第三周 四则运算
2016-09-28 22:44
190 查看
结对编程:李峤 杜月
HTTP:https://git.coding.net/liqiao085/sizeyunsuan.git
ssh://git@git.coding.net:liqiao085/sizeyunsuan.git
功能2 :
void gao(char str[])
{
int cnt = 0;
stack<int> st;//符号栈
int len = strlen(str);
for(int i = 0;i<len;i++)
{
if(str[i]=='(') st.push(str[i]);//对左括号的处理
else if(str[i]==')')//对右括号的处理
{
while(!st.empty())
{
int u = st.top();
st.pop();
if(u=='(') break;
s[cnt++] = u;
}
}
else if(str[i]=='+'||str[i]=='-'||str[i]=='*'||str[i]=='/')//对运算符的处理
{
while(!st.empty())
{
int u = st.top();
if(u=='('||val[str[i]]>val[u]) break;
st.pop();
s[cnt++] = u;
}
st.push(str[i]);
}
else//数字直接加入字符串
{
s[cnt++] = str[i];
}
}
//把还在栈中的符号出栈
while(!st.empty())
{
int u = st.top();
st.pop();
s[cnt++] = u;
}
s[cnt] = 0;
}
int check(char str[])//判断str字符串是否合法
{
int a = 0;
int len = strlen(str);
for(int i = 0;i<len;i++)
{
if(str[i]=='(') a++;
else if(str[i]==')')a--;
if(a<0) return 0;
if(str[i]==')'&&i>=2&&str[i-2]=='(') return 0;
}
if(str[0]=='('&&str[strlen(str)-1]==')') return 0;
return 1;
}
void pro(char str[])//随机产生一个四则运算
{
int sum = rand()%3; //随机括号的个数
for(int i = 0;i<=6;i++)
{
if(i%2==0)
{
str[i] = rand()%9+1+'0';
}
else
{
int temp = rand()%4;
if(temp==0) str[i] = '+';
if(temp==1) str[i] = '-';
if(temp==2) str[i] = '*';
if(temp==3) str[i] = '/';
}
}
str[7] = 0;
for(int i= 1;i<=sum;i++)//循环增加括号
{
int a = rand()%(7+2*i-2);
int b = rand()%(7+2*i-2);
while(!ok(a,b,str))
{
a = rand()%(7+2*i-2);
b = rand()%(7+2*i-2);
}
char ss[100];
int cnt = 0;
for(int j = 0;j<7+2*i-2;j++)
{
if(j==a)
{
ss[cnt++] = '(';
ss[cnt++] = str[j];
}
else if(j==b)
{
ss[cnt++] = str[j];
ss[cnt++] = ')';
}
else ss[cnt++] = str[j];
}
ss[cnt] = 0;
int flag = check(ss);
if(!flag) i--;
else
{
strcpy(str,ss);
}
}
// printf("%s\n",str);
// gao(str);
// pair<int,int> pa = cal(s);
// printf("%d %d\n",pa.first,pa.second);
}
int is_ok(int a, int b)//判断a/b是否是无限循环小数
{
if(b==0) return 0;
if(a==0) return 1;
map<int,int> ma;
while(1)
{
while(a<b) a*=10;
if(a%b==0) return 1;
if(ma[a%b]) return 0;
ma[a%b] = 1;
a = a%b;
}
}
void pri(int a,int b)//打印a/b的值
{
if(a==0)//如果为0直接输出防止后面运算出bug
{
printf("0");
return;
}
printf("%d.",a/b);
a = a%b;
//a*=10;
while(1)
{
int flag = 0;
while(a<b)
{
a*=10;
if(flag)
