Android 通用标题栏简单封装实现
2016-03-20 12:00
507 查看
1。titlebar
2。xml
3。aty
4。xml 4aty
结果;
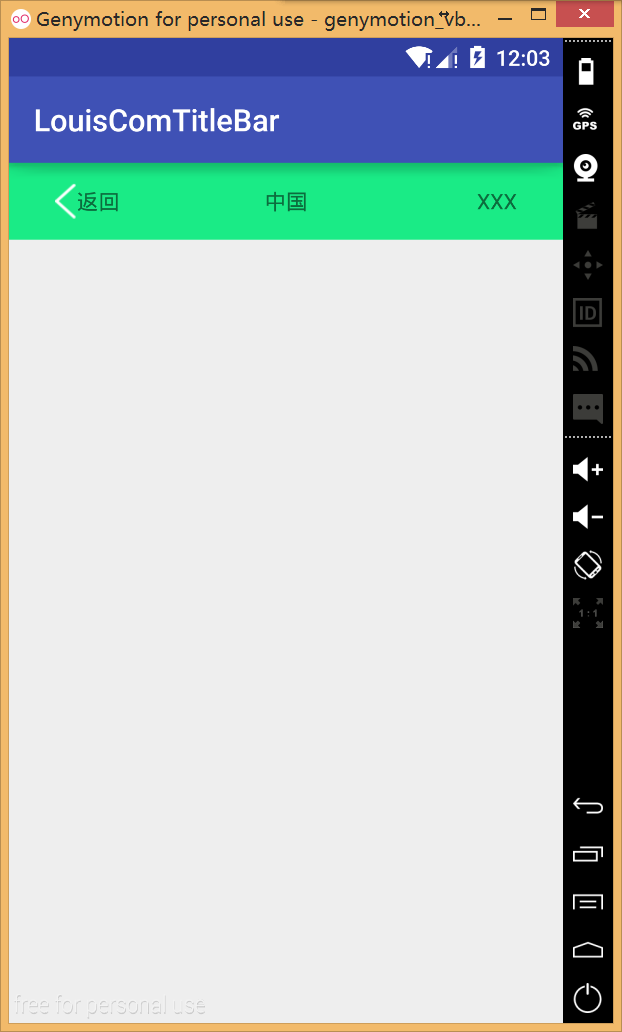
demo下载:http://download.csdn.net/detail/richiezhu/9467089
package com.louis.louiscomtitlebar; import android.content.Context; import android.content.res.ColorStateList; import android.graphics.drawable.Drawable; import android.os.Build; import android.util.AttributeSet; import android.view.LayoutInflater; import android.view.View; import android.widget.RelativeLayout; import android.widget.TextView; /** * Created by Administrator on 2016/3/18. */ public class LouisTitleBar extends RelativeLayout implements View.OnClickListener { TextView tv_left; TextView tv_center; TextView tv_right; int left_img_resid=0; String left_text=null; int right_img_resid=0; String right_text= null; int mDrawablePadding=5;//默认 TitleBarClickListener titleBarClickListener; Context mContext; RelativeLayout rl_titlebar; public LouisTitleBar(Context context) { super(context); } public LouisTitleBar(Context context, AttributeSet attrs) { super(context, attrs); mContext=context; View view = LayoutInflater.from(context).inflate(R.layout.louis_title_bar, this); tv_left = (TextView) view.findViewById(R.id.id_tv_left); tv_left.setOnClickListener(this); tv_center = (TextView) view.findViewById(R.id.id_tv_center); tv_center.setOnClickListener(this); tv_right = (TextView) view.findViewById(R.id.id_tv_right); tv_right.setOnClickListener(this); rl_titlebar= (RelativeLayout) findViewById(R.id.id_rl_titlebar); } public void setTitleBarClickListener(TitleBarClickListener titleBarClickListener) { this.titleBarClickListener = titleBarClickListener; } public interface TitleBarClickListener { void leftClick(); void centerClick(); void rightClick(); } protected void setTitleBarBackgroundColor(Object colorOrDrawable){ if(colorOrDrawable instanceof Integer){ rl_titlebar.setBackgroundColor((Integer) colorOrDrawable); }else if(colorOrDrawable instanceof Drawable){ this.setDrawableByVersion(rl_titlebar,(Drawable) colorOrDrawable); } } protected void setCenterText(String text){ tv_center.setText(text); } protected void setCenterTextSize(float size){ tv_center.setTextSize(size); } protected void setCenterTextColor(Object colorOrColorStateList){ if(colorOrColorStateList instanceof Integer) { tv_center.setTextColor((Integer) colorOrColorStateList); }else if(colorOrColorStateList instanceof ColorStateList) { tv_center.setTextColor((ColorStateList) colorOrColorStateList); } } protected void setLeftAndRightTextSize(float size){ tv_left.setTextSize(size); tv_right.setTextSize(size); } protected void setLeftAndRightTextColor(Object colorOrColorStateList){ if(colorOrColorStateList instanceof Integer) { tv_left.setTextColor((Integer) colorOrColorStateList); tv_right.setTextColor((Integer) colorOrColorStateList); }else if(colorOrColorStateList instanceof ColorStateList) { tv_left.setTextColor((ColorStateList) colorOrColorStateList); tv_right.setTextColor((ColorStateList) colorOrColorStateList); } } protected void setCenterTextBackground(Object colorOrDrawable){ if(colorOrDrawable