【C++】Geekband-专题四:traints的使用
2016-04-23 19:42
465 查看
1. 基本定义
2. Fixed Traits
2.1 传统方法
2.2 使用Traits方法
2.3 总结
3. Value Traits
4. Parameterized Traits
Reference
In some cases the extra parameters are entirely determined by a few main paameters. 因此需要通过几个核心参数型别的定义,来设计不同的模板,满足不同需求。
Fixed主要指,一旦T在模板中被定义,则无法再调用中改变。
[/code]
调用该模板
[/code]
存在问题,当调用的是
[/code]
调用方法不变。
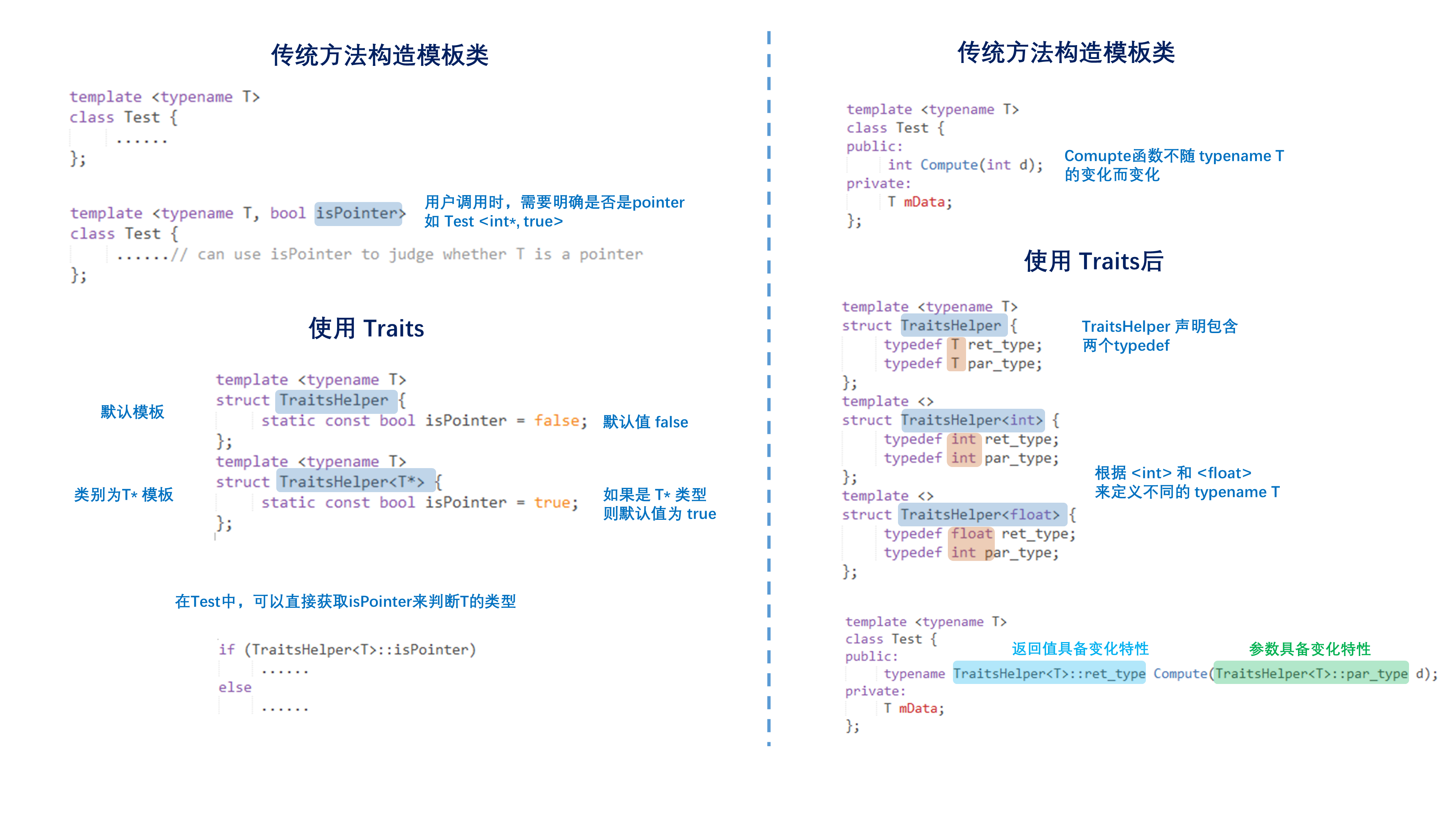
模板的书写,引入
[/code]
调用方法不变
总结
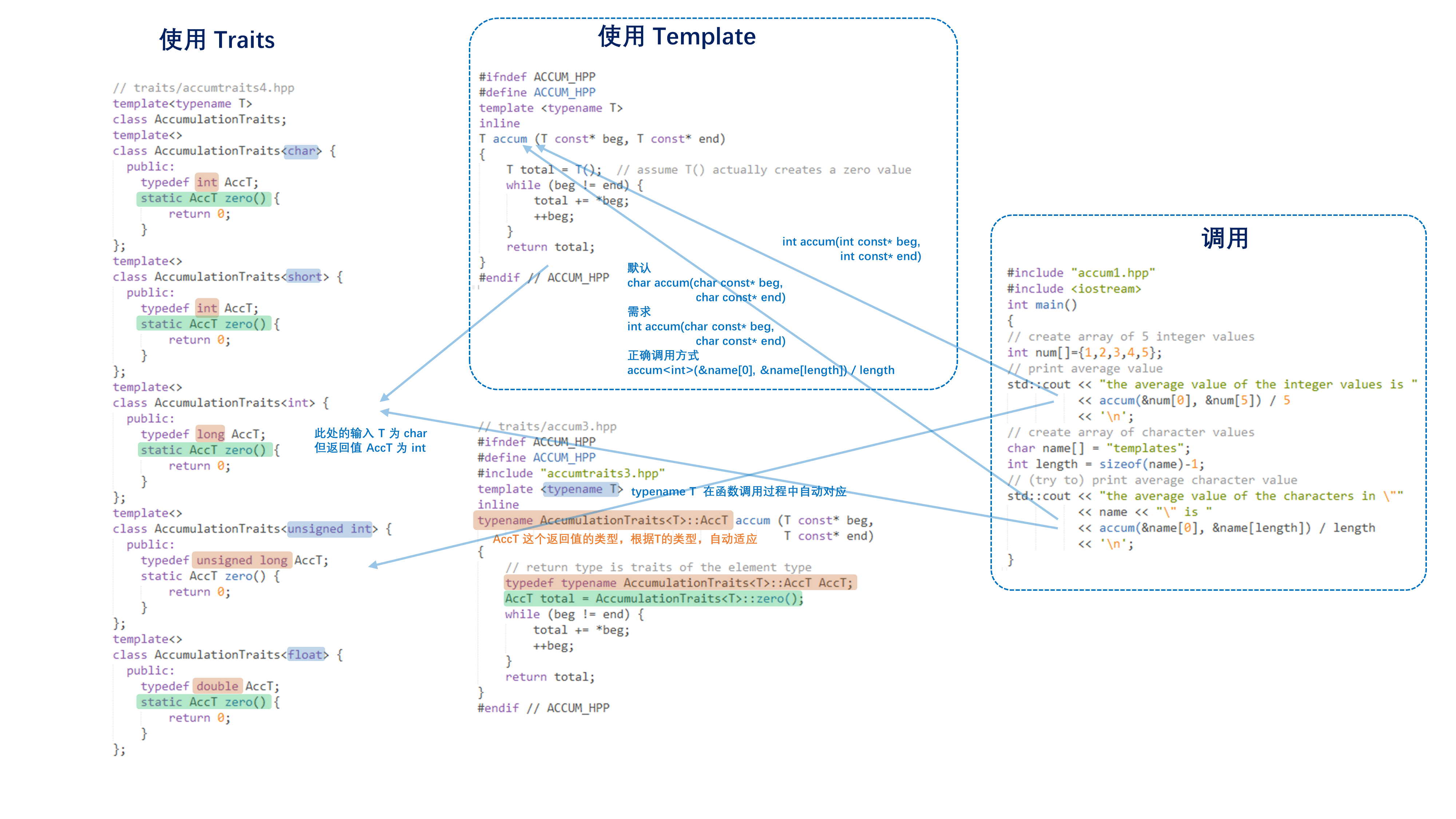
有两种方法,一种是基于
[/code]
另一种是将
[/code]
总结
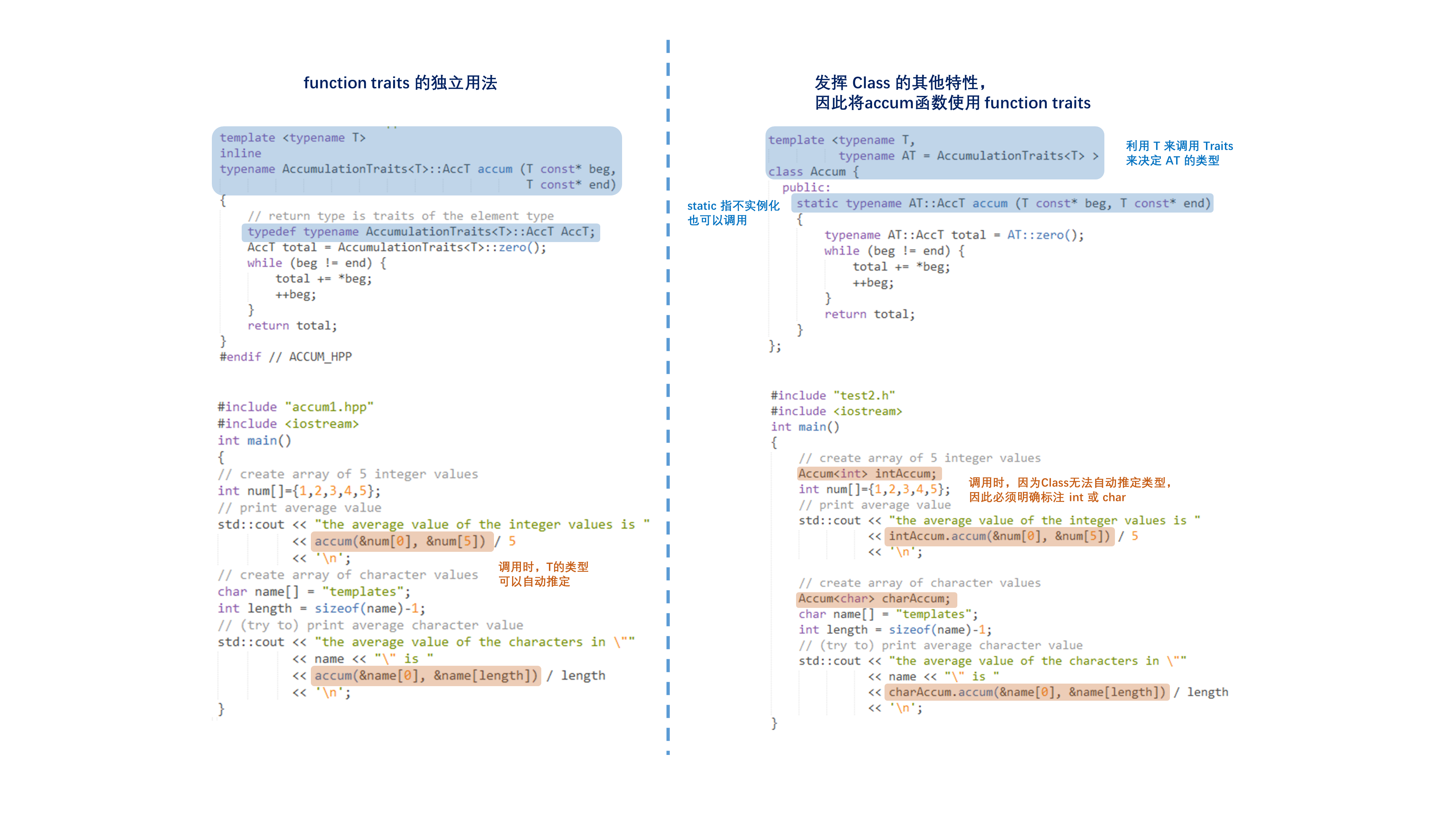
http://blog.csdn.net/my_business/article/details/8098417
Traits的一个举例
http://www.cnblogs.com/hellogiser/p/cplusplus-traits.html
C++ Templates - The Complete Guide - Chp. 15
2. Fixed Traits
2.1 传统方法
