PHP学习笔记【二】之《数据库抽象层PDO---PDO连接数据库》
2017-02-24 18:51
736 查看
一、PDO连接数据库
1、通过参数形式连接数据库
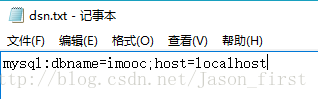
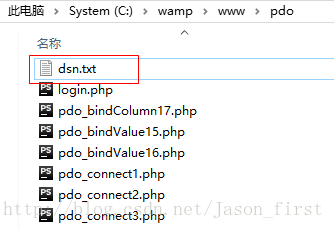
2、通过URI形式连接数据库
二、PDO和PDOStatement对象的方法
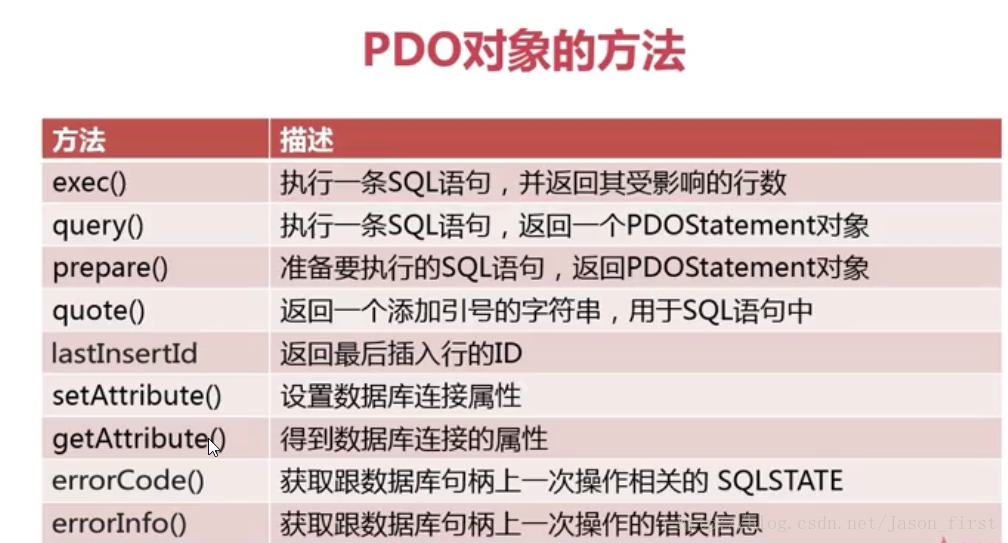

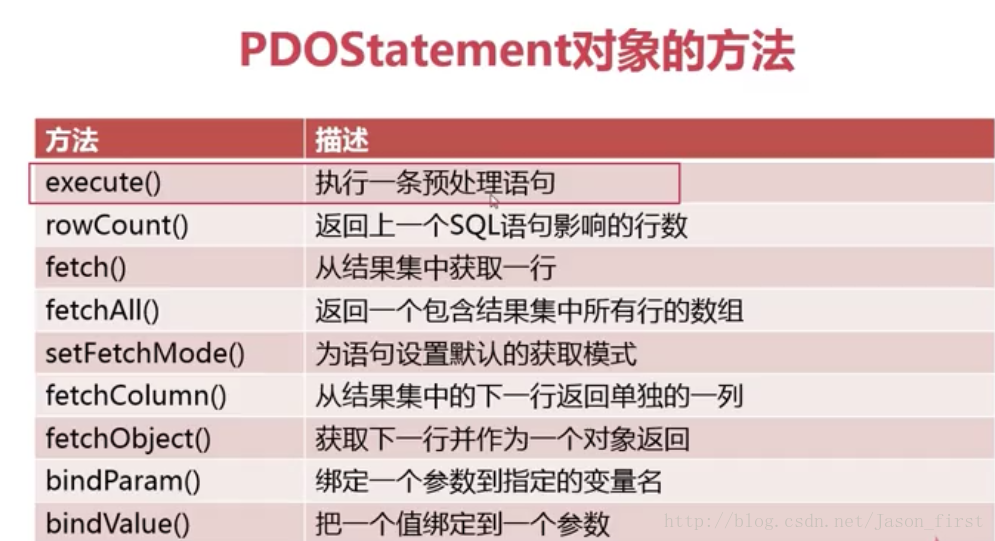
1、exec()方法执行插入记录操作
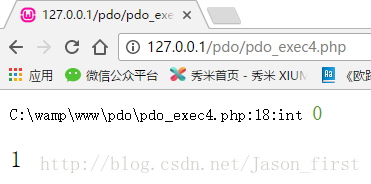
exec()显示其受SQL语句影响的记录的条数。
2、errorCode()和errorInfo()方法查看错误信息
errorCode()返回SQLSTATE的值
errorInfo():返回的错误信息的数组,数组中包含3个单元
//0=>SQLSTATE,1=>CODE,2=>INFO
3、query()方法执行查询语句
query(),执行SQL语句,返回PDOStatement对象
4、prepare()和execute()方法执行查询语句
1、通过参数形式连接数据库
<?php //通过参数形式连接数据库 try{ $dsn='mysql:host=localhost;dbname=my_db'; $username='root'; $password=''; $pdo=new PDO($dsn, $username, $password); var_dump($pdo); }catch(PDOException $e){ echo $e->getMessage(); }
2、通过URI形式连接数据库
<?php //通过uri的形式连接数据库 try{ $dsn='uri:file://C:\wamp\www\pdo\dsn.txt'; $username='root'; $password=''; $pdo=new PDO($dsn,$username,$password); var_dump($pdo); }catch(PDOException $e){ echo $e->getMessage(); }
二、PDO和PDOStatement对象的方法
1、exec()方法执行插入记录操作
<?php try{ $pdo=new PDO('mysql:host=localhost;dbname=my_db','root',''); //exec():执行一条sql语句并返回其受影响的记录的条数,如果没有受影响的记录,他返回0 //exec对于select没有作用 $sql=<<<EOF CREATE TABLE IF NOT EXISTS user( id INT UNSIGNED AUTO_INCREMENT KEY, username VARCHAR(20) NOT NULL UNIQUE, password CHAR(32) NOT NULL, email VARCHAR(30) NOT NULL ); EOF; //<<<EOF及EOF是php的定界符,在<<<EOF 和 EOF;之间的文本, 可以不用转义, //比如单引号和双引号,一般用于输出长的html文本或者文本赋值 //这样写sql语句,可以不用对字符型字段两边的单引号进行转义 $res=$pdo->exec($sql); var_dump($res); $sql='INSERT user(username,password,email) VALUES("king","'.md5('king').'","123456789@qq.com")'; //echo $sql; $res=$pdo->exec($sql); echo $res; }catch(PDOException $e){ echo $e->getMessage(); }
exec()显示其受SQL语句影响的记录的条数。
2、errorCode()和errorInfo()方法查看错误信息
<?php header('content-type:text/html;charset=utf-8'); try{ $pdo=new PDO('mysql:host=localhost;dbname=my_db','root',''); $sql='delete from user12 where id=1'; $res=$pdo->exec($sql); //echo $res.'条记录被影响'; //var_dump($res); if($res===false){ //$pdo->errorCode():SQLSTATE的值 echo $pdo->errorCode(); echo '<hr/>'; //$pdo->errorInfo():返回的错误信息的数组,数组中包含3个单元 //0=>SQLSTATE,1=>CODE,2=>INFO $errInfo=$pdo->errorInfo(); print_r($errInfo); } // echo '<hr/>'; // echo $pdo->lastInsertId(); }catch(PDOException $e){ echo $e->getMessage(); }
