python自动化--模块操作之re、MySQL、Excel
2017-03-17 13:28
726 查看
一、python自有模块正则
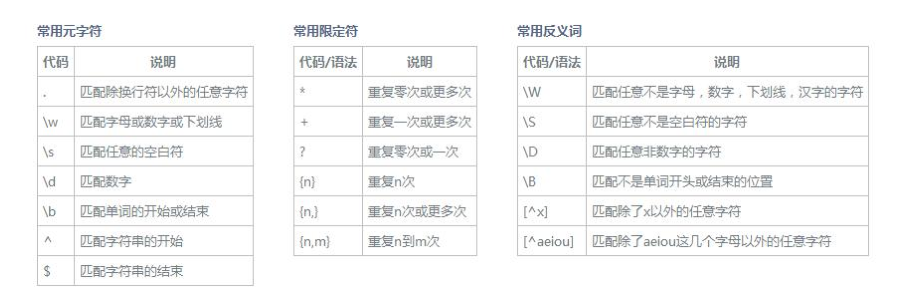
二、python第三方模块操作MySQL
连接数据库:
插入数据:
删除数据:
修改数据:
查询数据:
三、python第三方模块操作Excel
注意高能:openpyxl只能操作xlsx文件而不能操作xls文件!所以在创建的时候一定要新建.xlsx格式的Excel!!!
小练习:
1、Python里面match()和search()的区别?
2、以<div><span>test</span></div>进行匹配
3、获取URL的后缀名
1 import re 2 3 # re.match只匹配字符串的开始,如果字符串开始不符合正则表达式,则匹配失败,函数返回None 4 print(re.match("www","wwwwccc").group()) #在起始位置匹配 5 print(re.match("www","wcccwww")) #不在起始位置匹配,返回None 6 7 # re.search扫描整个字符串并返回第一个成功的匹配 8 print(re.search("haha","woshihahanishishui").group()) 9 10 # re.findall从左到右扫描字符串,按顺序返回匹配,如果无匹配结果则返回空列表 11 print(re.findall("\d","one1two2three3four4")) 12 print(re.findall("\d","onetwothreefour")) 13 14 # sub用于替换字符串中的匹配项 15 print(re.sub("g..t","good","goot geet up")) 16 # split返回切割后的列表 17 print(re.split("\+","123+456*789+abcd"))
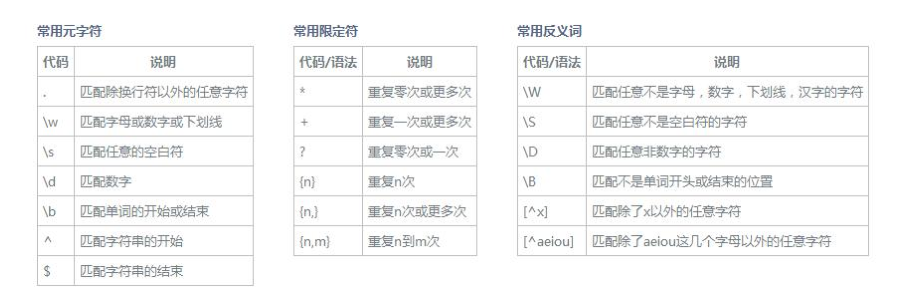
1 # ['AD123453', 'AC342123', 'AR343212'] 2 # [A-Z]{2}\d{6} 3 4 # ^(13|14|15|17|18)\d{9} 5 # ^1[34578]\d{9}
二、python第三方模块操作MySQL
连接数据库:
1 import mysql.connector 2 config = { 3 "host":"127.0.0.1", 4 "user":"root", 5 "passwd":"vertrigo", 6 "port":3306, 7 "db":"test", 8 "charset":"utf8" 9 } 10 11 try: 12 db = mysql.connector.connect(**config) 13 except mysql.connector.Error as e: 14 print("连接数据库失败!",str(e))
插入数据:
1 2 cursor = db.cursor(buffered=True) #buffered=True会把结果集保存到本地并一次性返回,这样可以提高性能 3 try: 4 #第一种:直接字符串插入方式 5 # sql_insert1="insert into student (name, age) values ('xiao', 27)" 6 # cursor.execute(sql_insert1) 7 8 #第二种:元组连接插入方式 9 sql_insert2="insert into student (name, age) values (%s, %s)" 10 #此处的%s为占位符,而不是格式化字符串,所以age用%s 11 # data=('xiaoqiang',18) 12 # cursor.execute(sql_insert2,data) 13 data = [("xiao",20), 14 ("xian",25), 15 ("rourou",27), 16 ("juju",28)] 17 cursor.executemany(sql_insert2,data) 18 19 #如果表引擎为Innodb,执行完成后需执行commit进行事务提交 20 db.commit() 21 #cursor.execute('commit') 22 except mysql.connector.Error as e: 23 print('插入失败!', str(e)) 24 finally: 25 cursor.close() 26 db.close()
删除数据:
1 cursor = db.cursor(buffered=True) 2 try: 3 sql_del = "delete from student where name=%s and age=%s" 4 data_del = [ 5 ("cui",28), 6 ("hao",27)] 7 cursor.executemany(sql_del,data_del) 8 db.commit() 9 except mysql.connector.Error as e: 10 print("删除数据失败!",str(e)) 11 finally: 12 cursor.close() 13 db.close()
修改数据:
1 cursor = db.cursor(buffered=True) 2 try: 3 sql_update = "update student set age = 28 where name='kai'" 4 cursor.execute(sql_update) 5 db.commit() 6 except mysql.connector.Error as e: 7 print('修改数据失败',str(e)) 8 finally: 9 cursor.close() 10 db.close()