instanceof Integer){ tv_center.setBackgroundColor((Integer) colorOrDrawable); }else if(colorOrDrawable instanceof Drawable){ this.setDrawableByVersion(tv_center,(Drawable) colorOrDrawable); } } protected void setBarLeft(int imgResID,String tv_text){ left_img_resid=imgResID; left_text=tv_text; setBarLeftConfig(); } protected void setBarLeft(int imgResID){ left_img_resid=imgResID; left_text=""; setBarLeftConfig(); } protected void setBarLeft(String tv_text){ left_text=tv_text; left_img_resid=0; setBarLeftConfig(); } private void setBarLeftConfig(){ if (left_text!=null){ tv_left.setText(left_text); } if (left_img_resid!=0){ Drawable drawable=getDrawableByVersion(left_img_resid,mContext); drawable.setBounds(0, 0, drawable.getMinimumWidth(), drawable.getMinimumHeight()); tv_left.setCompoundDrawables(drawable, null, null, null);//设置TextView的drawableleft tv_left.setCompoundDrawablePadding(mDrawablePadding);//设置图片和text之间的间距 }else { tv_left.setCompoundDrawables(null, null, null, null);//设置TextView的drawableleft tv_left.setCompoundDrawablePadding(0); } } protected void setBarRight(int imgResID,String tv_text){ right_img_resid=imgResID; right_text=tv_text; setBarRightConfig(); } protected void setBarRight(int imgResID){ right_img_resid=imgResID; right_text=""; setBarRightConfig(); } protected void setBarRight(String tv_text){ right_img_resid=0; right_text=tv_text; setBarRightConfig(); } private void setBarRightConfig(){ if (right_text!=null){ tv_right.setText(right_text); } if (right_img_resid!=0){ Drawable drawable=getDrawableByVersion(right_img_resid,mContext); drawable.setBounds(0, 0, drawable.getMinimumWidth(), drawable.getMinimumHeight()); tv_right.setCompoundDrawables(null, null, drawable, null);//设置TextView的drawableleft tv_right.setCompoundDrawablePadding(mDrawablePadding);//设置图片和text之间的间距 }else { tv_right.setCompoundDrawables(null, null, null, null);//设置TextView的drawableleft tv_right.setCompoundDrawablePadding(0); } } private Drawable getDrawableByVersion(int resid, Context context){ if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) { return context.getResources().getDrawable(resid, context.getTheme()); } else { return context.getResources().getDrawable(resid); } } private void setDrawableByVersion(View view,Drawable drawable){ if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) { view.setBackground(drawable); }else{ view. setBackgroundDrawable(drawable); } } @Override public void onClick(View v) { switch (v.getId()) { case R.id.id_tv_left: titleBarClickListener.leftClick(); break; case R.id.id_tv_center: titleBarClickListener.centerClick(); break; case R.id.id_tv_right: titleBarClickListener.rightClick(); break; } } public void init(String titleText,int left_img_resid,String left_text,int right_img_resid,String right_text,int drawablePadding){ tv_center.setText(titleText); mDrawablePadding=drawablePadding; if (left_img_resid!=0) { this.setBarLeft(left_img_resid, left_text); }else { this.setBarLeft(left_text); } if (right_img_resid!=0) { this.setBarRight(right_img_resid, right_text); }else { this.setBarRight(right_text); } } }