2.2 使用Traits方法
2.3 总结
3. Value Traits
4. Parameterized Traits
Reference
1. 基本定义
Think of a trait as a small object whose main purpose is to carry information used by another object or algorithm to determine “policy” or “implementation details”.In some cases the extra parameters are entirely determined by a few main paameters. 因此需要通过几个核心参数型别的定义,来设计不同的模板,满足不同需求。
2. Fixed Traits
主要是构造适应各种类型的函数Fixed主要指,一旦T在模板中被定义,则无法再调用中改变。
2.1 传统方法
传统方法定义的模板#ifndef ACCUM_HPP
#define ACCUM_HPP
template <typename T>
inline
T accum (T const* beg, T const* end)
{
T total = T(); // assume T() actually creates a zero value
while (beg != end) {
total += *beg;
++beg;
}
return total;
}
#endif // ACCUM_HPP
[/code]
调用该模板
#include "accum1.hpp"
#include <iostream>
int main()
{
// create array of 5 integer values
int num[]={1,2,3,4,5};
// print average value
std::cout << "the average value of the integer values is "
<< accum(&num[0], &num[5]) / 5
<< '\n';
// create array of character values
char name[] = "templates";
int length = sizeof(name)-1;
// (try to) print average character value
std::cout << "the average value of the characters in \""
<< name << "\" is "
<< accum(&name[0], &name[length]) / length
<< '\n';
}
[/code]
存在问题,当调用的是
char时,模板自动将返回函数也定义为
char,而不是期望的
int。
2.2 使用Traits方法
模板定义template<typename T>
class AccumulationTraits;
template<>
class AccumulationTraits<char> {
public:
typedef int AccT;
};
template<>
class AccumulationTraits<short> {
public:
typedef int AccT;
};
template<>
