PHP单例模式
2014-08-12 20:54
239 查看
单例模式三大原则:(单例就是一个类,只能拥有一个实例!)
1.构造函数需要标记为非public[只能声明成私有模式private](防止外部使用new操作符创建对象),单例类不能在其他类中实例化,只能被其自身实例化;
2.拥有一个保存类的实例的静态成员变量$_instance;
3.拥有一个访问这个实例的公共的静态方法
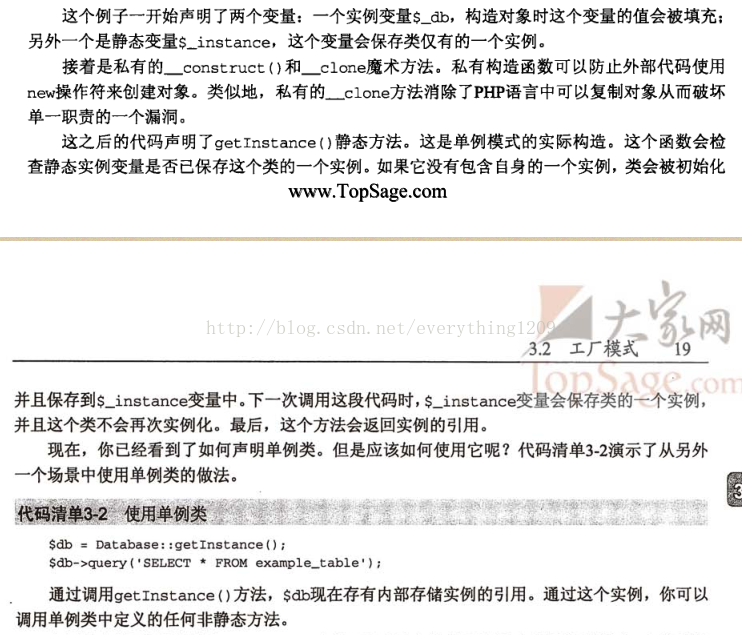
1.构造函数需要标记为非public[只能声明成私有模式private](防止外部使用new操作符创建对象),单例类不能在其他类中实例化,只能被其自身实例化;
2.拥有一个保存类的实例的静态成员变量$_instance;
3.拥有一个访问这个实例的公共的静态方法
<?php class single_model{ private $_db; static $_instance; private function __construct(){ $this ->_db = mysql_connect('127.0.0.1','root','root') or die(mysql_error()); } private function __clone(){} public static function get_instance(){ if(!self::$_instance instanceof self){ self::$_instance = new self(); } return self::$_instance; } public function query($sql){ return mysql_query($sql); } public function test(){ echo 11; } } $res = single_model::get_instance()->test();
<?php class singleton { private static $instances; public function __construct() { $c = get_class($this); if(isset(self::$instances[$c])) { throw new Exception('You can not create more than one copy of a singleton.'); } else { self::$instances[$c] = $this; } } public function get_instance() { $c = get_called_class(); if (!isset(self::$instances[$c])) { $args = func_get_args(); $reflection_object = new ReflectionClass($c); self::$instances[$c] = $reflection_object->newInstanceArgs($args); } return self::$instances[$c]; } public function __clone() { throw new Exception('You can not clone a singleton.'); } } class singleton_test extends singleton { } $o = singleton_test::get_instance(); print get_class($o);
<?php /** +----------------------------------------- * 四脚猫每日一题-用PHP实现一个单例模式 +----------------------------------------- * @description 完美的单例模式???? * @author 诺天 thinkercode@sina.com * @date 2014年5月7日 +----------------------------------------- */ class singleton{ public static $instance = null; /** * 对象初始化方法,防止继承覆盖和new该类 * @access private * @return void */ final private function __construct(){} /** * 获取对象实例 * @access public * @return object */ static public function getInstances(){ if(!(self::$instances instanceof self)){ self::$instance = new self; } return self::$instance ; } /** * 让克隆也实现单例 * @access public * @return void */ final public function __clone(){ return self::$instance ; } /** * 让克隆不能使用 * @access private * @return void */ //final private function __clone(){} }
相关文章推荐
- 一种PHP设计模式:DPT
- PHP单子模式(SINGLETON)的简单实现
- 创造世界上最简单的 PHP 开发模式第1/5页
- 五种常见的PHP设计模式
- php实现的单例模式
- 五种常见的PHP设计模式(择自互联网非本人所写)
- smarty+adodb+部分自定义类的php开发模式
- PHP试写装饰模式(Java、C#亦同)
- PHP 中的 XML 拉模式解析
- 创造世界上最简单的------php开发模式
- [介绍]PHP设计模式:DAO(数据访问对象模式)
- [转]五种常见的PHP设计模式
- 如何使用动态共享对象的模式来安装PHP
- smarty+adodb+部分自定义类的php开发模式
- 创造世界上最简单的 PHP 开发模式
- 一种PHP设计模式:DPT
- UPDATE注射(mysql+php)的两个模式
- 五种常见的PHP设计模式
- php的开发模式—基础篇