jquery实现瀑布流效果 jquery下拉加载新数据
2016-12-12 00:00
656 查看
瀑布流效果在很多网站还是有的,这种错落有致的排布看着还是很不错的呢。今天我就来记录一下关于用jquery实现瀑布流效果的代码;
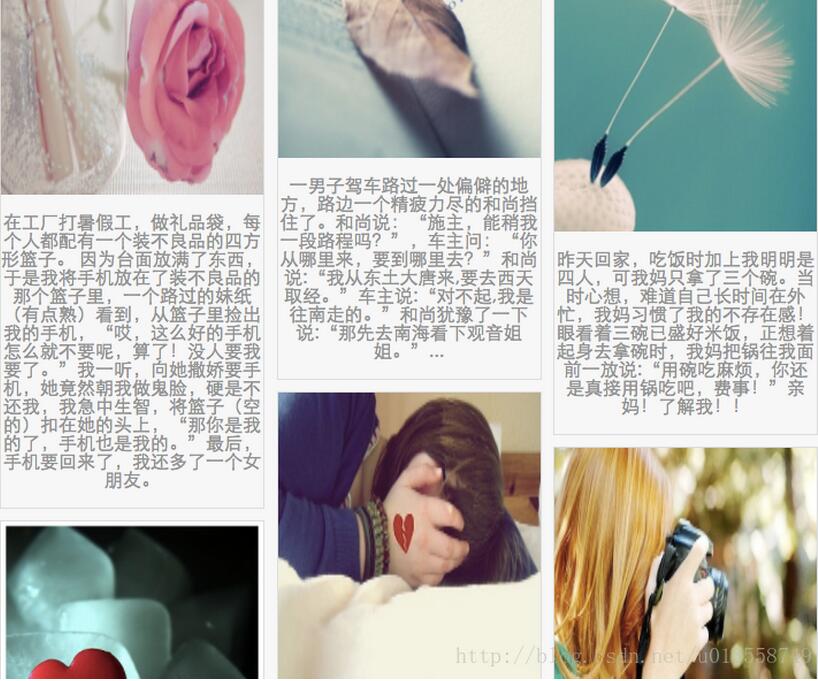
一、页面基本排版
1. items盒子主要用来存放我们需要摆放的数据item;
2. tips是页面加载数据的时候用来提示用户的文本;
二、css样式(控制提示语句摆放的位置,还有数据展示的样式)
三、模版的引用(由于本例子中数据的展示样式都一致,在这里我引用模版artTemplate)关
1. 记得要先引入这个模版的js包;
2. 定义模版的模块要有一个id,还有设置type;
四、js控制瀑布流的效果
1. 这里,我请求了两个php分别返回了两个模拟数据;
五、最后在这里奉上的是自定义模拟数据,以及简单编写的php返回数据(别忘了,用此种方式获取数据的话,需要开启本地服务器哦~);
如下为返回数据的基本模式,如果想要定义多条数据,只要多复制几条对象就可;
如下为php代码:
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持脚本之家。
jQuery 瀑布流 浮动布局(一)(延迟AJAX加载图片)
jQuery瀑布流插件Wookmark使用实例
jQuery Masonry瀑布流插件使用详解
jQuery 瀑布流 绝对定位布局(二)(延迟AJAX加载图片)
jQuery实现下拉加载功能实例代码
jQuery模拟原生态App上拉刷新下拉加载更多页面及原理
jQuery+AJAX实现无刷新下拉加载更多
jQuery实现瀑布流布局
jQuery.lazyload+masonry改良图片瀑布流代码
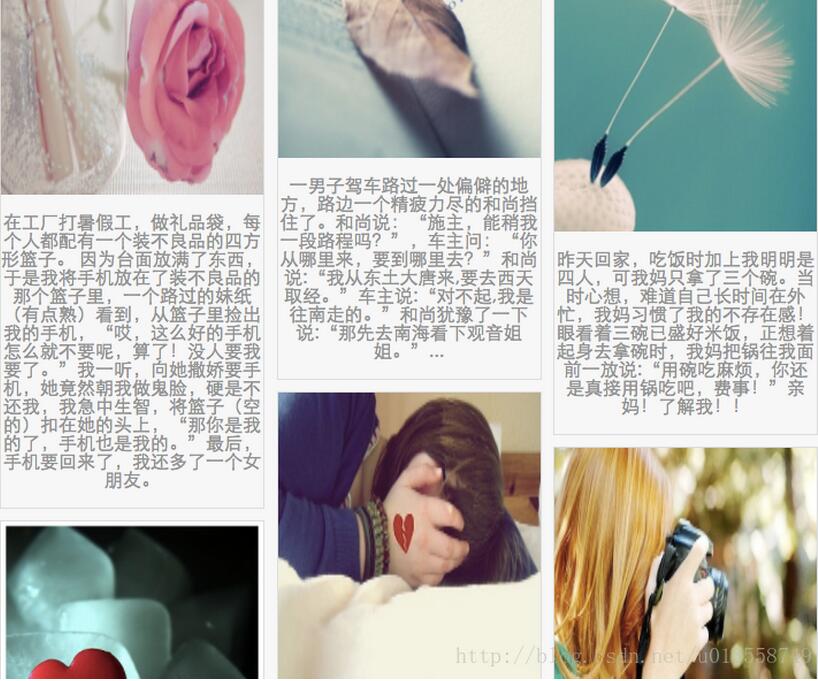
一、页面基本排版
1. items盒子主要用来存放我们需要摆放的数据item;
2. tips是页面加载数据的时候用来提示用户的文本;
<div class="wrapper"> <div class="items"> <div class="item"></div> </div> <p class="tips loading">正在加载...</p> </div>
二、css样式(控制提示语句摆放的位置,还有数据展示的样式)
body { text-align: center; margin: 0; padding: 0; background-color: #F7F7F7; font-family: '微软雅黑'; } .wrapper { padding: 50px; } img { display: block; width: 100%; height: 300px; } .items { position: relative; padding-bottom: 10px; } .item { width: 228px; position: absolute; border: 1px solid #ddd; } .tips { width: 280px; height: 40px; margin: 30px auto 0; text-align: center; line-height: 40px; background-color: #CCC; border-radius: 6px; font-size: 16px; cursor: pointer; position: fixed; bottom: 0px; left: 50%; margin-left: -140px; opacity: 0; color: #666; } .tips.loading { /*background-color: transparent;*/ background-color: #dadada; }
三、模版的引用(由于本例子中数据的展示样式都一致,在这里我引用模版artTemplate)关
1. 记得要先引入这个模版的js包;
2. 定义模版的模块要有一个id,还有设置type;
<script src="../js/template_native.js"></script> <script type="text/html" id="template"> <% for(var i = 0; i < items.length; i++){ %> <div class='item'> <img src="<%=items[i].path%>" alt=""> <p> <%=items[i].text%> </p> </div> <% } %> </script>
