JavaPersistenceWithHibernate第二版笔记-第六章-Mapping inheritance-002Table per concrete class with implicit polymorphism(@MappedSuperclass、@AttributeOverride)
2016-04-07 20:49
337 查看
一、结构
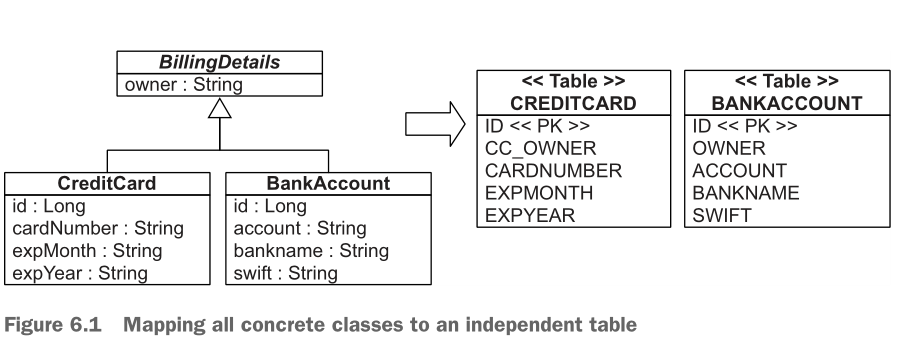
二、代码
1.
2.
3.
三、存在的问题
1.it doesn’t support polymorphic associations very well。You can’t have another entity mapped with a foreign key “referencing BILLINGDETAILS ”—there is no such table. This would be problematic in the domain model, because BillingDetails is associated with User ; both the CREDITCARD and BANKACCOUNT tables would need a foreign key reference to the USERS table. None of these issues can be easily resolved, so you should consider an alternative mapping strategy.
2.查父类时要查每个表。Hibernate must execute a query against the superclass as several SQL SELECT s, one for each concrete subclass. The JPA query select bd from BillingDetails bd requires two SQL statements:
3.several different columns, of different tables, share exactly the same semantics.
结论:We recommend this approach (only) for the top level of your class hierarchy,where polymorphism isn’t usually required, and when modification of the superclass in the future is unlikely.
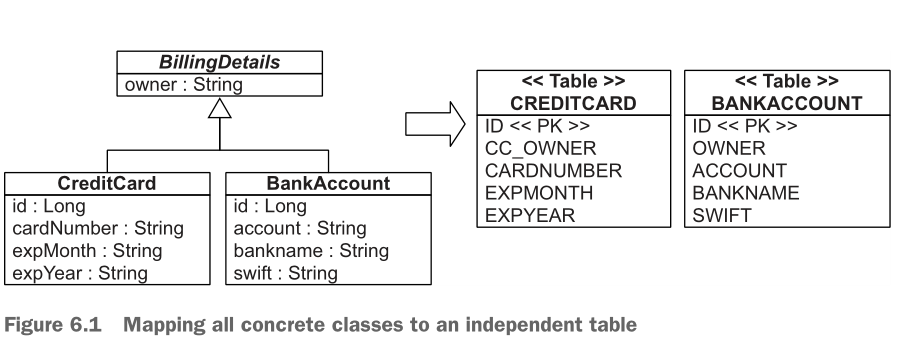
二、代码
1.
package org.jpwh.model.inheritance.mappedsuperclass; import javax.persistence.MappedSuperclass; import javax.validation.constraints.NotNull; @MappedSuperclass public abstract class BillingDetails { @NotNull protected String owner; // ... protected BillingDetails() { } protected BillingDetails(String owner) { this.owner = owner; } public String getOwner() { return owner; } public void setOwner(String owner) { this.owner = owner; } }
2.
