《剑指offer》:[27]二叉搜索树与双向链表的转化过程
2016-06-09 21:36
453 查看
题目:输入一课二叉搜索树,将该二叉搜索树转换成一个排序的双向链表。要求不能创建任何新的结点,只能调整树中结点指针的指向。
因为树的结构和循环链表的结构十分类似,所以使它们之间的转换成为了可能。
具体方法:
原先指向左子结点的指针调整为链表中指向前一节点的指针;原先指向右子结点的指针调整为链表中指向后一个结点的指针。
因为要求转换之后的链表时排好序的,所以我们可以借助中序遍历。当遍历的时候我们将树看成三部分:根节点,左子树,右子树。
如下图:
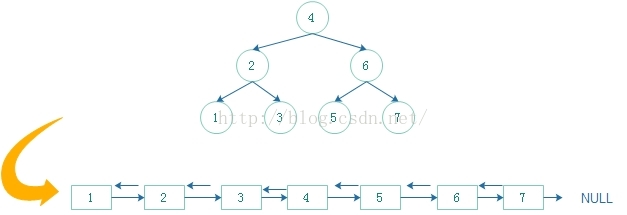
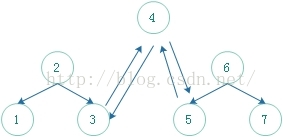
具体实现代码如下;
运行结果如下:
因为树的结构和循环链表的结构十分类似,所以使它们之间的转换成为了可能。
具体方法:
原先指向左子结点的指针调整为链表中指向前一节点的指针;原先指向右子结点的指针调整为链表中指向后一个结点的指针。
因为要求转换之后的链表时排好序的,所以我们可以借助中序遍历。当遍历的时候我们将树看成三部分:根节点,左子树,右子树。
如下图:
具体实现代码如下;
#include <iostream> using namespace std; struct BinaryTree { int data; BinaryTree *pLeft; BinaryTree *pRight; }; int arr[7]={4,2,6,1,3,5,7}; BinaryTree *pRoot=NULL; void InsertTree(BinaryTree *root,int data) { BinaryTree *node=root; if(node->data > data) { if(NULL==node->pLeft) { BinaryTree *pNode=new BinaryTree; pNode->data=data; pNode->pLeft=pNode->pRight=NULL; node->pLeft=pNode; } else InsertTree(node->pLeft,data); } else { if(NULL==node->pRight) { BinaryTree *pNode=new BinaryTree; pNode->data=data; pNode->pLeft=pNode->pRight=NULL; node->pRight=pNode; } else InsertTree(node->pRight,data); } } void CreateTree(BinaryTree **root,int *array,int length) { for(int i=0;i<7;i++) { if(NULL==*root) { BinaryTree *pNode=new BinaryTree; pNode->data=array[0]; pNode->pLeft=pNode->pRight=NULL; *root=pNode; } else { InsertTree(*root,array[i]); } } } void PreOrder(BinaryTree *root) { BinaryTree *temp=root; if(temp) { cout<<temp->data<<" "; PreOrder(temp->pLeft); PreOrder(temp->pRight); } } void ConvertHelp(BinaryTree *pNode,BinaryTree **pLastnode) { if(pNode==NULL) return ; BinaryTree *current=pNode; if(current->pLeft!=NULL) ConvertHelp(current->pLeft,pLastnode); current->pLeft=*pLastnode; if(*pLastnode!=NULL) (*pLastnode)->pRight=current; *pLastnode=current; if(current->pRight!=NULL) ConvertHelp(current->pRight,pLastnode); } BinaryTree *Convert(BinaryTree *root) { BinaryTree *pLastNode=NULL;//指向双向链表的尾结点; ConvertHelp(root,&pLastNode); //返回头结点 BinaryTree *pHeadList=pLastNode; while(pHeadList!=NULL && pHeadList->pLeft!=NULL) pHeadList=pHeadList->pLeft; return pHeadList; } void showList(BinaryTree *list) { BinaryTree *head=list; while(head) { cout<<head->data<<" "; head=head->pRight; } } int main() { CreateTree(&pRoot,arr,7); cout<<"树的前序遍历:"; PreOrder(pRoot); cout<<endl<<"循环链表的遍历:"; showList(Convert(pRoot)); cout<<endl; system("pause"); return 0; }
运行结果如下:
相关文章推荐
- CSS:给 input 中 type="text" 设置CSS样式
- 《剑指offer》:[26]复杂链表的复制
- 《剑指offer》:[25]二叉树中和为某一值的路径
- 《剑指offer》:[24]判断一个序列是否为二叉树的后序遍历序列
- 《CSS权威指南》学习记录——颜色和背景
- 《JavaScript学习笔记》:Ajax的应用
- CSS---解决浏览器兼容性
- html5实现本地图片预览功能
- 《剑指offer》:[23]从上往下打印二叉树
- 解决引入JS中文乱码问题
- 剑指offer(四十六)之树的子结构
- Ajax过程 事件代理
- JavaScript Interview Questions: Event Delegation and This
- 滚动条样式
- 丑数
- [Nutch]Hadoop动态增加DataNode节点和TaskTracker节点
- leetcode 19. Remove Nth Node From End of List
- CSS---利用伪类绘制倒三角形
- (转载)Bootstrap 栅格系统的精妙之处
- fastJSON使用(一)——对象转换成json