查询数据:
1 cursor = db.cursor(buffered=True) 2 try: 3 # sql_select1 = "select * from student where age>%s" 4 # cursor.execute(sql_select1,(1,)) 5 6 sql_select2 = "select * from student where age>%s" 7 cursor.execute(sql_select2,(26,)) 8 datas1 = cursor.fetchall() #如果在后加上[1]代表是取第一条数据 9 10 cursor.execute(sql_select2,(20,)) 11 datas2 = cursor.fetchone()[1] #如果在后加上[1]代表是取第一个字段 12 datas3 = cursor.fetchmany(5) 13 14 print(datas1) 15 print(datas2) 16 print(datas3) 17 except mysql.connector.Error as e: 18 print("查询数据失败!",str(e)) 19 finally: 20 cursor.close() 21 db.close()
三、python第三方模块操作Excel
注意高能:openpyxl只能操作xlsx文件而不能操作xls文件!所以在创建的时候一定要新建.xlsx格式的Excel!!!
1 import openpyxl 2 #打开文件 3 path_file = "D:/PycharmProjects/open.xlsx" 4 wp = openpyxl.load_workbook(path_file) 5 6 print("获取所有工作表名:",wp.get_sheet_names()) 7 # sheet = wp.get_sheet_by_name("Sheet1") #获取指定的工作表sheet 8 # print("获取指定的工作表名:",sheet.title) 9 sheet2 = wp.get_active_sheet() #获取活动的工作表sheet,一般是当前打开的sheet页 10 print("获取活动的工作表名:",sheet2.title) 11 12 13 # 操作单元格:数据的读取与写入 14 # 获取单元格数据 15 print(sheet2['A1'].value) 16 print(sheet2.cell(row=2,column=1).value) #获取第二行第一列的值 17 18 # 数据的写入 19 sheet2.cell(row=4,column=1).value = "工作总结" 20 sheet2['C3']='cs' 21 22 print("最大列数",sheet2.max_column) 23 print("最大行数",sheet2.max_row) 24 25 # wp.save('D:/PycharmProjects/open1.xlsx') #另存为 26 wp.save("open.xlsx") #保存,默认保存在当前目录下 27 wp.save(path_file) #覆盖保存
小练习:
1、Python里面match()和search()的区别?
python正则中的match是在字符串的起始位置进行匹配,如果起始位置匹配不成功就会报错。如下例子: import re print(re.match("www","wwccc").group()) python正则中的search是在字符串中匹配第一次匹配成功的字符串并返回。如下例子: import re print(re.search("ws","wwcccwss").group())
2、以<div><span>test</span></div>进行匹配
<.*>意思为:匹配以<为开始的所有的字符,遇到回车换行中断匹配 <.*>结果为:<div><span>test</span></div> <.*?>意思为:匹配以<为开始的字符串,出现一次就返回 <.*?>结果为: <div> <span> <span> <div>
3、获取URL的后缀名
abc = ("http://www.baidu.cn/archive/6688431.html") print(abc.split('.')[-1])
相关文章推荐
- Python自动化运维笔记(七):XlsxWriter模块实现Excel操作(上)
- 用Python 模块xlrd 操作excel,并将数据导入MySQL
- Python 使用xlwt模块操作Excel写
- Python之re模块 —— 正则表达式操作
- Python的SQLalchemy模块连接与操作MySQL的基础示例
- Python之re模块 —— 正则表达式操作
- python数据库操作之pymysql模块和sqlalchemy模块(项目必备)
- Python的re模块(正则表达式操作)
- Python的re模块正则表达式操作
- Python的SQLalchemy模块连接与操作MySQL的基础示例
- Python使用xlrd模块操作Excel数据导入的方法
- Python3.x 操作Excel(写)——XlsxWriter 模块
- Python操作Excel——win32com模块和xlrd+xlwt+xlutils组合
- Python使用xlrd模块操作Excel数据导入的方法
- Python之re模块 —— 正则表达式操作
- Python使用xlrd模块操作Excel数据导入的方法
- Python之re模块 —— 正则表达式操作[转]
- Python使用xlrd模块操作Excel数据导入的方法
- Python(SQLAlchemy-ORM)模块之mysql操作