CodeForce Round 257 div2
2014-07-26 05:13
423 查看
官方题解 :http://codeforces.com/blog/entry/13112
A. Jzzhu and Children
time limit per test
1 second
memory limit per test
256 megabytes
input
standard input
output
standard output
There are n children in Jzzhu's school. Jzzhu is going to give some candies to them. Let's number all the children from 1 to n.
The i-th child wants to get at least ai candies.
Jzzhu asks children to line up. Initially, the i-th child stands at the i-th
place of the line. Then Jzzhu start distribution of the candies. He follows the algorithm:
Give m candies to the first child of the line.
If this child still haven't got enough candies, then the child goes to the end of the line, else the child go home.
Repeat the first two steps while the line is not empty.
Consider all the children in the order they go home. Jzzhu wants to know, which child will be the last in this order?
Input
The first line contains two integers n, m (1 ≤ n ≤ 100; 1 ≤ m ≤ 100).
The second line contains n integers a1, a2, ..., an (1 ≤ ai ≤ 100).
Output
Output a single integer, representing the number of the last child.
Sample test(s)
input
output
input
output
Note
Let's consider the first sample.
Firstly child 1 gets 2 candies and go home. Then child 2 gets 2 candies and go to the end of the line. Currently the line looks like [3, 4, 5, 2] (indices of the children in order of the line). Then child 3 gets 2 candies and go home, and then child 4 gets
2 candies and goes to the end of the line. Currently the line looks like [5, 2, 4]. Then child 5 gets 2 candies and goes home. Then child 2 gets two candies and goes home, and finally child 4 gets 2 candies and goes home.
Child 4 is the last one who goes home.
题意:n个小朋友, 每个小朋友需要a[i]块糖果,我们挨着顺序每次给一个人m块,如果m>=a[i],这个小朋友就可以走了,否则的话就排在队列的最后面,问最后一个走的是哪个小朋友。
其实就是求ceil(ai / m)最最大最靠后的那个。
可以这么写 x = (a[i] + m - 1 ) / m。
B. Jzzhu and Sequences
time limit per test
1 second
memory limit per test
256 megabytes
input
standard input
output
standard output
Jzzhu has invented a kind of sequences, they meet the following property:

You are given x and y, please calculate fn modulo 1000000007 (109 + 7).
Input
The first line contains two integers x and y (|x|, |y| ≤ 109).
The second line contains a single integer n (1 ≤ n ≤ 2·109).
Output
Output a single integer representing fn modulo 1000000007 (109 + 7).
Sample test(s)
input
output
input
output
Note
In the first sample, f2 = f1 + f3, 3 = 2 + f3, f3 = 1.
In the second sample, f2 = - 1; - 1 modulo (109 + 7) equals (109 + 6).
题意就是一个递推式,让求模后输出。
我用矩阵写了,其实这题很容易发现周期是6.
C. Jzzhu and Chocolate
time limit per test
1 second
memory limit per test
256 megabytes
input
standard input
output
standard output
Jzzhu has a big rectangular chocolate bar that consists of n × m unit squares. He wants to cut this bar exactly k times.
Each cut must meet the following requirements:
each cut should be straight (horizontal or vertical);
each cut should go along edges of unit squares (it is prohibited to divide any unit chocolate square with cut);
each cut should go inside the whole chocolate bar, and all cuts must be distinct.
The picture below shows a possible way to cut a 5 × 6 chocolate for 5 times.
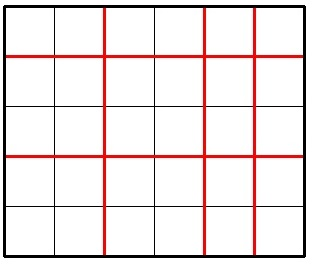
Imagine Jzzhu have made k cuts and the big chocolate is splitted into several pieces. Consider the smallest (by area) piece of the chocolate, Jzzhu wants
this piece to be as large as possible. What is the maximum possible area of smallest piece he can get with exactlyk cuts? The area of a chocolate piece
is the number of unit squares in it.
Input
A single line contains three integers n, m, k (1 ≤ n, m ≤ 109; 1 ≤ k ≤ 2·109).
Output
Output a single integer representing the answer. If it is impossible to cut the big chocolate k times, print -1.