四、js控制瀑布流的效果
1. 这里,我请求了两个php分别返回了两个模拟数据;
$(function() { //页面一加载就出现的图片,对应实际百度图片中点击搜索图片 $.ajax({ url: "./reset.php", dataType: "json", success: function(data) { var obj = { items: data }; var result = template("template", obj); $(".items").html(result); waterfall(); } }); }); // 编写瀑布流js function waterfall() { //计算出显示盒子宽度 var totalWidth = $(".items").width(); //计算出单张图片宽度(每张图片宽度是一致的) var eachWidth = $(".items .item").width(); //计算出一行能排布几张图片 var columNum = Math.floor(totalWidth / eachWidth); //将剩余的空间设置成外边距 var margin = (totalWidth - eachWidth * columNum) / (columNum + 1); //定义一个数组用来填充高度值 var heightArr = []; for (var i = 0; i < columNum; i++) { heightArr[i] = 0; } //摆放位置 摆放在最小高度处 var elementItems = $(".items .item"); elementItems.each(function(idx, ele) { //取得一行中高度最小值及其索引 //定义初始的最小值及其索引值 var minIndex = 0; var minValue = heightArr[minIndex]; for (var i = 0; i < heightArr.length; i++) { if (heightArr[i] < minValue) { minIndex = i; minValue = heightArr[i]; } } $(ele).css({ //注意点:这儿乘上的是最小值所在的索引idx left: margin + (margin + eachWidth) * minIndex, top: minValue }); //重新计算高度,更新高度数组 var oldHeight = heightArr[minIndex]; oldHeight += $(ele).height() + margin; heightArr[minIndex] = oldHeight; }); return heightArr; } $(window).on("scroll", function() { if (toBottom()) { $(".tips").css("opacity", 1); $.ajax({ url: "./index.php", dataType: "json", success: function(data) { var dataItem = { items: data }; var res = template("template", dataItem); $(".items").append(res); waterfall(); $(".tips").css("opacity", 0); } }); } }); //判断是否已经到底部了 function toBottom() { var scrollTop = $(window).scrollTop(); var clientHeight = $(window).height(); var offsetTop = $(".items .item:last-child").offset().top; console.log(scrollTop + "..." + clientHeight + "..." + offsetTop); if (scrollTop + clientHeight > offsetTop) { return true; } else { return false; } }
