【Android笔记】WebView的使用
2016-09-28 00:00
246 查看
摘要: WebView的基本使用
1)加载本地文件:
2)加载web资源
是页面获得焦点
优先使用缓存:
不使用缓存:
看一个实例:
1.新建一个项目,在activity_main.xml中添加WebView
2.在MainActivity.java中添加代码:
运行效果:
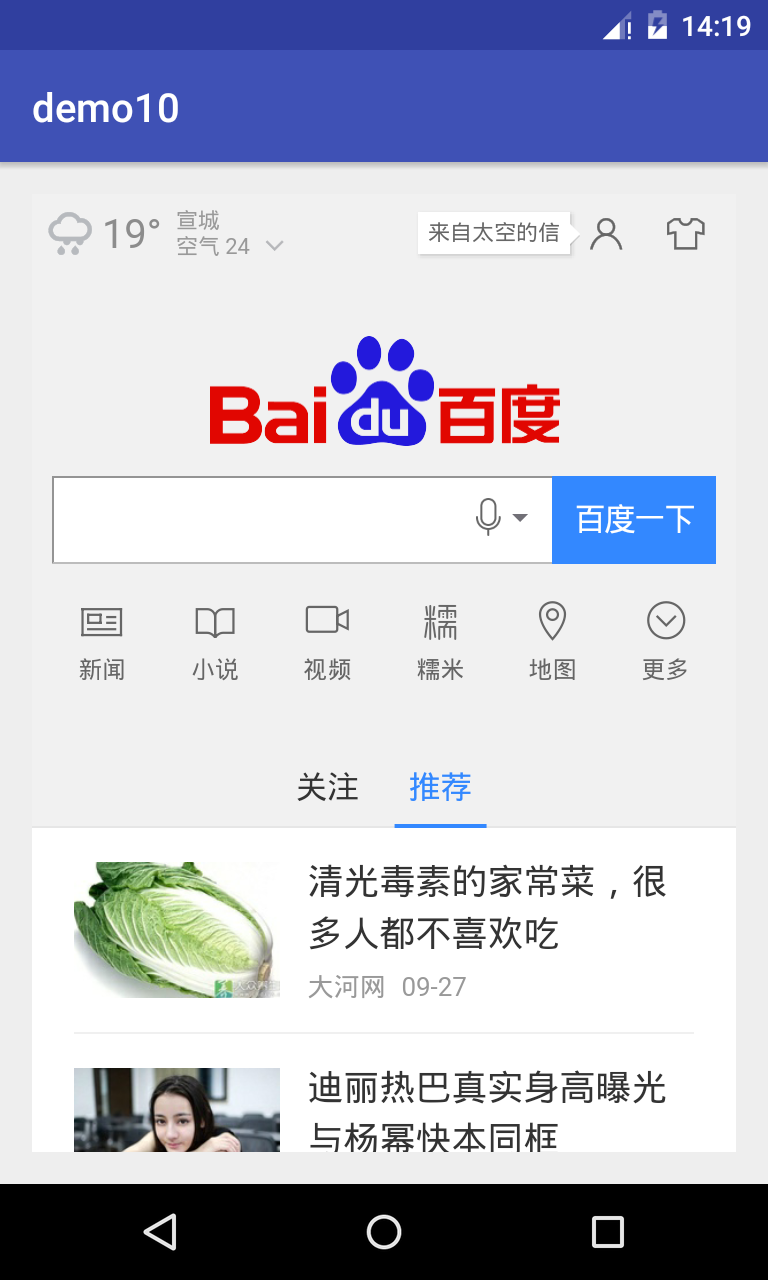
1.加入WebView
<WebView android:id="@+id/webView" android:layout_width="match_parent" android:layout_height="match_parent"> </WebView>
2.使用WebView加载页面
要用WebView加载页面,使用loadUrl()1)加载本地文件:
webView.loadUrl("file:///android_asset/xx.html"); //本地文件存放在assets文件中
2)加载web资源
webView.loadUrl("http://www.baidu.com");
是页面获得焦点
webView.requestFocus();
3.获取网络访问权限
在AndroidManifest.xml文件中添加: <uses-permission android:name="android.permission.INTERNET"/>
4.处理页面导航
当用户点击一个WebView中的页面的链接时,通常是由默认的浏览器打开并加载目标URL的。然而,你可以在WebView中覆盖这一行为,那么链接就会在WebView中打开。//WebView加载web资源 webView.loadUrl("http://www.baidu.com"); //覆盖webView默认通过第三方或者是系统浏览器打开网页的方法,使得网页可以在WebView中打开 webView.setWebViewClient(new WebViewClient(){ @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { //返回值是true的时候控制网页在WebView中去打开,如果为false则调用系统浏览器打开 view.loadUrl(url); return true; } });
5.在WebView中使用Javascript
如果你想要加载在WebView中的web页面使用Javascript,你需要在WebView中启用Javascript。去哦用Javascript,你可以通过WebView中带有的WebView来启用它。你可以通过getSettings()来获取WebSettings的值,然后通过setJavaScriptEnabled()来启用Javascript。//启用支持Javascript WebSettings webSettings = webView.getSettings(); webSettings.setJavaScriptEnabled(true);
6.后退与前进