package org.jpwh.model.inheritance.mappedsuperclass; import org.jpwh.model.Constants; import javax.persistence.AttributeOverride; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.validation.constraints.NotNull; @Entity @AttributeOverride( name = "owner", column = @Column(name = "CC_OWNER", nullable = false)) public class CreditCard extends BillingDetails { @Id @GeneratedValue(generator = Constants.ID_GENERATOR) protected Long id; @NotNull protected String cardNumber; @NotNull protected String expMonth; @NotNull protected String expYear; // ... public CreditCard() { super(); } public CreditCard(String owner, String cardNumber, String expMonth, String expYear) { super(owner); this.cardNumber = cardNumber; this.expMonth = expMonth; this.expYear = expYear; } public Long getId() { return id; } public String getCardNumber() { return cardNumber; } public void setCardNumber(String cardNumber) { this.cardNumber = cardNumber; } public String getExpMonth() { return expMonth; } public void setExpMonth(String expMonth) { this.expMonth = expMonth; } public String getExpYear() { return expYear; } public void setExpYear(String expYear) { this.expYear = expYear; } }
3.
package org.jpwh.model.inheritance.mappedsuperclass; import org.jpwh.model.Constants; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.validation.constraints.NotNull; @Entity public class BankAccount extends BillingDetails { @Id @GeneratedValue(generator = Constants.ID_GENERATOR) protected Long id; @NotNull protected String account; @NotNull protected String bankname; @NotNull protected String swift; public BankAccount() { super(); } public BankAccount(String owner, String account, String bankname, String swift) { super(owner); this.account = account; this.bankname = bankname; this.swift = swift; } public Long getId() { return id; } public String getAccount() { return account; } public void setAccount(String account) { this.account = account; } public String getBankname() { return bankname; } public void setBankname(String bankname) { this.bankname = bankname; } public String getSwift() { return swift; } public void setSwift(String swift) { this.swift = swift; } }
三、存在的问题
1.it doesn’t support polymorphic associations very well。You can’t have another entity mapped with a foreign key “referencing BILLINGDETAILS ”—there is no such table. This would be problematic in the domain model, because BillingDetails is associated with User ; both the CREDITCARD and BANKACCOUNT tables would need a foreign key reference to the USERS table. None of these issues can be easily resolved, so you should consider an alternative mapping strategy.
2.查父类时要查每个表。Hibernate must execute a query against the superclass as several SQL SELECT s, one for each concrete subclass. The JPA query select bd from BillingDetails bd requires two SQL statements:
select ID, OWNER, ACCOUNT, BANKNAME, SWIFT from BANKACCOUNT select ID, CC_OWNER, CARDNUMBER, EXPMONTH, EXPYEAR from CREDITCARD
3.several different columns, of different tables, share exactly the same semantics.
结论:We recommend this approach (only) for the top level of your class hierarchy,where polymorphism isn’t usually required, and when modification of the superclass in the future is unlikely.
相关文章推荐
- android中的相对布局RelativeLayout
- Swift UITextField/UITextView(placeholder的制作)
- 单例在Swift中的正确实现方式
- [IOS]动画效果
- Android:应用宝省流量更新
- Android 屏蔽recent task 按钮
- JavaPersistenceWithHibernate第二版笔记-第六章-Mapping inheritance-001Hibernate映射继承的方法
- Android APP开发需要的内容和优秀的开源网站
- object 类 “equals” 方法与 “ == " 之间的差别
- Unity3d 残影效果(狂拽炫酷叼炸天)
- 关于移动端开发宽度高度,字体以及rem宽度使用的总结
- iOS开发中一些常用的check事项2
- AndroidStudio插件GsonFormat
- ios开发中的一些注册流程的Check
- IOS开发基础知识--碎片36
- Android——适配器其他组件(AutoCompleteTextView:自动完成文本编辑框;Spinner:下拉列表)
- 个人偏好设置,归档,解档
- Android Fragment应用实战,使用碎片向ActivityGroup说再见
- Android客户端性能优化(魅族资深工程师毫无保留奉献)
- [iOS]关于横屏后状态栏不显示的问题