Android自定义带下划线的TextView
2016-06-06 00:00
513 查看
Android自定义带下划线的TextView
本例是从本博客android客户端中抠出来的一个组件,代码很简单,实现一个带下划线的文本框。先看效果图:
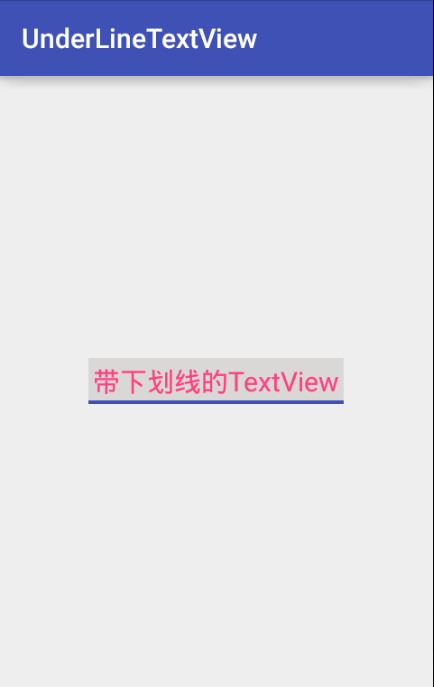
我们可以通过继承TextView,然后再绘制的时候,底部多绘制一个下划线即可。
我们希望可以直接在xml中配置下划线颜色和高度,我们需要在values资源目录下的attrs.xml(没有请新建)中定义好我们要自定义的属性:
xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="UnderlineTextView"> <attr name="underline_color" format="color"/> <attr name="underline_height" format="dimension"/> </declare-styleable> </resources>
可以看到有两个字段,分别是下划线的颜色和高度。
接下来就是编写UnderLineTextView这个类了,具体的解释都放到代码注释里面了,这样大家看起来也更清晰:
package com.anxpp.underlinetextview; import android.content.Context; import android.content.res.TypedArray; import android.graphics.Canvas; import android.graphics.Paint; import android.util.AttributeSet; import android.util.TypedValue; import android.widget.TextView; /** * 带下划线的TextView * 可以设置下划线高度和颜色 * 可以按需扩展其他属性(如下划线宽度等) * * @author http://anxpp.com/ */ public class UnderlineTextView extends TextView { //Paint即画笔,在绘图过程中起到了极其重要的作用,画笔主要保存了颜色, //样式等绘制信息,指定了如何绘制文本和图形,画笔对象有很多设置方法, //大体上可以分为两类,一类与图形绘制相关,一类与文本绘制相关 private final Paint paint = new Paint(); //下划线高度 private int underlineHeight = 0; //下划线颜色 private int underLineColor; //通过new创建实例是调用这个构造函数 //这种情况下需要添加额外的一些函数供外部来控制属性,如set*(...); public UnderlineTextView(Context context) { this(context, null); } //通过XML配置但不定义style时会调用这个函数 public UnderlineTextView(Context context, AttributeSet attrs) { this(context, attrs, 0); //获取自定义属性 TypedArray typedArray = context.obtainStyledAttributes(attrs,R.styleable.UnderlineTextView); //获取具体属性值 underLineColor = typedArray.getColor(R.styleable.UnderlineTextView_underline_color, getTextColors().getDefaultColor()); underlineHeight = (int)typedArray.getDimension(R.styleable.UnderlineTextView_underline_height, TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP, 2, getResources().getDisplayMetrics())); } //通过XML配置且定义样式时会调用这个函数 public UnderlineTextView(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); } //防止下划线高度大到一定值时会覆盖掉文字,需从写此方法 @Override public void setPadding(int left, int top, int right, int bottom) { super.setPadding(left, top, right, bottom + underlineHeight); } //绘制下划线 @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); //设置下划线颜色 paint.setColor(underLineColor); //float left, float top, float right, float bottom canvas.drawRect(0, getHeight() - underlineHeight, getWidth(), getHeight(), paint); } }
然后在布局文件中添加这个控件:
xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <com.anxpp.underlinetextview.UnderlineTextView android:text="@string/title" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:padding="3dp" android:textSize="20sp" android:textColor="@color/colorAccent" android:background="#dad7d7" app:underline_color="@color/colorPrimary" app:underline_height="3dp"/> </RelativeLayout>
可以看到这里是配置了两个属性的,用到的颜色:
xml version="1.0" encoding="utf-8"?> <resources> <color name="colorPrimary">#3F51B5</color> <color name="colorPrimaryDark">#303F9F</color> <color name="colorAccent">#FF4081</color> </resources>
OK,控件就写好了,如果希望不仅仅能在XML中配置属性,可以在UnderlineTextView类中扩展相应的功能即可。
github:https://github.com/anxpp/UnderLineTextView.git
源码下载:UnderLineTextView.7z
相关文章推荐
- Android 之创建内容提供程序
- Android 之Intent和Intent过滤器
- Android 之Fragment
- Android 之绑定服务
- Android 之联系人提供程序
- Android 之Tasks和Back Stack(任务和返回栈)
- Android 之Loader(加载器)
- Android 之Overview Screen(任务列表)
- Android 之Activity
- 版本扫盲及最新android studio下载
- Android 之常见Intent
- Android数据的四种存储方式一 —— SharedPreference
- Android 之Service
- Android 之内容提供程序基础
- Android中AsyncTask的简单用法
- 使用 Android Studio自定义View04——视频音量调控
- Android四角圆形背景
- Android 屏幕分辨率工具类使用
- 使用服务注册广播接收者
- geekband android #5 第十四周分享(设计模式)