Android app更新版本
2017-04-21 09:47
337 查看
Android APP检测更新新版本是一个常见的问题
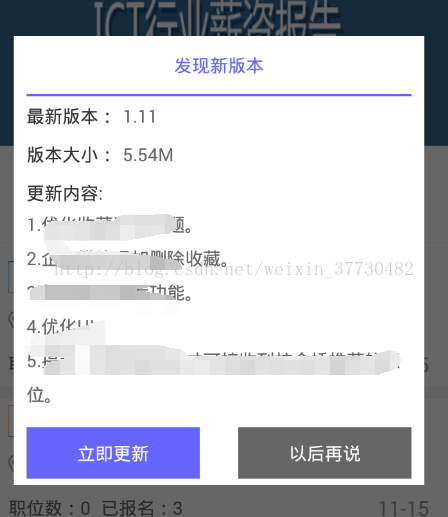
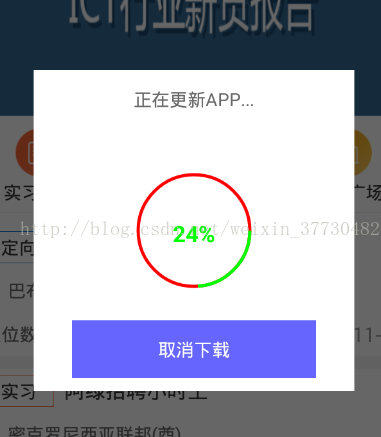
图片1对应的代码
package com.xiaoqiqiao.bridgebetweencollegesstudent.mywidget;
import android.annotation.SuppressLint;
import android.app.Dialog;
import android.content.Context;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
import com.xiaoqiqiao.bridgebetweencollegesstudent.R;
/**
* 自定义对话框 更新软件
* */
public class UpdateAppDialog extends Dialog implements OnClickListener{
private TextView versiontv;//最新版本
private TextView sizetv;//最新版本大小
private TextView updatetv;//更新内容
private String version;//最新版本
private String size;//版本大小
private String update;//更新内容
private Button update_btn;//立即更新
private Button cancal_btn;//稍后更新
private LoadAppDialog load;//自定义下载app对话框
private String url;//下载apk的url
private Context context;
public UpdateAppDialog(Context context) {
super(context);
this.context=context;
}
public UpdateAppDialog(Context context,int theme,String url,String version,String size,String update) {
super(context, theme);
this.context=context;
this.url=url;
this.version=version;
this.size=size;
this.update=update;
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.updateapp_dialog);
//调用初始化各种view的方法
initView();
}
/**
* 初始化各种view的方法
* */
public void initView(){
versiontv=(TextView) findViewById(R.id.updateapp_versiontv);
sizetv=(TextView) findViewById(R.id.updateapp_sizetv);
updatetv=(TextView) findViewById(R.id.updateapp_updatetv);
update_btn=(Button) findViewById(R.id.updateapp_surebtn);
update_btn.setOnClickListener(this);
cancal_btn=(Button) findViewById(R.id.updateapp_cancelbtn);
cancal_btn.setOnClickListener(this);
if("".equals(version)||"null".equals(version)||null==version){
versiontv.setText("不详");
}else{
versiontv.setText(version);
}
if("".equals(size)||"null".equals(size)||null==size){
sizetv.setText("不详");
}else{
sizetv.setText(size);
}
if("".equals(update)||"null".equals(update)||null==update){
updatetv.setText("不详");
}else{
updatetv.setText(update);
}
}
/**
* 各种点击事件
* */
@SuppressLint("ShowToast")
@Override
public void onClick(View v) {
switch(v.getId()){
case R.id.updateapp_surebtn://立即更新
load=new LoadAppDialog(context, R.style.Dialog,url);
if(load!=null){//显示下载对话框
load.show();
}
this.dismiss();
break;
case R.id.updateapp_cancelbtn://稍后更新
this.dismiss();
break;
}
}
}
图片2对应的代码
package com.xiaoqiqiao.bridgebetweencollegesstudent.mywidget;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import android.annotation.SuppressLint;
import android.app.Dialog;
import android.content.Context;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import com.xiaoqiqiao.bridgebetweencollegesstudent.R;
/**
* 自定义对话框 下载软件
* */
public class LoadAppDialog extends Dialog implements OnClickListener{
private RoundProgressBar pb;//下载进度条
private Button cancelbtn;//取消下载按钮
private static final int DOWNLOAD = 1;//下载中
private static final int DOWNLOAD_FINISH = 2;//下载结束
private boolean cancelUpdate = false;
private String Url;//下载url
private String mSavePath;//下载保存路径
private int progress;//下载进度条数量
private Context context;
public LoadAppDialog(Context context) {
super(context);
this.context=context;
}
public LoadAppDialog(Context context,int theme,String url) {
super(context, theme);
this.context=context;
this.Url=url;
