Python基础(三)
2016-05-09 14:02
537 查看
本章内容:
深浅拷贝
函数(全局与局部变量)
内置函数
文件处理
三元运算
lambda 表达式
递归(斐波那契数列)
冒泡排序
一、数字和字符串
对于 数字 和 字符串 而言,赋值、浅拷贝和深拷贝无意义,因为其永远指向同一个内存地址。
3.x
三、管理上下文
为了避免打开文件后忘记关闭,可以通过管理上下文,即:
如此方式,当with代码块执行完毕时,内部会自动关闭并释放文件资源。
在Python 2.7 及以后,with又支持同时对多个文件的上下文进行管理,即:
三元运算(三目运算),是对简单的条件语句的缩写。
对于简单的函数,存在一种简便的表示方式,即:lambda表达式
递归算法是一种直接或者间接地调用自身算法的过程。在计算机编写程序中,递归算法对解决一大类问题是十分有效的,它往往使算法的描述简洁而且易于理解。
递归算法解决问题的特点:
递归就是在过程或函数里调用自身。
在使用递归策略时,必须有一个明确的递归结束条件,称为递归出口。
递归算法解题通常显得很简洁,但递归算法解题的运行效率较低。所以一般不提倡用递归算法设计程序。
在递归调用的过程当中系统为每一层的返回点、局部量等开辟了栈来存储。递归次数过多容易造成栈溢出等。所以一般不提倡用递归算法设计程序。
利用函数编写如下数列:
斐波那契数列指的是这样一个数列 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233,377,610,987,1597,2584,4181,6765,10946,17711,28657,46368...
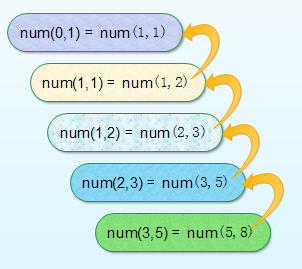
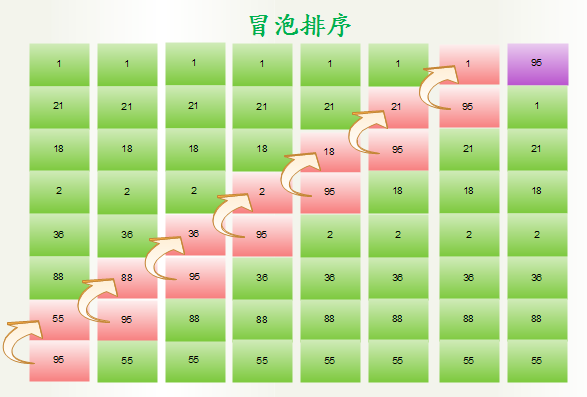
将一个不规则的数组按从小到大的顺序进行排序
深浅拷贝
函数(全局与局部变量)
内置函数
文件处理
三元运算
lambda 表达式
递归(斐波那契数列)
冒泡排序
深浅拷贝 |
对于 数字 和 字符串 而言,赋值、浅拷贝和深拷贝无意义,因为其永远指向同一个内存地址。
import copy #定义变量 数字、字符串 n1 = 123 #n1 = 'nick' print(id(n1)) #赋值 n2 = n1 print(id(n2)) #浅拷贝 n3 = copy.copy(n1) print(id(n3)) #深拷贝 n4 = copy.deepcopy(n1) print(id(n4))
class TextIOWrapper(_TextIOBase): """ Character and line based layer over a BufferedIOBase object, buffer. encoding gives the name of the encoding that the stream will be decoded or encoded with. It defaults to locale.getpreferredencoding(False). errors determines the strictness of encoding and decoding (see help(codecs.Codec) or the documentation for codecs.register) and defaults to "strict". newline controls how line endings are handled. It can be None, '', '\n', '\r', and '\r\n'. It works as follows: * On input, if newline is None, universal newlines mode is enabled. Lines in the input can end in '\n', '\r', or '\r\n', and these are translated into '\n' before being returned to the caller. If it is '', universal newline mode is enabled, but line endings are returned to the caller untranslated. If it has any of the other legal values, input lines are only terminated by the given string, and the line ending is returned to the caller untranslated. * On output, if newline is None, any '\n' characters written are translated to the system default line separator, os.linesep. If newline is '' or '\n', no translation takes place. If newline is any of the other legal values, any '\n' characters written are translated to the given string. If line_buffering is True, a call to flush is implied when a call