printf("0");
flag++;
}
printf("%d",a/b);
a = a%b;
if(a==0) break;
}
}
功能4:
int is_num(char str[])//判断str是否为正整数
{
int sum = 0;
int len = strlen(str);
for(int i = 0;i<len;i++)
{
if(str[i]>='0'&&str[i]<='9') sum = sum*10+str[i]-'0';
else return -1;
}
if(sum==0) return -1;
else return sum;
}
pair<int,int> cal(char str[])//计算值,已分数表示
{
int a,b;
stack<pair<int,int> > st;//存放值的栈,用分数表示
int len = strlen(str);
for(int i = 0;i<len;i++)
{
if(str[i]>='0'&&str[i]<='9')
{
st.push(make_pair(str[i]-'0',1));//现转化为分数
}
else
{
pair<int,int> pa1 = st.top();
st.pop();
pair<int,int> pa2 = st.top();
st.pop();
int LCM = lcm(pa1.second,pa2.second);
if(str[i]=='+')//对加法的处理
{
//if(pa1.second==0||pa2.second==0) return make_pair(0,0);
int a = pa1.first*(LCM/pa1.second);
int b = pa2.first*(LCM/pa2.second);
pa1.first = a+b;
pa1.second = LCM;
st.push(pa1);//入栈
}
else if(str[i]=='-')//对减法的处理
{
int a = pa1.first*(LCM/pa1.second);
int b = pa2.first*(LCM/pa2.second);
pa1.first = b-a;
pa1.second = LCM;
st.push(pa1);
}
else if(str[i]=='*')//对乘法的处理
{
pa1.first = pa1.first*pa2.first;
pa1.second = pa1.second*pa2.second;
st.push(pa1);
}
else//对除法的处理
{
if(pa1.first==0) return make_pair(0,0);//改进了对除0的处理
int a = pa2.first*pa1.second;
int b = pa2.second*pa1.first;
pa1.first = a,pa1.second = b;
st.push(pa1);
}
}
}
pair<int,int> pa = st.top();
st.pop();
int GCD = gcd(pa.first,pa.second);
pa.first/=GCD,pa.second/=GCD;//化成最简形式
return pa;
}
功能3,4 :
if(argc==3)
{
int flag = is_num(argv[2]);
if(flag==-1) {printf("题目数量必须是 正整数。\n");return 0;}
for(int i = 1;i<=flag;i++)
{
char str[100];
pro(str);
gao(str);
printf("%-50s%",str);
pair<int,int> pa = cal(s);
if(pa.second==0) {
i--;continue;
}
if(pa.first%pa.second==0) printf("%d\n",pa.first/pa.second);
else
{
int flag = 0;
if(pa.first<0) flag++;
if(pa.second<0) flag++;
if(flag%2) printf("-");
pa.first = abs(pa.first);//正负提取,取绝对值
pa.second = abs(pa.second);
if(pa.first/pa.second) printf("%d ",pa.first/pa.second);
pa.first-=pa.first/pa.second*pa.second;
printf("%d/%d\n",pa.first,pa.second);//输出答案
}
}
HTTP:https://git.coding.net/liqiao085/sizeyunsuan.git
ssh://git@git.coding.net:liqiao085/sizeyunsuan.git
功能2 :
void gao(char str[])
{
int cnt = 0;
stack<int> st;//符号栈
int len = strlen(str);