class AccumulationTraits<int> {
public:
typedef long AccT;
};
template<>
class AccumulationTraits<unsigned int> {
public:
typedef unsigned long AccT;
};
template<>
class AccumulationTraits<float> {
public:
typedef double AccT;
};
template <typename T>
inline
typename AccumulationTraits<T>::AccT accum (T const* beg,
T const* end)
{
// return type is traits of the element type
typedef typename AccumulationTraits<T>::AccT AccT;
AccT total = AccT(); // assume T() actually creates a zero value
while (beg != end) {
total += *beg;
++beg;
}
return total;
}
[/code]
调用方法不变。
2.3 总结
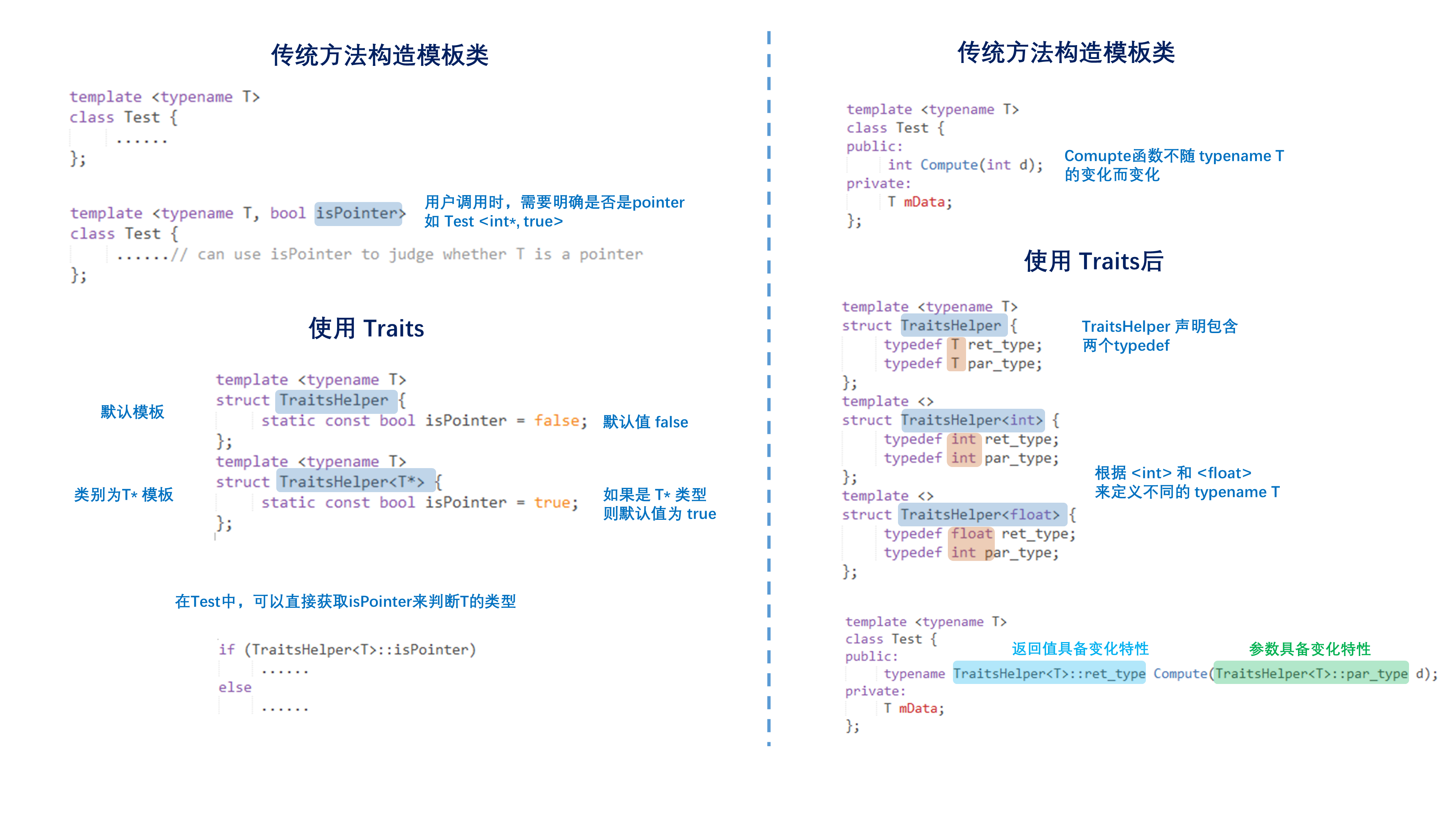
3. Value Traits
对模板中特定数值,特别是初始话时候的操作方法模板的书写,引入
zero()
template<typename T>
class AccumulationTraits;
template<>
class AccumulationTraits<char> {
