邮件发送模型及其Python应用实例
2016-05-21 13:51
459 查看
SMTP(Simple Mail Transfer Protocol)
制定:First:RFC 788 in 1981
Last:RFC 5321 in 2008
端口:
TCP 25(SMTP), TCP 465/587(SMTP_SSL)
功能:
用户客户端:
发送消息:SMTP
接收和管理消息:POP3、IMAP
邮件服务器:
发送和接收消息:SMTP
说明:
SMTP仅定义了消息传输格式(如消息发送者参数),而非消息内容(如消息头和消息体)。
邮件发送模型
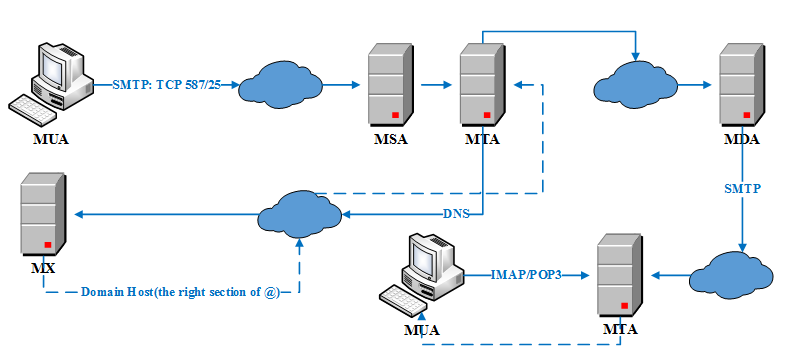
流程描述:
(1) 使用SMTP协议,通过MUA(Mail User Agent)提交邮件到MSA(Mail Submission Agent),然后MSA递送邮件到MTA(Mail Transfer Agent)[通常而言,MSA和MTA是同一邮件服务器上的两个不同例程,其也被称为SMTP服务器]。
(2) MTA通过DNS在MX(Mail Exchanger Record)上查询接收者的邮件服务器。
(3) MTA发送邮件到目标邮件服务器MTA,其间可能是两个MTA直接通信,或者经过多个SMTP服务器,最终到达MDA(Mail Delivery Agent)。
(4) MDA直接递送或者通过SMTP协议,将邮件递送到本地MTA。
(5) 终端应用程序通过IMAP/POP3协议接收和管理邮件。
SMTP协议
SMTP由3部分构成:MAIL:建立发送者地址。
RCPT:建立接收者地址。
DATA:标志邮件内容的开始。邮件内容由两部分构成:邮件头和以空行分割的邮件体。DATA是一组命令,服务器需要做两次回复:第一次回复表明其准备好接收邮件,第二次回复表明其接收/拒绝整个邮件。
一个简单的SMTP发送示例(主要为了说明SMTP的3个部分),如sender给receiver1和receiver2发送邮件:
S:表示SMTP服务器 C:表示SMTP客户端
客户端C建立TCP连接后,服务端S通过greeting建立会话(附带服务器的FQDN(Fully Qualified Domain Name)),客户端C通过HELLO响应会话(附带客户端的FQDN)。
# 建立会话 S: 220 smtp.example.com ESMTP Postfix C: HELO client.example.org S: 250 Hello client.example.org, I am glad to meet you # 发送消息 C: MAIL FROM:<sender@example.org> S: 250 Ok C: RCPT TO:<receiver1@example.com> S: 250 Ok C: RCPT TO:<receiver2@example.com> S: 250 Ok C: DATA S: 354 End data with <CR><LF>.<CR><LF> C: From: "Sender Example" <sender@example.org> C: To: "Receiver1 Example" <receiver1@example.com> C: Cc: receiver2@example.com C: Date: Tue, 15 January 2008 16:02:43 -0500 C: Subject: Test message C: C: Hello Receiver1. C: This is a test message with 5 header fields and 4 lines in the message body. C: Your friend, C: Sender C: . S: 250 Ok: queued as 12345 C: QUIT S: 221 Bye {The server closes the connection}
Python邮件模块
python中邮件发送使用smtplib模块,其一般流程为:初始化连接->发送邮件->退出。其中包含的类有SMTP,SMTP_SSL,LMTP。如上SMTP中的会话示例在python中对应的部分如下:C: HELO client.example.org --- SMTP.helo([hostname]): 使用HELO向SMTP服务器标识自己。 S: 250 Hello client.example.org, I am glad to meet you --- SMTP.verify(address):服务器用来验证地址的有效性,返回一个元组(250,相关地址)或者(>=400, 错误消息字符串)。 C: MAIL FROM:<sender@example.org> --- low-level methods: mail() C: RCPT TO:<receiver1@example.com> --- low-level methods: rcpt() C: DATA --- low-level methods: data() C: QUIT --- SMTP.quit(): 终止会话,关闭连接。
Python Email Demos
# -*- coding:utf-8 -*- from __future__ import print_function from __future__ import with_statement __author__ = 'xxx' __all__ = ['BTEmail'] import smtplib import sys import os import time import mimetypes from email import encoders from email.header import Header from email.utils import parseaddr, formataddr from email.mime.text import MIMEText from email.mime.multipart import MIMEMultipart from email.mime.image import MIMEImage from email.mime.audio import MIMEAudio from email.mime.base import MIMEBase # ----- The following information should be in the configuration file. from_addr = 'xxx@sina.com' to_addrs = ['yyy@126.com', 'zzz@163.com'] cc_addrs = ['xxx@sina.com', 'www@qq.com'] bcc_addrs = [] username = 'xxx@sina.com' password = '---' # ------------------------------------------------------------------------------------ class BTEmail(object): def __init__(self, host='smtp.sina.com', port=465, username=None, password=None): self.host = host self.port = port self.username = username self.password = password @classmethod def _format_addr(cls, envolope_string): # parseaddr() only parses single address or string. (name, addr) = parseaddr(envolope_string) return formataddr((\ Header(name, 'utf-8').encode(),\ addr.encode('utf-8') if isinstance(addr, unicode) else addr)) @classmethod def construct_msg(cls, contentfile, contenttype='html', charset='utf-8', attach_flag=False, attachpath=None): with open(contentfile, 'rb') as fp: content = MIMEText(fp.read(), contenttype, charset) if not attach_flag: msg = content else: # Construct root container. msg = MIMEMultipart() # Attach mail contents to the container. msg.attach(content) # Attach attachments to the container. for filename in os.listdir(attachpath): item = os.path.join(attachpath, filename) if not os.path.isfile(item): continue # Guess the content type based on the file's extension. (ctype, encoding) = mimetypes.guess_type(item) if ctype