Quickhit快速击键
2016-05-30 15:23
429 查看
一、项目分析
根据输入速率和正确率将玩家分为不同等级,级别越高,一次显示的字符数越多,玩家正确输入一次的得分也越高。如果玩家在规定时间内完成规定次数的输入,正确率达到规定要求,则玩家升级。玩家最高级别为6级,初始级别一律为一级!
二、掌握的技能点
①面向对象设计的思想
②使用类图理解类的关系
③类的封装
④构造方法的使用
⑤this和static关键字的使用
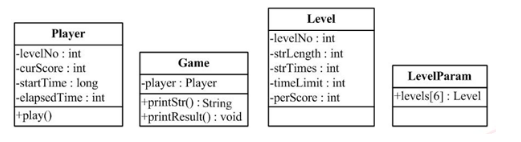
类的属性:
①玩家(Player)类的属性:当前级别号levelNo、当前级别积分currScore、当前级别开始时间startTime和当前级别已用时间elapsedTime
②级别(Level)类的属性:各级别编号levelNo、各级别一次输出字符串的长度strLength、各级别输出字符串的次数strTime、各级别闯关的时间限制timeLimit和各级别正确输入一次得分
类的方法:
游戏Game类的主要方法有2个:输出字符串、返回字符串用于和玩家的输入进行比较[String printStr]确认玩家输入是否正确[void printResult(String out,String in)],比较游戏输出out和玩家输入in
玩家Player类的方法:玩游戏play()
LevelParam类:定义一个长度为6的Level数组,用来存放各级别的具体参数信息
关键代码:
Player类:
Level类:
LevelParam类
Game类:
测试Test类:
-------------------------------------------------------------------------------------------------------------
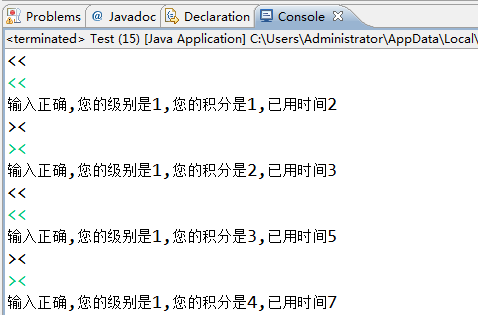
------------------------------------------------------------------------------------------------

----------------------------------------------------------------------------------------------------
根据输入速率和正确率将玩家分为不同等级,级别越高,一次显示的字符数越多,玩家正确输入一次的得分也越高。如果玩家在规定时间内完成规定次数的输入,正确率达到规定要求,则玩家升级。玩家最高级别为6级,初始级别一律为一级!
二、掌握的技能点
①面向对象设计的思想
②使用类图理解类的关系
③类的封装
④构造方法的使用
⑤this和static关键字的使用
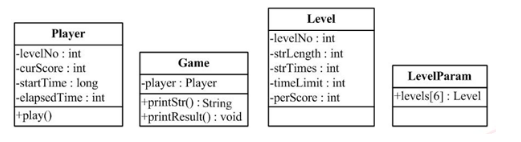
类的属性:
①玩家(Player)类的属性:当前级别号levelNo、当前级别积分currScore、当前级别开始时间startTime和当前级别已用时间elapsedTime
②级别(Level)类的属性:各级别编号levelNo、各级别一次输出字符串的长度strLength、各级别输出字符串的次数strTime、各级别闯关的时间限制timeLimit和各级别正确输入一次得分
类的方法:
游戏Game类的主要方法有2个:输出字符串、返回字符串用于和玩家的输入进行比较[String printStr]确认玩家输入是否正确[void printResult(String out,String in)],比较游戏输出out和玩家输入in
玩家Player类的方法:玩游戏play()
LevelParam类:定义一个长度为6的Level数组,用来存放各级别的具体参数信息
关键代码:
Player类:
package cn.happy; import java.util.Scanner; public class Player { public int levelNo; //级别号 public int curScore; //积分 public long startTime; //开始时间 public int elapsedTime; //已用时间 public int getLevelNo() { return levelNo; } public void setLevelNo(int levelNo) { this.levelNo = levelNo; } public int getCurScore() { return curScore; } public void setCurScore(int curScore) { this.curScore = curScore; } public long getStartTime() { return startTime; } public void setStartTime(long startTime) { this.startTime = startTime; } public int getElapsedTime() { return elapsedTime; } public void setElapsedTime(int elapsedTime) { this.elapsedTime = elapsedTime; } public Player() {} public Player(int levelNo,int curScore,long startTime,int elapsedTime) { this.levelNo=levelNo; this.curScore=curScore; this.startTime=startTime; this.elapsedTime=elapsedTime; } //玩游戏的方法 public void play() { Game game=new Game(this); Scanner input=new Scanner(System.in); //外层循环,循环一次级别晋一级 for (int i = 0; i < LevelParam.levels.length; i++) { //晋级 this.levelNo+=1; //晋级后计时清零,积分清零 this.startTime=System.currentTimeMillis(); this.curScore=0; //内层循环 循环一次完成一次字符串的输入,输出,比较 for (int j = 0; j < LevelParam.levels[levelNo-1].getStrTimes(); j++) { //游戏输出字符串 String outstr=game.printStr(); //接收用户输入 String instr=input.next(); //游戏判断玩家输入的是否正确 game.printResult(outstr,instr); } } } }
Level类:
package cn.happy; public class Level { public int levelNo; //各级别编号 public int strLength;// 各级别一次输出字符串的长度 public int strTimes;// 各级别输出字符串的次数 public int timeLimit;// 各级别闯关的时间限制 public int perScore;// 各级别正确输入一次的得分 public int getLevelNo() { return levelNo; } public void setLevelNo(int levelNo) { this.levelNo = levelNo; } public int getStrLength() { return strLength; } public void setStrLength(int strLength) { this.strLength = strLength; } public int getStrTimes() { return strTimes; } public void setStrTimes(int strTimes) { this.strTimes = strTimes; } public int getTimeLimit() { return timeLimit; } public void setTimeLimit(int timeLimit) { this.timeLimit = timeLimit; } public int getPerScore() { return perScore; } public void setPerScore(int perScore) { this.perScore = perScore; } public Level() {} public Level(int levelNo,int strLength,int strTimes,int timeLimit,int perScore) { this.levelNo=levelNo; this.strLength = strLength; this.strTimes = strTimes; this.timeLimit = timeLimit; this.perScore = perScore; } }