to write contains a newline character. """ def close(self, *args, **kwargs): # real signature unknown 关闭文件 pass def fileno(self, *args, **kwargs): # real signature unknown 文件描述符 pass def flush(self, *args, **kwargs): # real signature unknown 刷新文件内部缓冲区 pass def isatty(self, *args, **kwargs): # real signature unknown 判断文件是否是同意tty设备 pass def read(self, *args, **kwargs): # real signature unknown 读取指定字节数据 pass def readable(self, *args, **kwargs): # real signature unknown 是否可读 pass def readline(self, *args, **kwargs): # real signature unknown 仅读取一行数据 pass def seek(self, *args, **kwargs): # real signature unknown 指定文件中指针位置 pass def seekable(self, *args, **kwargs): # real signature unknown 指针是否可操作 pass def tell(self, *args, **kwargs): # real signature unknown 获取指针位置 pass def truncate(self, *args, **kwargs): # real signature unknown 截断数据,仅保留指定之前数据 pass def writable(self, *args, **kwargs): # real signature unknown 是否可写 pass def write(self, *args, **kwargs): # real signature unknown 写内容 pass def __getstate__(self, *args, **kwargs): # real signature unknown pass def __init__(self, *args, **kwargs): # real signature unknown pass @staticmethod # known case of __new__ def __new__(*args, **kwargs): # real signature unknown """ Create and return a new object. See help(type) for accurate signature. """ pass def __next__(self, *args, **kwargs): # real signature unknown """ Implement next(self). """ pass def __repr__(self, *args, **kwargs): # real signature unknown """ Return repr(self). """ pass buffer = property(lambda self: object(), lambda self, v: None, lambda self: None) # default closed = property(lambda self: object(), lambda self, v: None, lambda self: None) # default encoding = property(lambda self: object(), lambda self, v: None, lambda self: None) # default errors = property(lambda self: object(), lambda self, v: None, lambda self: None) # default line_buffering = property(lambda self: object(), lambda self, v: None, lambda self: None) # default name = property(lambda self: object(), lambda self, v: None, lambda self: None) # default newlines = property(lambda self: object(), lambda self, v: None, lambda self: None) # default _CHUNK_SIZE = property(lambda self: object(), lambda self, v: None, lambda self: None) # default _finalizing = property(lambda self: object(), lambda self, v: None, lambda self: None) # default
3.x
三、管理上下文
为了避免打开文件后忘记关闭,可以通过管理上下文,即:
with open('log','r') as f: ...
