MyBatis入门(五)---延时加载、缓存
2015-10-31 23:51
218 查看
一、创建数据库
1.1、建立数据库
数据表和数据
二、创建项目
2.1、创建项目
三、建立实体类与Mapper接口
3.1、3个实体类package com.pb.mybatis.po; import java.util.Date; /** * * @ClassName: Author * @Description: TODO(作者) * @author 刘楠 * @date 2015-10-31 下午12:39:33 * */ public class Author { //作者id private Integer authorId; //作者姓名 private String authorUserName; //作者密码 private String authorPassword; //作者邮箱 private String authorEmail; //作者介绍 private String authroBio; //注册时间 private Date registerTime; public Integer getAuthorId() { return authorId; } public void setAuthorId(Integer authorId) { this.authorId = authorId; } public String getAuthorUserName() { return authorUserName; } public void setAuthorUserName(String authorUserName) { this.authorUserName = authorUserName; } public String getAuthorPassword() { return authorPassword; } public void setAuthorPassword(String authorPassword) { this.authorPassword = authorPassword; } public String getAuthorEmail() { return authorEmail; } public void setAuthorEmail(String authorEmail) { this.authorEmail = authorEmail; } public String getAuthroBio() { return authroBio; } public void setAuthroBio(String authroBio) { this.authroBio = authroBio; } public Date getRegisterTime() { return registerTime; } public void setRegisterTime(Date registerTime) { this.registerTime = registerTime; } @Override public String toString() { return "Author [authorId=" + authorId + ", authorUserName=" + authorUserName + ", authorPassword=" + authorPassword + ", authorEmail=" + authorEmail + ", authroBio=" + authroBio + ", registerTime=" + registerTime + "]"; } }
package com.pb.mybatis.po; import java.util.List; public class Blog { //Blog,ID private Integer blogId; //Blog 名称标题 private String blogTitle; //外键authorId //作者 private Author author; //文章 列表 private Integer authorId; //文章列表 private List<Posts> postsList; public Integer getBlogId() { return blogId; } public void setBlogId(Integer blogId) { this.blogId = blogId; } public String getBlogTitle() { return blogTitle; } public void setBlogTitle(String blogTitle) { this.blogTitle = blogTitle; } public Author getAuthor() { return author; } public void setAuthor(Author author) { this.author = author; } public Integer getAuthorId() { return authorId; } public void setAuthorId(Integer authorId) { this.authorId = authorId; } public List<Posts> getPostsList() { return postsList; } public void setPostsList(List<Posts> postsList) { this.postsList = postsList; } @Override public String toString() { return "Blog [blogId=" + blogId + ", blogTitle=" + blogTitle + ", authorId=" + authorId + "]"; } }
package com.pb.mybatis.po; import java.util.Date; public class Posts { //文章ID private Integer postId; //文章标题 private String postTitle; //文章主体内容 private String postBody; //外键 private Integer blogId; //建立时间 private Date createTime; public Integer getPostId() { return postId; } public void setPostId(Integer postId) { this.postId = postId; } public String getPostTitle() { return postTitle; } public void setPostTitle(String postTitle) { this.postTitle = postTitle; } public String getPostBody() { return postBody; } public void setPostBody(String postBody) { this.postBody = postBody; } public Date getCreateTime() { return createTime; } public void setCreateTime(Date createTime) { this.createTime = createTime; } public Integer getBlogId() { return blogId; } public void setBlogId(Integer blogId) { this.blogId = blogId; } @Override public String toString() { return "Posts [postId=" + postId + ", postTitle=" + postTitle + ", postBody=" + postBody + ", createTime=" + createTime + ", blogId=" + blogId + "]"; } }
3.2、3个接口
