即使用ADO.NET,也要轻量级实体映射,比Dapper和Ormlite均快
2015-06-12 18:37
459 查看
不管出于什么原因,有时候框架人员摒弃了NH或EF,而使用原生数据库访问对象。
为了优美的编程,用上我写的轻量级映射扩展方法吧
目的:将SqlDataReader自动转换成T类型
代码如下:
在项目中再也需要reader["fieldname"]这样的惹人厌的写法了
换言之,只需要这样写:
基本原理当然少不了反射,实体的属性可以用DataField特性标记在数据表中的字段名称,否则与属性同名,字段名称不区分大小写。
DataField特性是自己写的,只有一个Name属性。
是不是很方便,虽然重复造轮子了,但是即使用了原生数据库访问对象,又轻松了转换了实体,带来的方便性可以弥补一切。
记得点【推荐】
性能测试:
与OrmLite对比:
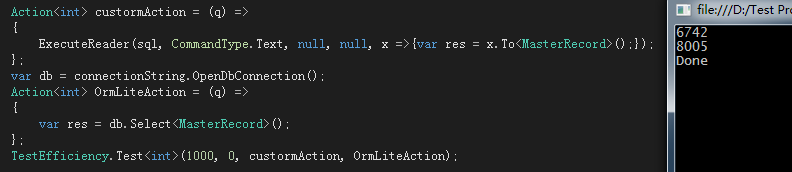
从图中看出,在测试1000次的时候,本文的扩展方法比OrmLite的方法快1263ms,测试多次效率均与该结果基本相符。
Test测试源码如下:
为了优美的编程,用上我写的轻量级映射扩展方法吧
目的:将SqlDataReader自动转换成T类型
代码如下:
/// <summary> /// 提供将SqlDataReader转成T类型的扩展方法 /// </summary> public static class SqlDataReaderEx { private static object _obj = new object(); /// <summary> /// 属性反射信息缓存 key:类型的hashCode,value属性信息 /// </summary> private static Dictionary<int, Dictionary<string, PropertyInfo>> propInfoCache = new Dictionary<int, Dictionary<string, PropertyInfo>>(); /// <summary> /// 将SqlDataReader转成T类型 /// </summary> /// <typeparam name="T"></typeparam> /// <param name="reader"></param> /// <returns></returns> public static T To<T>(this SqlDataReader reader) where T : new() { if (reader == null || reader.HasRows == false) return default(T); var res = new T(); var propInfos = GetFieldnameFromCache<T>(); for (int i = 0; i < reader.FieldCount; i++) { var n = reader.GetName(i).ToLower(); if (propInfos.ContainsKey(n)) { PropertyInfo prop = propInfos ; var IsValueType = prop.PropertyType.IsValueType; object defaultValue = null;//引用类型或可空值类型的默认值 if (IsValueType) { if ((!prop.PropertyType.IsGenericType) ||(prop.PropertyType.IsGenericType&&!prop.PropertyType.GetGenericTypeDefinition().Equals(typeof(Nullable<>)))) { defaultValue = 0;//非空值类型的默认值 } } var v = reader.GetValue(i); prop.SetValue(res, (Convert.IsDBNull(v) ? defaultValue : v), null); } } return res; } private static Dictionary<string, PropertyInfo> GetFieldnameFromCache<T>() { Dictionary<string, PropertyInfo> res = null; var hashCode = typeof(T).GetHashCode(); var filedNames = GetFieldname<T>(); lock (_obj) { if (!propInfoCache.ContainsKey(hashCode)) { propInfoCache.Add(hashCode, filedNames); } } res = propInfoCache[hashCode]; return res; } /// <summary> /// 获取一个类型的对应数据表的字段信息 /// </summary> /// <typeparam