Hibernate入门_增删改查
2016-08-28 16:51
225 查看
一、Hibernate入门案例剖析:
①创建实体类Student 并重写toString方法
② 创建学生对象 并赋值
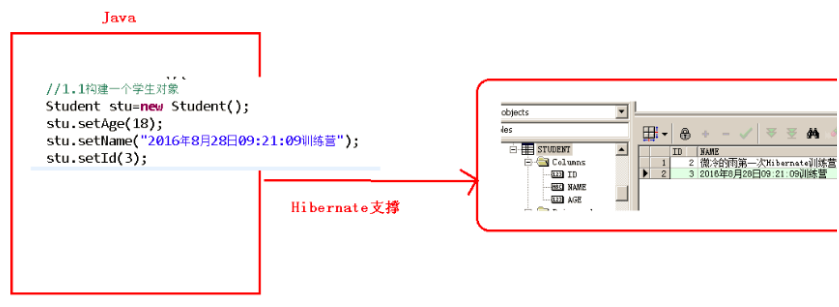
③引入jar包
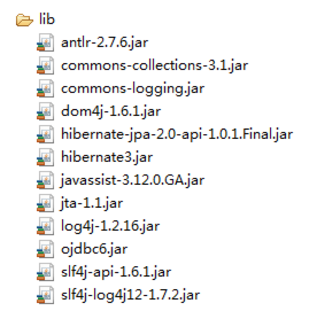
④ 构建大配置<hibernate.cfg.xml>
可分为以下步骤:
1.连接数据库的语句
2.sql方言
3.可省的配置(show_sql、format_sql 取值为true)
4.让程序生成底层数据表(hbm2ddl.auto) update/create。create是每次将数据表删除后,重新创建
5.关联小配置
<mapping resource="cn/happy/entity/Student.hbm.xml" />
关键代码如下:
⑤ 构建小配置(Student.hbm.xml)
⑥ 工具类HibernateUtil、构建私有静态的Configuration、SessionFactory对象、定义返回session以及关闭session的方法
⑦测试类【增删改查】 使用标记After、Before可简化代码
①创建实体类Student 并重写toString方法
public class Student { private Integer sid; private Integer age; private String name; public Integer getSid() { return sid; } public void setSid(Integer sid) { this.sid = sid; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } @Override public String toString() { return "Student [sid=" + sid + ", age=" + age + ", name=" + name + "]"; } }
② 创建学生对象 并赋值
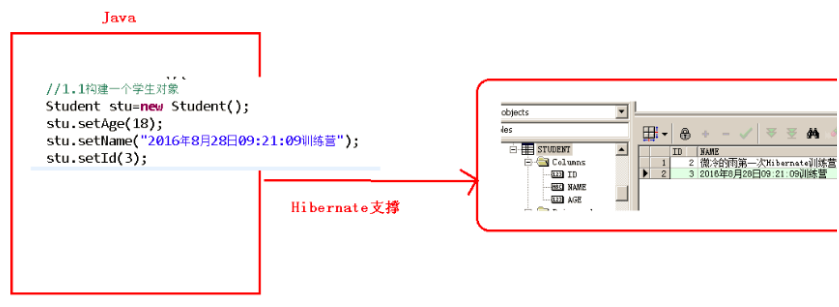
③引入jar包
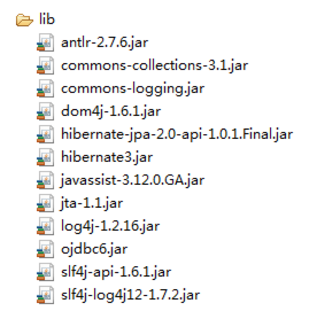
④ 构建大配置<hibernate.cfg.xml>
可分为以下步骤:
1.连接数据库的语句
2.sql方言
3.可省的配置(show_sql、format_sql 取值为true)
4.让程序生成底层数据表(hbm2ddl.auto) update/create。create是每次将数据表删除后,重新创建
5.关联小配置
<mapping resource="cn/happy/entity/Student.hbm.xml" />
关键代码如下:
<?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <!-- Database connection settings 数据库连接设置--> <!-- 驱动类 --> <property name="connection.driver_class">oracle.jdbc.OracleDriver</property> <!-- url地址 --> <property name="connection.url">jdbc:oracle:thin:@localhost:1521:orcl3</property> <property name="connection.username">wj</property> <property name="connection.password">9090</property> <!-- SQL dialect (SQL 方言) --> <property name="dialect">org.hibernate.dialect.Oracle10gDialect</property> <!--在控制台打印后台的SQL语句 --> <property name="show_sql">true</property> <!-- 格式化显示SQL --> <!-- <property name="format_sql">true</property> --> <!-- 自动生成student表 --> <property name="hbm2ddl.auto">update</property> <!-- 关联小配置 --> <mapping resource="cn/happy/entity/Student.hbm.xml" /> <!-- <mapping class="cn.happy.entity.Grade"/> --> </session-factory> </hibernate-configuration>
⑤ 构建小配置(Student.hbm.xml)
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping package="cn.happy.entity"> <class name="Student" table="STUDENT"> <id name="sid" column="SID"> <!-- 主键生成策略:native: native:如果后台是Oracle 后台是MySQL,自动应用自增 assigned:程序员给主键赋值 uuid:32位的16进制数 sequence native --> <generator class="assigned"> <param name="sequence">SEQ_NUM</param> </generator> </id> <!-- <version name="version"></version> --> <property name="name" type="string" column="NAME"/> <property name="age"/> </class> </hibernate-mapping>
⑥ 工具类HibernateUtil、构建私有静态的Configuration、SessionFactory对象、定义返回session以及关闭session的方法