Sample test(s)
input
output
input
output
input
output
Note
In the first sample, Jzzhu can cut the chocolate following the picture below:
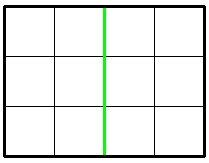
In the second sample the optimal division looks like this:
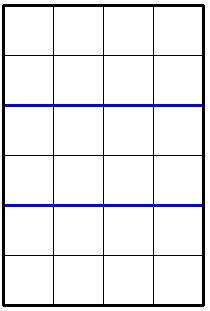
In the third sample, it's impossible to cut a 2 × 3 chocolate 4 times.
题意:给定一个m×n的格子,让切k刀,问最后得到的最小的那块面积最大是多少。 不够切k刀的话输出-1.
We assume that n ≤ m (if n > m,
we can simply swap n and m).
If we finally cut the chocolate into x rows and y columns (1 ≤ x ≤ n, 1 ≤ y ≤ m, x + y = k + 2),
we should maximize the narrowest row and maximize the narrowest column, so the answer will be floor(n / x) * floor(m / y).
There are two algorithms to find the optimal (x, y).
Notice that if x * y is smaller, the answer usually will be better. Then we can find that if k < n,
the optimal (x, y) can only be {x = 1, y = k + 1} or {x = k + 1, y = 1}.
If n ≤ k < m, the optimal (x, y) can
only be {x = 1, y = k + 1}. If m ≤ k ≤ n + m - 2,
the optimal (x, y) can only be {x = k + 2 - m, y = m},
because let t = m - n, n / (k + 2 - m) ≥ (n + t) / (k + 2 - m + t) ≥ 1.
floor(n / x) has at most

values,
so we can enum it and choose the maximum x for each value.
贪心:先把横向或纵向切完,再切另外一个。至于先切横向还是纵向,取最大值。
D. Jzzhu and Cities
time limit per test
2 seconds
memory limit per test
256 megabytes
input
standard input
output
standard output
Jzzhu is the president of country A. There are n cities numbered from 1 to n in
his country. City 1 is the capital of A. Also there are mroads
connecting the cities. One can go from city ui to vi (and
vise versa) using the i-th road, the length of this road is xi.
Finally, there are k train routes in the country. One can use the i-th
train route to go from capital of the country to city si (and
vise versa), the length of this route is yi.
Jzzhu doesn't want to waste the money of the country, so he is going to close some of the train routes. Please tell Jzzhu the maximum number of the train routes which can be closed under the following condition: the length of the shortest path from every city
to the capital mustn't change.
Input
The first line contains three integers n, m, k (2 ≤ n ≤ 105; 1 ≤ m ≤ 3·105; 1 ≤ k ≤ 105).
Each of the next m lines contains three integers ui, vi, xi (1 ≤ ui, vi ≤ n; ui ≠ vi; 1 ≤ xi ≤ 109).
Each of the next k lines contains two integers si and yi (2 ≤ si ≤ n; 1 ≤ yi ≤ 109).
It is guaranteed that there is at least one way from every city to the capital. Note, that there can be multiple roads between two cities. Also, there can be multiple routes going to the same city from the capital.
Output
Output a single integer representing the maximum number of the train routes which can be closed.
Sample test(s)
input
output
input
output
题意:n个城市,其中1号城市是首都,m条双向道路,给定长度。k条双向火车,是直接在某个城市和首都之间往返。
可能删除一些火车路之后,使得所有城市到首都的最短长度不变,问最多可以删除多少个。
题目保证肯定每个城市可以到首都,两个城市之间可能有多条路。
E. Jzzhu and Apples
time limit per test
1 second
memory limit per test
256 megabytes
input
standard input
output
standard output
Jzzhu has picked n apples from his big apple tree. All the apples are numbered from 1 to n.
Now he wants to sell them to an apple store.
Jzzhu will pack his apples into groups and then sell them. Each group must contain two apples, and the greatest common divisor of numbers of the apples in each group must be greater than 1. Of course, each apple can be part of at most one group.
Jzzhu wonders how to get the maximum possible number of groups. Can you help him?
Input
A single integer n (1 ≤ n ≤ 105),
the number of the apples.