package com.pb.mybatis.mapper; import com.pb.mybatis.po.Author; public interface AuthorMapper { /** * * @Title: findAuthorById * @Description: TODO(根据id查找) * @param @param id * @param @return 设定文件 * @return Author 返回类型 * @throws */ public Author findAuthorById(int id); }
package com.pb.mybatis.mapper; import com.pb.mybatis.po.Blog; public interface BlogMapper { /** * * @Title: findBlogById * @Description: TODO(根据BLOGID查找BLOG,并关联author,与posts实现延时加载) * @param @param id * @param @return 设定文件 * @return Blog 返回类型 * @throws */ public Blog findBlogById(int blogId); }
package com.pb.mybatis.mapper; import java.util.List; import com.pb.mybatis.po.Posts; public interface PostsMapper { /** * * @Title: findPostsByBlogId * @Description: TODO(根据BLOGID查找文章列表) * @param @param blogId * @param @return 设定文件 * @return List<Posts> 返回类型 * @throws */ public List<Posts> findPostsByBlogId(int blogId); }
四、建立mapper.xml与confinguration.xml
4.1、相对应的mapper.xmlAuthorMapper.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.pb.mybatis.mapper.AuthorMapper"> <!--映射作者Author --> <resultMap type="Author" id="authorResultMap"> <id property="authorId" column="author_id"/> <result property="authorUserName" column="author_username"/> <result property="authorPassword" column="author_password"/> <result property="authorEmail" column="author_email"/> <result property="authroBio" column="author_bio"/> <result property="registerTime" column="register_time"/> </resultMap> <!-- 根据ID查找 --> <select id="findAuthorById" parameterType="int" resultMap="authorResultMap"> select * from author where author_id=#{id} </select> </mapper>
BlogMapper.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.pb.mybatis.mapper.BlogMapper"> <!--Blog映射 --> <resultMap type="Blog" id="blogResultMap"> <!--Blog映射 --> <id property="blogId" column="blog_id"/> <result property="blogTitle" column="blog_title"/> <result property="authorId" column="author_id"/> <!--一对一 --> <association property="author" javaType="Author" column="author_id" select="com.pb.mybatis.mapper.AuthorMapper.findAuthorById"/> <!--关联作者 外键 column:外键 select:引用Authro的namespace.方法 --> <!--一对多 ofType属性指定集合中元素的对象类型。--> <collection property="postsList" ofType="Posts" javaType="ArrayList" column="blog_id" select="com.pb.mybatis.mapper.PostsMapper.findPostsByBlogId"/> </resultMap> <!-- 根据ID查找 --> <select id="findBlogById" parameterType="int" resultMap="blogResultMap"> SELECT * FROM blog WHERE blog_id=#{blogId} </select> </mapper>
PostsMapper.xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.pb.mybatis.mapper.PostsMapper"> <!--映射作者文章 --> <resultMap type="Posts" id="postsResultMap"> <id property="postId" column="post_id"/> <result property="postTitle" column="post_subject"/> <result property="postBody" column="post_body"/> <result property="blogId" column="blog_id"/> <result property="createTime" column="createtime"/> </resultMap> <!-- 根据ID查找 --> <select id="findPostsByBlogId" parameterType="int" resultMap="postsResultMap"> select * from posts where blog_id=#{blogId} </select> </mapper>
4.2、configuration.xml
db.properties
driver=com.mysql.jdbc.Driver url=jdbc:mysql://localhost:3306/mybatis?character=utf8 username=root password=root
开启延时加载,同时关闭立即加载
<settings> <!--开启延时加载 --> <setting name="lazyLoadingEnabled" value="true"/> <!--关闭立即加载 --> <setting name="aggressiveLazyLoading" value="false"/> </settings>
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!--资源文件 -->
<properties resource="db.properties"/>
<settings> <!--开启延时加载 --> <setting name="lazyLoadingEnabled" value="true"/> <!--关闭立即加载 --> <setting name="aggressiveLazyLoading" value="false"/> </settings>
<!-- 别名 -->
<typeAliases>
<!-- <typeAlias type="com.pb.mybatis.po.User" alias="User"/> -->
