Laravel5.1 与 Laypage 结合进行分页
2017-04-03 23:53
288 查看
demo地址:http://lara.ytlwin.top/orm
路由
控制器
视图层
效果图
路由
Route::match(array('get','post'),'/orm','StuController@orm');
控制器
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Http\Requests; use App\User; //user表的模型 use App\Phone; //phone表的模型 class StuController extends Controller { /* *@brief 搜索 分页 */ private $current_page; //私有属性 当前页 private $page_size = 5; //私有属性 每页条数 public function __construct(Request $request) { $this->current_page = $request->input('current_page', 1); } public function orm(Request $request) { $search_text = $request->input('search_text',''); $pay_type = intval($request->input('pay_type',6)); if($search_text == '') { $count = User::join('phone','phone.user_id','=','user.user_id','left')->count(); $page_count = ceil($count/$this->page_size); // dd($page_count); $data = User::join('phone','phone.user_id','=','user.user_id','left')->get(); }else{ // 关联 $count = User::join('phone','phone.user_id','=','user.user_id','left')->with('phone')->where('username','like','%'.$search_text.'%')->count(); $page_count = ceil($count/$this->page_size); $data = User::join('phone','phone.user_id','=','user.user_id','left')->with('phone')->where('username','like','%'.$search_text.'%')->get(); } if($pay_type !== 6 ) { $data = collect($data) -> where('pay_type',$pay_type); //对已有数据进行处理支付类型筛选 } $count = $data->count(); //多少数据 $page_count = ceil($count/$this->page_size); //多少页 //collect和slice切片选择页数内容 $data = collect($data)->slice(($this->current_page -1)*$this->page_size,$this->page_size); return view('phone',compact('data','search_text','pay_type')) ->with('current_page', $this->current_page) ->with('page_count', $page_count); } }
视图层
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>电话联系人</title> <script type="text/javascript" src="{{asset('/jquery.min.js')}}"></script> <script type="text/javascript" src="{{asset('/laypage/laypage.js')}}"></script> <script type="text/javascript" src="{{asset('/layer/layer.js')}}"></script> </head> <body> <input type="text" name="search_text" id="search_text" placeholder="请输入姓名搜索" @if($search_text != '') value="{{$search_text}} @endif"><button name="search_btn" id="search_btn" type="submit">搜索</button> <span> <select name="pay_type" id="pay_type" onchange="pay()"> <option value="6" @if($pay_type==6)selected="selected" @endif>全部</option> <option value="0" @if($pay_type==0)selected="selected" @endif>在线支付</option> <option value="1" @if($pay_type==1)selected="selected" @endif>快递支付</option> </select> </span> <div style="height:15px;"></div> <table> <thead> <tr> <th width="40">选择</th> <th width="40">序号</th> <th width="40">姓名</th> <th width="60">手机</th> <th width="80">支付方式</th> <th width="80">发货状态</th> </tr> </thead> @foreach($data as $key=>$d) <tbody> <tr> <th width="40"><input type="checkbox" name="like[]"></th> <th width="40">{{$key+1}}</th> <th width="40">{{$d->username}}</th> <th width="60">{{$d->phone}}</th> <th width="120"> @if($d->pay_type==0) 在线支付 @endif @if($d->pay_type==1) 快递到付 @endif </th> <th width="60"> @if($d->is_send==0) 未发 @endif @if($d->is_send==1) 已发 @endif </th> </tr> </tbody> @endforeach </table> <div class="row cl" style="margin-top: 20px;float:left;" > <label class="form-label col-1 text-l" id="record">共 {{$page_count}} 页</label> <div class="formControls col-11 text-r" id="page" style="float:left;"> </div> </div> <script type="text/javascript"> // alert($); var search_btn = $('#search_btn'); var search_text = $('#search_text'); var pay_type = ''; $(function(){ if (parseInt("{{$page_count}}") > 0) { pay_type = $('#pay_type option:selected').val(); laypage({ cont: 'page', pages: '{{$page_count}}', //可以叫服务端把总页数放在某一个隐藏域,再获取。假设我们获取到的是18 curr: function() { //通过url获取当前页,也可以同上(pages)方式获取 var page = "{{$current_page}}"; return page; }(), jump: function(e, first) { //触发分页后的回调 if (!first) { //一定要加此判断,否则初始时会无限刷新 location.href = GetUrlRelativePath() + '?search_text=' + search_text.val().trim() + '&pay_type=' + pay_type + '¤t_page=' + e.curr; } } }); } }); search_btn.click(function(){ pay_type = $('#pay_type option:selected').val(); location.href = GetUrlRelativePath() + '?search_text=' + search_text.val().trim()+ '&pay_type=' + pay_type; //重定向到地址 带参数进行查询 }); function pay() { pay_type = $('#pay_type option:selected').val(); location.href = GetUrlRelativePath() + '?search_text=' + search_text.val().trim() + '&pay_type=' + pay_type; } console.log(GetUrlRelativePath()); function GetUrlRelativePath() { var url = document.location.toString(); //得出当前地址 var arrUrl = url.split("//"); //以//为标识 分割为两个数组 [http:,haha.com/orm] var start = arrUrl[1].indexOf("/"); // 下标为1 的即为去除http头的 域名部分 indexOf('') 某个字符首次出现的位置 从0开始计数 / 在第8 var relUrl = arrUrl[1].substring(start); //substring(n) 去除0-(n-1) 的字符串 if(relUrl.indexOf("?") != -1) { relUrl = relUrl.split("?")[0]; //去除参数 } return relUrl; } //得出地址 </script> </body> </html>
效果图
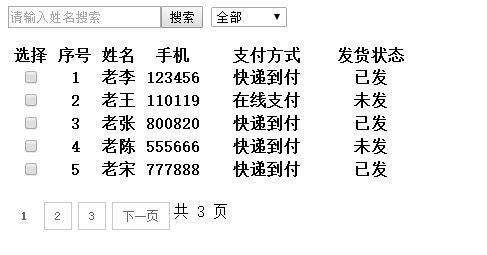
相关文章推荐
- TP3.23 与Laypage 结合进行分页
- jfinal结合layPage进行分页查询操作
- page-taglib标签结合SSH框架进行Java分页
- 使用Laypage进行分页
- page-taglib标签结合SSH框架进行java分页
- Silverlight中DataGrid控件动态生成列并结合DataPager进行分页
- spring boot 整合mybatis分页插件pagehelper5.1
- Oracle通用分页存储过程和AspNetPage结合
- Mybatis插件原理和PageHelper结合实战分页插件
- 使用laravel5.4结合easywechat进行微信开发--基本配置
- laravel5.1 jquery ajax 搜索分页
- lucene.net 3.0.3、结合盘古分词进行搜索的小例子(分页功能)
- laypage分页完整案例
- laypage.js分页插件使用总结
- mybatis使用pageHelper插件进行查询分页
- datalist结合PagedDataSource 类进行分页
- Yii2 使DataProvider分页时,page超过最大页数不进行处理
- JS:layPage:一款多功能的js分页组件
- MyBatis(七)——使用PageHelper插件进行分页
- 拿来主义:layPage分页插件的使用