}
/**
*Handler机制
* */
@SuppressLint("HandlerLeak")
private Handler mHandler = new Handler() {
public void handleMessage(Message msg) {
switch (msg.what) {
//正在下载
case DOWNLOAD:
//设置进度条位置
pb.setProgress(progress);
break;
case DOWNLOAD_FINISH:
//安装文件
installApk();
break;
default:
break;
}
};
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.updateapp_progress);
pb=(RoundProgressBar) findViewById(R.id.loadapp_pb);
cancelbtn=(Button) findViewById(R.id.update_btn);
cancelbtn.setOnClickListener(this);
downloadApk();//下载app
}
/**
* 下载apk文件
*/
private void downloadApk() {
//启动新线程下载软件
new downloadApkThread().start();
}
/**
* 下载文件线程
*/
private class downloadApkThread extends Thread {
@Override
public void run() {
try {
//判断SD卡是否存在,并且是否具有读写权限
if(Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
//获得存储卡的路径
String sdpath=Environment.getExternalStorageDirectory()+ "/";
mSavePath=sdpath + "bridgebetweencollegesstudent";
URL url = new URL(Url);
// 创建连接
HttpURLConnection conn=(HttpURLConnection) url.openConnection();
conn.connect();
//获取文件大小
int length=conn.getContentLength();
//创建输入流
InputStream is=conn.getInputStream();
File file=new File(mSavePath);
//判断文件目录是否存在
if(!file.exists()){
file.mkdir();
}
File apkFile=new File(mSavePath, "bridgebetweencollegesstudent.apk");
FileOutputStream fos=new FileOutputStream(apkFile);
int count=0;
//缓存
byte buf[]=new byte[1024];
//写入到文件中
do {
int numread=is.read(buf);
count+=numread;
//计算进度条位置
progress = (int) (((float) count / length) * 100);
//更新进度
mHandler.sendEmptyMessage(DOWNLOAD);
if(numread <= 0){
//下载完成
mHandler.sendEmptyMessage(DOWNLOAD_FINISH);
break;
}
//写入文件
fos.write(buf, 0, numread);
} while (!cancelUpdate);//点击取消就停止下载.
fos.close();
is.close();
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
LoadAppDialog.this.dismiss();
}
};
/**
* 安装APK文件
*/
private void installApk() {
File apkfile = new File(mSavePath, "bridgebetweencollegesstudent.apk");
if (!apkfile.exists()) {
return;
}
// 通过Intent安装APK文件
Intent i = new Intent(Intent.ACTION_VIEW);
i.setDataAndType(Uri.parse("file://" + apkfile.toString()),"application/vnd.android.package-archive");
context.startActivity(i);
}
/**
* 各种点击事件的方法
* */
@Override
public void onClick(View v) {
switch(v.getId()){
case R.id.update_btn://取消下载
cancelUpdate=true;//设置取消状态
LoadAppDialog.this.dismiss();
break;
}
}
}
知识点1.
//判断SD卡是否存在,并且是否具有读写权限
if(Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
//获得存储卡的路径
String sdpath=Environment.getExternalStorageDirectory()+ "/";
mSavePath=sdpath + "bridgebetweencollegesstudent";
URL url = new URL(Url);
// 创建连接
HttpURLConnection conn=(HttpURLConnection) url.openConnection();
conn.connect();
//获取文件大小
int length=conn.getContentLength();
//创建输入流
InputStream is=conn.getInputStream();
File file=new File(mSavePath);
//判断文件目录是否存在
if(!file.exists()){
file.mkdir();
}
File apkFile=new File(mSavePath, "bridgebetweencollegesstudent.apk");
FileOutputStream fos=new FileOutputStream(apkFile);
int count=0;
//缓存
byte buf[]=new byte[1024];
//写入到文件中
do {
int numread=is.read(buf);
count+=numread;
//计算进度条位置
progress = (int) (((float) count / length) * 100);
//更新进度
mHandler.sendEmptyMessage(DOWNLOAD);
if(numread <= 0){
//下载完成
mHandler.sendEmptyMessage(DOWNLOAD_FINISH);
break;
}
//写入文件
fos.write(buf, 0, numread);
} while (!cancelUpdate);//点击取消就停止下载.