<package name="com.pb.mybatis.po"/>
</typeAliases>
<!--配置 -->
<environments default="development">
<environment id="development">
<!--事务 -->
<transactionManager type="JDBC"/>
<!--数据源 -->
<dataSource type="POOLED">
<property name="driver" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
</dataSource>
</environment>
</environments>
<mappers>
<package name="com.pb.mybatis.mapper"/>
</mappers>
</configuration>
五、测试
5.1、JUNITpackage com.pb.mybatis.mapper; import java.io.InputStream; import java.util.List; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import org.junit.Before; import org.junit.Test; import com.pb.mybatis.po.Blog; import com.pb.mybatis.po.Posts; public class BlogMapperTest { private SqlSessionFactory sqlSessionFactory; @Before public void setUp() throws Exception { String config="configuration.xml"; InputStream resource=Resources.getResourceAsStream(config); sqlSessionFactory=new SqlSessionFactoryBuilder().build(resource); } /** * * @Title: testFindBlogs * @Description: TODO(延时加载) * @param 设定文件 * @return void 返回类型 * @throws */ @Test public void testFindBlogById() { //建立会话 SqlSession sqlSession=sqlSessionFactory.openSession(); //获取Mapper接口对象 BlogMapper blogMapper=sqlSession.getMapper(BlogMapper.class); //调用Mapper方法 Blog blog=blogMapper.findBlogById(1); System.out.println(blog.getBlogId()+"..."+blog.getBlogTitle()); System.out.println("===========开始延时加载作者Author==========="); System.out.println(blog.getAuthor()); System.out.println("===========开始延时加载文章==========="); List<Posts> list=blog.getPostsList(); /*int count=0; for(Posts p:list){ System.out.println("count+"+count++); System.out.println(p.getPostId()); System.out.println(p.getPostTitle()); System.out.println(p.getPostBody()); System.out.println(p.getCreateTime()); System.out.println(p.getBlogId()); }*/ } }
结果:
DEBUG [main] - ==> Preparing: SELECT * FROM blog WHERE blog_id=? DEBUG [main] - ==> Parameters: 1(Integer) DEBUG [main] - <== Total: 1 1...小张的Blog ===========开始延时加载作者Author=========== DEBUG [main] - ==> Preparing: select * from author where author_id=? DEBUG [main] - ==> Parameters: 1(Integer) DEBUG [main] - <== Total: 1 Author [authorId=1, authorUserName=张三, authorPassword=123456, authorEmail=123@qq.com, authroBio=张三是个新手,刚开始注册, registerTime=Thu Oct 29 10:23:59 CST 2015] ===========开始延时加载文章=========== DEBUG [main] - ==> Preparing: select * from posts where blog_id=? DEBUG [main] - ==> Parameters: 1(Integer) DEBUG [main] - <== Total: 2
六、一级缓存
6.1、mybatis一级缓存mybatis,默认开启了一级缓存,sqlSession中默认有一个hashMap
当查询时,先去hashMap中查找,
如果有就直接,取出,不再操作数据库
如果没有就去数据库查找,并放在haspMap中
当做事物时,如添加,删除,修改,后有commit时会清空一级缓存
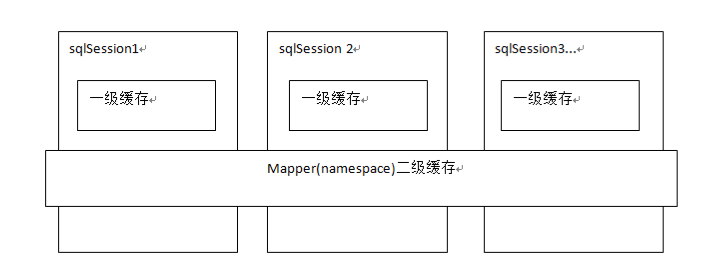
sqlSession是互不影响的,一级缓存
6.2、还是上级的例子
测试:查询用户
package com.pb.mybatis.mapper; import static org.junit.Assert.*; import java.io.InputStream; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import org.junit.Before; import org.junit.Test; import com.pb.mybatis.po.Author; public class AuthorMapperTest { private SqlSessionFactory sqlSessionFactory; @Before public void setUp() throws Exception { String config="configuration.xml"; InputStream resource=Resources.getResourceAsStream(config); sqlSessionFactory=new SqlSessionFactoryBuilder().build(resource); } @Test public void testFindAuthorById() { //获取会话工厂 SqlSession sqlSession=sqlSessionFactory.openSession(); AuthorMapper authorMapper=sqlSession.getMapper(AuthorMapper.class); //第一次查询 Author author1=authorMapper.findAuthorById(2); System.out.println(author1); //再次查询同样的 Author author2=authorMapper.findAuthorById(2); System.out.println(author2); //再次查询不的同样的 Author author3=authorMapper.findAuthorById(4); System.out.println(author3); } }
