POJ 2195:Going Home
2015-12-08 21:00
417 查看
Going Home
Description
On a grid map there are n little men and n houses. In each unit time, every little man can move one unit step, either horizontally, or vertically, to an adjacent point. For each little man, you need to pay a $1 travel fee for every step he moves, until he enters
a house. The task is complicated with the restriction that each house can accommodate only one little man.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates
there is a little man on that point.
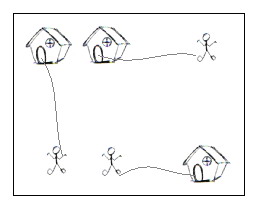
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Input
There are one or more test cases in the input. Each case starts with a line giving two integers N and M, where N is the number of rows of the map, and M is the number of columns. The rest of the input will be N lines describing the map. You may assume both
N and M are between 2 and 100, inclusive. There will be the same number of 'H's and 'm's on the map; and there will be at most 100 houses. Input will terminate with 0 0 for N and M.
Output
For each test case, output one line with the single integer, which is the minimum amount, in dollars, you need to pay.
Sample Input
Sample Output
在看最小费用最大流,这道题应该是这个类型最基础最典型的了。
题意是地图上有一堆人,一堆房子。每个房子只能住一个人,一个人到一个房子的代价是横坐标之差+纵坐标之差。问这些人都进入房子,总共需要最小的代价是多少。
其实和最大流的思想是一模一样的,网上的思想也都是大同小异的(这时候又想起了三鲜的那篇日志,让我很有罪恶感,但是这个题不学这个算法真的想不到。。。)。就是在满足这个路能走流量的情况下,不断去找代价最小的那条路径,不断找增广路。然后在遍历一遍,找到一条路径中的最小流量,乘以每段路的代价。不断迭代。
代码:
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 20223 | Accepted: 10257 |
On a grid map there are n little men and n houses. In each unit time, every little man can move one unit step, either horizontally, or vertically, to an adjacent point. For each little man, you need to pay a $1 travel fee for every step he moves, until he enters
a house. The task is complicated with the restriction that each house can accommodate only one little man.
Your task is to compute the minimum amount of money you need to pay in order to send these n little men into those n different houses. The input is a map of the scenario, a '.' means an empty space, an 'H' represents a house on that point, and am 'm' indicates
there is a little man on that point.
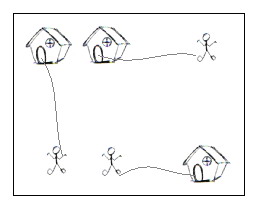
You can think of each point on the grid map as a quite large square, so it can hold n little men at the same time; also, it is okay if a little man steps on a grid with a house without entering that house.
Input
There are one or more test cases in the input. Each case starts with a line giving two integers N and M, where N is the number of rows of the map, and M is the number of columns. The rest of the input will be N lines describing the map. You may assume both
N and M are between 2 and 100, inclusive. There will be the same number of 'H's and 'm's on the map; and there will be at most 100 houses. Input will terminate with 0 0 for N and M.
Output
For each test case, output one line with the single integer, which is the minimum amount, in dollars, you need to pay.