name="T"></typeparam> /// <returns></returns> private static Dictionary<string, PropertyInfo> GetFieldname<T>() { var res = new Dictionary<string, PropertyInfo>(); var props = typeof(T).GetProperties(); foreach (PropertyInfo item in props) { res.Add(item.GetFieldName(), item); } return res; } /// <summary> /// 将SqlDataReader转成List<T>类型 /// </summary> /// <typeparam name="T"></typeparam> /// <param name="reader"></param> /// <returns></returns> public static List<T> ToList<T>(this SqlDataReader reader) where T : new() { if (reader == null || reader.HasRows == false) return null; var res = new List<T>(); while (reader.Read()) { res.Add(reader.To<T>()); } return res; } /// <summary> /// 获取该属性对应到数据表中的字段名称 /// </summary> /// <param name="propInfo"></param> /// <returns></returns> public static string GetFieldName(this PropertyInfo propInfo) { var fieldname = propInfo.Name; var attr = propInfo.GetCustomAttributes(false); foreach (var a in attr) { if (a is DataFieldAttribute) { fieldname = (a as DataFieldAttribute).Name; break; } } return fieldname.ToLower(); } }
在项目中再也需要reader["fieldname"]这样的惹人厌的写法了
换言之,只需要这样写:
Model model=SqlDataReader.To<Model>();
基本原理当然少不了反射,实体的属性可以用DataField特性标记在数据表中的字段名称,否则与属性同名,字段名称不区分大小写。
DataField特性是自己写的,只有一个Name属性。
public class DataFieldAttribute : Attribute { public DataFieldAttribute() { } public DataFieldAttribute(string name) { m_name = name; } private string m_name = null; public string Name { get { return m_name; } set { m_name = value; } } }
是不是很方便,虽然重复造轮子了,但是即使用了原生数据库访问对象,又轻松了转换了实体,带来的方便性可以弥补一切。
记得点【推荐】
性能测试:
与OrmLite对比:
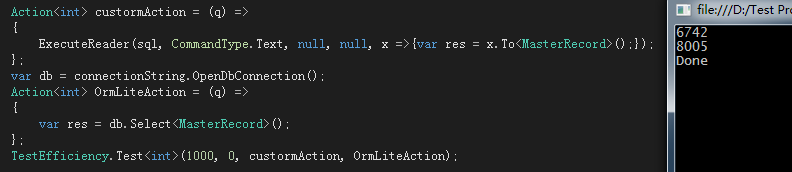
从图中看出,在测试1000次的时候,本文的扩展方法比OrmLite的方法快1263ms,测试多次效率均与该结果基本相符。
Test测试源码如下:
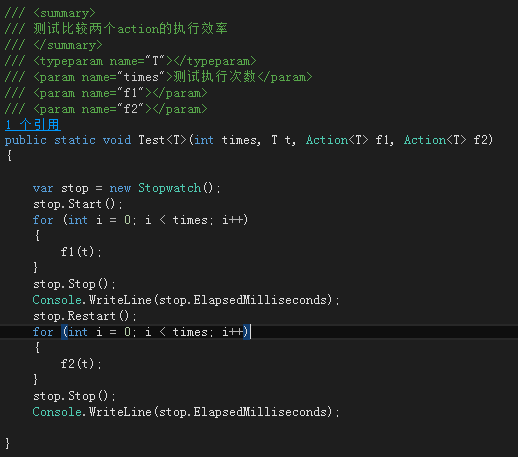
相关文章推荐
- Flex 4.6 在mxml中创建复杂Object
- 获取android项目相关信息
- Android_数据存储详解
- android 自定义progressbar 样式
- quick-cocos2dx在xcode下如何添加额外的文件夹
- quick-cocos2dx的windows版如何显示标题栏下方的菜单栏
- codeforces #549G Happy Line
- 如何使用Android MediaStore裁剪大图片
- 实现Activity的滑动返回效果
- IOS 需要学习的地方
- iBokan_ios80_自学系列_1(循环结构初练)
- 在Android studio 中配置高德地图api后显示地图遇到的问题
- IOS 调试lldb命令常用----po
- iOS scrollsToTop属性失效
- android实现程序开机自启动
- iOS 项目中用到的一些开源库和第三方组件
- Android内存泄露优化总结
- Objective_C学习笔记(2)类的使用学习概要
- iOS Code Signing 学习笔记
- Android开发者必须深入学习的10个应用开源项目