android之DPAD上下左右四个键控制
2016-02-28 12:58
435 查看
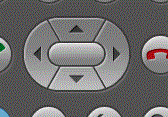
我们代码的目的很简单,那就是监听上下左右中这几个键的事件触发。直接上代码:
dpad.xml
[xhtml] view
plain copy
<?xml version="1.0" encoding="utf-8"?>
<AbsoluteLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#FFFFFF"
>
<Button
android:id="@+id/myButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="move me"
android:layout_x="20px"
android:layout_y="40px" />
</AbsoluteLayout>
activity代码:
[java] view
plain copy
package cn.com.chenzheng_java;
import android.app.Activity;
import android.os.Bundle;
import android.util.DisplayMetrics;
import android.view.KeyEvent;
import android.widget.AbsoluteLayout;
import android.widget.Button;
import android.widget.Toast;
/**
* @description 控制手机的上下左右四个方向键
* @author chenzheng_java
*
*/
public class DpadActivity extends Activity {
Button button;
DisplayMetrics metrics = new DisplayMetrics();
int screenx = 0 ;//屏幕宽度
int screeny = 0 ;//屏幕高度
int buttonWidth = 80;//按钮宽度
int buttonHeight = 40 ;// 按钮高度
int currentX = 0;// 按钮的当前x坐标
int currentY = 0;// 按钮的当前Y坐标
int step = 0;//移动时候的步长
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.dpad);
button = (Button) findViewById(R.id.myButton1);
getWindowManager().getDefaultDisplay().getMetrics(metrics);
screenx = metrics.widthPixels;
screeny = metrics.heightPixels;
/* buttonWidth = button.getWidth();
buttonHeight = button.getHeight();*/
currentX = (screenx-buttonWidth)/2;
currentY = (screeny-buttonHeight)/2;
step = 2;
button.setLayoutParams(new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, currentX, currentY));
}
/**
* 当前后左右键被按下的时候,被触发(这里可是有前提的哦,那就是当前的activity中必须没有view正在监听按键
* ,例如:当前如果有一个EditText正在等待输入,当我们按下dpad时,不会触发事件哦)
* Activity.onKeyDown();
当某个键被按下时会触发,但不会被任何的该Activity内的任何view处理。
默认按下KEYCODE_BACK键后会回到上一个Activity。
*/
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
switch (keyCode) {
case KeyEvent.KEYCODE_DPAD_DOWN://按向下键
moveDown();
break;
case KeyEvent.KEYCODE_DPAD_UP:// 按向上键
moveUp();
case KeyEvent.KEYCODE_DPAD_LEFT://按向左键
moveLeft();
case KeyEvent.KEYCODE_DPAD_RIGHT://按向右键
moveRight();
default:
break;
}
return super.onKeyDown(keyCode, event);
}
@SuppressWarnings("deprecation")
private void moveDown(){
int temp = currentY+step;
if(temp>(screeny-buttonHeight)){
showToast("到头了哦!");
button.setLayoutParams(new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, screenx, screeny-buttonHeight));
}
else{
currentY = currentY+step;
AbsoluteLayout.LayoutParams params =
new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, currentX, currentY);
button.setLayoutParams(params);
}
//button.setLayoutParams(new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, currentX, currentY-2));
}
@SuppressWarnings("deprecation")
private void moveUp(){
int temp = currentY-step;
if(temp<=0){
showToast("往上到头了哦!");
button.setLayoutParams(new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, screenx, 0));
}
else{
currentY = currentY-step;
AbsoluteLayout.LayoutParams params =
new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, currentX, currentY);
button.setLayoutParams(params);
}
}
@SuppressWarnings("deprecation")
private void moveLeft(){
int temp = currentX-step;
if(temp<=0){
showToast("往左边到头了哦!");
button.setLayoutParams(new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, 0, screeny));
}
else{
currentX = currentX-step;
AbsoluteLayout.LayoutParams params =
new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, currentX, currentY);
button.setLayoutParams(params);
}
}
@SuppressWarnings("deprecation")
private void moveRight(){
int temp = currentX+step;
if(temp>=(screenx-buttonWidth)){
showToast("往右边到头了哦!");
button.setLayoutParams(new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, screenx-buttonWidth, currentY));
}
else{
currentX = currentX+step;
AbsoluteLayout.LayoutParams params =
new AbsoluteLayout.LayoutParams(buttonWidth, buttonHeight, currentX, currentY);
button.setLayoutParams(params);
}
}
/**
* 弹出提示信息
* @param text 提示信息
*/
private void showToast(String text){
Toast.makeText(this, text, Toast.LENGTH_LONG).show();
}
}
---------------------------------------------------------------------------------------
这里我们可以看到,要想监听这几个方向键,那么我们必须重写 public boolean onKeyDown(int keyCode, KeyEvent event)这个方法,该方法定义在Activity中,其中keyCode便是代表着你点击的那个键的标识符,KeyEvent 则是一个事件。
需要注意的是,坐标问题:我们控制的按钮的坐标实际上是左上角的坐标。而屏幕的向左是X轴,向下是Y轴,也就是说,只要在屏幕内显示的组件,其坐标都是正数的。
当然键盘中所有的键都可以监听的,我们亦可以来监听A-Z这些键,他们的keyCode依次是KEYCODE_A -------KEYCODE_Z.
这里有一个小技巧,可以判断出用户输入的是什么A-Z那个字符,代码如下:
[java] view
plain copy
int code = 'A'+keycode-29;
char ch = (char)code;
如果我们无法获取当前的按键,我们可以通过keycode_unknown来捕捉。
控制音量大小:keycode_volume_down / keycode_volume_up.
-----------------------------------------------------------------------------------------
关于KeyEvent,它代表了我们点击键盘时的事件,看下图,我们可以创建自己的键盘事件

其中参数中的action,代表了用户的操作,值为ACTION_DOWN、ACTION_UP、ACTION_MULTIPLE其中的一个。而code则代表了我们的标识符,入上面提到的keycode_volume_down 就是一个code。
相关文章推荐
- Android进行短信备份的一个工具类,支持进度条显示
- #Android学习#Android权限
- Android分析第三方应用layout的神器
- Android性能优化系列---避免ANR
- [PhoneGap] Android開發Facebook取得Key Hashes
- Android学习资料整理
- [Android]自定义控件LoadMoreRecyclerView
- Android: 解决动画完成后位置恢复到初始位置的问题
- Android测试系列之Local Unit Test
- Android拍照得到全尺寸图片并进行压缩
- Android Kotlin入门-控制流
- 2.11 Android Studio的常用快捷键
- HelloWorld-----Google手机操作系统Android应用开发入门
- Android DPAD按键无法使用
- [android] logcat简介
- Android:Canvas中drawText的尺寸计算
- Monkey日志信息Event percentages说明
- android 异常处理--java.io.IOException: 您的主机中的软件中止了一个已建立的连接
- Ubuntu完美下载Android源码
- Android自动化压力测试图解教程——Monkey工具