Output
The first line must contain a single integer m, representing the maximum number of groups he can get. Each of the next m lines
must contain two integers — the numbers of apples in the current group.
If there are several optimal answers you can print any of them.
Sample test(s)
input
output
input
output
input
output
题意:给1-N这N个数,问能最多分多少组。 其中每组两个数,这两个数的gcd必须大于1.
输出组数,及怎么分组。(任意一种就行)
官方题解:注意到1和大于N/2的素数是不可能被分组的,直接无视。 然后对于 2 < p <= N/2的素数p, 我们考虑所有未匹配的且有因子p的数的个数,若为偶数个(包含p),正好匹配,否则,我们匹配除了2*p之外的这偶数个。
最后剩下的数都是偶数,两个两个匹配。
和官方题解,没有判断素数也能ac稍有不同。
A. Jzzhu and Children
time limit per test
1 second
memory limit per test
256 megabytes
input
standard input
output
standard output
There are n children in Jzzhu's school. Jzzhu is going to give some candies to them. Let's number all the children from 1 to n.
The i-th child wants to get at least ai candies.
Jzzhu asks children to line up. Initially, the i-th child stands at the i-th
place of the line. Then Jzzhu start distribution of the candies. He follows the algorithm:
Give m candies to the first child of the line.
If this child still haven't got enough candies, then the child goes to the end of the line, else the child go home.
Repeat the first two steps while the line is not empty.
Consider all the children in the order they go home. Jzzhu wants to know, which child will be the last in this order?
Input
The first line contains two integers n, m (1 ≤ n ≤ 100; 1 ≤ m ≤ 100).
The second line contains n integers a1, a2, ..., an (1 ≤ ai ≤ 100).
Output
Output a single integer, representing the number of the last child.
Sample test(s)
input
5 2 1 3 1 4 2
output
4
input
6 41 1 2 2 3 3
output
6
Note
Let's consider the first sample.
Firstly child 1 gets 2 candies and go home. Then child 2 gets 2 candies and go to the end of the line. Currently the line looks like [3, 4, 5, 2] (indices of the children in order of the line). Then child 3 gets 2 candies and go home, and then child 4 gets
2 candies and goes to the end of the line. Currently the line looks like [5, 2, 4]. Then child 5 gets 2 candies and goes home. Then child 2 gets two candies and goes home, and finally child 4 gets 2 candies and goes home.
Child 4 is the last one who goes home.
题意:n个小朋友, 每个小朋友需要a[i]块糖果,我们挨着顺序每次给一个人m块,如果m>=a[i],这个小朋友就可以走了,否则的话就排在队列的最后面,问最后一个走的是哪个小朋友。
其实就是求ceil(ai / m)最最大最靠后的那个。
可以这么写 x = (a[i] + m - 1 ) / m。
#include <iostream> using namespace std; /* CF_Round257_A dezhonger */ int main() { int n, m, a[110]; while(cin >> n >> m) { int x = -1, ans; for(int i = 0;i < n; i++ ) { cin >> a[i]; int t; if(a[i] % m == 0) t = a[i] / m; else t = a[i] / m + 1; if( t >= x ) { x = t; ans = i; } } cout << ans + 1 << endl; } return 0; }
B. Jzzhu and Sequences
time limit per test
1 second
memory limit per test
256 megabytes
input
standard input
output
standard output
Jzzhu has invented a kind of sequences, they meet the following property:

You are given x and y, please calculate fn modulo 1000000007 (109 + 7).
Input
The first line contains two integers x and y (|x|, |y| ≤ 109).
The second line contains a single integer n (1 ≤ n ≤ 2·109).
Output
Output a single integer representing fn modulo 1000000007 (109 + 7).
Sample test(s)
input
2 3 3
output
1
input
0 -12
output
1000000006
Note
In the first sample, f2 = f1 + f3, 3 = 2 + f3, f3 = 1.
In the second sample, f2 = - 1; - 1 modulo (109 + 7) equals (109 + 6).
题意就是一个递推式,让求模后输出。
我用矩阵写了,其实这题很容易发现周期是6.
