android学习摘记——TabHost使用代码
2015-12-16 15:03
567 查看
一.实现Tab样式布局
现在的app设计多数会采用tab的形式,IOS有TabViewController这个控制器 android有一个TabHost,
以下这种是继承TabActivity的做法:
但是android的TabHost有几个注意点
1.TabHost标签的ID 必须是 :tabhost
2.FrameLayout标签的ID必须是:tabcontent
3. TabWidget标签的ID必须是:tabs
否则会报错
TabHost有顶部和底部展示的 Ta依赖这个属性来设置
android:layout_alignParentBottom="true"
1.创建一个TabHost的Activity :activity_main.xml
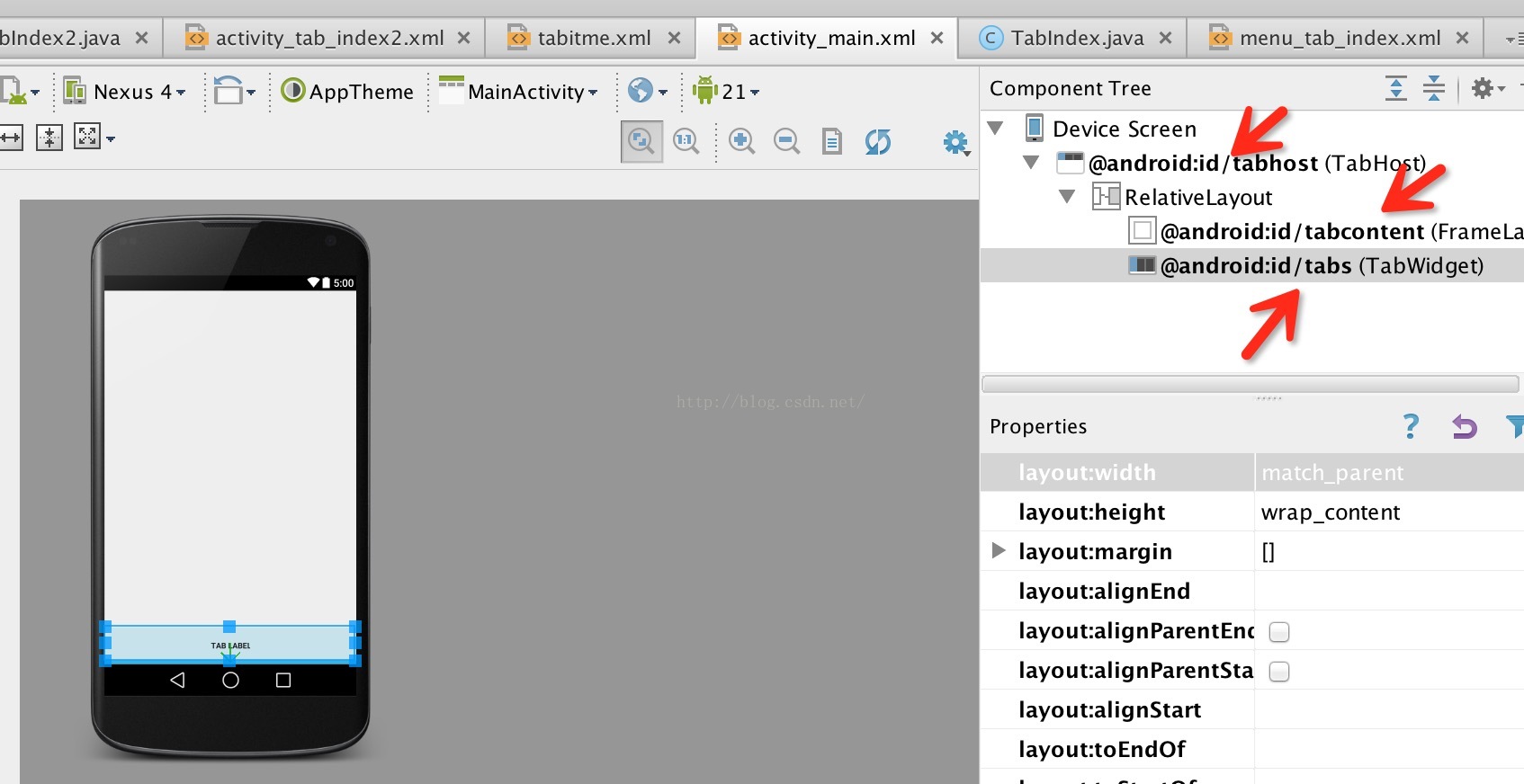
(注意以上的ID)TabWidget可以设置分割线 但是似乎不美观 android:showDividers="none"