当你的WebView覆盖了URL加载,它会自动生成历史访问记录,你可以通过goBack()或goForward()向前或向后访问已访问过的站点。//改写物理按键返回的逻辑 @Override public boolean onKeyDown(int keyCode, KeyEvent event) { if(keyCode == KeyEvent.KEYCODE_BACK) { if(webView.canGoBack()) { //如果WebView可以返回,则返回上一个页面 webView.goBack(); return true; } else { //退出 System.exit(0); } } return super.onKeyDown(keyCode, event); }
7.页面加载过程进度条
由于有些网页可能加载缓慢,所以我们需要去判断页面的加载过程,制作进度条给予用户良好的体验效果。//在网页打开过程中添加一个进度条对话框 webView.setWebChromeClient(new WebChromeClient(){ @Override public void onProgressChanged(WebView view, int newProgress) { //newProgress: 1~100之间的整数 if(newProgress == 100) { //网页加载完毕 closeDialog(); }else { //网页正在加载,打开ProgressDialog openDialog(newProgress); } } }); //关闭进度条对话框 private void closeDialog() { if(progressDialog != null && progressDialog.isShowing()) { progressDialog.dismiss();; progressDialog = null; } } //打开网页时显示进度条对话框 private void openDialog(int newProgress) { if(progressDialog == null) { progressDialog = new ProgressDialog(MainActivity.this); progressDialog.setTitle("正在加载..."); progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL); progressDialog.setProgress(newProgress); progressDialog.show(); } else { progressDialog.setProgress(newProgress); } }
8.WebView缓存的运用
使用缓存可以加快网页反应速度。优先使用缓存:
WebSettings webSettings = webView.getSettings(); //WebView加载页面优先使用缓存加载 webSettings.setCacheMode(WebSettings.LOAD_CACHE_ELSE_NETWORK);
不使用缓存:
//不使用缓存 webSettings.setCacheMode(WebSettings.LOAD_NO_CACHE);
看一个实例:
1.新建一个项目,在activity_main.xml中添加WebView
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main"
android:layout_width="match_parent" android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.demo10.MainActivity">
