字典树Trie实战
2016-03-19 17:21
274 查看
问起起源于在学堂在线上的《软件工程》这门课,一般每章后面都会有一个实践作业,发现其难度可以比拟大一大二的课程大作业,早有这样的训练强度该有多好呢?呵呵O(∩_∩)O~
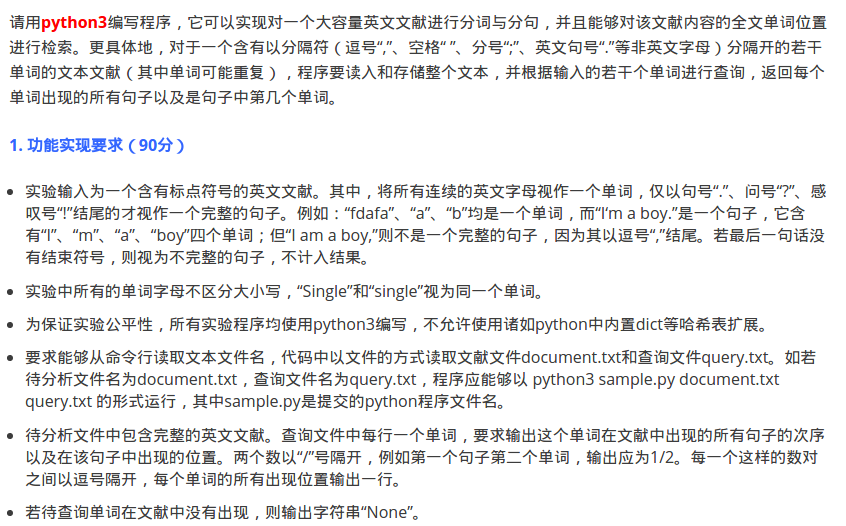
以上是基础的要求,其实字符串的题目一般都可以随便暴力做,所以评价一个算法的优劣,主要是看它在特定应用场景下的效率,比如:
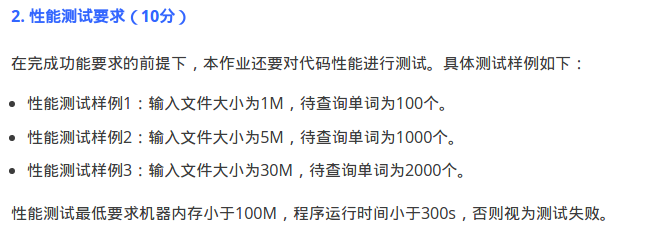
基于上面的要求,我决定使用“字典树”Trie来实现基于字符串的快速查找,字典树的描述请自行搜索,这里暂时只分享我自己的python 3实现。
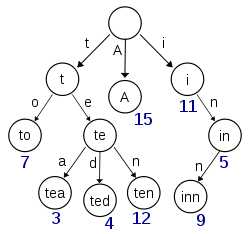
图片来自wiki百科
A. 问题描述
以上是基础的要求,其实字符串的题目一般都可以随便暴力做,所以评价一个算法的优劣,主要是看它在特定应用场景下的效率,比如:
基于上面的要求,我决定使用“字典树”Trie来实现基于字符串的快速查找,字典树的描述请自行搜索,这里暂时只分享我自己的python 3实现。
B. 实现
初次实现,有点乱,先mark一下,过些日子回来完善【未完待续】# !/usr/bin/env python3 # -*- coding: utf-8 -*- __author__ = 'jacket' import sys import re SIZE = 27 def char2int(ch): # return ord(ch) - ord('a') + 1 # for quick calculation, ord('a') = 97, so the above equal: return ord(ch) - 96 class TrieNode: def __init__(self, data=None): self.data = data self.cnt = 0 self.leafNode = None self.children = [] def haveChildren(self): return len(self.children) == SIZE def extend(self): self.children = [TrieNode() for _ in range(SIZE)] def addToLeaf(self, word): if not self.leafNode: self.leafNode = TrieNode(word) self.leafNode.cnt += 1 return self.leafNode.cnt - 1 def addToChild(self, ch, index): if not self.children[index].data: self.children[index].data = ch self.children[index].cnt += 1 if not self.leafNode: self.leafNode = TrieNode() self.leafNode.cnt += 1 class Trie: def __init__(self): self.root = TrieNode() def insert(self, word): now = self.root for ch in word: index = char2int(ch) if not now.haveChildren(): now.extend() now.addToChild(ch, index) now = now.children[index] return now.addToLeaf(word) def main(args): t = Trie() with open(args[0], 'r') as f: for line in f: if line[-1] == '\n': line = line[0:-1] if len(line) > 0 t.insert(line.lower()) print('Trie build') while True: q = input() if q == '0': break print(t.insert(q.lower())) return 0 if __name__ == '__main__': exit(main(sys.argv[1:]))
C. More
图片来自wiki百科
相关文章推荐
- 书评:《算法之美( Algorithms to Live By )》
- 动易2006序列号破解算法公布
- Ruby实现的矩阵连乘算法
- C#插入法排序算法实例分析
- 超大数据量存储常用数据库分表分库算法总结
- C#数据结构与算法揭秘二
- C#冒泡法排序算法实例分析
- 算法练习之从String.indexOf的模拟实现开始
- C#算法之关于大牛生小牛的问题
- C#实现的算24点游戏算法实例分析
- c语言实现的带通配符匹配算法
- 浅析STL中的常用算法
- 算法之排列算法与组合算法详解
- C++实现一维向量旋转算法
- Ruby实现的合并排序算法
- 字典树的基本知识及使用C语言的相关实现
- C#折半插入排序算法实现方法
- 基于C++实现的各种内部排序算法汇总
- C++线性时间的排序算法分析
- C++实现汉诺塔算法经典实例