练习php小项目:简单的雇员管理系统
2014-11-24 16:31
639 查看
这是一个简单的php练习小项目,因为比较简单所以就没有用Smarty模板去把这个项目彻底的输入,处理,输出分离,只是简单的用MVC的设计模式去写的。这个项目主要是为了练习Cookie和Session的使用。
数据库用的是MySQL,建了一个empmanage的数据库,里面有两张表。如图:

表的结构如图:



这是登陆界面,因为主要想练习后台相关知识,所以前台页面写的比较简单。实际上是先写的index.php,里面就只是一个跳转。
index.php
login.php


在Main页面你可以选择管理用户,添加用户,查询用户(用户可以理解为雇员)。那么点击查询用户后会跳转到selectEmp.php去。
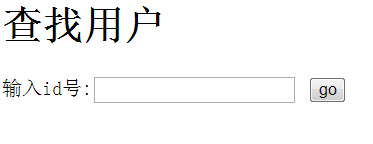
若你在Main界面点击的是添加用户,则会跳转到addEmp.php。

为了简单没有用js正则表达式去对表单中所输入的内容进行验证是否符合格式。输入完表单后会跳转到empProcess.php去验证。如果添加成功会跳转到ok.php,否则会跳转到err.php。

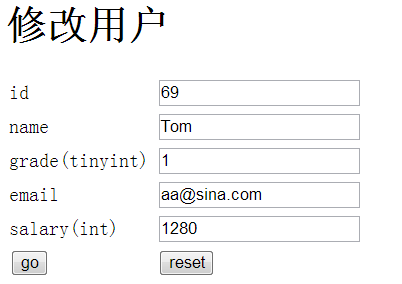
数据库用的是MySQL,建了一个empmanage的数据库,里面有两张表。如图:

表的结构如图:



这是登陆界面,因为主要想练习后台相关知识,所以前台页面写的比较简单。实际上是先写的index.php,里面就只是一个跳转。
index.php
<?php header("Location:login.php"); ?>
login.php
<html> <head> <meta http-equiv="content-type" content="text/html;charset=utf-8"> <title>emp_system</title> </head> <?php require_once 'common.php';?> <h1>emp login</h1> <form action="loginProcess.php" method="post"> 用户名<input type="text" name="username" value="<?php echo getCookie("username")?>"/><br/> 密码 <input type="password" name="password"/><br/> sava cookie<input type="checkbox" name="cookie"/><br/> <input type="submit" value="登陆"/> </form> <?php if(!empty($_GET['errNo'])){ $errNo=$_GET['errNo']; echo "<br/><font color='red'>输入的密码或用户名错误,错误信息</font>".$errNo; } ?> </html>login.php中require_once的common.php
<?php function getLastTime(){ date_default_timezone_set ( 'UTC' ); if(!empty($_COOKIE)){ echo "上次登录时间:".$_COOKIE['last_visit_time']; setcookie("last_visit_time",date("Y-m-d H:i:s"),time()+24*3600); }else{ echo "第一次登录"; setcookie("last_visit_time",date("Y-m-d H:i:s"),time()+24*3600); } } function getCookie($key){ if(!empty($_COOKIE[$key])){ return $_COOKIE[$key]; }else{ return ""; } } function checkUserLegal(){ session_start(); if(empty($_SESSION['id'])){ header("Location:login.php?errNo=1"); } } ?>login.php中表单提交到loginProcess.php去。
<?php require_once 'adminService.class.php'; if(!empty($_POST['cookie'])){ if(!empty($_POST['username'])){ $username=$_POST['username']; setcookie("username","$username",time()+300); } if(!empty($_POST['password'])){ $password=$_POST['password']; setcookie("password","$password",time()+300); } }else{ if(!empty($_POST['username'])){ $username=$_POST['username']; } if(!empty($_POST['password'])){ $password=$_POST['password']; } } $adminService=new adminService(); if($id=$adminService->checkUser($username, $password)){ //合法 session_start(); $_SESSION['id']=$id; header("Location:Main.php?id=$id"); exit(); }else{ //非法 header("Location:login.php?errNo=2"); exit(); } ?>loginProcess中require_once的adminService.class.php。
<?php require_once 'SqlHelper.class.php'; class adminService{ //验证用户是否合法 public function checkUser($username,$password){ $sqlHelper=new SqlHelper(); $sql="select password,id from admin where name='".$username."'"; $res=$sqlHelper->execute_dql($sql); if($row=mysql_fetch_assoc($res)){ if($row['password']==md5($password)){ $id=$row['id']; return $id; }else{ return null; } }else{ return null; } //释放资源 mysql_free_result($res); //关闭连接 $sqlHelper->close_connent(); } } ?>adminService.class.php中require_once的SqlHelper.class.php。