is None or encoding is not None: ctype = 'application/octet-stream' (maintype, subtype) = ctype.split('/', 1) # Load and encode attachments. """ # Use MIMEBase class construct attachments. with open(item, 'rb') as fp: attachment = MIMEBase(_maintype=maintype, _subtype=subtype) attachment.set_payload(fp.read()) encoders.encode_base64(attachment) """ # Use concrete type class construct attachments. if maintype == 'text': with open(item, 'rb') as fp: attachment = MIMEText(fp.read(), _subtype=subtype) elif maintype == 'image': with open(item, 'rb') as fp: attachment = MIMEImage(fp.read(), _subtype=subtype) elif maintype == 'audio': with open(item, 'rb') as fp: attachment = MIMEAudio(fp.read(), _subtype=subtype) else: with open(item, 'rb') as fp: attachment = MIMEBase(_maintype=maintype, _subtype=subtype) attachment.set_payload(fp.read()) encoders.encode_base64(attachment) # Set attachment header. attachment.add_header('Content-Disposition', 'attachment', filename=filename) msg.attach(attachment) return msg @classmethod def make_envolope(cls, msg, from_addr, to_addrs, subject='A Simple SMTP Demo'): if msg: msg['From'] = cls._format_addr(u'BT<%s>' % from_addr) # msg['To'] should be a string, not a list. msg['To'] = ','.join(to_addrs) msg['Cc'] = ','.join(cc_addrs) msg['Bcc'] = ','.join(bcc_addrs) msg['Subject'] = Header(u'主题<%s>' % subject, 'utf-8').encode() def send_mail(self, from_addr, to_addrs, msg): smtp_server = smtplib.SMTP_SSL() try: #smtp_server.set_debuglevel(True) RECONNECT = 10 # Reconnect times. for i in xrange(RECONNECT): try: smtp_server.connect(self.host, self.port) except smtplib.SMTPConnectError: if i < RECONNECT: print('Connecting to smtp server...') time.sleep(6) continue else: sys.exit('Can not connect to smtp server.') except smtplib.SMTPServerDisconnected: sys.exit('SMTP server is lost.') else: break try: smtp_server.starttls() except smtplib.SMTPException: print('SMTP server does not support the STARTTLS extension.') try: smtp_server.login(username, password) except smtplib.SMTPAuthenticationError: print('Username or Password is incorrect.') try: refusal_dict = smtp_server.sendmail(from_addr, to_addrs, msg.as_string()) except smtplib.SMTPSenderRefused: sys.exit('SMTP server does not accept the %s.' % from_addr) except smtplib.SMTPDataError: sys.exit('SMTP server replied with an unexpected error code .') else: if refusal_dict: print('*****SMTP server rejects the following recipients:*****') print(list(refusal_dict.items())) print('*******************************************************') finally: smtp_server.quit() def main(): # Send text/plain email. """ email_obj = BTEmail(host='smtp.sina.com', port=465, username=username, password=password) msg = email_obj.construct_msg(r'content\mail.txt', 'plain', 'utf-8') email_obj.make_envolope(msg, from_addr, to_addrs, subject='This is a plain text mail.') email_obj.send_mail(from_addr, to_addrs + cc_addrs, msg) """ # Send text/html email. """ email_obj = BTEmail(host='smtp.sina.com', port=465, username=username, password=password) msg = email_obj.construct_msg(r'content\mail.html', 'html', 'utf-8') email_obj.make_envolope(msg, from_addr, to_addrs, subject='This is a html mail.') email_obj.send_mail(from_addr, to_addrs + cc_addrs, msg) """ # Send email with attachments. email_obj = BTEmail(host='smtp.sina.com', port=465, username=username, password=password) msg = email_obj.construct_msg(r'content\mail.html', 'html', 'utf-8', attach_flag=True, attachpath=r'attachment') email_obj.make_envolope(msg, from_addr, to_addrs, subject='A html mail with attachments.') email_obj.send_mail(from_addr, to_addrs + cc_addrs, msg) if __name__ == '__main__': main()
相关文章推荐
- Python Tricks(九)—— 递归遍历目录下所有文件
- Python Anaconda2 (64-bit) 安装后启动jupyter-notebook默认目录更改
- Python模拟登录
- Python内置方法的时间复杂度
- Python性能优化的20条建议
- Python面向对象之类的定义与继承
- Python之路—Day2作业
- 练习014-015
- 用Python玩转数据》 南京大学 张莉 讲的不错,因为会讲知识背后的原理和思维方式
- python 识别验证码
- python 2.5源代码编绎
- Python装饰器模式学习总结
- Python装饰器模式学习总结
- windows10下sublime_python配置
- Python学习之NumPy篇
- python结构
- 用python播放音乐--pygame package
- python多线程备份MYSQL数据库并删除旧的备份。
- Python: Convert rst to html
- python scrapy cannot import name xmlrpc_client的解决方案,解决办法