fos.close();
is.close();
}
知识点2
/**
* 安装APK文件
*/
private void installApk() {
File apkfile = new File(mSavePath, "bridgebetweencollegesstudent.apk");
if (!apkfile.exists()) {
return;
}
// 通过Intent安装APK文件
Intent i = new Intent(Intent.ACTION_VIEW);
i.setDataAndType(Uri.parse("file://" + apkfile.toString()),"application/vnd.android.package-archive");
context.startActivity(i);
}
图片1对应的代码
package com.xiaoqiqiao.bridgebetweencollegesstudent.mywidget;
import android.annotation.SuppressLint;
import android.app.Dialog;
import android.content.Context;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
import com.xiaoqiqiao.bridgebetweencollegesstudent.R;
/**
* 自定义对话框 更新软件
* */
public class UpdateAppDialog extends Dialog implements OnClickListener{
private TextView versiontv;//最新版本
private TextView sizetv;//最新版本大小
private TextView updatetv;//更新内容
private String version;//最新版本
private String size;//版本大小
private String update;//更新内容
private Button update_btn;//立即更新
private Button cancal_btn;//稍后更新
private LoadAppDialog load;//自定义下载app对话框
private String url;//下载apk的url
private Context context;
public UpdateAppDialog(Context context) {
super(context);
this.context=context;
}
public UpdateAppDialog(Context context,int theme,String url,String version,String size,String update) {
super(context, theme);
this.context=context;
this.url=url;
this.version=version;
this.size=size;
this.update=update;
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.updateapp_dialog);
//调用初始化各种view的方法
initView();
}
/**
* 初始化各种view的方法
* */
public void initView(){
versiontv=(TextView) findViewById(R.id.updateapp_versiontv);
sizetv=(TextView) findViewById(R.id.updateapp_sizetv);
updatetv=(TextView) findViewById(R.id.updateapp_updatetv);
update_btn=(Button) findViewById(R.id.updateapp_surebtn);
update_btn.setOnClickListener(this);
cancal_btn=(Button) findViewById(R.id.updateapp_cancelbtn);
cancal_btn.setOnClickListener(this);
if("".equals(version)||"null".equals(version)||null==version){
versiontv.setText("不详");
}else{
versiontv.setText(version);
}
if("".equals(size)||"null".equals(size)||null==size){
sizetv.setText("不详");
}else{
sizetv.setText(size);
}
if("".equals(update)||"null".equals(update)||null==update){
updatetv.setText("不详");
}else{
updatetv.setText(update);
}
}
/**
* 各种点击事件
* */
@SuppressLint("ShowToast")
@Override
public void onClick(View v) {
switch(v.getId()){
case R.id.updateapp_surebtn://立即更新
load=new LoadAppDialog(context, R.style.Dialog,url);
if(load!=null){//显示下载对话框
load.show();
}
this.dismiss();
break;
case R.id.updateapp_cancelbtn://稍后更新
this.dismiss();
break;
}
}
}
图片2对应的代码
package com.xiaoqiqiao.bridgebetweencollegesstudent.mywidget;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import android.annotation.SuppressLint;
import android.app.Dialog;
import android.content.Context;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import com.xiaoqiqiao.bridgebetweencollegesstudent.R;
/**
* 自定义对话框 下载软件
* */
public class LoadAppDialog extends Dialog implements OnClickListener{
private RoundProgressBar pb;//下载进度条
private Button cancelbtn;//取消下载按钮
private static final int DOWNLOAD = 1;//下载中
private static final int DOWNLOAD_FINISH = 2;//下载结束
private boolean cancelUpdate = false;
private String Url;//下载url
private String mSavePath;//下载保存路径
private int progress;//下载进度条数量
private Context context;
public LoadAppDialog(Context context) {
super(context);
this.context=context;
}
public LoadAppDialog(Context context,int theme,String url) {
super(context, theme);
this.context=context;
this.Url=url;
}
/**
*Handler机制
* */
@SuppressLint("HandlerLeak")
private Handler mHandler = new Handler() {
public void handleMessage(Message msg) {
switch (msg.what) {
//正在下载
case DOWNLOAD:
//设置进度条位置
pb.setProgress(progress);
break;
case DOWNLOAD_FINISH:
//安装文件
installApk();
break;
default:
break;
}
};
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.updateapp_progress);
pb=(RoundProgressBar) findViewById(R.id.loadapp_pb);
cancelbtn=(Button) findViewById(R.id.update_btn);
cancelbtn.setOnClickListener(this);
downloadApk();//下载app
}
/**
* 下载apk文件
*/
private void downloadApk() {
//启动新线程下载软件
new downloadApkThread().start();
}
/**
* 下载文件线程
*/
private class downloadApkThread extends Thread {
@Override
public void run() {
try {
//判断SD卡是否存在,并且是否具有读写权限
if(Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
//获得存储卡的路径
String sdpath=Environment.getExternalStorageDirectory()+ "/";
mSavePath=sdpath + "bridgebetweencollegesstudent";
URL url = new URL(Url);
// 创建连接
HttpURLConnection conn=(HttpURLConnection) url.openConnection();
conn.connect();
//获取文件大小
int length=conn.getContentLength();
//创建输入流
InputStream is=conn.getInputStream();
File file=new File(mSavePath);
//判断文件目录是否存在
if(!file.exists()){
file.mkdir();
}
File apkFile=new File(mSavePath, "bridgebetweencollegesstudent.apk");
FileOutputStream fos=new FileOutputStream(apkFile);
int count=0;
//缓存
byte buf[]=new byte[1024];
//写入到文件中
do {
int numread=is.read(buf);
count+=numread;
//计算进度条位置
progress = (int) (((float) count / length) * 100);
//更新进度
mHandler.sendEmptyMessage(DOWNLOAD);
if(numread <= 0){
//下载完成
mHandler.sendEmptyMessage(DOWNLOAD_FINISH);
break;
}
//写入文件
fos.write(buf, 0, numread);
} while (!cancelUpdate);//点击取消就停止下载.