#include <iostream> using namespace std; /* CF_Round257_B dezhonger */ #define MOD 1000000007 struct M { int a[2][2]; M() { a[0][0] = 1; a[0][1] = -1; a[1][0] = 1; a[1][1] = 0; } void danwei() { a[0][0] = a[1][1] = 1; a[1][0] = a[0][1] = 0; } M operator *(M b) { M aa; int i, j, k; for(i = 0; i < 2; i++ ) { for(j = 0; j < 2; j++ ) { aa.a[i][j] = 0; for(k = 0; k < 2; k++ ) { aa.a[i][j] += a[i][k] * b.a[k][j]; } } } return aa; } void print() { cout << a[0][0] << " " << a[0][1] << endl; cout << a[1][0] << " " << a[1][1] << endl; } }; int mod(int x) { while( x < 0) { x += MOD; } return x % MOD; } M cal(int p) { M ret; M a; // a.print(); if(p == 1) return ret; ret.danwei(); while(p) { if(p & 1) ret = ret * a; // a.print(); a = a * a; p /= 2; } return ret; } int main() { int x, y, n; while( cin >> x >> y) { cin >> n; if(n == 1) cout << mod(x) << endl; else { M ans = cal(n - 1); // cout << "ans" << endl; // ans.print(); int tt = ans.a[1][0] * y + ans.a[1][1] * x; // cout << tt << endl; cout << mod (tt) << endl; } } return 0; }
#include<iostream> using namespace std; int main() { int x,y,n,m=(int)(1e9+7); cin>>x>>y>>n; int ans[]={x-y,x,y,y-x,-x,-y}; cout<<((ans[n%6]%m)+m)%m<<endl; }
C. Jzzhu and Chocolate
time limit per test
1 second
memory limit per test
256 megabytes
input
standard input
output
standard output
Jzzhu has a big rectangular chocolate bar that consists of n × m unit squares. He wants to cut this bar exactly k times.
Each cut must meet the following requirements:
each cut should be straight (horizontal or vertical);
each cut should go along edges of unit squares (it is prohibited to divide any unit chocolate square with cut);
each cut should go inside the whole chocolate bar, and all cuts must be distinct.
The picture below shows a possible way to cut a 5 × 6 chocolate for 5 times.
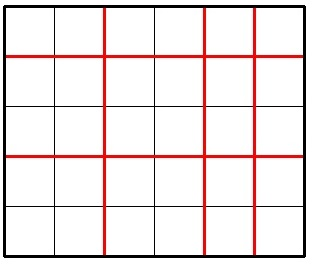
Imagine Jzzhu have made k cuts and the big chocolate is splitted into several pieces. Consider the smallest (by area) piece of the chocolate, Jzzhu wants
this piece to be as large as possible. What is the maximum possible area of smallest piece he can get with exactlyk cuts? The area of a chocolate piece
is the number of unit squares in it.
Input
A single line contains three integers n, m, k (1 ≤ n, m ≤ 109; 1 ≤ k ≤ 2·109).
Output
Output a single integer representing the answer. If it is impossible to cut the big chocolate k times, print -1.
Sample test(s)
input
3 4 1
output
6
input
6 4 2
output
8
input
2 3 4
output
-1
Note
In the first sample, Jzzhu can cut the chocolate following the picture below:
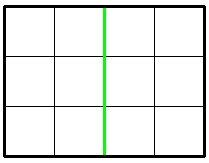
In the second sample the optimal division looks like this:
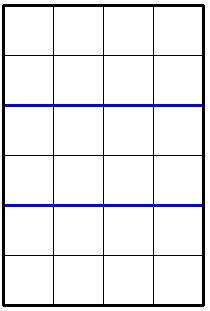
In the third sample, it's impossible to cut a 2 × 3 chocolate 4 times.
题意:给定一个m×n的格子,让切k刀,问最后得到的最小的那块面积最大是多少。 不够切k刀的话输出-1.
We assume that n ≤ m (if n > m,
we can simply swap n and m).
If we finally cut the chocolate into x rows and y columns (1 ≤ x ≤ n, 1 ≤ y ≤ m, x + y = k + 2),
we should maximize the narrowest row and maximize the narrowest column, so the answer will be floor(n / x) * floor(m / y).
There are two algorithms to find the optimal (x, y).
Notice that if x * y is smaller, the answer usually will be better. Then we can find that if k < n,
the optimal (x, y) can only be {x = 1, y = k + 1} or {x = k + 1, y = 1}.