如此方式,当with代码块执行完毕时,内部会自动关闭并释放文件资源。
在Python 2.7 及以后,with又支持同时对多个文件的上下文进行管理,即:
with open('log1') as obj1, open('log2') as obj2: pass
###### 从一文件挨行读取并写入二文件 ######### with open('test.log','r') as obj1 , open('test1.log','w') as obj2: for line in obj1: obj2.write(line)
三元运算 |
result = 值1 if 条件 else 值2 # 如果条件成立,那么将 “值1” 赋值给result变量,否则,将“值2”赋值给result变量
########## 三 元 运 算 ############ name = "nick" if 1==1 else "jenny" print(name)
lambda表达式 |
######## 普 通 函 数 ######## # 定义函数(普通方式) def func(arg): return arg + 1 # 执行函数 result = func(123) ######## lambda 表 达 式 ######## # 定义函数(lambda表达式) my_lambda = lambda arg : arg + 1 # 执行函数 result = my_lambda(123)
##### 列表重新判断操作排序 ##### li = [11,15,9,21,1,2,68,95] s = sorted(map(lambda x:x if x > 11 else x * 9,li)) print(s) ###################### ret = sorted(filter(lambda x:x>22, [55,11,22,33,])) print(ret)
递归 |
递归算法解决问题的特点:
递归就是在过程或函数里调用自身。
在使用递归策略时,必须有一个明确的递归结束条件,称为递归出口。
递归算法解题通常显得很简洁,但递归算法解题的运行效率较低。所以一般不提倡用递归算法设计程序。
在递归调用的过程当中系统为每一层的返回点、局部量等开辟了栈来存储。递归次数过多容易造成栈溢出等。所以一般不提倡用递归算法设计程序。
利用函数编写如下数列:
斐波那契数列指的是这样一个数列 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233,377,610,987,1597,2584,4181,6765,10946,17711,28657,46368...
def func(arg1,arg2): if arg1 == 0: print arg1, arg2 arg3 = arg1 + arg2 print arg3 func(arg2, arg3) func(0,1)
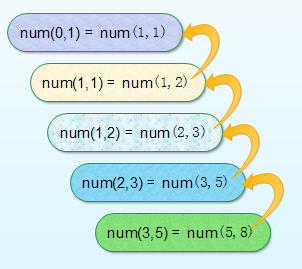
#写函数,利用递归获取斐波那契数列中的第 10 个数 #方法一 def fie(n): if n == 0 or n == 1: return n else: return (fie(n-1)+fie(n-2)) ret = fie(10) print(ret) #方法二 def num(a,b,n): if n == 10: return a print(a) c = a + b a = num(b,c,n + 1) return a s = num(0,1,1) print(s)
冒泡排序 |
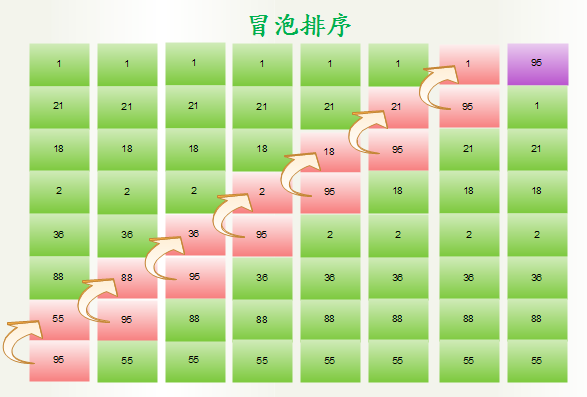
将一个不规则的数组按从小到大的顺序进行排序
data = [10,4,33,21,54,8,11,5,22,2,17,13,3,6,1,] print("before sort:",data) for j in range(1,len(data)): for i in range(len(data) - j): if data[i] > data[i + 1]: temp = data[i] data[i] = data[i + 1] data[i + 1] = temp print(data) print("after sort:",data)
相关文章推荐
- python利用paramiko连接远程服务器执行命令
- python利用paramiko连接远程服务器执行命令
- 正则表达式 python3.x (一)
- wxPython 客户端登录流程的拟定
- Skip the header of a file with Python's CSV reader
- wxPython通过py2exe编译的应用程序如何去掉黑框
- python3使用requests发闪存
- Python学习笔记:字符串(str)有关函数
- python中的闭包
- 安装过 python3.X 后,python 2.7.x 打不开 idle
- ImportError: no module named win32api
- python-百度语音识别与google语音识别测试
- 扯扯python调用rpc实现分布式系统
- Python 模版引擎
- Python正则表达式
- caffe cpu版 Anaconda3 python 接口安装
- 输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。
- python之PyMongo使用总结
- 百度网盘爬虫(如何爬取百度网盘)
- 将Python自带版本(2.6.6)升级到2.7.9