2,定义TabItem的样式布局 tabitem.xml
3.接下来就是在Activity里面的操作了
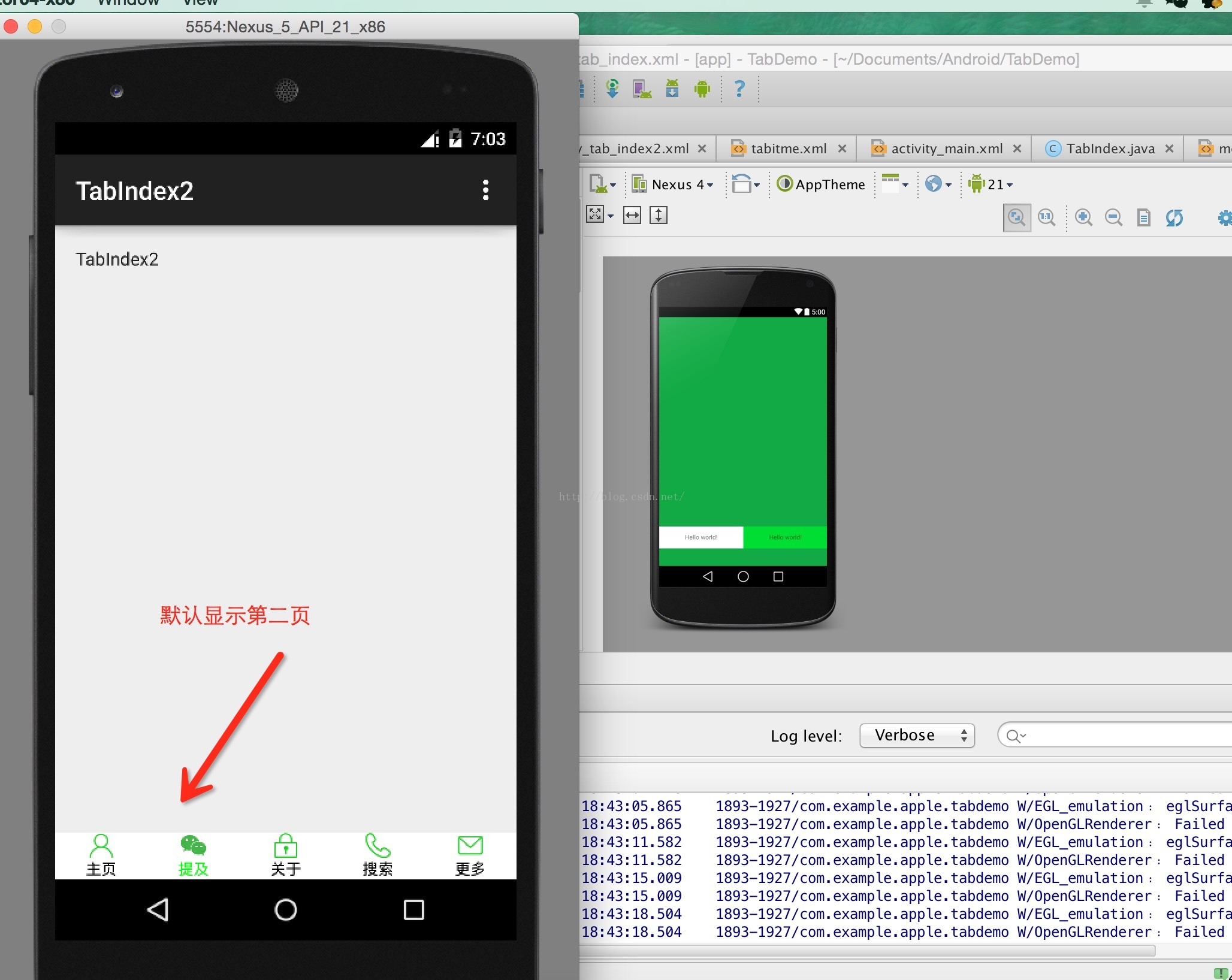
和IOS的TabViewController一样 是用Tab承载几个VC页面,里面的 prepareIntent()就是负责VC页面的承载 与IOS的TabItem不一样的是 android的TabHost的Itme选中样式要自己修改 但是这样比IOS更灵活 tabHost.setOnTabChangedListener(new TabHost.OnTabChangeListener() 兼听tab切换的事件 然后做对应的tab的字体,图片或者背景的修改。
默认显示第几个VC的 使用:
//默认显示第几个VC
int showIndex = 1;
tabHost.setCurrentTab(showIndex);
configTabItemStyle(showIndex,true);
经过以上的3个步骤 Tab的框架页面已经基本完成了
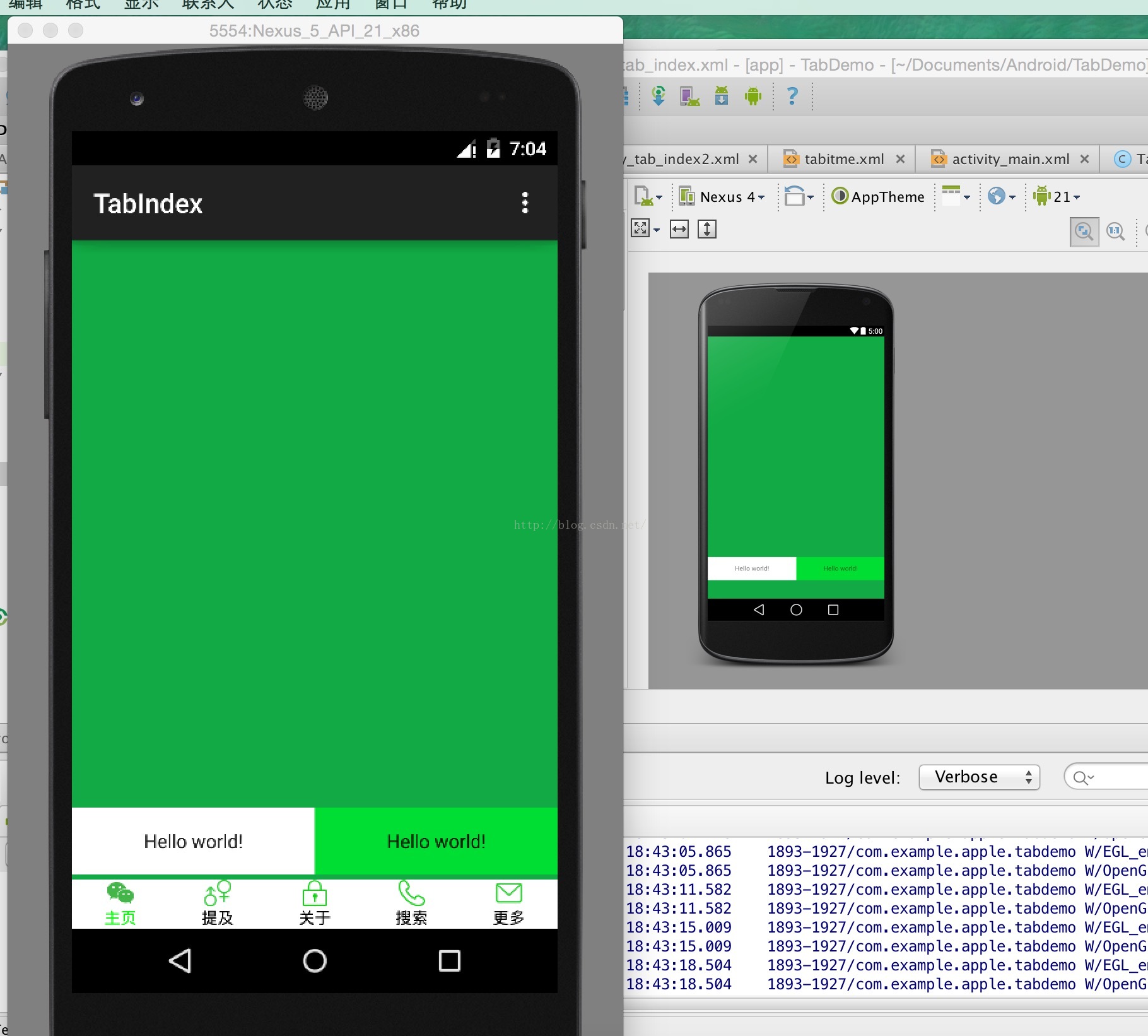