结果:
DEBUG [main] - ==> Preparing: select * from author where author_id=? DEBUG [main] - ==> Parameters: 2(Integer) DEBUG [main] - <== Total: 1 Author [authorId=2, authorUserName=李四, authorPassword=123asf, authorEmail=lisi@163.com, authroBio=魂牵梦萦 , registerTime=Thu Oct 29 10:24:29 CST 2015] Author [authorId=2, authorUserName=李四, authorPassword=123asf, authorEmail=lisi@163.com, authroBio=魂牵梦萦 , registerTime=Thu Oct 29 10:24:29 CST 2015] DEBUG [main] - ==> Preparing: select * from author where author_id=? DEBUG [main] - ==> Parameters: 4(Integer) DEBUG [main] - <== Total: 1 Author [authorId=4, authorUserName=赵六, authorPassword=123098sdfa, authorEmail=zhaoliu@qq.com, authroBio=花午骨, registerTime=Thu Oct 29 10:26:09 CST 2015]
6.3、当查询后,做事务,再查询第一次的
接口中做添加方法
/** * * @Title: addAuthor * @Description: TODO(添加) * @param @param author * @param @return 设定文件 * @return int 返回类型 * @throws */ public int addAuthor(Author author);
mapper.xml中做同样的select
<!--添加 --> <insert id="addAuthor" parameterType="Author" useGeneratedKeys="true" keyProperty="authorId"> INSERT INTO author(author_username,author_password,author_email,author_bio) VALUES(#{authorUserName},#{authorPassword},#{authorEmail},#{authroBio}) </insert>
测试
package com.pb.mybatis.mapper; import static org.junit.Assert.*; import java.io.InputStream; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import org.junit.Before; import org.junit.Test; import com.pb.mybatis.po.Author; public class AuthorMapperTest { private SqlSessionFactory sqlSessionFactory; @Before public void setUp() throws Exception { String config="configuration.xml"; InputStream resource=Resources.getResourceAsStream(config); sqlSessionFactory=new SqlSessionFactoryBuilder().build(resource); } @Test public void testFindAuthorById() { //获取会话工厂 SqlSession sqlSession=sqlSessionFactory.openSession(); AuthorMapper authorMapper=sqlSession.getMapper(AuthorMapper.class); //第一次查询 Author author1=authorMapper.findAuthorById(2); System.out.println(author1); System.out.println("=======下面有事务处理========"); Author newAuthor=new Author(); newAuthor.setAuthorEmail("qq.com@qq.com"); newAuthor.setAuthorPassword("fdsfds"); newAuthor.setAuthorUserName("郭靖"); newAuthor.setAuthroBio("射雕英雄传"); int num=authorMapper.addAuthor(newAuthor); sqlSession.commit(); System.out.println(newAuthor.getAuthorId()); System.out.println("num="+num); System.out.println("再次查询"); //再次查询同样的 Author author2=authorMapper.findAuthorById(2); System.out.println(author2); } }
结果:
DEBUG [main] - ==> Preparing: select * from author where author_id=? DEBUG [main] - ==> Parameters: 2(Integer) DEBUG [main] - <== Total: 1 Author [authorId=2, authorUserName=李四, authorPassword=123asf, authorEmail=lisi@163.com, authroBio=魂牵梦萦 , registerTime=Thu Oct 29 10:24:29 CST 2015] =======下面有事务处理======== DEBUG [main] - ==> Preparing: INSERT INTO author(author_username,author_password,author_email,author_bio) VALUES(?,?,?,?) DEBUG [main] - ==> Parameters: 郭靖(String), fdsfds(String), qq.com@qq.com(String), 射雕英雄传(String) DEBUG [main] - <== Updates: 1 DEBUG [main] - Committing JDBC Connection [com.mysql.jdbc.JDBC4Connection@42bb0406] 11 num=1 再次查询 DEBUG [main] - ==> Preparing: select * from author where author_id=? DEBUG [main] - ==> Parameters: 2(Integer) DEBUG [main] - <== Total: 1 Author [authorId=2, authorUserName=李四, authorPassword=123asf, authorEmail=lisi@163.com, authroBio=魂牵梦萦 , registerTime=Thu Oct 29 10:24:29 CST 2015]
七、二级缓存
7.1、mybatis二级缓存与一级缓存
sqlSession是互不影响的,一级缓存
Mapper(namespace)是二级缓存
多个sqlSession可以共享一个Mapper的二级缓存区域
二级缓存按namespace分,其它的mapper也有自己的二级缓存区域namespace
第一个namespace的mapper都有一个二级缓存区域,2个mapper的namespace如果相同,这2个mapper执行sql查询数据将存在相同的二级缓存区域中.