<WebView android:id="@+id/webView" android:layout_width="match_parent" android:layout_height="match_parent"> </WebView>
</RelativeLayout>
2.在MainActivity.java中添加代码:
package com.example.demo10;
import android.app.ProgressDialog;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.KeyEvent;
import android.webkit.WebChromeClient;
import android.webkit.WebSettings;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private WebView webView;
private ProgressDialog progressDialog;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
init();
}
private void init() {
webView = (WebView) findViewById(R.id.webView);
//WebView加载本地资源
//webView.loadUrl("file:///android_asset/index.html");
//WebView加载web资源 webView.loadUrl("http://www.baidu.com"); //覆盖webView默认通过第三方或者是系统浏览器打开网页的方法,使得网页可以在WebView中打开 webView.setWebViewClient(new WebViewClient(){ @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { //返回值是true的时候控制网页在WebView中去打开,如果为false则调用系统浏览器打开 view.loadUrl(url); return true; } });
//启用支持Javascript WebSettings webSettings = webView.getSettings(); webSettings.setJavaScriptEnabled(true);
//WebView加载页面优先使用缓存加载
webSettings.setCacheMode(WebSettings.LOAD_CACHE_ELSE_NETWORK);
//在网页打开过程中添加一个进度条对话框
webView.setWebChromeClient(new WebChromeClient(){
@Override
public void onProgressChanged(WebView view, int newProgress) {
//newProgress: 1~100之间的整数
if(newProgress == 100) { //网页加载完毕
closeDialog();
}else { //网页正在加载,打开ProgressDialog
openDialog(newProgress);
