面向对象的JavaScript-008-Function介绍
2016-05-24 17:04
555 查看
1.
// 函数 /* Declare the function 'myFunc' */ function myFunc(theObject) { theObject.brand = "Toyota"; } /* * Declare variable 'mycar'; * create and initialize a new Object; * assign reference to it to 'mycar' */ var mycar = { brand: "Honda", model: "Accord", year: 1998 }; /* Logs 'Honda' */ console.log(mycar.brand); /* Pass object reference to the function */ myFunc(mycar); /* * Logs 'Toyota' as the value of the 'brand' property * of the object, as changed to by the function. */ console.log(mycar.brand); //var y = function x() {}; //alert(x); // throws an error //var foo = new Function("alert(anonymous);"); //foo(); //Uncaught ReferenceError: anonymous is not defined foo(); // alerts FOO! function foo() { alert('FOO!'); } var foo = (new Function("var bar = \'FOO!\';\nreturn(function() {\n\talert(bar);\n});"))(); foo(); // The segment "function() {\n\talert(bar);\n}" of the function body string is not re-parsed. var x = 0; // source element if (x == 0) { // source element x = 10; // not a source element function boo() {} // not a source element } function foo() { // source element var y = 20; // source element function bar() {} // source element while (y == 10) { // source element function blah() {} // not a source element y++; // not a source element } } // function declaration function foo() {} // function expression (function bar() {}) // function expression x = function hello() {} if (x) { // function expression function world() {} } // function declaration function a() { // function declaration function b() {} if (0) { // function expression function c() {} } } // This function returns a string padded with leading zeros function padZeros(num, totalLen) { var numStr = num.toString(); // Initialize return value as string var numZeros = totalLen - numStr.length; // Calculate no. of zeros for (var i = 1; i <= numZeros; i++) { numStr = "0" + numStr; } return numStr; } var result; result = padZeros(42,4); // returns "0042" console.log(result); result = padZeros(42,2); // returns "42" console.log(result); result = padZeros(5,4); // returns "0005" console.log(result); // 菲波那其数 var factorial = function fac(n) { return n<2 ? 1 : n*fac(n-1) }; console.log(factorial(3)); function map(f,a) { var result = [], // Create a new Array i; for (i = 0; i != a.length; i++) result[i] = f(a[i]); return result; } console.log(map(function(x) {return x * x * x}, [0, 1, 2, 5, 10])); // returns [0, 1, 8, 125, 1000]. // 阶乘 function factorial(n){ if ((n === 0) || (n === 1)) return 1; else return (n * factorial(n - 1)); } var a, b, c, d, e; a = factorial(1); // a gets the value 1 b = factorial(2); // b gets the value 2 c = factorial(3); // c gets the value 6 d = factorial(4); // d gets the value 24 e = factorial(5); // e gets the value 120 console.log(a); console.log(b); console.log(c); console.log(d); console.log(e); // Function scope // The following variables are defined in the global scope var num1 = 20, num2 = 3, name = "Chamahk"; // This function is defined in the global scope function multiply() { return num1 * num2; } multiply(); // Returns 60 // A nested function example function getScore () { var num1 = 2, num2 = 3; function add() { return name + " scored " + (num1 + num2); } return add(); } console.log(getScore()); // Returns "Chamahk scored 5" var x = 0; while (x < 10) { // "x < 10" is the loop condition // do stuff x++; } // can be converted into a recursive function and a call to that function: function loop(x) { if (x >= 10) // "x >= 10" is the exit condition (equivalent to "!(x < 10)") return; // do stuff loop(x + 1); // the recursive call } loop(0); // However, some algorithms cannot be simple iterative loops. For example, getting all the nodes of a tree structure (e.g. the DOM) is more easily done using recursion: function walkTree(node) { if (node == null) // return; // do something with node for (var i = 0; i < node.childNodes.length; i++) { walkTree(node.childNodes[i]); } } // function foo(i) { // if (i < 0) // return; // console.log('begin:' + i); // foo(i - 1); // console.log('end:' + i); // } //foo(3); // Output: // begin:3 // begin:2 // begin:1 // begin:0 // end:0 // end:1 // end:2 // end:3 // Nested functions and closures function addSquares(a,b) { function square(x) { return x * x; } return square(a) + square(b); } a = addSquares(2,3); // returns 13 b = addSquares(3,4); // returns 