<?php class SqlHelper{ public $conn; public $dbname="empmanage"; public $host="localhost"; public $username="root"; public $password="root"; public function __construct(){ $conn=mysql_connect($this->host,$this->username,$this->password); if(!$conn){ die("connect error".mysql_errno()); } mysql_select_db($this->dbname) or die(mysql_errno()); //mysql_query("set names utf8",$this->conn); } //dql public function execute_dql($sql){ $res=mysql_query($sql) or die(mysql_error()); return $res; } //dql2 返回一格数组 可以直接关闭结果集 public function execute_dql2($sql){ $arr=array(); $i=0; $res=mysql_query($sql) or die(mysql_error()); while($row=mysql_fetch_assoc($res)){ $arr[$i++]=$row; } mysql_free_result($res); return $arr; } //分页查询 public function execute_dql_fenye($sql1,$sql2,$fenyePage){ $res=mysql_query($sql1) or die(mysql_error()); $arr=array(); while($row=mysql_fetch_assoc($res)){ $arr[]=$row; } mysql_free_result($res); $fenyePage->res_array=$arr; $res2=mysql_query($sql2) or die(mysql_error()); if($row=mysql_fetch_row($res2)){ $fenyePage->rowCount=$row[0]; $fenyePage->pageCount=ceil($row[0]/$fenyePage->pageSize); } mysql_free_result($res2); $daohangtiao=""; if($fenyePage->pageNow>1){ $prePage=$fenyePage->pageNow-1; $fenyePage->daohangtiao= "<a href='{$fenyePage->gotoUrl}?pageNow=$prePage'>上一页</a>"; } if($fenyePage->pageNow<$fenyePage->pageCount){ $nextPage=$fenyePage->pageNow+1; $fenyePage->daohangtiao.= "<a href='{$fenyePage->gotoUrl}?pageNow=$nextPage'>下一页</a>"; } //用for打印10页超链接 $start=floor(($fenyePage->pageNow-1)/10)*10+1; $index=$start; if($fenyePage->pageNow>10){ $fenyePage->daohangtiao.= " <a href='{$fenyePage->gotoUrl}?pageNow=".($start-1)."'><<</a>"; } for(;$start<$index+10;$start++){ $fenyePage->daohangtiao.= "<a href='{$fenyePage->gotoUrl}?pageNow=$start'>[$start]</a>"; } $fenyePage->daohangtiao.= " <a href='{$fenyePage->gotoUrl}?pageNow=$start'>>></a>"; $fenyePage->daohangtiao.= " 当前{$fenyePage->pageNow}页/共{$fenyePage->pageCount}页"; } //dml public function execute_dml($sql){ $res=mysql_query($sql) or die(mysql_error()); if(!$res){ return 0;//没有用户匹配 } if(mysql_affected_rows($this->conn)>0){ return 1;//ok }else{ return 2;//没有行受到影响 } } //关闭连接 public function close_connent(){ if(!empty($this->conn)){ mysql_close($this->conn); } } } ?>验证是合法用户跳转到Main.php,非法跳转到login.php并把errNo带回去并用红色输出。如图:
<html> <head> <meta http-equiv="content-type" content="text/html;charset=utf-8"> </head> <?php require_once 'common.php'; checkUserLegal(); $id=$_REQUEST['id']; echo "欢迎".$id."登陆"; // setcookie("last_visit_time","",time()-100); getLastTime(); ?> <h1>Main View</h1> <a href="empList.php">管理用户</a><br/> <a href="addEmp.php">添加用户</a><br/> <a href="selectEmp.php">查询用户</a><br/> </html>