for(int i = 0;i<len;i++)
{
if(str[i]=='(') st.push(str[i]);//对左括号的处理
else if(str[i]==')')//对右括号的处理
{
while(!st.empty())
{
int u = st.top();
st.pop();
if(u=='(') break;
s[cnt++] = u;
}
}
else if(str[i]=='+'||str[i]=='-'||str[i]=='*'||str[i]=='/')//对运算符的处理
{
while(!st.empty())
{
int u = st.top();
if(u=='('||val[str[i]]>val[u]) break;
st.pop();
s[cnt++] = u;
}
st.push(str[i]);
}
else//数字直接加入字符串
{
s[cnt++] = str[i];
}
}
//把还在栈中的符号出栈
while(!st.empty())
{
int u = st.top();
st.pop();
s[cnt++] = u;
}
s[cnt] = 0;
}
int check(char str[])//判断str字符串是否合法
{
int a = 0;
int len = strlen(str);
for(int i = 0;i<len;i++)
{
if(str[i]=='(') a++;
else if(str[i]==')')a--;
if(a<0) return 0;
if(str[i]==')'&&i>=2&&str[i-2]=='(') return 0;
}
if(str[0]=='('&&str[strlen(str)-1]==')') return 0;
return 1;
}
void pro(char str[])//随机产生一个四则运算
{
int sum = rand()%3; //随机括号的个数
for(int i = 0;i<=6;i++)
{
if(i%2==0)
{
str[i] = rand()%9+1+'0';
}
else
{
int temp = rand()%4;
if(temp==0) str[i] = '+';
if(temp==1) str[i] = '-';
if(temp==2) str[i] = '*';
if(temp==3) str[i] = '/';
}
}
str[7] = 0;
for(int i= 1;i<=sum;i++)//循环增加括号
{
int a = rand()%(7+2*i-2);
int b = rand()%(7+2*i-2);
while(!ok(a,b,str))
{
a = rand()%(7+2*i-2);
b = rand()%(7+2*i-2);
}
char ss[100];
int cnt = 0;
for(int j = 0;j<7+2*i-2;j++)
{
if(j==a)
{
ss[cnt++] = '(';
ss[cnt++] = str[j];
}
else if(j==b)
{
ss[cnt++] = str[j];
ss[cnt++] = ')';
}
else ss[cnt++] = str[j];
}
ss[cnt] = 0;
int flag = check(ss);
if(!flag) i--;
else
{
strcpy(str,ss);
}
}
// printf("%s\n",str);
// gao(str);
// pair<int,int> pa = cal(s);
// printf("%d %d\n",pa.first,pa.second);
}
int is_ok(int a, int b)//判断a/b是否是无限循环小数
{
if(b==0) return 0;
if(a==0) return 1;
map<int,int> ma;
while(1)
{
while(a<b) a*=10;
if(a%b==0) return 1;
if(ma[a%b]) return 0;
ma[a%b] = 1;
a = a%b;
}
}
void pri(int a,int b)//打印a/b的值
{
if(a==0)//如果为0直接输出防止后面运算出bug
{
printf("0");
return;
}
printf("%d.",a/b);
a = a%b;
//a*=10;
while(1)
{
int flag = 0;
while(a<b)
{
a*=10;
if(flag)
printf("0");
flag++;
}
printf("%d",a/b);
a = a%b;
if(a==0) break;
}
}
功能4:
int is_num(char str[])//判断str是否为正整数
{
int sum = 0;
int len = strlen(str);
for(int i = 0;i<len;i++)
{
if(str[i]>='0'&&str[i]<='9') sum = sum*10+str[i]-'0';