}
}
});
}
//关闭进度条对话框
private void closeDialog() {
if(progressDialog != null && progressDialog.isShowing()) {
progressDialog.dismiss();;
progressDialog = null;
}
}
//打开网页时显示进度条对话框
private void openDialog(int newProgress) {
if(progressDialog == null) {
progressDialog = new ProgressDialog(MainActivity.this);
progressDialog.setTitle("正在加载...");
progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
progressDialog.setProgress(newProgress);
progressDialog.show();
} else {
progressDialog.setProgress(newProgress);
}
}
//改写物理按键返回的逻辑
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
if(keyCode == KeyEvent.KEYCODE_BACK) {
Toast.makeText(MainActivity.this, webView.getUrl(), Toast.LENGTH_SHORT).show();
if(webView.canGoBack()) { //如果WebView可以返回,则返回上一个页面
webView.goBack();
return true;
} else { //退出
System.exit(0);
}
}
return super.onKeyDown(keyCode, event);
}
}
运行效果:
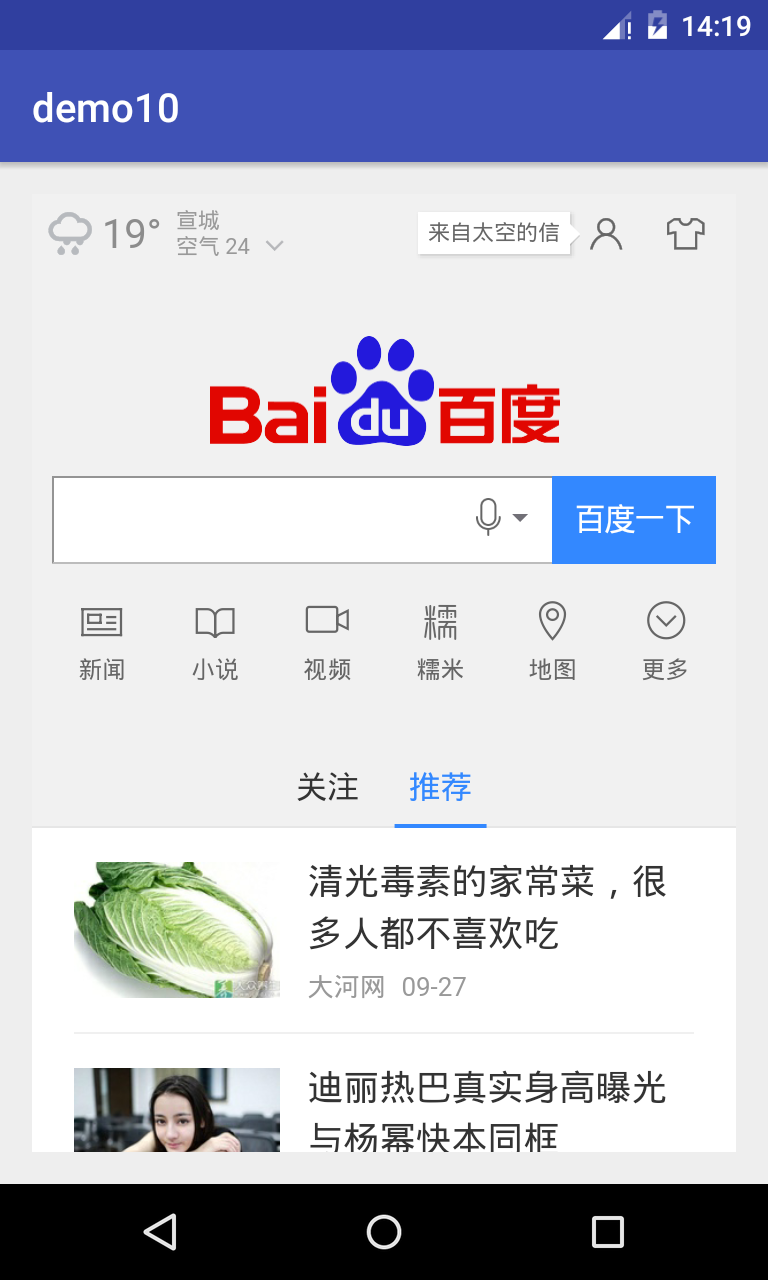
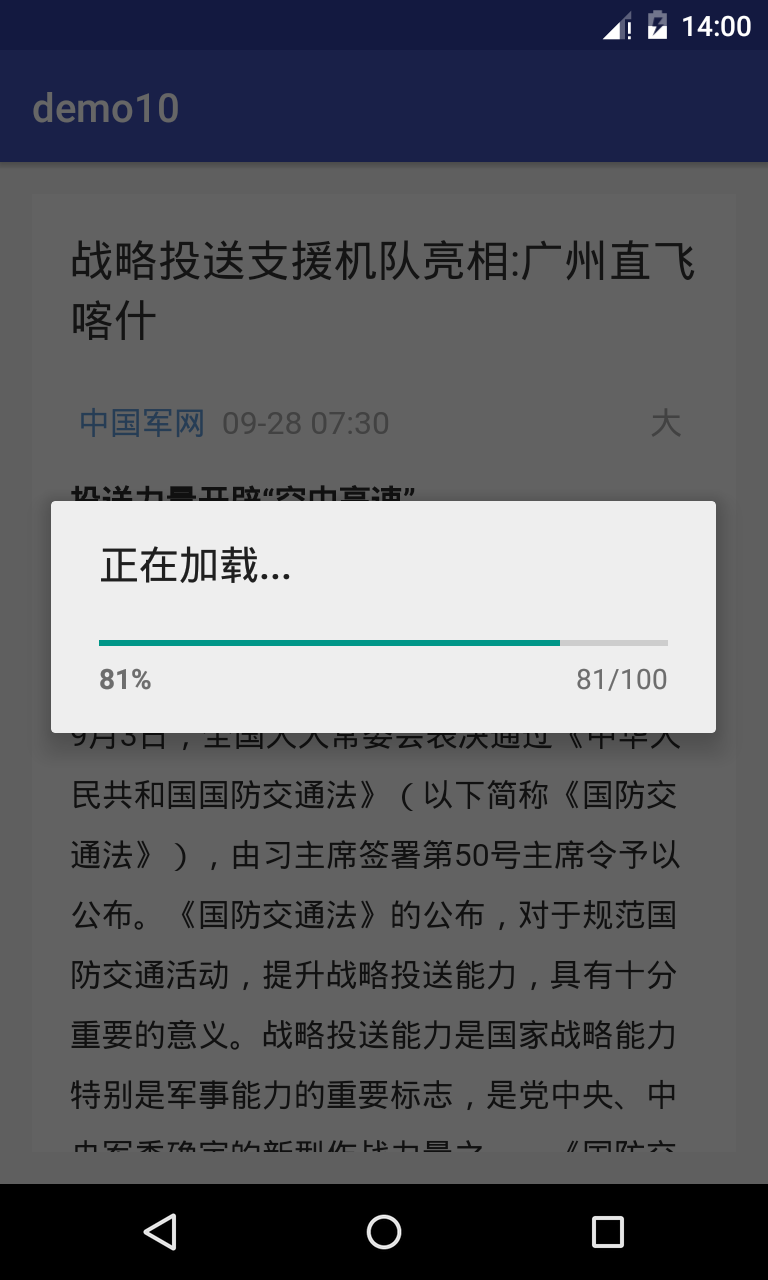
相关文章推荐
- 使用C++实现JNI接口需要注意的事项
- Android IPC进程间通讯机制
- Android Manifest 用法
- [转载]Activity中ConfigChanges属性的用法
- Android之获取手机上的图片和视频缩略图thumbnails
- Android之使用Http协议实现文件上传功能
- Android学习笔记(二九):嵌入浏览器
- android string.xml文件中的整型和string型代替
- i-jetty环境搭配与编译
- android之定时器AlarmManager
- android wifi 无线调试
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- android 代码实现控件之间的间距
- android FragmentPagerAdapter的“标准”配置
- Android"解决"onTouch和onClick的冲突问题
- android:installLocation简析
- android searchView的关闭事件
- SourceProvider.getJniDirectories