public:
typedef int AccT;
static AccT zero() {
return 0;
}
};
template<>
class AccumulationTraits<short> {
public:
typedef int AccT;
static AccT zero() {
return 0;
}
};
template<>
class AccumulationTraits<int> {
public:
typedef long AccT;
static AccT zero() {
return 0;
}
};
template<>
class AccumulationTraits<unsigned int> {
public:
typedef unsigned long AccT;
static AccT zero() {
return 0;
}
};
template<>
class AccumulationTraits<float> {
public:
typedef double AccT;
static AccT zero() {
return 0;
}
};
template <typename T>
inline
typename AccumulationTraits<T>::AccT accum (T const* beg,
T const* end)
{
// return type is traits of the element type
typedef typename AccumulationTraits<T>::AccT AccT;
AccT total = AccumulationTraits<T>::zero();
while (beg != end) {
total += *beg;
++beg;
}
return total;
}
[/code]
调用方法不变
总结
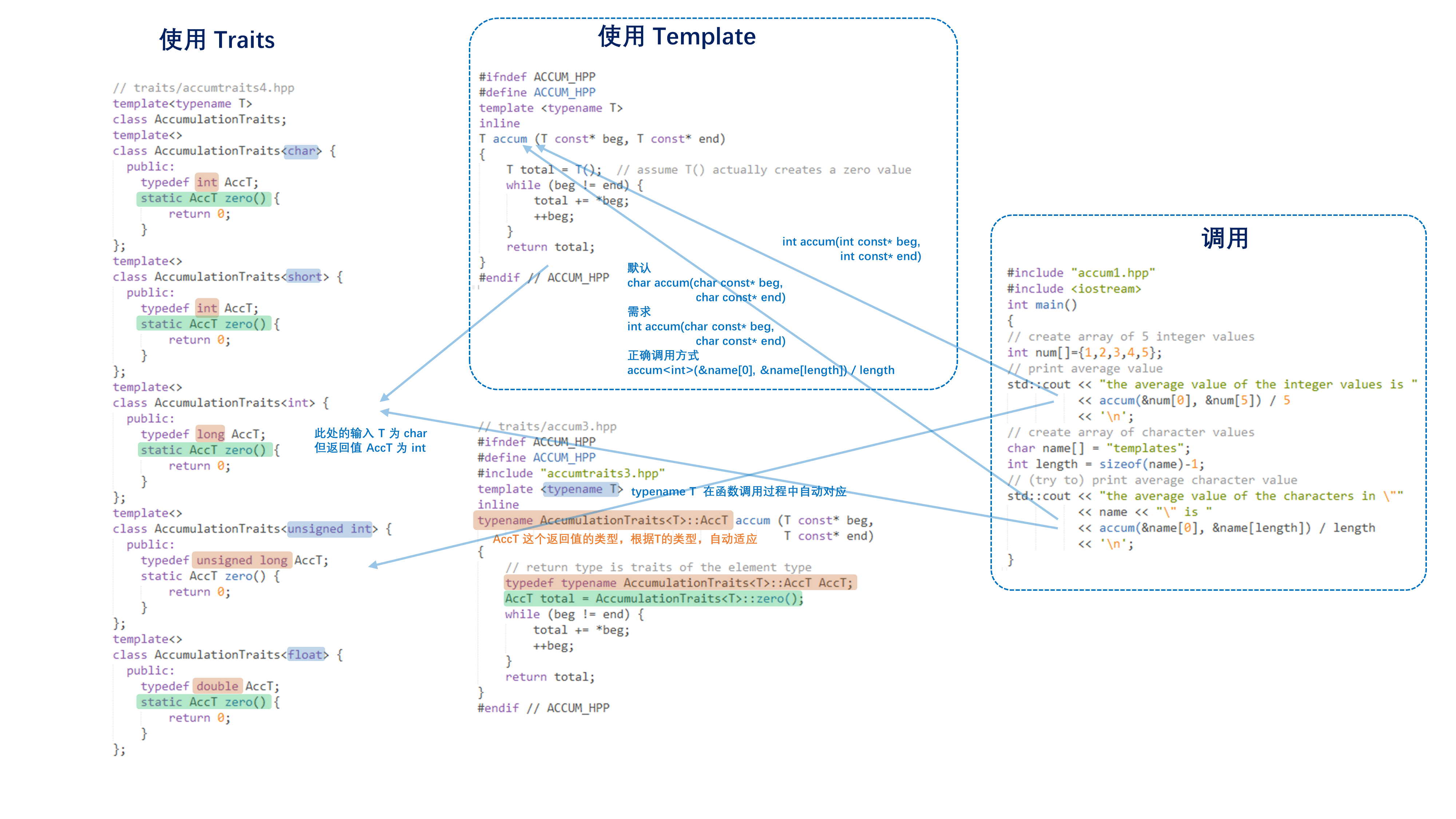
4. Parameterized Traits
为了增加灵活性,引入另一个参数(AT),使其来决定参数(T)的类型。有两种方法,一种是基于
function traits的方法
template <typename T>
inline
typename AccumulationTraits<T>::AccT accum (T const* beg,
T const* end)
{
return Accum<T>::accum(beg, end);
}
template <typename Traits, typename T>
inline
typename Traits::AccT accum (T const* beg, T const* end)
{
return Accum<T, Traits>::accum(beg, end);
}
[/code]
另一种是将
function traits方法引入Class
template <typename T,
typename AT = AccumulationTraits<T> >
class Accum {
public:
static typename AT::AccT accum (T const* beg, T const* end)
{
typename AT::AccT total = AT::zero();
