PHP SOAP实现WebService
2016-04-21 15:03
274 查看
第一种:WSDL模式
Client.php客户端请求文件
http://localhost/soap/Wsdl/wsdl.php?wsdl 修改为自己目录的对应路径 ?wsdl 必须带着否则会报错
SoapDiscovery.class.php 核心类
wsdl.php 处理文件
yourCode.php 自定义的代码,必须为一个类,类名在wsdl.php 中使用,注意对应修改。
说明:
client.php 客户端请求文件
http://localhost/soap/Wsdl/wsdl.php?wsdl 修改为自己目录的对应路径 ?wsdl 必须带着否则会报错
Wsdl/
SoapDiscovery.class.php 核心类
wsdl.php 处理文件
yourCode.php 自定义的代码,必须为一个类,类名在wsdl.php 中使用,注意对应修改。
在项目中和其他公司java程序员做接口,他们那边只会Web Services来做接口,之后整理该扩展,分享一下。
访问 http://localhost/soap/Wsdl/wsdl.php?wsdl 的部分截图。
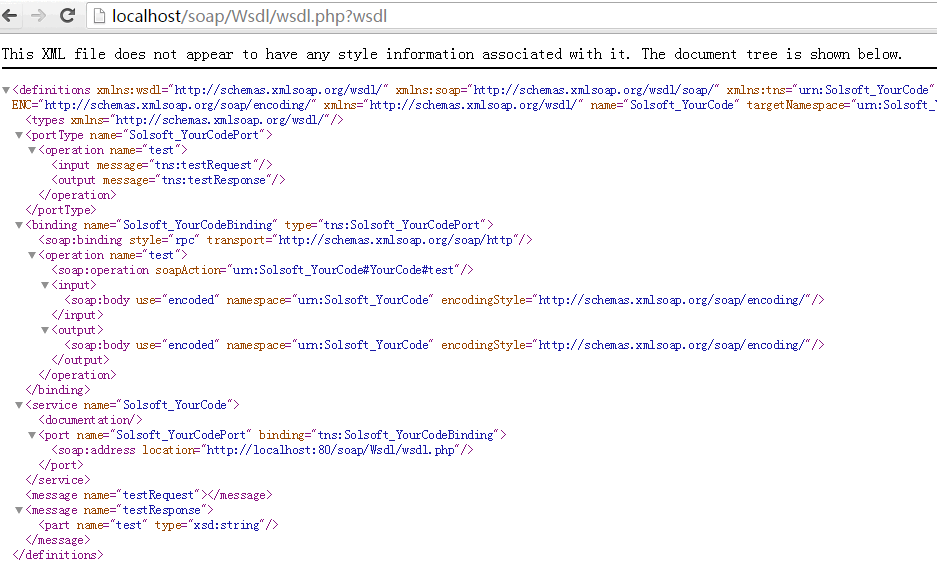
文件结构:
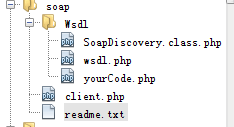
第二种:NO-WSDL模式
php soap 使用实例
文件列表
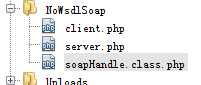
client.php
server.php
soapHandle.class.php
参考
分享php中四种webservice实现的简单架构方法及实例
Client.php客户端请求文件
http://localhost/soap/Wsdl/wsdl.php?wsdl 修改为自己目录的对应路径 ?wsdl 必须带着否则会报错
<?php $client = new SoapClient("http://localhost/soap/Wsdl/wsdl.php?wsdl",array('soap_version'=> SOAP_1_2)); try{ $result = $client->test(); echo $result; }catch (SoapFault $f){ echo "Error Message: {$f->getMessage()}"; } ?>
SoapDiscovery.class.php 核心类
<?php /** * @package SoapDiscovery 核心 * @author 囚鸟先生 * @copyright Copyright (c) 2013 * @access public **/ class SoapDiscovery { private $class_name = ''; private $service_name = ''; /** * SoapDiscovery::__construct() SoapDiscovery class Constructor. * * @param string $class_name * @param string $service_name **/ public function __construct($class_name = '', $service_name = '') { $this->class_name = $class_name; $this->service_name = $service_name; } /** * 获取WSDL * * @return string **/ public function getWSDL() { if (empty($this->service_name)) { throw new Exception('No service name.'); } $headerWSDL = "<?xml version=\"1.0\" ?>\n"; $headerWSDL.= "<definitions name=\"$this->service_name\" targetNamespace=\"urn:$this->service_name\" xmlns:wsdl=\"http://schemas.xmlsoap.org/wsdl/\" xmlns:soap=\"http://schemas.xmlsoap.org/wsdl/soap/\" xmlns:tns=\"urn:$this->service_name\" xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" xmlns:SOAP-ENC=\"http://schemas.xmlsoap.org/soap/encoding/\" xmlns=\"http://schemas.xmlsoap.org/wsdl/\">\n"; $headerWSDL.= "<types xmlns=\"http://schemas.xmlsoap.org/wsdl/\" />\n"; if (empty($this->class_name)) { throw new Exception('No class name.'); } $class = new ReflectionClass($this->class_name); if (!$class->isInstantiable()) { throw new Exception('Class is not instantiable.'); } $methods = $class->getMethods(); $portTypeWSDL = '<portType name="'.$this->service_name.'Port">'; $bindingWSDL = '<binding name="'.