else return -1;
}
if(sum==0) return -1;
else return sum;
}
pair<int,int> cal(char str[])//计算值,已分数表示
{
int a,b;
stack<pair<int,int> > st;//存放值的栈,用分数表示
int len = strlen(str);
for(int i = 0;i<len;i++)
{
if(str[i]>='0'&&str[i]<='9')
{
st.push(make_pair(str[i]-'0',1));//现转化为分数
}
else
{
pair<int,int> pa1 = st.top();
st.pop();
pair<int,int> pa2 = st.top();
st.pop();
int LCM = lcm(pa1.second,pa2.second);
if(str[i]=='+')//对加法的处理
{
//if(pa1.second==0||pa2.second==0) return make_pair(0,0);
int a = pa1.first*(LCM/pa1.second);
int b = pa2.first*(LCM/pa2.second);
pa1.first = a+b;
pa1.second = LCM;
st.push(pa1);//入栈
}
else if(str[i]=='-')//对减法的处理
{
int a = pa1.first*(LCM/pa1.second);
int b = pa2.first*(LCM/pa2.second);
pa1.first = b-a;
pa1.second = LCM;
st.push(pa1);
}
else if(str[i]=='*')//对乘法的处理
{
pa1.first = pa1.first*pa2.first;
pa1.second = pa1.second*pa2.second;
st.push(pa1);
}
else//对除法的处理
{
if(pa1.first==0) return make_pair(0,0);//改进了对除0的处理
int a = pa2.first*pa1.second;
int b = pa2.second*pa1.first;
pa1.first = a,pa1.second = b;
st.push(pa1);
}
}
}
pair<int,int> pa = st.top();
st.pop();
int GCD = gcd(pa.first,pa.second);
pa.first/=GCD,pa.second/=GCD;//化成最简形式
return pa;
}
功能3,4 :
if(argc==3)
{
int flag = is_num(argv[2]);
if(flag==-1) {printf("题目数量必须是 正整数。\n");return 0;}
for(int i = 1;i<=flag;i++)
{
char str[100];
pro(str);
gao(str);
printf("%-50s%",str);
pair<int,int> pa = cal(s);
if(pa.second==0) {
i--;continue;
}
if(pa.first%pa.second==0) printf("%d\n",pa.first/pa.second);
else
{
int flag = 0;
if(pa.first<0) flag++;
if(pa.second<0) flag++;
if(flag%2) printf("-");
pa.first = abs(pa.first);//正负提取,取绝对值
pa.second = abs(pa.second);
if(pa.first/pa.second) printf("%d ",pa.first/pa.second);
pa.first-=pa.first/pa.second*pa.second;
printf("%d/%d\n",pa.first,pa.second);//输出答案
}
}
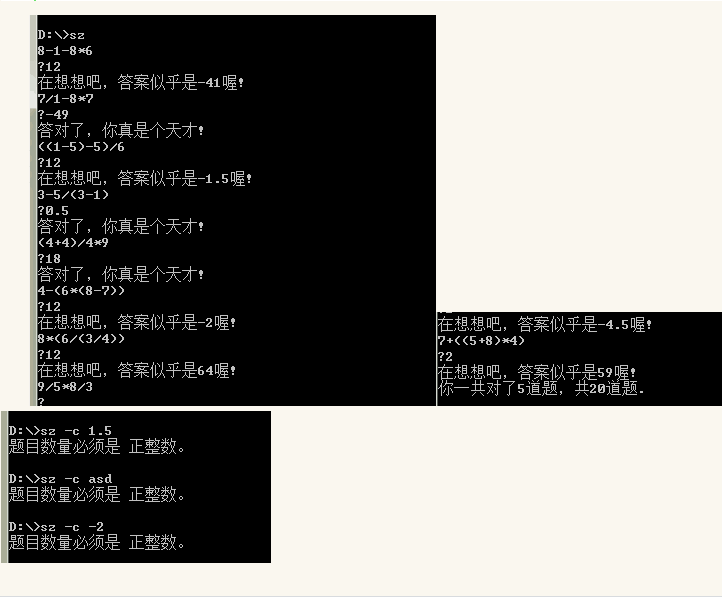
相关文章推荐
- 第三周项目四:考了语文数学的学生(多文件形式)
- 初级阶段的 四则运算
- 第三周项目五 数组做数据成员
- 第三周项目四
- 第三周 项目五-数组做数据成员(四)文件操作-指针
- 软件工程——四则运算Ⅲ
- 第三周项目3 多文件组织
- 第三周项目1:三角形类1
- 第三周上机实践项目1-三角形类
- 第三周上机项目1三角形类
- 第三周项目:三角形类1
- 第三周【项目1-三角形类1】
- 第三周项目1-三角形类1
- 第三周(三角形拓展)
- 第三周项目三程序的多文件组织
- 第三周项目四 成绩单并多文件组织
- 四则运算
- 第三周 项目一(3):三角形类的构造函数(有默认参数的构造函数)
- 第三周上机实践项目5-工资类(3、4从文件中读入数据并把结果保存在另一个文件中)
- 简单的四则运算