LevelParam类
package cn.happy; public class LevelParam { //级别参数类,配置各个级别参数 //对应6个级别 public final static Level levels[]=new Level[6]; static{ levels[0]=new Level(1,2,10,30,1); levels[1]=new Level(2,3,9,26,2); levels[2]=new Level(3,4,8,22,5); levels[3]=new Level(4,5,7,18,8); levels[4]=new Level(5,6,6,15,10); levels[5]=new Level(6,7,5,12,15); } }
Game类:
package cn.happy; import java.util.Random; public class Game { //玩家 private Player player; public Game() {} public Game(Player player) { this.player=player; } public String printStr() { // 获取级别对应的要输出字符串的长度 int strLength = LevelParam.levels[player.getLevelNo() -1].getStrLength(); StringBuffer buffer=new StringBuffer(); Random random=new Random(); //通过循环生成要输出的字符串 for (int i = 0; i < strLength; i++) { //产生随机数 int rand=random.nextInt(strLength); //根据随机数拼接字符串 switch(rand) { case 0: buffer.append(">"); break; case 1: buffer.append("<"); break; case 2: buffer.append("*"); break; case 3: buffer.append("$"); break; case 4: buffer.append("%"); break; case 5: buffer.append("#"); break; } } // 输出字符串 System.out.println(buffer); // 返回该字符串的值,用于和用户输入字符串的值作比较 return buffer.toString(); } //判断玩家输入的是否正确,并输出相应的结果 public String printResult(String outstr,String instr) { boolean flag=false; if(outstr.equals(instr)) { flag=true; } else { System.out.println("输入错误!哈哈"); System.exit(0); } if(flag) { long currentTime=System.currentTimeMillis(); //如果超时 if((currentTime-player.getStartTime())/100>LevelParam.levels[player.getLevelNo()-1].getTimeLimit()) { System.out.println("你输入的太慢了,已经超时,退出!"); System.exit(1); } //计算玩家当前积分 player.setCurScore(player.getCurScore()+LevelParam.levels[player.getLevelNo()-1].getPerScore()); //计算玩家已用时间 player.setElapsedTime((int)(currentTime-player.getStartTime())/1000); //输出玩家当前级别,当前积分,当前时间 System.out.println("输入正确,您的级别是"+player.levelNo+",您的积分是"+player.curScore+",已用时间"+player.elapsedTime+""); } return "hh"; } }
测试Test类:
package cn.happy; public class Test { /** * @param args */ public static void main(String[] args) { Player player=new Player(); player.play(); } }
-------------------------------------------------------------------------------------------------------------
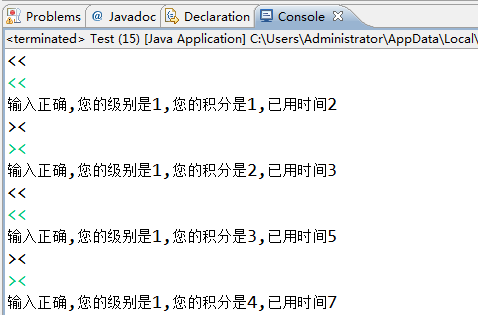
------------------------------------------------------------------------------------------------

----------------------------------------------------------------------------------------------------
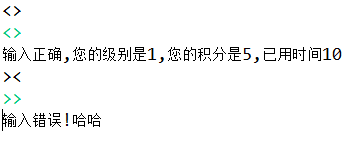
相关文章推荐
- request对象
- StringBuilder和StringBuffer源码分析
- Android Studio 2.2 预览版 - 全新的 UI 设计器和约束布局
- iOS开发 - 第02篇 - UI进阶 - 08 - 私人通讯录
- SQLSERVER TRUE、FALSE、UNKNOWN
- The constructor BASE64Encoder() is not accessible due to restriction on required
- iOS开发之:dispatch_async 与 dispatch_get_global_queue 的使用方法
- ArrayBlockingQueue源码中为什么方法要用局部变量引用类变量
- IBM MQQueueManager 直接连接
- Java中String、StringBuffer、StringBuilder的区别及面试经常出现的问题
- 存储过程 返回值 procedure return values
- Java中String、StringBuffer、StringBuilder的区别及面试经常出现的问题
- PKIX path building failed 的问题
- ueditor 跨域上传问题
- DECLARE_WAITQUEUE(wait, current)的分析
- UI进阶 SQLite错误码
- UIDatePicker 轮转日期选择器 时间选择器
- 解决UIScrollView和滑动返回手势的冲突
- Android高级UI GestureDetector监听各种手势
- ValidateRequest="false"