If n ≤ k < m, the optimal (x, y) can
only be {x = 1, y = k + 1}. If m ≤ k ≤ n + m - 2,
the optimal (x, y) can only be {x = k + 2 - m, y = m},
because let t = m - n, n / (k + 2 - m) ≥ (n + t) / (k + 2 - m + t) ≥ 1.
floor(n / x) has at most

values,
so we can enum it and choose the maximum x for each value.
贪心:先把横向或纵向切完,再切另外一个。至于先切横向还是纵向,取最大值。
#include <iostream> #include <cmath> #include <algorithm> #include <cstring> #include <cstdio> #include <vector> #include <set> #include <map> #include <queue> #include <cstdlib> using namespace std; #define rep(i, a, b) for( i = (a); i <= (b); i++) #define reps(i, a, b) for( i = (a); i < (b); i++) #define pb push_back #define ps push #define mp make_pair #define CLR(x,t) memset(x,t,sizeof x) #define LEN(X) strlen(X) #define F first #define S second #define Debug(x) cout<<#x<<"="<<x<<endl; const int inf=~0U>>1; const int MOD = int(1e9) + 7; const double EPS=1e-6; typedef long long LL; int main() { LL n, m, k, a, b; cin >> n >> m >> k; if(k > n + m - 2) {cout << "-1" << endl; return 0;} if(n > m) swap(m, n);// m is max if(k < m) { a = n / (k + 1) * m; b = m / (k + 1) * n; cout << max(a, b) << endl; } else { a = n / (k - m + 2); b = m / (k - n + 2); cout << max(a, b) << endl; } return 0; }
D. Jzzhu and Cities
time limit per test
2 seconds
memory limit per test
256 megabytes
input
standard input
output
standard output
Jzzhu is the president of country A. There are n cities numbered from 1 to n in
his country. City 1 is the capital of A. Also there are mroads
connecting the cities. One can go from city ui to vi (and
vise versa) using the i-th road, the length of this road is xi.
Finally, there are k train routes in the country. One can use the i-th
train route to go from capital of the country to city si (and
vise versa), the length of this route is yi.
Jzzhu doesn't want to waste the money of the country, so he is going to close some of the train routes. Please tell Jzzhu the maximum number of the train routes which can be closed under the following condition: the length of the shortest path from every city
to the capital mustn't change.
Input
The first line contains three integers n, m, k (2 ≤ n ≤ 105; 1 ≤ m ≤ 3·105; 1 ≤ k ≤ 105).
Each of the next m lines contains three integers ui, vi, xi (1 ≤ ui, vi ≤ n; ui ≠ vi; 1 ≤ xi ≤ 109).
Each of the next k lines contains two integers si and yi (2 ≤ si ≤ n; 1 ≤ yi ≤ 109).
It is guaranteed that there is at least one way from every city to the capital. Note, that there can be multiple roads between two cities. Also, there can be multiple routes going to the same city from the capital.
Output
Output a single integer representing the maximum number of the train routes which can be closed.
Sample test(s)
input
5 5 3
1 2 12 3 21 3 3
3 4 41 5 5
3 5
4 5
5 5
output
2
input
2 2 3
1 2 22 1 3
2 12 22 3
output
2
题意:n个城市,其中1号城市是首都,m条双向道路,给定长度。k条双向火车,是直接在某个城市和首都之间往返。
可能删除一些火车路之后,使得所有城市到首都的最短长度不变,问最多可以删除多少个。
题目保证肯定每个城市可以到首都,两个城市之间可能有多条路。
E. Jzzhu and Apples
time limit per test
1 second
memory limit per test
256 megabytes
input
standard input
output
standard output
Jzzhu has picked n apples from his big apple tree. All the apples are numbered from 1 to n.
Now he wants to sell them to an apple store.
Jzzhu will pack his apples into groups and then sell them. Each group must contain two apples, and the greatest common divisor of numbers of the apples in each group must be greater than 1. Of course, each apple can be part of at most one group.
Jzzhu wonders how to get the maximum possible number of groups. Can you help him?
Input
A single integer n (1 ≤ n ≤ 105),
the number of the apples.
Output
The first line must contain a single integer m, representing the maximum number of groups he can get. Each of the next m lines
must contain two integers — the numbers of apples in the current group.
If there are several optimal answers you can print any of them.