private static Configuration cf=new Configuration().configure(); private static SessionFactory sf=cf.buildSessionFactory(); //方法返回session public static Session getSession(){ return sf.openSession(); } //关闭Session public static void CloseSession(){ getSession().close(); } 复制代码
⑦测试类【增删改查】 使用标记After、Before可简化代码
package cn.happy.test; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; import org.junit.After; import org.junit.Before; import org.junit.Test; import cn.happy.entity.Student; import cn.happy.util.HibernateUtil; public class Test1 { Session session; Transaction tx; @After public void afterTest(){ tx.commit(); HibernateUtil.CloseSession(); } @Before public void initData(){ session=HibernateUtil.getSession(); tx=session.beginTransaction(); } /* * get方法查询 */ @Test public void getData(){ Student stu=(Student)session.get(Student.class, 3); System.out.println(stu); } /* * 增加 */ @Test public void addData(){ Student stu=new Student(); stu.setSid(12); stu.setAge(11); stu.setName("李小龙1"); //读取大配置文件 获取连接信息 Configuration cfg=new Configuration().configure(); //创建SessionFactory SessionFactory fa=cfg.buildSessionFactory(); //加工Session Session se=fa.openSession(); Transaction tx = se.beginTransaction(); //保存 se.save(stu); //事务提交 tx.commit(); se.close(); System.out.println("Save ok!"); } /* * 删除 */ @Test public void delData(){ Session session=HibernateUtil.getSession(); Student stu=new Student(); stu.setSid(2); Transaction tx=session.beginTransaction(); session.delete(stu); tx.commit(); HibernateUtil.CloseSession(); System.out.println("del ok!"); } /* * 修改 */ @Test public void updateData(){ Session session=HibernateUtil.getSession(); Student stu=(Student)session.load(Student.class,3); stu.setName("呵呵"); Transaction tx=session.beginTransaction(); session.update(stu); tx.commit(); HibernateUtil.CloseSession(); System.out.println("update ok!"); } }
相关文章推荐
- spring入门(12)-spring与hibernate整合完成增删改查的操作(继承HibernateDaoSupport调用hibernateTemplate类)
- Rhythmk 学习 Hibernate 01 - maven 创建Hibernate 项目之 增删改查入门
- MyEclipe+Hibernate增删查改快速入门
- Hibernate-基础入门案例,增删改查
- Java自学笔记之Hibernate-Hibernate入门和单表增删改查
- hibernate 入门增删改查demo
- spring入门-spring与hibernate整合完成增删改查的操作(封装HibernateTemplate模版类对象)
- spring入门(12)-spring与hibernate整合完成增删改查的操作(继承HibernateDaoSupport调用hibernateTemplate类)
- Hibernate入门-搭建框架实现基本的增删改查
- 三大框架之hibernate入门学习教程增删改查
- spring入门之spring与hibernate整合完成增删改查的操作(封装HibernateTemplate模版类对象)
- spring入门(11)-spring与hibernate整合完成增删改查的操作(封装HibernateTemplate模版类对象)
- Hibernate入门案例及增删改查
- hibernate教程--快速入门(增删改查)
- hibernate入门教程2增删查改
- Hibernate入门案例及增删改查
- spring入门(11)-spring与hibernate整合完成增删改查的操作(封装HibernateTemplate模版类对象)
- 【Hibernate】Hibernate入门2-简单的增删改查源码
- Hibernate学习-03:入门案例(CRUD(增删改查)操作之添加记录)
- Hibernate入门案例及增删改查