U3D架构系列之- FSM有限状态机设计三
2016-02-02 11:37
716 查看
由 Benz 于 星期一, 2015-02-02 11:37 发表
在设计二中,我们实现了有限状态机管理类,接下来,我们实现FSState这个类,这里类主要是状态的基本操作以及事件触发。在这里我们定义了在FiniteStateMachine类里声明的三个委托。在FSState里面使用的代码如下:
1 protected FiniteStateMachine.EnterState mEnterDelegate;
2 protected FiniteStateMachine.PushState mPushDelegate;
3 protected FiniteStateMachine.PopState mPopDelegate;
这个FSState是独立的一个类,不继承Mono,我们定义了一个构造函数,将我们的委托进行了初始化:
1 public FSState(IState obj, FiniteStateMachine owner, string name, FiniteStateMachine.EnterState e, FiniteStateMachine.PushState pu, FiniteStateMachine.PopState po) {
2 mStateObject = obj;
3 mStateName = name;
4 mOwner = owner;
5 mEnterDelegate = e;
6 mPushDelegate = pu;
7 mPopDelegate = po;
8 mTranslationEvents = new Dictionary();
9
10 }
我们声明了FSEvent事件处理函数用于将事件的名字和事件加入的Dictionary里面
1public FSEvent On(string eventName) {
2 FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
3 mTranslationEvents.Add(eventName, newEvent);
4 return newEvent;
5
6 }
加入到列表后,我们需要从表里面取出去执行,这就需要Trigger触发函数:
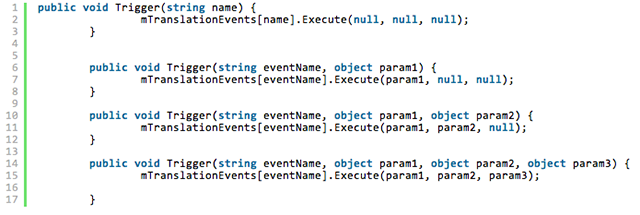
以上也是FSState类的核心代码,闲话少说,FSState类的整个代码,如下:
using System;
using System.Collections;
using System.Collections.Generic;
public class FSState {
protected FiniteStateMachine.EnterState mEnterDelegate;
protected FiniteStateMachine.PushState mPushDelegate;
protected FiniteStateMachine.PopState mPopDelegate;
protected IState mStateObject;
protected string mStateName;
protected FiniteStateMachine mOwner;
protected Dictionary mTranslationEvents;
public FSState(IState obj, FiniteStateMachine owner, string name, FiniteStateMachine.EnterState e, FiniteStateMachine.PushState pu, FiniteStateMachine.PopState po) {
mStateObject = obj;
mStateName = name;
mOwner = owner;
mEnterDelegate = e;
mPushDelegate = pu;
mPopDelegate = po;
mTranslationEvents = new Dictionary();
}
public IState StateObject {
get {
return mStateObject;
}
}
public string StateName {
get {
return mStateName;
}
}
public FSEvent On(string eventName) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
mTranslationEvents.Add(eventName, newEvent);
return newEvent;
}
public void Trigger(string name) {
mTranslationEvents[name].Execute(null, null, null);
}
public void Trigger(string eventName, object param1) {
mTranslationEvents[eventName].Execute(param1, null, null);
}
public void Trigger(string eventName, object param1, object param2) {
mTranslationEvents[eventName].Execute(param1, param2, null);
}
public void Trigger(string eventName, object param1, object param2, object param3) {
mTranslationEvents[eventName].Execute(param1, param2, param3);
}
public FSState On(string eventName, Func action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T param1;
try { param1 = (T)o1; } catch { param1 = default(T); }
action(param1);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Action action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T param1;
try { param1 = (T)o1; } catch { param1 = default(T); }
action(param1);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Func action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T1 param1;
T2 param2;
try { param1 = (T1)o1; } catch { param1 = default(T1); }
try { param2 = (T2)o2; } catch { param2 = default(T2); }
action(param1, param2);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Action action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T1 param1;
T2 param2;
try { param1 = (T1)o1; } catch { param1 = default(T1); }
try { param2 = (T2)o2; } catch { param2 = default(T2); }
action(param1, param2);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Func action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T1 param1;
T2 param2;
T3 param3;
try { param1 = (T1)o1; } catch { param1 = default(T1); }
try { param2 = (T2)o2; } catch { param2 = default(T2); }
try { param3 = (T3)o3; } catch { param3 = default(T3); }
action(param1, param2, param3);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Action action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T1 param1;
T2 param2;
T3 param3;
try { param1 = (T1)o1; } catch { param1 = default(T1); }
try { param2 = (T2)o2; } catch { param2 = default(T2); }
try { param3 = (T3)o3; } catch { param3 = default(T3); }
action(param1, param2, param3);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
}
接下来会在设计四中继续讲解 FSEvent类
本文出自 “海游移动” 博客,请务必保留此出处 /article/4237452.html
在设计二中,我们实现了有限状态机管理类,接下来,我们实现FSState这个类,这里类主要是状态的基本操作以及事件触发。在这里我们定义了在FiniteStateMachine类里声明的三个委托。在FSState里面使用的代码如下:
1 protected FiniteStateMachine.EnterState mEnterDelegate;
2 protected FiniteStateMachine.PushState mPushDelegate;
3 protected FiniteStateMachine.PopState mPopDelegate;