$this->service_name.'Binding" type="tns:'.$this->service_name."Port\">\n<soap:binding style=\"rpc\" transport=\"http://schemas.xmlsoap.org/soap/http\" />\n"; $serviceWSDL = '<service name="'.$this->service_name."\">\n<documentation />\n<port name=\"".$this->service_name.'Port" binding="tns:'.$this->service_name."Binding\"><soap:address location=\"http://".$_SERVER['SERVER_NAME'].':'.$_SERVER['SERVER_PORT'].$_SERVER['PHP_SELF']."\" />\n</port>\n</service>\n"; $messageWSDL = ''; foreach ($methods as $method) { if ($method->isPublic() && !$method->isConstructor()) { $portTypeWSDL.= '<operation name="'.$method->getName()."\">\n".'<input message="tns:'.$method->getName()."Request\" />\n<output message=\"tns:".$method->getName()."Response\" />\n</operation>\n"; $bindingWSDL.= '<operation name="'.$method->getName()."\">\n".'<soap:operation soapAction="urn:'.$this->service_name.'#'.$this->class_name.'#'.$method->getName()."\" />\n<input><soap:body use=\"encoded\" namespace=\"urn:$this->service_name\" encodingStyle=\"http://schemas.xmlsoap.org/soap/encoding/\" />\n</input>\n<output>\n<soap:body use=\"encoded\" namespace=\"urn:$this->service_name\" encodingStyle=\"http://schemas.xmlsoap.org/soap/encoding/\" />\n</output>\n</operation>\n"; $messageWSDL.= '<message name="'.$method->getName()."Request\">\n"; $parameters = $method->getParameters(); foreach ($parameters as $parameter) { $messageWSDL.= '<part name="'.$parameter->getName()."\" type=\"xsd:string\" />\n"; } $messageWSDL.= "</message>\n"; $messageWSDL.= '<message name="'.$method->getName()."Response\">\n"; $messageWSDL.= '<part name="'.$method->getName()."\" type=\"xsd:string\" />\n"; $messageWSDL.= "</message>\n"; } } $portTypeWSDL.= "</portType>\n"; $bindingWSDL.= "</binding>\n"; //返回一个格式化字符串 return sprintf('%s%s%s%s%s%s', $headerWSDL, $portTypeWSDL, $bindingWSDL, $serviceWSDL, $messageWSDL, '</definitions>'); } /** * 返回 WSDL * * @return string **/ public function returnWSDL() { return "<?xml version=\"1.0\" ?>\n<disco:discovery xmlns:disco=\"http://schemas.xmlsoap.org/disco/\" xmlns:scl=\"http://schemas.xmlsoap.org/disco/scl/\">\n<scl:contractRef ref=\"http://".$_SERVER['SERVER_NAME'].':'.$_SERVER['SERVER_PORT'].$_SERVER['PHP_SELF']."?wsdl\" />\n</disco:discovery>"; } } ?>
wsdl.php 处理文件