errorCode()返回SQLSTATE的值
errorInfo():返回的错误信息的数组,数组中包含3个单元
//0=>SQLSTATE,1=>CODE,2=>INFO
3、query()方法执行查询语句
<?php header('content-type:text/html;charset=utf-8'); try{ $pdo=new PDO('mysql:host=localhost;dbname=my_db','root',''); //$sql='select * from user where id=2'; $sql='select id,username,email from user'; //$pdo->query($sql),执行SQL语句,返回PDOStatement对象 $stmt=$pdo->query($sql); var_dump($stmt); echo '<hr/>'; foreach($stmt as $row){ //print_r($row); echo '编号:'.$row['id'],'<br/>'; echo '用户名:'.$row['password'],'<br/>'; echo '邮箱:'.$row['email'],'<br/>'; echo '<hr/>'; } }catch(PDOException $e){ echo $e->getMessage(); }
<?php header('content-type:text/html;charset=utf-8'); try{ $pdo=new PDO('mysql:host=localhost;dbname=my_db','root',''); $sql='INSERT user(username,password,email) VALUES("king11","'.md5('king11').'","123456789@qq.com")'; $stmt=$pdo->query($sql); var_dump($stmt); }catch(PDOException $e){ echo $e->getMessage(); }
query(),执行SQL语句,返回PDOStatement对象
4、prepare()和execute()方法执行查询语句
<?php header('content-type:text/html;charset=utf-8'); try{ $pdo=new PDO('mysql:host=localhost;dbname=my_db','root',''); $sql='select * from user where username="king11"'; //prepare($sql):准备SQL语句 $stmt=$pdo->prepare($sql); //execute():执行预处理语句 $res=$stmt->execute(); //var_dump($res); //fetch():得到结果集中的一条记录 $row=$stmt->fetch(); print_r($row); //var_dump($stmt); }catch(PDOException $e){ echo $e->getMessage(); }
相关文章推荐
- php学习笔记之PDO连接数据库及一些操作
- PHP学习笔记(15)PDO数据库操作+AJAX无刷新技术删除用户
- PHP学习笔记:PDO连接MySQL
- PHP学习笔记:用mysqli连接数据库
- PHP学习笔记【三】之《数据库抽象层PDO---PDOStatement对象的使用》
- PHP 开发 APP 接口 学习笔记与总结 - APP 接口实例 [1] 单例模式连接数据库
- PHP学习笔记【一】之《数据库抽象层PDO---PDO简介、配置与启用》
- PHP学习笔记(二):数据库连接
- PHP学习 PDO连接数据库和设备常量的操作
- PHP学习笔记【四】之《数据库抽象层PDO---PDO事务处理》
- [php学习笔记]PDO类操作数据库
- php学习笔记(一)——数据库连接及数据获取和添加
- php学习笔记(2)- 数据库操作
- PHP学习笔记之三:数据库基本操作
- php学习笔记(二)之保存至数据库
- php连接mssql数据库 初学php笔记
- PHP PDO基础认知和学习笔记
- php 连接mssql数据库 初学php笔记
- PHP PDO 学习笔记