这个FSState是独立的一个类,不继承Mono,我们定义了一个构造函数,将我们的委托进行了初始化:
1 public FSState(IState obj, FiniteStateMachine owner, string name, FiniteStateMachine.EnterState e, FiniteStateMachine.PushState pu, FiniteStateMachine.PopState po) {
2 mStateObject = obj;
3 mStateName = name;
4 mOwner = owner;
5 mEnterDelegate = e;
6 mPushDelegate = pu;
7 mPopDelegate = po;
8 mTranslationEvents = new Dictionary();
9
10 }
我们声明了FSEvent事件处理函数用于将事件的名字和事件加入的Dictionary里面
1public FSEvent On(string eventName) {
2 FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
3 mTranslationEvents.Add(eventName, newEvent);
4 return newEvent;
5
6 }
加入到列表后,我们需要从表里面取出去执行,这就需要Trigger触发函数:
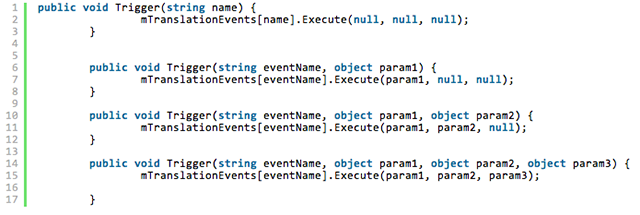
以上也是FSState类的核心代码,闲话少说,FSState类的整个代码,如下:
using System;
using System.Collections;
using System.Collections.Generic;
public class FSState {
protected FiniteStateMachine.EnterState mEnterDelegate;
protected FiniteStateMachine.PushState mPushDelegate;
protected FiniteStateMachine.PopState mPopDelegate;
protected IState mStateObject;
protected string mStateName;
protected FiniteStateMachine mOwner;
protected Dictionary mTranslationEvents;
public FSState(IState obj, FiniteStateMachine owner, string name, FiniteStateMachine.EnterState e, FiniteStateMachine.PushState pu, FiniteStateMachine.PopState po) {
mStateObject = obj;
mStateName = name;
mOwner = owner;
mEnterDelegate = e;
mPushDelegate = pu;
mPopDelegate = po;
mTranslationEvents = new Dictionary();
}
public IState StateObject {
get {
return mStateObject;
}
}
public string StateName {
get {
return mStateName;
}
}
public FSEvent On(string eventName) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
mTranslationEvents.Add(eventName, newEvent);
return newEvent;
}
public void Trigger(string name) {
mTranslationEvents[name].Execute(null, null, null);
}
public void Trigger(string eventName, object param1) {
mTranslationEvents[eventName].Execute(param1, null, null);
}
public void Trigger(string eventName, object param1, object param2) {
mTranslationEvents[eventName].Execute(param1, param2, null);
}
public void Trigger(string eventName, object param1, object param2, object param3) {
mTranslationEvents[eventName].Execute(param1, param2, param3);
}
public FSState On(string eventName, Func action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T param1;
try { param1 = (T)o1; } catch { param1 = default(T); }
action(param1);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Action action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T param1;
try { param1 = (T)o1; } catch { param1 = default(T); }
action(param1);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Func action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T1 param1;
T2 param2;
try { param1 = (T1)o1; } catch { param1 = default(T1); }
try { param2 = (T2)o2; } catch { param2 = default(T2); }
action(param1, param2);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Action action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T1 param1;
T2 param2;
try { param1 = (T1)o1; } catch { param1 = default(T1); }
try { param2 = (T2)o2; } catch { param2 = default(T2); }
action(param1, param2);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Func action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T1 param1;
T2 param2;
T3 param3;
try { param1 = (T1)o1; } catch { param1 = default(T1); }
try { param2 = (T2)o2; } catch { param2 = default(T2); }
try { param3 = (T3)o3; } catch { param3 = default(T3); }
action(param1, param2, param3);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
public FSState On(string eventName, Action action) {
FSEvent newEvent = new FSEvent(eventName, null, this, mOwner, mEnterDelegate, mPushDelegate, mPopDelegate);
newEvent.mAction = delegate (object o1, object o2, object o3) {
T1 param1;
T2 param2;
T3 param3;
try { param1 = (T1)o1; } catch { param1 = default(T1); }
try { param2 = (T2)o2; } catch { param2 = default(T2); }
try { param3 = (T3)o3; } catch { param3 = default(T3); }
action(param1, param2, param3);
return true;
};
mTranslationEvents.Add(eventName, newEvent);
return this;
}
}
接下来会在设计四中继续讲解 FSEvent类
本文出自 “海游移动” 博客,请务必保留此出处 /article/4237452.html
相关文章推荐
- U3D架构系列之- FSM有限状态机设计二
- U3d架构系列之:FSM有限状态机设计一
- 架构思想
- android基本架构
- Code Project网站上关注的一些人
- JavaBean——四舍五入(保留一定的小数),MVC设计模式,EL表达式和JSTL标签快速入门,软件三层架构设计
- WordPress优化:为网站添加个性化缩略图标
- IIS express 7.5 配置和多网站执行
- 理解RESTful架构
- iOS应用架构
- web架构设计经验分享
- 屏幕尺寸、密度,分辨率查询网站
- 网站性能优化·前端篇
- 动图表情网站 Giphy 发家史:我们才不是小黄图图库
- LVS+Keepalived实现高可用负载均衡
- 小型电商网站的架构
- 理解RESTful架构
- 架构演化
- (1)架构和目录结构
- 为满足不断增长的业务需求 升级现有的服务器架构--达达