统计二叉树每层节点个数并打印每层节点
2014-08-02 10:36
253 查看
先看百度一道面试题:
有一颗二叉树,定义树的高度为从根到叶子节点的最长距离,树的宽度为每层节点的最大值,树的面积定义为高度和宽度的乘积。写一个函数计算一个二叉树的面积。(15分)
解决这道题参考资料剑指offer和编程之美,分别求求二叉树高度和宽度。
都是采用递归:
求高度参考:http://blog.csdn.net/buyingfei8888/article/details/38345591
求每层节点个数需要先求出高度
代码:
#include <iostream>
using namespace std;
typedef struct node{
int data;
struct node *lchild;
struct node *rchild;
}Node ,*pNode;
void createTree(pNode & root){
int temp;
scanf("%d",&temp);
if(0 != temp){
root=(pNode) malloc (sizeof(Node));
root->data = temp;
createTree(root->lchild);
createTree(root->rchild);
}else{
root=NULL;
}
}
void printAll(const pNode root){
pNode p = root;
if(p){
cout<< p->data<< " ";
printAll(p->lchild);
printAll(p->rchild);
}
}
//打印一层节点
int printNodeAtLevel(pNode root,int level,int &count){
if(!root || level <0)
return 0;
if(0 == level){
cout<< root->data<< " ";
count++;
return 1;
}
return printNodeAtLevel(root->lchild,level-1,count) + printNodeAtLevel(root->rchild,level-1,count);
}
//类似后序遍历,效率比较高,不会重复访问节点
void btDepth(const pNode root,int &depth){
if(NULL == root){
depth=0;
return ;
}
int left ;
btDepth(root->lchild,left);
int right ;
btDepth(root->rchild,right);
depth = left > right ? left +1 : right +1;
}
int main(){
pNode root=NULL;
createTree(root);
cout<<endl;
printAll(root);
cout<<endl;
int depth=0;
btDepth(root,depth);
cout<<endl<<depth;
cout<<endl;
//循环遍历 ,打印各层几点
for(int i=0;i<depth;i++){
int count=0;
printNodeAtLevel(root,i,count);
cout << " 节点个数为:"<< count<<endl;
}
return 0;
}
运行结果:
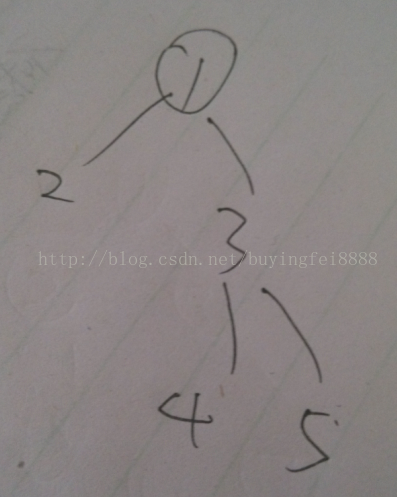
有一颗二叉树,定义树的高度为从根到叶子节点的最长距离,树的宽度为每层节点的最大值,树的面积定义为高度和宽度的乘积。写一个函数计算一个二叉树的面积。(15分)
解决这道题参考资料剑指offer和编程之美,分别求求二叉树高度和宽度。
都是采用递归:
求高度参考:http://blog.csdn.net/buyingfei8888/article/details/38345591
求每层节点个数需要先求出高度
代码:
#include <iostream>
using namespace std;
typedef struct node{
int data;
struct node *lchild;
struct node *rchild;
}Node ,*pNode;
void createTree(pNode & root){
int temp;
scanf("%d",&temp);
if(0 != temp){
root=(pNode) malloc (sizeof(Node));
root->data = temp;
createTree(root->lchild);
createTree(root->rchild);
}else{
root=NULL;
}
}
void printAll(const pNode root){
pNode p = root;
if(p){
cout<< p->data<< " ";
printAll(p->lchild);
printAll(p->rchild);
}
}
//打印一层节点
int printNodeAtLevel(pNode root,int level,int &count){
if(!root || level <0)
return 0;
if(0 == level){
cout<< root->data<< " ";
count++;
return 1;
}
return printNodeAtLevel(root->lchild,level-1,count) + printNodeAtLevel(root->rchild,level-1,count);
}
//类似后序遍历,效率比较高,不会重复访问节点
void btDepth(const pNode root,int &depth){
if(NULL == root){
depth=0;
return ;
}
int left ;
btDepth(root->lchild,left);
int right ;
btDepth(root->rchild,right);
depth = left > right ? left +1 : right +1;
}
int main(){
pNode root=NULL;
createTree(root);
cout<<endl;
printAll(root);
cout<<endl;
int depth=0;
btDepth(root,depth);
cout<<endl<<depth;
cout<<endl;
//循环遍历 ,打印各层几点
for(int i=0;i<depth;i++){
int count=0;
printNodeAtLevel(root,i,count);
cout << " 节点个数为:"<< count<<endl;
}
return 0;
}
运行结果:
相关文章推荐
- 打印二叉树两个叶子节点间的路径
- 按层打印二叉树的节点【层次遍历变形】
- 统计一个二叉树的每一层 节点个数
- 数据结构复习之二叉树:遍历、搜索节点&路径、查找、与单链表互转、逐层打印
- 打印二叉树的叶子节点
- 打印二叉树中距离根节点为k的所有节点
- 从上往下打印出二叉树的每个节点,同层节点从左至右打印。 java
- (树的层序遍历)从上往下打印出二叉树的每个节点,同层节点从左至右打印。
- 打印二叉树中一个节点的所有祖先节点
- 打印二叉树中某个节点的所有父节点
- [leetcode-二叉树层次遍历并统计每层节点数]--103. Binary Tree Zigzag Level Order Traversal
- 2013-03-17---二叉树递归,非递归实现(附代码)深度,叶子节点数量,逐行打印二叉树
- 打印二叉树的边界节点-代码指南
- 统计二叉树中叶子节点的数目
- 二叉树问题---打印二叉树的边界节点
- 打印二叉树中节点的所有祖先
- 从上往下打印出二叉树的每个节点,同层节点从左至右打印。
- 在二叉树中打印出从某个节点(r)开始和为定值(sum)的所有路径
- 从上往下打印出二叉树的每个节点,同层节点从左至右打印。
- 打印二叉树中第m层第k个节点(递归+非递归)