C++实现栈
2016-04-08 00:55
375 查看
1.栈
定义:栈是只允许末端进行插入和删除的线性表,具有先进后出或先进后出的特性
栈用数组实现较好,因为它只在尾上进行操作,进行增删比较方便。
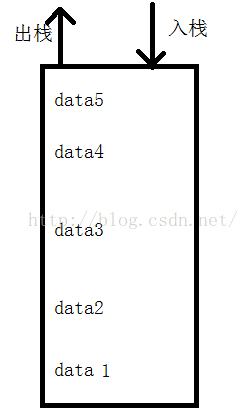
1.栈
测试用例:
如有不正确的地方,希望能够指出,一起学习,谢谢了。
定义:栈是只允许末端进行插入和删除的线性表,具有先进后出或先进后出的特性
栈用数组实现较好,因为它只在尾上进行操作,进行增删比较方便。
1.栈
<span style="font-family:Microsoft YaHei;font-size:18px;">#pragma once //栈具有后进先出的原则, template<class T> class Stack { public: //构造函数 Stack() :_a(NULL) , _top(0) , _capcity(0) {} //析构函数 ~Stack() { Clear(); } //push void PushBack(const T& x) { //1.先检查容量 2.向数据中插入新数据 3.++_top CheckCapcity(); _a[_top++] = x; } //pop void PopBack() { if (_top == 0) { cout << "NULL" << endl;; return; //记得加这句,不然停不下来,越界访问,有bug } else --_top; } //size int Size() { return _top; } //empty bool Empty() { return _top == 0; } //top T& Top() { return _a[_top-1]; } void Print() { for (int i = 0; i < _top; i++) { cout << _a[i] << " "; } cout << endl; } protected: void CheckCapcity() { if (_top == _capcity) {//扩充的是_capcity,所以只改变_capcity,其他的不用动 _capcity = _capcity * 2 + 3; T* tmp = new T[_capcity]; //重新开辟空间 for (int i = 0; i < _top; i++) { tmp[i] = _a[i]; //将数据都拷贝到新空间中 } delete[] _a; //释放原空间, _a = tmp; //然后指针重新指向新空间 } } void Clear() { if (_a!=NULL) { delete[] _a; _top = 0; _capcity = 0; } } protected: T* _a; //栈底层封装数组,利用数组实现栈,这样做就可以限制只在尾上做处理 size_t _top;//指向最上面的元素,相当关于以前数组的的size size_t _capcity;//当前栈的总容量 };</span>
测试用例:
<span style="font-family:Microsoft YaHei;font-size:18px;">#define _CRT_SECURE_NO_WARNINGS 1 #include<iostream> using namespace std; #include"Stack.h" //Test Push void Test1() { Stack<int> s1; s1.Push(1); s1.Push(2); s1.Push(3); s1.Push(4); s1.Push(5); while (!s1.Empty()) { cout << s1.Top() << endl; s1.Pop(); } } //Test Pop void Test2() { Stack<int> s1; s1.Push(1); s1.Push(2); s1.Push(3); s1.Push(4); s1.Push(5); s1.Pop(); s1.Pop(); s1.Pop(); s1.Pop(); s1.Pop(); s1.Pop(); s1.Pop(); while (!s1.Empty()) { cout << s1.Top() << endl; s1.Pop(); } } //Test size() void Test3() { Stack<int> s1; s1.Push(1); cout << s1.size() << endl; s1.Push(2); cout << s1.size() << endl; s1.Push(3); cout << s1.size() << endl; s1.Push(4); cout << s1.size() << endl; s1.Push(5); cout << s1.size() << endl; } //test empty void Test4() { Stack<int> s1; s1.Push(1); s1.Push(2); s1.Pop(); s1.Pop(); cout << s1.Empty() << endl; } //test void Test5() { Stack<int> s1; s1.Push(1); cout<<s1.Top()<<endl; s1.Push(2); cout << s1.Top() << endl; s1.Push(3); cout << s1.Top() << endl; s1.Push(4); cout << s1.Top() << endl; s1.Push(5); cout << s1.Top() << endl; } int main() { //Test1(); //Test2(); //Test3(); //Test4(); Test5(); system("pause"); return 0; } </span>
如有不正确的地方,希望能够指出,一起学习,谢谢了。
相关文章推荐
- C语言 详解多级指针与指针类型的关系
- 好久没写过博客,写一道今天的笔试题
- 2016第七届蓝桥杯C/C++ B组省赛题解 A题
- 关于C语言的问卷调查!!!!!!!!!!
- C语言问卷调查
- 《高质量C++/C编程指南》读书笔记二
- MySQL Connector/C++ Mac Xcode
- 关于Bjarne Stroustrups教授与C++
- 关于c++的命名空间
- CFILE文件类CFile::Read
- 一个关于C语言单链表的简单应用程序
- C++中常量指针,指针常量(const 和*)的使用方法和理解方法
- C++混合编程之idlcpp教程Python篇(5)
- 结构体练手
- C++实验3-本月有几天?
- C++中的操作符重载
- Splay Tree的C++实现
- 快速排序和冒泡排序的时间复杂度分析(C++算法实现对比)
- C++第3次作业
- 关于C语言的问卷调查