7.2、开启二级缓存
1.二级缓存是mapper范围级别的,除了在configuration.xml设置二级缓存的总开关,还在在个体的mapper.xml中开启二级缓存
<settings> <!--开启延时加载 --> <setting name="lazyLoadingEnabled" value="true"/> <!--关闭立即加载 --> <setting name="aggressiveLazyLoading" value="false"/> <!--开启二级缓存 --> <setting name="cacheEnabled" value="true" /> </settings>
2.mapper对就的pojo类,实现序列化

3.在authorMapper中开启二级缓存
<!--开启本mapper下的二级缓冲 --> <cache />
7.3、测试
@Test public void testCache() { // 获取会话工厂 SqlSession sqlSession1 = sqlSessionFactory.openSession(); AuthorMapper authorMapper1 = sqlSession1.getMapper(AuthorMapper.class); // 第一次查询 Author author1 = authorMapper1.findAuthorById(2); //必须关闭不数据无法写到缓存区域 sqlSession1.close(); // 获取会话工厂 /*SqlSession sqlSession2 = sqlSessionFactory.openSession(); AuthorMapper authorMapper2 = sqlSession2.getMapper(AuthorMapper.class); sqlSession2.close();*/ // 获取会话工厂 SqlSession sqlSession3 = sqlSessionFactory.openSession(); AuthorMapper authorMapper3 = sqlSession3.getMapper(AuthorMapper.class); // 第一次查询 Author author3 = authorMapper3.findAuthorById(2); sqlSession3.close(); }
DEBUG [main] - Cache Hit Ratio [com.pb.mybatis.mapper.AuthorMapper]: 0.0 DEBUG [main] - Opening JDBC Connection DEBUG [main] - Created connection 873769339. DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b] DEBUG [main] - ==> Preparing: select * from author where author_id=? DEBUG [main] - ==> Parameters: 2(Integer) DEBUG [main] - <== Total: 1 DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b] DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b] DEBUG [main] - Returned connection 873769339 to pool. DEBUG [main] - Cache Hit Ratio [com.pb.mybatis.mapper.AuthorMapper]: 0.5
中间增加commit操作
@Test public void testCache() { // 获取会话工厂 SqlSession sqlSession1 = sqlSessionFactory.openSession(); AuthorMapper authorMapper1 = sqlSession1.getMapper(AuthorMapper.class); // 第一次查询 Author author1 = authorMapper1.findAuthorById(2); //必须关闭不数据无法写到缓存区域 sqlSession1.close(); // 获取会话工厂 SqlSession sqlSession2 = sqlSessionFactory.openSession(); AuthorMapper authorMapper2 = sqlSession2.getMapper(AuthorMapper.class); Author author2 = authorMapper2.findAuthorById(2); //更新 author2.setAuthorUserName("董事长"); author2.setAuthroBio("公司"); authorMapper2.updateAuthor(author2); //commit会清空缓存区域 sqlSession2.commit(); sqlSession2.close(); // 获取会话工厂 SqlSession sqlSession3 = sqlSessionFactory.openSession(); AuthorMapper authorMapper3 = sqlSession3.getMapper(AuthorMapper.class); // 第一次查询 Author author3 = authorMapper3.findAuthorById(2); sqlSession3.close(); }
结果:
DEBUG [main] - Cache Hit Ratio [com.pb.mybatis.mapper.AuthorMapper]: 0.0 DEBUG [main] - Opening JDBC Connection DEBUG [main] - Created connection 873769339. DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b] DEBUG [main] - ==> Preparing: select * from author where author_id=? DEBUG [main] - ==> Parameters: 2(Integer) DEBUG [main] - <== Total: 1 DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b] DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b] DEBUG [main] - Returned connection 873769339 to pool. DEBUG [main] - Cache Hit Ratio [com.pb.mybatis.mapper.AuthorMapper]: 0.5
DEBUG [main] - Opening JDBC Connection
DEBUG [main] - Checked out connection 873769339 from pool.
DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b]
DEBUG [main] - ==> Preparing: update author SET author_username=?, author_password=?, author_email=?, author_bio=?, register_time=? where author_id=?
DEBUG [main] - ==> Parameters: 董事长(String), 123asf(String), lisi@163.com(String), 公司(String), 2015-10-29 10:24:29.0(Timestamp), 2(Integer)
DEBUG [main] - <== Updates: 1
DEBUG [main] - Committing JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b]
DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b]
DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b]
DEBUG [main] - Returned connection 873769339 to pool.
DEBUG [main] - Cache Hit Ratio [com.pb.mybatis.mapper.AuthorMapper]: 0.3333333333333333
DEBUG [main] - Opening JDBC Connection
DEBUG [main] - Checked out connection 873769339 from pool.
DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b]
DEBUG [main] - ==> Preparing: select * from author where author_id=?
DEBUG [main] - ==> Parameters: 2(Integer)
DEBUG [main] - <== Total: 1
DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b]
DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@3414a97b]
DEBUG [main] - Returned connection 873769339 to pool.
八、Mybatis与ehcache整合
8.1、把jar包导入项目
8.2、建立ehcache.xml
ehcache.xml
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../config/ehcache.xsd"> <diskStore path="F:\develop\ehcache" /> <defaultCache maxElementsInMemory="1000" maxElementsOnDisk="10000000" eternal="false" overflowToDisk="false" timeToIdleSeconds="120" timeToLiveSeconds="120" diskExpiryThreadIntervalSeconds="120" memoryStoreEvictionPolicy="LRU"> </defaultCache> </ehcache>
8.3、在mapper的cache中指定type
<!--开启本mapper下的二级缓冲 type指定为ehcachecache类开 在ehcache和mybatis的整合包中 --> <cache type="org.mybatis.caches.ehcache.EhcacheCache"/>
8.4、测试
@Test public void testCache() { // 获取会话工厂 SqlSession sqlSession1 = sqlSessionFactory.openSession(); AuthorMapper authorMapper1 = sqlSession1.getMapper(AuthorMapper.class); // 第一次查询 Author author1 = authorMapper1.findAuthorById(2); //必须关闭不数据无法写到缓存区域 sqlSession1.close(); // 获取会话工厂 SqlSession sqlSession2 = sqlSessionFactory.openSession(); AuthorMapper authorMapper2 = sqlSession2.getMapper(AuthorMapper.class); Author author2 = authorMapper2.findAuthorById(2); //更新 author2.setAuthorUserName("董事长"); author2.setAuthroBio("公司"); authorMapper2.updateAuthor(author2); //commit会清空缓存区域 sqlSession2.commit(); sqlSession2.close(); // 获取会话工厂 SqlSession sqlSession3 = sqlSessionFactory.openSession(); AuthorMapper authorMapper3 = sqlSession3.getMapper(AuthorMapper.class); // 第一次查询 Author author3 = authorMapper3.findAuthorById(2); sqlSession3.close(); }
结果:
DEBUG [main] - Configuring ehcache from ehcache.xml found in the classpath: file:/E:/mywork/MybatisDemo2/bin/ehcache.xml DEBUG [main] - Configuring ehcache from URL: file:/E:/mywork/MybatisDemo2/bin/ehcache.xml DEBUG [main] - Configuring ehcache from InputStream DEBUG [main] - Ignoring ehcache attribute xmlns:xsi DEBUG [main] - Ignoring ehcache attribute xsi:noNamespaceSchemaLocation DEBUG [main] - Disk Store Path: F:\develop\ehcache DEBUG [main] - Creating new CacheManager with default config DEBUG [main] - propertiesString is null. DEBUG [main] - No CacheManagerEventListenerFactory class specified. Skipping... DEBUG [main] - No BootstrapCacheLoaderFactory class specified. Skipping... DEBUG [main] - CacheWriter factory not configured. Skipping... DEBUG [main] - No CacheExceptionHandlerFactory class specified. Skipping... DEBUG [main] - Initialized net.sf.ehcache.store.NotifyingMemoryStore for com.pb.mybatis.mapper.AuthorMapper DEBUG [main] - Initialised cache: com.pb.mybatis.mapper.AuthorMapper DEBUG [main] - CacheDecoratorFactory not configured for defaultCache. Skipping for 'com.pb.mybatis.mapper.AuthorMapper'. DEBUG [main] - Cache Hit Ratio [com.pb.mybatis.mapper.AuthorMapper]: 0.0 DEBUG [main] - Opening JDBC Connection DEBUG [main] - Created connection 1286943672. DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - ==> Preparing: select * from author where author_id=? DEBUG [main] - ==> Parameters: 2(Integer) DEBUG [main] - <== Total: 1 DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - Returned connection 1286943672 to pool. DEBUG [main] - Cache Hit Ratio [com.pb.mybatis.mapper.AuthorMapper]: 0.5 DEBUG [main] - Opening JDBC Connection DEBUG [main] - Checked out connection 1286943672 from pool. DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - ==> Preparing: update author SET author_username=?, author_password=?, author_email=?, author_bio=?, register_time=? where author_id=? DEBUG [main] - ==> Parameters: 董事长(String), 123asf(String), lisi@163.com(String), 公司(String), 2015-10-29 10:24:29.0(Timestamp), 2(Integer) DEBUG [main] - <== Updates: 1 DEBUG [main] - Committing JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - Returned connection 1286943672 to pool. DEBUG [main] - Cache Hit Ratio [com.pb.mybatis.mapper.AuthorMapper]: 0.3333333333333333 DEBUG [main] - Opening JDBC Connection DEBUG [main] - Checked out connection 1286943672 from pool. DEBUG [main] - Setting autocommit to false on JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - ==> Preparing: select * from author where author_id=? DEBUG [main] - ==> Parameters: 2(Integer) DEBUG [main] - <== Total: 1 DEBUG [main] - Resetting autocommit to true on JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - Closing JDBC Connection [com.mysql.jdbc.JDBC4Connection@4cb533b8] DEBUG [main] - Returned connection 1286943672 to pool.
相关文章推荐
- 鸟哥的linux私房菜(RIDA摘录)
- [Django与表单]写一个简单的Django form表单处理
- html+div+css
- HDOJ5522Numbers
- CWnd::WindowProc
- 终端服务器超出了最大允许连接数
- Leap Motion C++环境的配置
- nexus repair或update index 没反应 手动配置nexus index
- 创建含ListView的Dialog遇到的问题
- 抓翻倍牛股的简易方法 缘分战法(牛股突破战法)
- 委托
- LightOJ - 1217 Neighbor House (II)(dp)
- BestCoder Round #61 HDOJ5522 5523 5524题解
- ubuntu vim
- mysql 从一个表中查数据,插入另一个表
- IntentService报Unable to instantiate service 错误的解决方法
- 15个基础的jQuery面试问题
- 第二百一十二天 how can I 坚持
- spring security使用数据库获取资源、角色和权限保护web应用
- 第四章:Django 的模板系统(转)