Sample Input
2 2 .m H. 5 5 HH..m ..... ..... ..... mm..H 7 8 ...H.... ...H.... ...H.... mmmHmmmm ...H.... ...H.... ...H.... 0 0
Sample Output
2 10 28
在看最小费用最大流,这道题应该是这个类型最基础最典型的了。
题意是地图上有一堆人,一堆房子。每个房子只能住一个人,一个人到一个房子的代价是横坐标之差+纵坐标之差。问这些人都进入房子,总共需要最小的代价是多少。
其实和最大流的思想是一模一样的,网上的思想也都是大同小异的(这时候又想起了三鲜的那篇日志,让我很有罪恶感,但是这个题不学这个算法真的想不到。。。)。就是在满足这个路能走流量的情况下,不断去找代价最小的那条路径,不断找增广路。然后在遍历一遍,找到一条路径中的最小流量,乘以每段路的代价。不断迭代。
代码:
#pragma warning(disable:4996) #include <iostream> #include <algorithm> #include <cmath> #include <vector> #include <string> #include <cstring> #include <queue> #include <map> using namespace std; typedef long long ll; #define INF 200000 struct no { int x; int y; }man[505], house[505]; inline int step(int i, int j) { return abs(man[i].x - house[j].x) + abs(man[i].y - house[j].y); } int res; int n, m; int cnt_m, cnt_h; int d[505]; int vis[505]; int pre[505]; char val[105][105]; int cost[505][505]; int con[505][505]; void input() { int i, j, k; memset(val, 0, sizeof(val)); cnt_m = 0; cnt_h = 0; for (i = 1; i <= n; i++) { cin >> val[i] + 1; for (j = 1; j <= m; j++) { if (val[i][j] == 'm') { cnt_m++; man[cnt_m].x = i; man[cnt_m].y = j; } if (val[i][j] == 'H') { cnt_h++; house[cnt_h].x = i; house[cnt_h].y = j; } } } } bool spfa() { int i, j, k; for (i = 1; i <= cnt_h + cnt_m + 1; i++) { d[i] = INF; vis[i] = 0; pre[i] = i; } d[0] = 0; queue<int>qu; qu.push(0); while (!qu.empty()) { int x = qu.front(); qu.pop(); vis[x] = 0; for (int e = 1; e <= cnt_h + cnt_m + 1; e++) { if (con[x][e]&& d[e] > d[x] + cost[x][e]) { pre[e] = x; d[e] = d[x] + cost[x][e]; if (!vis[e]) { qu.push(e); vis[e] = 1; } } } } if (d[cnt_h + cnt_m + 1] < INF) { return true; } else { return false; } } void compute() { int i; int r = INF; for (i = cnt_h + cnt_m + 1; i != 0; i = pre[i]) { r = min(r, con[pre[i]][i]); } for (i = cnt_h + cnt_m + 1; i != 0; i = pre[i]) { con[pre[i]][i] -= r; con[i][pre[i]] += r; res += cost[pre[i]][i] * r; } } void solve() { int i, j; memset(con, 0, sizeof(con)); memset(cost, 0, sizeof(cost)); for (i = 1; i <= cnt_m; i++) { for (j = 1; j <= cnt_h; j++) { con[i][cnt_m + j] = 1; cost[i][cnt_m + j] = step(i, j); cost[cnt_m + j][i] = -cost[i][cnt_m + j]; } } for (i = 1; i <= cnt_m; i++) { con[0][i] = 1; } for (j = 1; j <= cnt_h; j++) { con[cnt_m + j][cnt_m + cnt_h + 1] = 1; } res = 0; while (spfa()) { compute(); } printf("%d\n", res); } int main() { //freopen("i.txt", "r", stdin); //freopen("o.txt", "w", stdout); while (scanf("%d%d", &n, &m) != EOF) { if (n == 0 && m == 0) break; input(); solve(); } return 0; }
相关文章推荐
- zzuoj10434: good string
- Google开源框架之MNIST入门
- UVALive 6835 - Space Golf
- 检测设备是否支持Google Play服务
- 类别,类扩展的区别
- UVALive 6835 - Space Golf
- chrome64下载\google拼音输入法下载
- Category
- 初探django-写一个小游戏charade
- Django1.9学习笔记
- UVALive 6835 Space Golf-计算几何
- Go语言几大命令简单介绍
- GridView自带分页 1总页数 首页 下一页 上一页 尾页 X 页 go 实现方法 .
- Django笔记教程:四、会话、注册、以及用户
- mongorestore 恢复mongodump文件报BSONObj size: 17794400 (0x10F8560) is invalid. Size must be between 0 and
- flowplayer设置视频logo
- self,super,protocol,category,extension 的用法
- Objc-C 知识点回顾 八 NSDate、 Extension、Category、Delegate
- Gosn的使用
- FakeID签名漏洞分析及利用(Google Bug 13678484)