while (beg != end) {
total += *beg;
++beg;
}
return total;
}
};
[/code]
总结
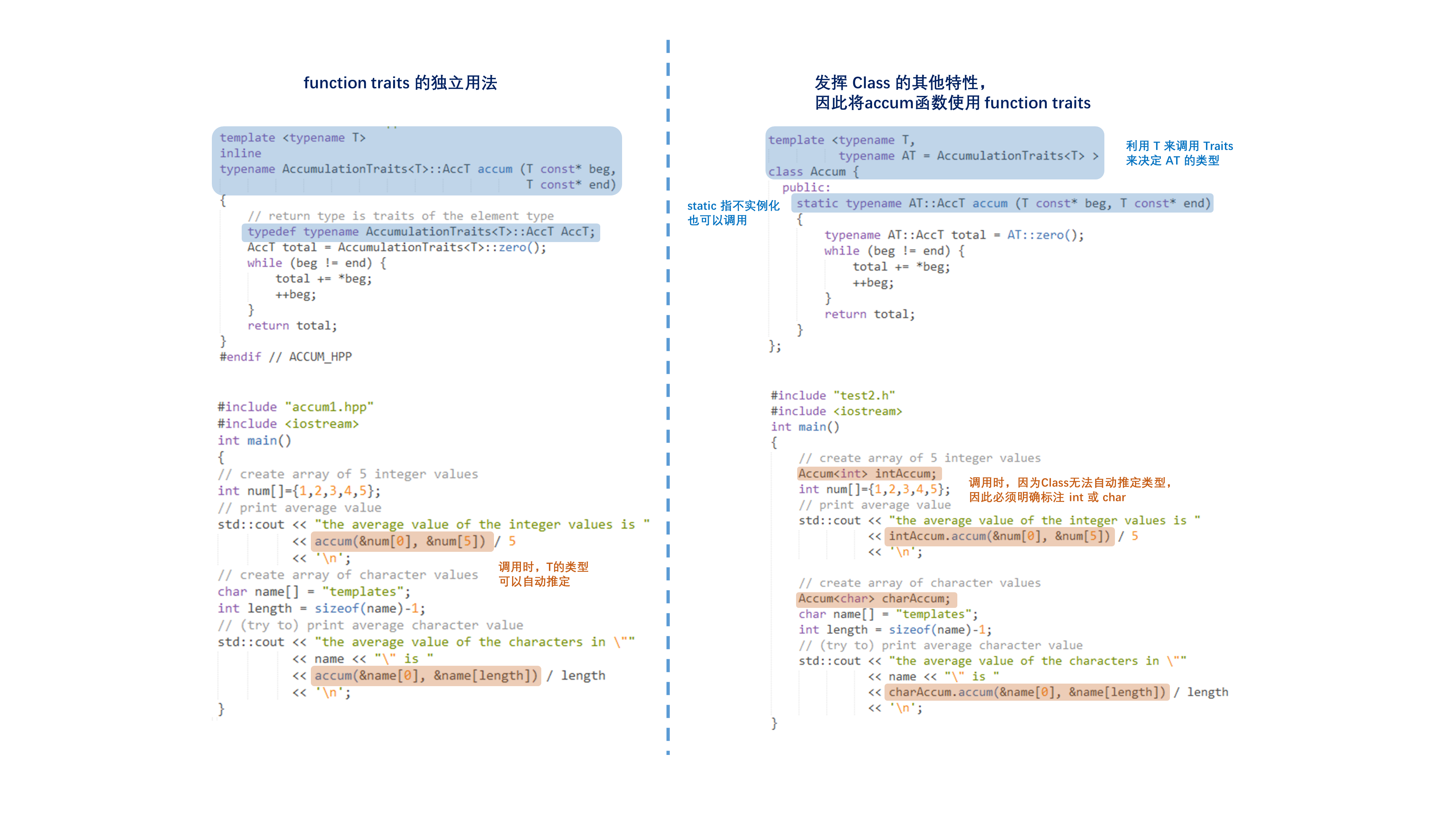
Reference
项目中一次活用C++模板(traits)的经历http://blog.csdn.net/my_business/article/details/8098417
Traits的一个举例
http://www.cnblogs.com/hellogiser/p/cplusplus-traits.html
C++ Templates - The Complete Guide - Chp. 15
相关文章推荐
- 用C语言实现简单的计算器(加、减、乘、除)
- 用C语言打印出杨辉三角
- C语言小游戏设计报告
- C++中的explicit关键字(转)
- 作业:C++作业4
- 用C语言做一个通讯录
- base64加密解密c++的简单实现
- 编程学习笔记之c++相关::vector学习心得
- C语言中,为什么字符串可以赋值给字符指针变量
- 字符串反转引发的char str[]="abc"和char *str="abc"思考
- 有符号十六进制转十进制 c++
- C++虚继承解说
- c++第4次作业
- 奇特的可变参数列表实现print函数
- 基于GDAL的栅格数据/遥感影像IO (非分块)
- C语言编程常用数值计算的高性能实现
- 1 C语言 gcc 介绍 C 语言编译 main接受参数
- c语言auto、static、extern、register、volatile存储的理解
- c++添加定时器
- c++如何生成随机数