因为是demo 所以这里的资源比较丑, 选中之后 修改字体颜色和替换icon
二.优化布局样式
以上发现布局特别难看,发现两个问题
1.tab高度太矮
2.内容页面的布局受影响(不能靠着底部)
IOS的Tab固定高度是44,android的tab高度我可以手动设置,在之前的activity_main.xml里面 我们可以修改tab的高度在TabWidget标签里面设置 android:layout_height="50dp" tab高度改为50dp,对应的tabitme.xml的布局也要做对应的修改,否则,文字和图标不居中也是很丑的,还有就是tabitme高度变化 会挡住内容页的底部,所以在activity_main.xml
的FrameLayout标签里面要加一个间距底部的高度
android:paddingBottom="50dp"
修改后的activity_main.xml样式
<?xml version="1.0" encoding="utf-8"?>
<TabHost xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@android:id/tabhost"
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<FrameLayout
android:id="@android:id/tabcontent"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="50dp"
/>
<TabWidget
android:id="@android:id/tabs"
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_alignParentBottom="true"
android:showDividers="none"></TabWidget>
</RelativeLayout>
</TabHost>
修改后的tabitem.xml样式
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="50dp"
android:orientation="vertical"
android:background="#fff"
>
<ImageView android:id="@+id/tab_iv_icon"
android:layout_width="match_parent"
android:layout_height="20.0dip"
android:scaleType="fitCenter"
android:layout_marginTop="10dp"
/>
<TextView android:id="@+id/tab_tv_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="12.0sp"
android:textColor="#000"
android:ellipsize="marquee"
android:gravity="center"
android:singleLine="true"
android:marqueeRepeatLimit="1"/>
</LinearLayout>
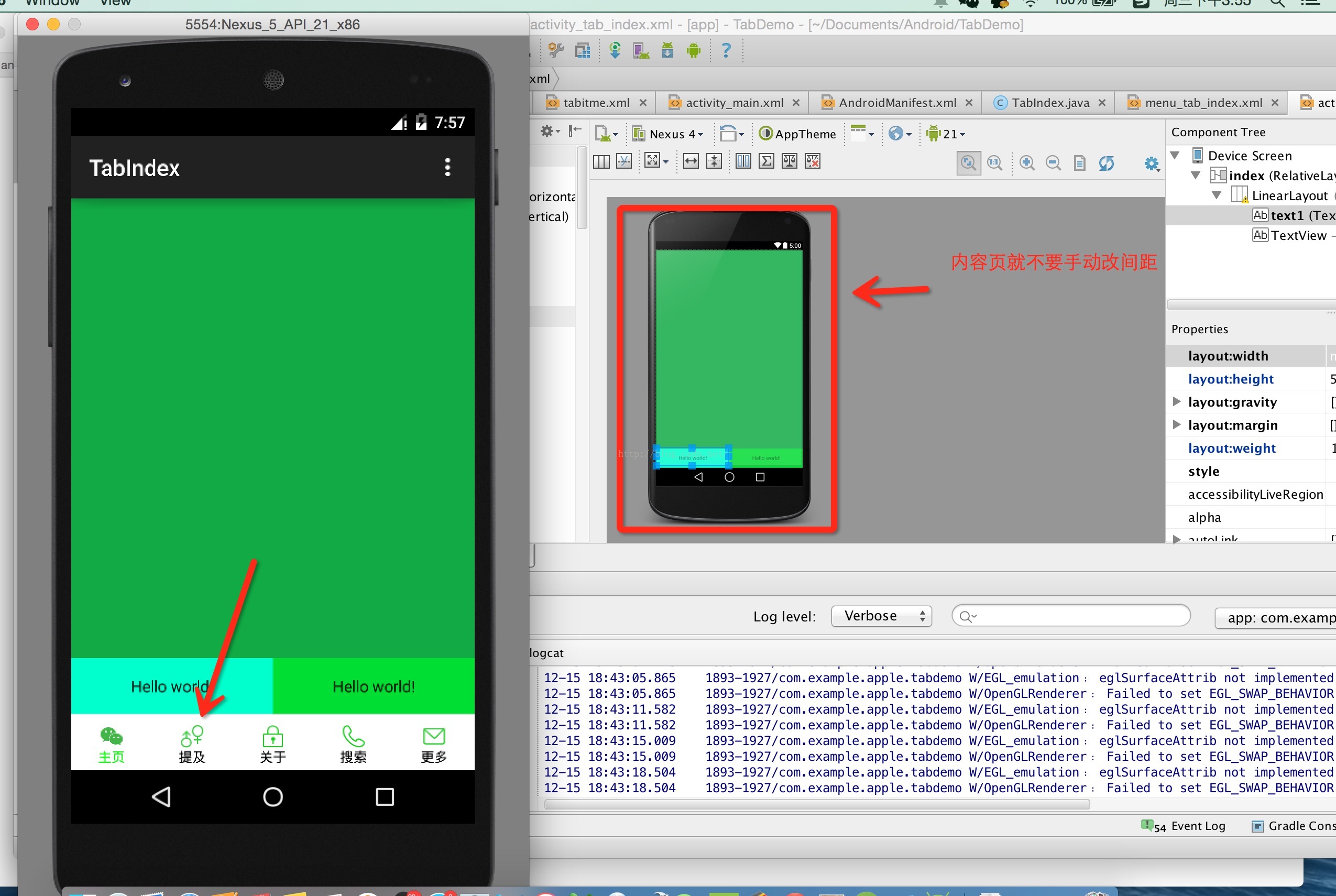
完成!