Sample test(s)
input
6
output
26 3
2 4
input
9
output
3
9 3
2 46 8
input
2
output
0
题意:给1-N这N个数,问能最多分多少组。 其中每组两个数,这两个数的gcd必须大于1.
输出组数,及怎么分组。(任意一种就行)
官方题解:注意到1和大于N/2的素数是不可能被分组的,直接无视。 然后对于 2 < p <= N/2的素数p, 我们考虑所有未匹配的且有因子p的数的个数,若为偶数个(包含p),正好匹配,否则,我们匹配除了2*p之外的这偶数个。
最后剩下的数都是偶数,两个两个匹配。
#include <iostream> using namespace std; /* CF Round div2 E / div1 C dezhonger */ const int maxn = 100000 + 10; int index; int v[maxn]; int ans[maxn]; bool judge(int x) { for(int i = 2; i * i <= x; i++ ) if(x % i == 0) return false; return true; } int main() { int N, i, j; cin >> N; for(i = 3; i <= N / 2; i++) { if( !judge(i) ) continue; int k = 0; for(j = i; j <= N; j += i) { if( !v[j] ) k++; } if(k & 1 && k > 1) //k is odd { for(j = i; j <= N; j += i ) { if(j == i * 2) continue; if(!v[j]) { ans[index++] = j; v[j] = 1; } } } else // k is even { for(j = i; j <= N; j += i ) { if(!v[j]) { ans[index++] = j; v[j] = 1; } } } } // cout << "index = " << index << endl; for(i = 2; i <= N; i += 2) { if(!v[i]) { ans[index++] = i; } } // cout << "index = " << index << endl; cout << index / 2 << endl; for(i = 0; i < index / 2; i++ ) { cout << ans[2 * i] << " "; cout << ans[2 * i + 1] << endl; } return 0; }
和官方题解,没有判断素数也能ac稍有不同。
#include <iostream> using namespace std; /* CF Round div2 E / div1 C dezhonger */ const int maxn = 100000 + 10; int index; int v[maxn]; int ans[maxn]; int main() { int N, i, j; cin >> N; for(i = 3; i <= N / 2; i++) { int k = 0; for(j = i; j <= N; j += i) { if( !v[j] ) k++; } if( k & 1 && k > 1) //k is odd, when k equlas 1, then will go to "else" statement. { for(j = i; j <= N; j += i ) { if(j == i * 2) continue; if(!v[j]) { ans[index++] = j; v[j] = 1; } } } else // k is even { if(k == 1) continue; //only one, conitnue; for(j = i; j <= N; j += i ) { if(!v[j]) { ans[index++] = j; v[j] = 1; } } } } // cout << "index = " << index << endl; for(i = 2; i <= N; i += 2) { if(!v[i]) { ans[index++] = i; } } // cout << "index = " << index << endl; cout << index / 2 << endl; for(i = 0; i < index / 2; i++ ) { cout << ans[2 * i] << " "; cout << ans[2 * i + 1] << endl; } return 0; }
相关文章推荐
- 【剁手】CodeForce Round 168 Div B1(熬夜就水出来一个我操)
- Codeforces Round #257 (Div. 1) D. Jzzhu and Numbers
- Codeforces Round #257 (Div. 1)449A - Jzzhu and Chocolate(贪心、数学)
- Codeforces Round #257 (Div. 2) C题
- Codeforces Round #257 (Div. 1)B题Jzzhu and Cities(spfa+slf优化)
- Codeforces Round #257 (Div. 2)E(数论+构造)
- Codeforce Round #211 Div2
- Codeforce Round #220 Div2
- Codeforce Round #224 Div2
- Codeforce Round #226 Div2
- Codeforce Round #228 Div2
- codeforce Round #232 Div2 map + 质因子 +组合
- CodeForce Round 219 Div2 E Watching Fireworks is Fun 单调队列DP
- Codeforce Round #382 (div 1) A
- CodeForce Round 215 DIV2 C 使用了线段树 但是多此一举...
- codeforce round 175 div2
- Codeforces Round #278 (Div. 2) B. Candy Boxes [brute force+constructive algorithms]
- Codeforces Round #257 (Div. 2) ABCD
- CodeForce Round 258 div2
- CodeForce Round 257 div1