<?php require_once './yourCode.php'; ini_set('soap.wsdl_cache_enabled','0'); //关闭WSDL缓存 //打开WSDL if (isset($_SERVER['REQUEST_METHOD']) && $_SERVER['REQUEST_METHOD']=='POST') { $url='http://'.$_SERVER['SERVER_NAME'].':'.$_SERVER['SERVER_PORT'].$_SERVER['PHP_SELF'].'?wsdl'; $servidorSoap = new SoapServer($url); $servidorSoap->setClass('YourCode'); $servidorSoap->handle(); }else{ require_once './SoapDiscovery.class.php'; // 创建WSDL $disco = new SoapDiscovery('YourCode','Solsoft_YourCode'); header("Content-type: text/xml"); if (isset($_SERVER['QUERY_STRING']) && strcasecmp($_SERVER['QUERY_STRING'],'wsdl')==0) { echo $disco->getWSDL(); }else { echo $disco->returnWSDL(); } } ?>
yourCode.php 自定义的代码,必须为一个类,类名在wsdl.php 中使用,注意对应修改。
<?php /** * @package YourCode * @author 囚鸟先生 * @access public **/ class YourCode { /** * YourCode::test(); Returns server timestamp. * * @return string **/ public function test() { return time(); } }
说明:
client.php 客户端请求文件
http://localhost/soap/Wsdl/wsdl.php?wsdl 修改为自己目录的对应路径 ?wsdl 必须带着否则会报错
Wsdl/
SoapDiscovery.class.php 核心类
wsdl.php 处理文件
yourCode.php 自定义的代码,必须为一个类,类名在wsdl.php 中使用,注意对应修改。
在项目中和其他公司java程序员做接口,他们那边只会Web Services来做接口,之后整理该扩展,分享一下。
访问 http://localhost/soap/Wsdl/wsdl.php?wsdl 的部分截图。
文件结构:
第二种:NO-WSDL模式
php soap 使用实例
文件列表
client.php
<?php try { $client = new SOAPClient(null, array( 'location' => 'http://localhost/NoWsdlSoap/server.php', // 设置server路径 'uri' => 'http://localhost/NoWsdlSoap/server.php', 'login' => 'fuck', // HTTP auth login 'password' => '123456' // HTTP auth password )); echo $client->strtolink() . '<br>'; // 直接调用server方法 echo $client->__soapCall('test', array()); // 间接调用server方法 } catch (SOAPFault $e) { print $e->getMessage(); } ?>
server.php
<?php // 服务器验证 if ($_SERVER['PHP_AUTH_USER']!='fuck' || $_SERVER['PHP_AUTH_PW']!='123456') { header('WWW-Authenticate: Basic realm="MyFramework Realm"'); header('HTTP/1.0 401 Unauthorized'); echo "You must enter a valid login ID and password to access this resource.\n"; exit; } require("soapHandle.class.php"); // 处理请求的class try{ $server = new SOAPServer(null, array('uri'=>'http://localhost/NoWsdlSoap/server.php')); $server->setClass('soapHandle'); //设置处理的class $server->handle(); }catch(SOAPFault $f){ print $f->faultString; // 打印出错信息 } ?>
soapHandle.class.php
<?php class soapHandle{ public function strtolink($url=''){ return sprintf('<a href="%s">%s</a>', $url, $url); } public function test(){ return 'fuck you'; } } ?>
参考
分享php中四种webservice实现的简单架构方法及实例
相关文章推荐
- ThinkPHP多表操作
- 如何在FTP服务器上传和下载文件
- 关于php中json_encode中文乱码问题
- php分享(三十六)mysql中关联表更新
- PHP间隔一段时间执行
- PHP 自定义方法实现数组合并
- ThinkPHP使用SQL函数进行查询
- laravel各种路径的获取方法
- LTP工具说明
- ios下使用rsa算法与php进行加解密通讯
- phpcms开发使用
- TP3.2整合kindeditor
- TP3.2整合uplodify文件上传
- php+coreseek/sphinx之中文全文搜索平台搭建
- yii2 GridView常见操作
- php慢日志记录和错误日志
- php代码20个实用技巧 ------ 转发自菜鸟教程
- phpstorm xdebug配置
- QPS和TPS解释
- Sublime Text sublimelinter 提示php