五、最后在这里奉上的是自定义模拟数据,以及简单编写的php返回数据(别忘了,用此种方式获取数据的话,需要开启本地服务器哦~);
如下为返回数据的基本模式,如果想要定义多条数据,只要多复制几条对象就可;
[ { "path": "./images/1.jpg", "text": "中学时候我们班两个同学打赌,内容是在 厕所吃方便面,谁先吃完谁赢,输了的请 赢了的吃一个月的饭,于是厕所里惊现两 个货蹲坑上吃泡面,这俩货还没有决出胜 负,旁边拉屎的哥们都吐了三回了!!!" }, { "path": "./images/2.jpg", "text": "亲戚有许多好兄弟,平时戏称为马哥,牛哥,等等动物名。一次,有人敲门,那时他儿子尚小,一开门,对着他爸妈就说:爸爸,妈妈,驴来了!" } ... ]
如下为php代码:
//reset.php <?php $jsonString = file_get_contents('info/reset.json'); $totalArr = json_decode($jsonString); echo json_encode($totalArr); ?>
//index.php 这里规定一次返回三条数据 <?php $jsonString = file_get_contents('info/data.json'); $totalArr = json_decode($jsonString); $randomKeyArr = array_rand($totalArr,3); $templateArr = array(); for ($i=0; $i <count($randomKeyArr) ; $i++) { $currentKey = $randomKeyArr[$i]; $currentObj = $totalArr[$currentKey]; $templateArr[$i] = $currentObj; } echo json_encode($templateArr); ?>
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持脚本之家。
您可能感兴趣的文章:
Jquery瀑布流插件使用介绍jQuery 瀑布流 浮动布局(一)(延迟AJAX加载图片)
jQuery瀑布流插件Wookmark使用实例
jQuery Masonry瀑布流插件使用详解
jQuery 瀑布流 绝对定位布局(二)(延迟AJAX加载图片)
jQuery实现下拉加载功能实例代码
jQuery模拟原生态App上拉刷新下拉加载更多页面及原理
jQuery+AJAX实现无刷新下拉加载更多
jQuery实现瀑布流布局
jQuery.lazyload+masonry改良图片瀑布流代码
相关文章推荐
- jquery实现瀑布流效果 jquery下拉加载新数据
- jquery实现瀑布流效果+下拉加载新数据
- jQuery实现模仿微博下拉滚动条加载数据效果
- jQuery实现模仿微博下拉滚动条加载数据效果
- jQUery 中masonry与infinitescroll结合 实现瀑布流,下拉加载
- ASP.NET仿新浪微博下拉加载更多数据瀑布流效果
- [给力原创]使用jQuery-ajax仿新浪微博通知折叠/显示效果,实现数据加载(二)
- 点击侧滑任何一个 菜单项,请求网络数据展示在主界面的xlistview中且实现下拉刷新效果和上拉加载的分页加载数据效果
- [给力原创]使用jQuery-ajax仿新浪微博通知折叠/显示效果,实现数据加载
- jQuery实现的瀑布流效果, 向下滚动即时加载内容
- jQuery实现的瀑布流效果, 向下滚动即时加载内容
- PHP+Jquery与ajax相结合实现下拉淡出瀑布流效果【无需插件】
- jQuery向下滚动即时加载内容实现的瀑布流效果
- jQuery实现的瀑布流效果, 向下滚动即时加载内容
- ASP.NET仿新浪微博下拉加载更多数据瀑布流效果
- jquery 实现滚动条至页面底端自动加载数据效果
- ASP.NET仿新浪微博下拉加载更多数据瀑布流效果
- jQuery类似瀑布流效果边下拉页面边加载内容
- jQuery实现的瀑布流加载效果示例
- PHP+Jquery与ajax相结合实现下拉淡出瀑布流效果【无需插件】