在Main页面你可以选择管理用户,添加用户,查询用户(用户可以理解为雇员)。那么点击查询用户后会跳转到selectEmp.php去。
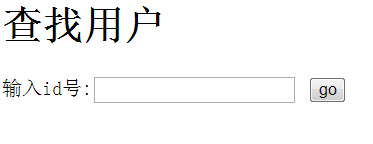
<html> <head> <meta http-equiv="content-type" content="text/html;charset=utf-8"> </head> <h1>查找用户</h1> <form action="empProcess.php" method="post"> 输入id号:<input type="text" name="id"/> <input type="hidden" name="flag" value="selectEmp"/> <input type="submit" value="go"/> </form> </html>在selectEmp.php中的表单会跳转到empProcess.php去。
<?php require_once 'empService.class.php'; require_once 'emp.class.php'; $empService=new empService(); if(!empty($_REQUEST['flag'])){ if($_REQUEST['flag']=="del"){ $id=$_REQUEST['id']; if($empService->deleteById($id)==1){ header("Location: ok.php"); exit(); }else{ header("Location: err.php"); exit(); } }else if($_REQUEST['flag']=="addEmp"){ $name=$_REQUEST['name']; $grade=$_REQUEST['grade']; $email=$_REQUEST['email']; $salary=$_REQUEST['salary']; if($empService->addEmp($name, $grade, $email, $salary)==1){ header("Location: ok.php"); exit(); }else{ header("Location: err.php"); exit(); } }else if($_REQUEST['flag']=="selectEmp"){ $id=$_REQUEST['id']; if($res=$empService->selectEmp($id)){ echo "id{$res[0]['id']} name{$res[0]['name']} grade{$res[0]['grade']} email{$res[0]['email']} salary{$res[0]['salary']}"; } }else if($_REQUEST['flag']=="updataEmp"){ $id=$_REQUEST['id']; $name=$_REQUEST['name']; $grade=$_REQUEST['grade']; $email=$_REQUEST['email']; $salary=$_REQUEST['salary']; if($empService->updataEmp($id, $name, $grade, $email, $salary)==1){ header("Location: ok.php"); exit(); }else{ header("Location: err.php"); exit(); } } } ?>这其中require_once的empService.class.php和emp.class.php。
<?php require_once 'SqlHelper.class.php'; class empService{ //计算出$PageCount public function getPageCount($pageSize){ $sqlHelper=new SqlHelper(); $sql="select count(id) from emp"; $res=$sqlHelper->execute_dql($sql); if($row=mysql_fetch_row($res)){ $rowCount=$row[0]; } $pageCount=ceil($rowCount/$pageSize); mysql_free_result($res); $sqlHelper->close_connent(); return $pageCount; } //取出结果集 public function getRes($pageNow,$pageSize){ $sqlHelper=new SqlHelper(); $sql="select id,name,grade,email,salary from emp limit ".($pageNow-1)*$pageSize.",$pageSize"; $res=$sqlHelper->execute_dql2($sql); $sqlHelper->close_connent(); return $res; } //分页 public function getFenyePage($fenyePage){ $sqlHelper=new SqlHelper(); $sql1="select id,name,grade,email,salary from emp limit ".($fenyePage->pageNow-1)*$fenyePage->pageSize.",$fenyePage->pageSize"; $sql2="select count(id) from emp"; $sqlHelper->execute_dql_fenye($sql1, $sql2, $fenyePage); $sqlHelper->close_connent(); } //删除用户 public function deleteById($id){ $sqlHelper=new SqlHelper(); $sql="delete from emp where id=$id"; return $sqlHelper->execute_dml($sql) or die(mysql_error()); $sqlHelper->close_connent(); } //添加用户 public function addEmp($name,$grade,$email,$salary){ $sqlHelper=new SqlHelper(); $sql="insert into emp(name,grade,email,salary) values ('$name','$grade','$email','$salary')"; return $sqlHelper->execute_dml($sql) or die(mysql_error()); $sqlHelper->close_connent(); } //查询用户 public function selectEmp($id){ $sqlHelper=new SqlHelper(); $sql="select * from emp where id=$id"; $res=$sqlHelper->execute_dql2($sql) or die(mysql_error()); $sqlHelper->close_connent(); return $res; } //修改用户 public function updataEmp($id,$name,$grade,$email,$salary){ $sqlHelper=new SqlHelper(); $sql="update emp set name='$name',grade=$grade,email='$email',salary=$salary where id=$id"; return $sqlHelper->execute_dml($sql) or die(mysql_error()); $sqlHelper->close_connent(); } } ?>
<?php class emp{ public $res_arr_emp; } ?>验证后若该用户存在输出该用户的详细信息,如id name grade email salary。这是查询,因为做的比较简单,所以没有写个超链接让它返回上一层。
若你在Main界面点击的是添加用户,则会跳转到addEmp.php。
<html> <head> <meta http-equiv="content-type" content="text/html;charset=utf-8"> </head> <h1>添加用户</h1> <form action="empProcess.php" method="post"> <table> <tr><td>name<td><td><input type="text" name="name"/><td></tr> <tr><td>grade(tinyint)<td><td><input type="text" name="grade"/><td></tr> <tr><td>email<td><td><input type="text" name="email"/><td></tr> <tr><td>salary(int)<td><td><input type="text" name="salary"/><td></tr> <tr><td><input type="submit" value="go"><td><td><input type="reset" value="reset"/><td></tr> <tr><input type="hidden" name="flag" value="addEmp"></tr> </table> </form> </html>