2。xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/id_rl_titlebar" android:layout_width="match_parent" android:layout_height="50dp" android:background="#1aeb86" > <TextView android:id="@+id/id_tv_left" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_centerVertical="true" android:paddingLeft="30dp" android:gravity="center" android:drawablePadding="5dp" android:paddingRight="30dp" /> <TextView android:id="@+id/id_tv_center" android:paddingLeft="20dp" android:paddingRight="20dp" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_centerVertical="true" android:layout_centerInParent="true" android:gravity="center" /> <TextView android:id="@+id/id_tv_right" android:paddingLeft="30dp" android:paddingRight="30dp" android:layout_alignParentRight="true" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_centerVertical="true" android:gravity="center" /> </RelativeLayout>
3。aty
package com.louis.louiscomtitlebar; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.widget.Toast; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); LouisTitleBar louisTitleBar= (LouisTitleBar) findViewById(R.id.id_louis_title_bar); //louisTitleBar.init("中国",0,null,0,null); louisTitleBar.init("中国", R.mipmap.btn_left, "返回", 0, null, 0); // louisTitleBar.setBarRight(R.mipmap.right); louisTitleBar.setBarRight("XXX"); //louisTitleBar.setTitleBarBackgroundColor(Color.parseColor("#EEFFEE")); // louisTitleBar.setLeftAndRightTextColor(Color.parseColor("#365663")); // louisTitleBar.setBarRight(R.mipmap.right,"xdd"); // louisTitleBar.setBarLeft(R.mipmap.left); // louisTitleBar.setBarLeft("yyy"); louisTitleBar.setTitleBarClickListener(new LouisTitleBar.TitleBarClickListener() { @Override public void leftClick() { Toast.makeText(MainActivity.this, "left", Toast.LENGTH_SHORT).show(); } @Override public void centerClick() { Toast.makeText(MainActivity.this, "center", Toast.LENGTH_SHORT).show(); } @Override public void rightClick() { Toast.makeText(MainActivity.this, "right", Toast.LENGTH_SHORT).show(); } }); } }
4。xml 4aty
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.louis.louiscomtitlebar.MainActivity"> <com.louis.louiscomtitlebar.LouisTitleBar android:id="@+id/id_louis_title_bar" android:layout_width="match_parent" android:layout_height="wrap_content"> </com.louis.louiscomtitlebar.LouisTitleBar> </RelativeLayout>
结果;
demo下载:http://download.csdn.net/detail/richiezhu/9467089
相关文章推荐
- 上百个Android开源项目分享
- Running Android Lint has encountered a... Failed. java.lang.NullPointerException
- 快速开发一个属于自己的android数据库类库
- Parsing Data for android-N failed Unsupported major.minor version 51.0
- Android Canvas save() restore()
- failed to find target with hash string 'android-22'导入adt工程,编译时遇到这个报错。
- android开发系列之socket编程
- 解决:Unable to execute dex: Multiple dex files define Landroid/annotation/AnimRes
- android之发送Get或Post请求至服务器接口
- android开发系列之多线程
- android简单的夜间模式
- android中常用的匿名内部类的写法
- ADB运行框架原理解析
- FragmentTabhost的使用
- android 源码下载
- android控件框架介绍
- Android学习第三周_自定义控件、Fragment和Handler
- Android获取屏幕宽度与高度
- android:gravity与android:layout_gravity的区别
- android:layout_weight新认识