现在的app设计多数会采用tab的形式,IOS有TabViewController这个控制器 android有一个TabHost,
以下这种是继承TabActivity的做法:
但是android的TabHost有几个注意点
1.TabHost标签的ID 必须是 :tabhost
2.FrameLayout标签的ID必须是:tabcontent
3. TabWidget标签的ID必须是:tabs
否则会报错
TabHost有顶部和底部展示的 Ta依赖这个属性来设置
android:layout_alignParentBottom="true"
1.创建一个TabHost的Activity :activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <TabHost xmlns:android="http://schemas.android.com/apk/res/android" android:id="@android:id/tabhost" android:layout_width="match_parent" android:layout_height="match_parent"> <RelativeLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <FrameLayout android:id="@android:id/tabcontent" android:layout_width="match_parent" android:layout_height="match_parent" /> <TabWidget android:id="@android:id/tabs" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:showDividers="none"></TabWidget> </RelativeLayout> </TabHost>
(注意以上的ID)TabWidget可以设置分割线 但是似乎不美观 android:showDividers="none"
2,定义TabItem的样式布局 tabitem.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:background="#fff" > <ImageView android:id="@+id/tab_iv_icon" android:layout_width="match_parent" android:layout_height="20.0dip" android:scaleType="fitCenter"/> <TextView android:id="@+id/tab_tv_text" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="12.0sp" android:textColor="#000" android:ellipsize="marquee" android:gravity="center" android:singleLine="true" android:marqueeRepeatLimit="1"/> </LinearLayout>
3.接下来就是在Activity里面的操作了
package com.example.apple.tabdemo; import android.app.TabActivity; import android.content.Intent; import android.graphics.Color; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.ImageView; import android.widget.TabHost; import android.widget.TextView; import android.widget.Toast; public class MainActivity extends TabActivity { private TabHost tabHost; private static final String HOME = "主页"; private static final String REFER = "提及"; private static final String ABOUT = "关于"; private static final String SEARCH = "搜索"; private static final String MORE = "更多"; //内容Intent private Intent homeIntent; private Intent referIntent; private Intent aboutIntent; private Intent searchIntent; private Intent moreIntent; public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);//设置TabHost使用的布局文件 tabHost=this.getTabHost(); //构建承载的页面 prepareIntent(); setupIntent(); tabHost.setOnTabChangedListener(new TabHost.OnTabChangeListener() { @Override public void onTabChanged(String tabId) { for (int i= 0;i<tabHost.getTabWidget().getChildCount();i++) { configTabItemStyle(i,false); } configTabItemStyle(tabHost.getCurrentTab(),true); } }); //默认显示第几个VC int showIndex = 1; tabHost.setCurrentTab(showIndex); configTabItemStyle(showIndex,true); } //配置tab itme的资源和对应的页面 private void setupIntent(){ tabHost.addTab(buildTabSpec(HOME,imageWithIndex(0,false), homeIntent)); tabHost.addTab(buildTabSpec(REFER,imageWithIndex(1,false), referIntent)); tabHost.addTab(buildTabSpec(ABOUT,imageWithIndex(2,false), aboutIntent)); tabHost.addTab(buildTabSpec(SEARCH,imageWithIndex(3,false), searchIntent)); tabHost.addTab(buildTabSpec(MORE,imageWithIndex(4,false), moreIntent)); } //构建Tab Item private TabHost.TabSpec buildTabSpec(String tag, int icon, Intent intent) { View view = View.inflate(MainActivity.this, R.layout.tabitme, null); ((ImageView)view.findViewById(R.id.tab_iv_icon)).setImageResource(icon); ((TextView)view.findViewById(R.id.tab_tv_text)).setText(tag); return tabHost.newTabSpec(tag).setIndicator(view).setContent(intent); } //初始化准备Tab对应的页面 private void prepareIntent() { homeIntent=new Intent(this, TabIndex.class); referIntent=new Intent(this, TabIndex2.class); aboutIntent=new