为了简单没有用js正则表达式去对表单中所输入的内容进行验证是否符合格式。输入完表单后会跳转到empProcess.php去验证。如果添加成功会跳转到ok.php,否则会跳转到err.php。
<?php header("Content-Type:text/html;charset=utf-8"); echo "操作成功"; echo "<a href=empList.php>返回上一级<a>"; ?>
<?php header("Content-Type:text/html;charset=utf-8"); echo "操作失败<br/>"; echo "<a href=empList.php>返回上一级<a>"; ?>若在Main页面点击管理用户则会跳转到empList.php。

<html> <head> <meta http-equiv="content-type" content="text/html;charset=utf-8"> </head> <?php require_once 'empService.class.php'; require_once 'FenyePage.class.php'; require_once 'common.php'; checkUserLegal(); $fenyePage=new FenyePage(); //分页 $fenyePage->pageNow=1;//显示第几页 用户输入 不停变化 if(!empty($_GET['pageNow'])){ $fenyePage->pageNow=$_GET['pageNow']; } $fenyePage->pageCount=0;//共几页 需要$rowCount计算 $fenyePage->pageSize=5;//一页显示多少条记录 $fenyePage->rowCount=0;//数据库中获取 共有几条记录 $fenyePage->gotoUrl="empList.php";//要跳转的页面 $empService=new empService(); $empService->getFenyePage($fenyePage); echo "<table border='1px' >"; echo "<tr><th>id</th><th>name</th><th>grade</th><th>email</th><th>salary</th><th><a href='#'>删除用户</a></th><th><a href='#'>修改用户</a></th></tr>"; for($i=0;$i<count($fenyePage->res_array);$i++){ $row=$fenyePage->res_array[$i]; echo "<tr><td>{$row['id']}</td><td>{$row['name']}</td><td>{$row['grade']}</td><td>{$row['email']}</td><td>{$row['salary']}</td><td><a href='empProcess.php?flag=del&id={$row['id']}'>删除用户</a></td><td><a href='updataEmp.php?flag=updataEmp&id={$row['id']}&name={$row['name']}&grade={$row['grade']}&email={$row['email']}&salary={$row['salary']}'>修改用户</a></td></tr>"; } echo "<table>"; //打印导航条 echo $fenyePage->daohangtiao; ?> </html>其中require_once的FenyePage.class.php。
<?php class FenyePage{ public $pageNow=1;//默认打开时第一页 public $pageSize; public $pageCount; public $rowCount; public $res_array; public $daohangtiao;//导航条 public $gotoUrl;//要跳转的页面 } ?>点击修改用户会跳转到updataEmp.php。
<html> <head> <meta http-equiv="content-type" content="text/html;charset=utf-8"> </head> <h1>修改用户</h1> <?php if($_REQUEST['flag']=="updataEmp"){ $id=$_REQUEST['id']; $name=$_REQUEST['name']; $grade=$_REQUEST['grade']; $email=$_REQUEST['email']; $salary=$_REQUEST['salary']; } ?> <form action="empProcess.php" method="post"> <table> <tr><td>id<td><td><input readonly="readonly" type="text" name="id" value="<?php echo $id;?>"/><td></tr> <tr><td>name<td><td><input type="text" name="name" value="<?php echo $name;?>"/><td></tr> <tr><td>grade(tinyint)<td><td><input type="text" name="grade" value="<?php echo $grade;?>"/><td></tr> <tr><td>email<td><td><input type="text" name="email" value="<?php echo $email;?>"/><td></tr> <tr><td>salary(int)<td><td><input type="text" name="salary" value="<?php echo $salary;?>"/><td></tr> <tr><td><input type="submit" value="go"><td><td><input type="reset" value="reset"/><td></tr> <tr><input type="hidden" name="flag" value="updataEmp"></tr> </table> </form> </html>
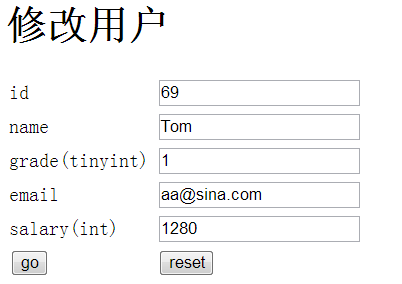
相关文章推荐
- [php笔记]项目开发五个阶段/雇员管理系统
- 01-php项目之雇员管理系统1-实现登录功能
- 通过JAVA编写一个简单的雇员管理系统小项目
- 简单的雇员管理系统
- struts2+hibernate4+spring3+maven搭建项目:简单账务管理系统(从头至尾,不断更新)
- 06-php雇员管理系统-实现显示雇员信息列表分页改进(可以处理大数据100000条)
- php--实现一个简单的学生管理系统
- 一点一点做网站开发--基于KIS的数据库项目管理(PHP系统二次开发) 上
- 07-php雇员管理系统-分层模式实现登录,分页
- 03-php雇员管理系统-实现显示用户名称
- 08-php雇员管理系统-分层模式实现通用分页
- struts2+hibernate4+spring3+maven搭建项目:简单账务管理系统第四篇:action层和jsp表现层
- 简单工程项目管理系统
- 项目一 简单的信息管理系统
- 一个极其简单的CMS(内容管理系统)PHP+MySQL技术解析
- PHP自学之路---雇员管理系统(1)
- PHP自学之路---雇员管理系统(1)
- php之用户管理系统的实现!(从简单到复杂)
- PHP一个最简单的CMS内容管理系统
- 05-php雇员管理系统-实现显示雇员信息列表分页