学习别人的算法,加油~~~
题目链接地址:https://leetcode.com/problems/majority-element/#/description
题目描述:
Given an array of size n, find the majority element. The majority element is the element that appears more than
⌊ n/2 ⌋times. You may assume that the array is non-empty and the majority element always exist in the array.
解析: 本题的意思是让你找出一个数组中占50%以上的元素,即寻找多数元素,并且多数元素是一定存在的假设。
思路1:将数组排序,则中间的那个元素一定是多数元素
public int majorityElement(int[] nums) {
Arrays.sort(nums);
return nums[nums.length/2];
}
思路2:利用HashMap来记录每个元素的出现次数
public int majorityElement(int[] nums) {
HashMap<Integer, Integer> map = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
if(!map.containsKey(nums[i])){
map.put(nums[i], 1);
}else {
int values = map.get(nums[i]);
map.put(nums[i], ++values);
}
}
int n = nums.length/2;
Set<Integer> keySet = map.keySet();
Iterator<Integer> iterator = keySet.iterator();
while(iterator.hasNext()){
int key = iterator.next();
int value = map.get(key);
if (value>n) {
return key;
}
}
return 0;
}
思路3:Moore voting algorithm--每找出两个不同的element,就成对删除即count--,最终剩下的一定就是所求的。时间复杂度:O(n)
public int majorityElement(int[] nums) {
int elem = 0;
int count = 0;
for(int i = 0; i < nums.length; i++) {
if(count == 0) {
elem = nums[i];
count = 1;
}
else {
if(elem == nums[i])
count++;
else
count--;
}
}
return elem;
}
思路4:位运算
public int majorityElement(int[] nums) {
int[] bit = new int[32];
for (int num: nums)
for (int i=0; i<32; i++)
if ((num>>(31-i) & 1) == 1)
bit[i]++;
int ret=0;
for (int i=0; i<32; i++) {
bit[i]=bit[i]>nums.length/2?1:0;
ret += bit[i]*(1<<(31-i));
}
return ret;
}
以下是leetcode解题思路:
可以看到每个算法的运行时间,而我设计的算法往往是运行时间贼大的那个(HashMap解法),唉,以后多学学别人的高明解法,别动不动就用各种集合,复杂度太高了。不好使不好使

如果对你有帮助,记得点赞哦~欢迎大家关注我的博客,可以进群366533258一起交流学习哦~
相关文章推荐
- LeetCode Problem 169: Majority Element查找多数元素
- [LeetCode]169. Majority Element(多数元素)
- 169. Majority Element 查找多数元素
- LeetCode-169:Majority Element (数组中的多数元素)
- [LeetCode] 169. Majority Element 多数元素
- Leetcode:169. Majority Element(找到数组中出现次数最多的元素)
- leetcode 169. Majority Element 多数投票算法(Boyer-Moore Majority Vote algorithm)
- 字符串算法——查找数组多数元素(Majority Element II)
- 【LeetCode题解】169_求众数(Majority-Element)
- LeetCode 169. Majority Element (数组的主要元素、摩尔投票算法)
- 169. Majority Element (寻找多数元素)
- 【LeetCode】169. Majority Element (多数投票算法 & 算法迁移能力)
- Leetcode题解 169. Majority Element
- leetcode题解-169. Majority Element && 189. Rotate Array
- LeetCode - 169. Majority Element
- 小白笔记-------------------------------leetcode(169. Majority Element)
- 2018.03.07 leetcode 打卡 #169. Majority Element
- 2018.03.07 leetcode 打卡 #169. Majority Element
- LeetCode 169. Majority Element(Python)
- LeetCode.169(229) Majority Element && II