25 c = addSquares(4,5); // returns 41 // Since the inner function forms a closure, you can call the outer function and specify arguments for both the outer and inner function: function outside(x) { function inside(y) { return x + y; } return inside; } fn_inside = outside(3); // Think of it like: give me a function that adds 3 to whatever you give it result = fn_inside(5); // returns 8 result1 = outside(3)(5); // returns 8 // Multiply-nested functions function A(x) { function B(y) { function C(z) { console.log(x + y + z); } C(3); } B(2); } A(1); // logs 6 (1 + 2 + 3) // Name conflicts function outside() { var x = 10; function inside(x) { return x; } return inside; } result = outside()(20); // returns 20 instead of 10 // Closures var pet = function(name) { // The outer function defines a variable called "name" var getName = function() { return name; // The inner function has access to the "name" variable of the outer function } return getName; // Return the inner function, thereby exposing it to outer scopes }, myPet = pet("Vivie"); myPet(); // Returns "Vivie" var createPet = function(name) { var sex; return { setName: function(newName) { name = newName; }, getName: function() { return name; }, getSex: function() { return sex; }, setSex: function(newSex) { if(typeof newSex === "string" && (newSex.toLowerCase() === "male" || newSex.toLowerCase() === "female")) { sex = newSex; } } } } var pet = createPet("Vivie"); pet.getName(); // Vivie pet.setName("Oliver"); pet.setSex("male"); pet.getSex(); // male pet.getName(); // Oliver var getCode = (function(){ var secureCode = "0]Eal(eh&2"; // A code we do not want outsiders to be able to modify... return function () { return secureCode; }; })(); getCode(); // Returns the secureCode var createPet = function(name) { // Outer function defines a variable called "name" return { setName: function(name) { // Enclosed function also defines a variable called "name" name = name; // ??? How do we access the "name" defined by the outer function ??? } } } // Using the arguments object function myConcat(separator) { var result = "", // initialize list i; // iterate through arguments for (i = 1; i < arguments.length; i++) { result += arguments[i] + separator; } return result; } // returns "red, orange, blue, " myConcat(", ", "red", "orange", "blue"); // returns "elephant; giraffe; lion; cheetah; " myConcat("; ", "elephant", "giraffe", "lion", "cheetah"); // returns "sage. basil. oregano. pepper. parsley. " myConcat(". ", "sage", "basil", "oregano", "pepper", "parsley"); // Default parameters function multiply(a, b) { b = typeof b !== 'undefined' ? b : 1; return a*b; } multiply(5); // 5 // function multiply2(a, b = 1) { // return a*b; // } // multiply2(5); // 5 // Rest parameters // function multiply(multiplier, ...theArgs) { // return theArgs.map(x => multiplier * x); // } // var arr = multiply(2, 1, 2, 3); // console.log(arr); // [2, 4, 6] // Arrow functions var a = [ "Hydrogen", "Helium", "Lithium", "Beryllium" ]; var a2 = a.map(function(s){ return s.length }); // var a3 = a.map( s => s.length ); // Lexical this function Person() { // The Person() constructor defines `this` as itself. this.age = 0; setInterval(function growUp() { // In nonstrict mode, the growUp() function defines `this` // as the global object, which is different from the `this` // defined by the Person() constructor. this.age++; }, 1000); } var p = new Person(); function Person() { var self = this; // Some choose `that` instead of `self`. // Choose one and be consistent. self.age = 0; setInterval(function growUp() { // The callback refers to the `self` variable of which // the value is the expected object. self.age++; }, 1000); } // function Person(){ // this.age = 0; // setInterval(() => { // this.age++; // |this| properly refers to the person object // }, 1000); // } // var p = new Person();
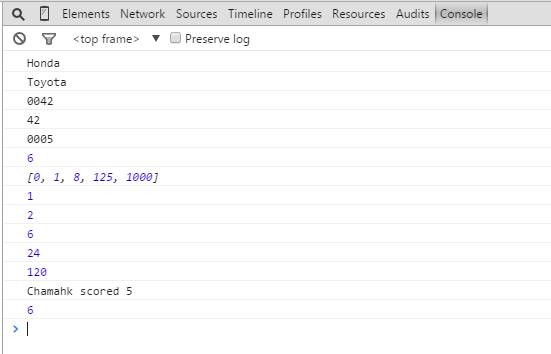
相关文章推荐
- JSON解析
- javascript之BOM事件注册和案例
- javascript之BOM事件注册和案例
- javascript jsscript .js xml html json soap
- net.sf.json.JSONException: Unquotted string "E44C2B0168A550F6503F59CCD56FC49B"
- 对JavaScript 中数组用法的总结
- JSP中如何自己写分页
- FK JavaScript之:ArcGIS JavaScript添加Graphic,地图界面却不显示
- JSON详解
- 异步加载的JS如何在chrome浏览器断点调试?
- jsp常用标签
- JavaScript学习
- JS+Canvas绘制时钟效果
- fastjson转String
- js学习笔记之锚点的用法
- jsp富文本编辑框截取前三十个文字内容作为内容摘要
- function函数部分
- javascript中文网学习
- [RxJS] What RxJS operators are
- javaScript异常处理