fos.close();
is.close();
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
LoadAppDialog.this.dismiss();
}
};
/**
* 安装APK文件
*/
private void installApk() {
File apkfile = new File(mSavePath, "bridgebetweencollegesstudent.apk");
if (!apkfile.exists()) {
return;
}
// 通过Intent安装APK文件
Intent i = new Intent(Intent.ACTION_VIEW);
i.setDataAndType(Uri.parse("file://" + apkfile.toString()),"application/vnd.android.package-archive");
context.startActivity(i);
}
/**
* 各种点击事件的方法
* */
@Override
public void onClick(View v) {
switch(v.getId()){
case R.id.update_btn://取消下载
cancelUpdate=true;//设置取消状态
LoadAppDialog.this.dismiss();
break;
}
}
}
知识点1.
//判断SD卡是否存在,并且是否具有读写权限
if(Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) {
//获得存储卡的路径
String sdpath=Environment.getExternalStorageDirectory()+ "/";
mSavePath=sdpath + "bridgebetweencollegesstudent";
URL url = new URL(Url);
// 创建连接
HttpURLConnection conn=(HttpURLConnection) url.openConnection();
conn.connect();
//获取文件大小
int length=conn.getContentLength();
//创建输入流
InputStream is=conn.getInputStream();
File file=new File(mSavePath);
//判断文件目录是否存在
if(!file.exists()){
file.mkdir();
}
File apkFile=new File(mSavePath, "bridgebetweencollegesstudent.apk");
FileOutputStream fos=new FileOutputStream(apkFile);
int count=0;
//缓存
byte buf[]=new byte[1024];
//写入到文件中
do {
int numread=is.read(buf);
count+=numread;
//计算进度条位置
progress = (int) (((float) count / length) * 100);
//更新进度
mHandler.sendEmptyMessage(DOWNLOAD);
if(numread <= 0){
//下载完成
mHandler.sendEmptyMessage(DOWNLOAD_FINISH);
break;
}
//写入文件
fos.write(buf, 0, numread);
} while (!cancelUpdate);//点击取消就停止下载.
fos.close();
is.close();
}
知识点2
/**
* 安装APK文件
*/
private void installApk() {
File apkfile = new File(mSavePath, "bridgebetweencollegesstudent.apk");
if (!apkfile.exists()) {
return;
}
// 通过Intent安装APK文件
Intent i = new Intent(Intent.ACTION_VIEW);
i.setDataAndType(Uri.parse("file://" + apkfile.toString()),"application/vnd.android.package-archive");
context.startActivity(i);
}
相关文章推荐
- Android(2)—Mono For Android App版本自动更新
- Android 轻松实现后台搭建+APP版本更新
- Android 轻松实现后台搭建+APP版本更新
- Android 轻松实现后台搭建+APP版本更新
- Android实现APP版本自动更新功能
- Android(3)—Mono For Android App版本自动更新(2)
- Android中实现app版本更新
- Android 轻松实现后台搭建+APP版本更新
- Android如何更新app的版本(中级)
- Android App 版本更新实现
- app 版本更新的时候,android开发人员需要的工作
- android app版本更新简介
- Android 轻松实现后台搭建+APP版本更新
- android的APP版本更新工具类(下载并安装)
- Android APP 版本更新通知代码
- Android如何更新app的版本
- Android studio appcompat-v7 更新23.0.x版本后出现问题
- Android 轻松实现后台搭建+APP版本更新
- android app版本更新升级
- android 自动更新APP版本,并使用ProgressDialog显示进度