Intent(this, TabIndex.class); searchIntent=new Intent(this,TabIndex.class); moreIntent=new Intent(this, TabIndex.class); } //配置Tab选中和非选中的样式 public void configTabItemStyle(int index,boolean isSelected) { View tabView = getTabHost().getTabWidget().getChildTabViewAt(index); TextView tv = (TextView)tabView.findViewById(R.id.tab_tv_text); //for Selected Tab ImageView imageView = (ImageView)tabView.findViewById(R.id.tab_iv_icon); //for Selected Tab if (isSelected) { tv.setTextColor(Color.GREEN); } else { tv.setTextColor(Color.BLACK); } imageView.setImageResource(imageWithIndex(index,isSelected)); } public int imageWithIndex(int index,boolean isSelected) { int imageRescource = 0; switch (index) { case 0: imageRescource = !isSelected ? R.drawable.icon_name : R.drawable.bg_icon_weixin; break; case 1: imageRescource = !isSelected ? R.drawable.icon_sex : R.drawable.bg_icon_weixin; break; case 2: imageRescource = !isSelected ? R.drawable.icon_password : R.drawable.bg_icon_weixin; break; case 3: imageRescource = !isSelected ? R.drawable.icon_phone : R.drawable.bg_icon_weixin; break; case 4: imageRescource = !isSelected ? R.drawable.icon_yanzheng : R.drawable.bg_icon_weixin; break; default: imageRescource = R.drawable.icon_yanzheng; break; } return imageRescource; } }
和IOS的TabViewController一样 是用Tab承载几个VC页面,里面的 prepareIntent()就是负责VC页面的承载 与IOS的TabItem不一样的是 android的TabHost的Itme选中样式要自己修改 但是这样比IOS更灵活 tabHost.setOnTabChangedListener(new TabHost.OnTabChangeListener() 兼听tab切换的事件 然后做对应的tab的字体,图片或者背景的修改。
默认显示第几个VC的 使用:
//默认显示第几个VC
int showIndex = 1;
tabHost.setCurrentTab(showIndex);
configTabItemStyle(showIndex,true);
经过以上的3个步骤 Tab的框架页面已经基本完成了
因为是demo 所以这里的资源比较丑, 选中之后 修改字体颜色和替换icon
二.优化布局样式
以上发现布局特别难看,发现两个问题
1.tab高度太矮
2.内容页面的布局受影响(不能靠着底部)
IOS的Tab固定高度是44,android的tab高度我可以手动设置,在之前的activity_main.xml里面 我们可以修改tab的高度在TabWidget标签里面设置 android:layout_height="50dp" tab高度改为50dp,对应的tabitme.xml的布局也要做对应的修改,否则,文字和图标不居中也是很丑的,还有就是tabitme高度变化 会挡住内容页的底部,所以在activity_main.xml
的FrameLayout标签里面要加一个间距底部的高度
android:paddingBottom="50dp"
修改后的activity_main.xml样式
<?xml version="1.0" encoding="utf-8"?>
<TabHost xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@android:id/tabhost"
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<FrameLayout
android:id="@android:id/tabcontent"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="50dp"
/>
<TabWidget
android:id="@android:id/tabs"
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_alignParentBottom="true"
android:showDividers="none"></TabWidget>
</RelativeLayout>
</TabHost>
修改后的tabitem.xml样式
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="50dp"
android:orientation="vertical"
android:background="#fff"
>
<ImageView android:id="@+id/tab_iv_icon"
android:layout_width="match_parent"
android:layout_height="20.0dip"
android:scaleType="fitCenter"
android:layout_marginTop="10dp"
/>
<TextView android:id="@+id/tab_tv_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="12.0sp"
android:textColor="#000"
android:ellipsize="marquee"
android:gravity="center"
android:singleLine="true"
android:marqueeRepeatLimit="1"/>
</LinearLayout>
完成!
相关文章推荐
- Android编程实现禁止系统锁屏与解锁亮屏的方法
- Android下获取各种存储目录
- android Tether 分析
- android studio 更新 Gradle错误解决方法
- Android-JNI(5)-C语言调用Java函数
- Android中线程池ExecutorService的使用
- android 关于读取SD卡或者U盘的一些方法
- Android Studio添加assets文件夹
- android - Activity启动模式
- Android Studio导入recyclerview,cardview
- Android5.0练习
- Android实战 - 音心播放器 (MusciActivity-专辑图片获得,基本控制实现)
- Android 设计模式 之 单例模式
- Android IntentService
- 学习Android系统控件SwipeRefreshLayout
- Android并发编程之如何使用ReentrantReadWriteLock替代synchronized来提高程序的效率
- Android:新手必备的常用代码片段整理(二)
- Android百度推送使用详解
- Android 中 SQLite 性能优化
- Android避免启动时闪一下黑屏