黑马程序员_javaIO流
2014-05-12 18:50
387 查看
------- android培训、java培训、期待与您交流! ----------
IO流用来处理设备之间的数据传输。
java对数据的操作是通过流的方式。
java用于操作流的对象都在IO包中。
流按照操作数据分为两种:字节流和字符流。
流按照流向分为:输入流和输出流。
IO流常用基类
字节流的抽象基类:
InputStream,OutputStream
字符流的抽象基类:
Reader,Writer
字符流
字符流继承体系图示:
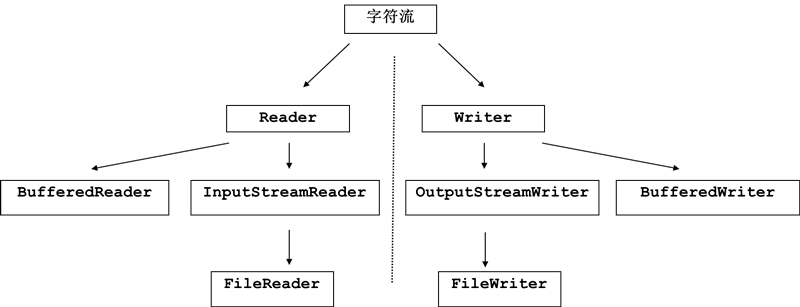
向文件中写入数据
public FileWriter(File file,boolean append):将append的值设置为true表示追加。
从文件中读取内容
字节流
字节流继承体系图示:
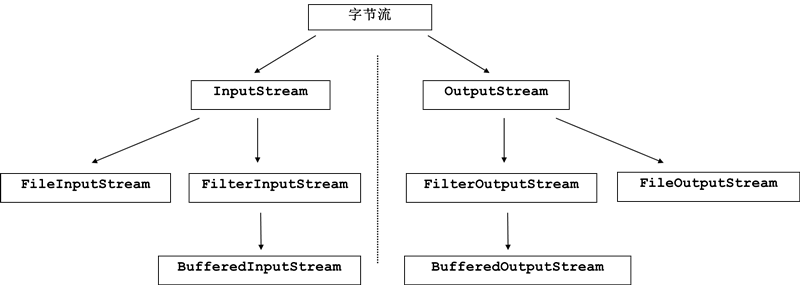
综合案例:
File类
用来将文件或者文件夹封装成对象,方便对文件和文件夹的属性信息进行操作。
File对象可以作为参数传递给流的构造函数。
File类常见方法:
1.创建
boolean createNewFile():在指定位置创建文件,如果该文件已经存在,则不创建,返回false。
和输出流不一样,输出流对象一建立创建文件。而且文件已经存在,会覆盖。
boolean mkdir():创建文件夹。
boolean mkdirs():创建多级文件夹。
2.删除
boolean delete():删除失败返回false。如果文件正在被使用,则删除不了返回falsel。
void deleteOnExit();在程序退出时删除指定文件。
3.判断
boolean exists() :文件是否存在.
isFile():
isDirectory();
isHidden();
isAbsolute();
4.获取信息
getName():
getPath():
getParent():
用递归的调用方式列出指定目录下的全部内容
Properties类
Properties是Hashtable的子类。
也就是说它具备map集合的特点。而且它里面存储的键值对都是字符串。
特点:
1.可以持久化存储数据。
2.键值都是字符串。
3.一般用于配置文件。
常见操作:
load():将流中的数据加载进集合。
store():写入各个项后,刷新输出流。
list():将集合的键值数据列出到指定的目的地。
IO包中的其他流
打印流
该流提供了打印方法,可以将各种数据类型的数据都原样打印。
字节打印流:
PrintStream
构造函数可以接收的参数类型:
1,file对象。File
2,字符串路径。String
3,字节输出流。OutputStream
字符打印流:
PrintWriter
构造函数可以接收的参数类型:
1,file对象。File
2,字符串路径。String
3,字节输出流。OutputStream
4,字符输出流,Writer。
例:
序列流
该类不算是IO体系中的子类,而是直接继承自Object。但是它是IO包中成员,因为它具备读和写功能。
内部封装了一个数组,而且通过指针对数组的元素进行操作。
可以通过getFilePointer获取指针位置,同时可以通过seek改变指针的位置。
其实完成读写的原理就是内部封装了字节输入流和输出流。
通过构造函数可以看出,该类只能操作文件。
而且操作文件还有模式:只读r,,读写rw等。
如果模式为只读 r。不会创建文件。会去读取一个已存在文件,如果该文件不存在,则会出现异常。
如果模式rw。操作的文件不存在,会自动创建。如果存则不会覆盖。
主要作用是可以进行两个线程间的通信,分为管道输出流和管道输入流。
IO流用来处理设备之间的数据传输。
java对数据的操作是通过流的方式。
java用于操作流的对象都在IO包中。
流按照操作数据分为两种:字节流和字符流。
流按照流向分为:输入流和输出流。
IO流常用基类
字节流的抽象基类:
InputStream,OutputStream
字符流的抽象基类:
Reader,Writer
字符流
字符流继承体系图示:
向文件中写入数据
class FileWriterDemo { public static void main(String[] args) { FileWriter fw = null; try { fw = new FileWriter("demo.txt"); fw.write("abcdefg"); } catch (IOException e) { e.printStackTrace(); } finally { try { if(fw!=null) fw.close(); } catch (IOException e) { System.out.println(e.toString()); } } } }在使用字符流操作时,也可以实现文件的追加功能,直接使用FileWriter类中的以下构造即可实现追加:
public FileWriter(File file,boolean append):将append的值设置为true表示追加。
从文件中读取内容
import java.io.*; class FileReaderDemo { public static void main(String[] args) throws IOException { FileReader fr = new FileReader("demo.txt"); //定义一个字符数组。用于存储读到字符。 //该read(char[])返回的是读到字符个数。 char[] buf = new char[1024]; int num = 0; while((num=fr.read(buf))!=-1) { System.out.println(new String(buf,0,num)); } fr.close(); } }例:
/* 通过缓冲区复制一个.java文件。 */ import java.io.*; class CopyTextByBuf { public static void main(String[] args) { BufferedReader bufr = null; BufferedWriter bufw = null; try { bufr = new BufferedReader(new FileReader("BufferedWriterDemo.java")); bufw = new BufferedWriter(new FileWriter("bufWriter_Copy.txt")); String line = null; while((line=bufr.readLine())!=null) { bufw.write(line); bufw.newLine(); bufw.flush(); } } catch (IOException e) { throw new RuntimeException("读写失败"); } finally { try { if(bufr!=null) bufr.close(); } catch (IOException e) { throw new RuntimeException("读取关闭失败"); } try { if(bufw!=null) bufw.close(); } catch (IOException e) { throw new RuntimeException("写入关闭失败"); } } } }
字节流
字节流继承体系图示:
综合案例:
import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; /** * 使用带缓冲功能的字节流复制文件。 * 思路: * 1.创建copyFile方法,该方法接收两个参数,一个是源文件的完整路径srcPath,另一个是源文件所要复制到的目标目录destDir。 * 2.进行一系列的判断: 源文件是否存在? 目标目录是否存在?如果不存在,系统将自动进行创建。 目标目录下是否有同名文件? * 3.创建输入流和输出流。 * 4.对文件大小进行判断。如果文件过大,会出现内存溢出情况,我们选择每次读一个字节的方法;反之,我们用数组接收读取到的字节,然后再把数组的数据一次性写入输出流。 * 5.最后关闭资源。 * @author Administrator */ public class Test { public static void main(String[] args) { String srcPath = args[0]; String destDir = args[1]; CopyFile.copyFile(srcPath,destDir); } } class CopyFile { public static void copyFile(String srcPath,String destDir) { File srcFilePath = new File(srcPath); File destFileDir = new File(destDir); //判断源文件是否存在 if(srcFilePath.exists() == false || srcFilePath.isFile() == false) { System.out.println("无效的文件,请检查!"); System.exit(0); } //判断所复制到的目标目录是否存在 if(destFileDir.isDirectory() == false) { System.out.println("您指定的目录不存在,系统将帮您自动创建!"); destFileDir.mkdirs(); } //判断目标目录下是否有同名文件 File destFilePath = new File(destFileDir + "\\" +srcFilePath.getName()); if (destFilePath.isFile()) { System.out.println("您指定的目录下具有同名文件!"); System.exit(0); } //创建输入流和输出流,并对不同大小的文件分别处理。 BufferedInputStream bis = null; BufferedOutputStream bos = null; try { FileInputStream fis = new FileInputStream(srcFilePath); bis = new BufferedInputStream(fis); FileOutputStream fos = new FileOutputStream(destFilePath); bos = new BufferedOutputStream(fos); long len = bis.available(); if(len > 1024*1024*200) { int b; while((b=bis.read())!=-1) { bos.write(b); } } else { byte[] bytes = new byte[(int)len]; bis.read(bytes); bos.write(bytes); } bis.close(); bos.close(); System.out.println("文件复制已经完成!"); } catch (Exception e) { e.printStackTrace(); } } }
File类
用来将文件或者文件夹封装成对象,方便对文件和文件夹的属性信息进行操作。
File对象可以作为参数传递给流的构造函数。
File类常见方法:
1.创建
boolean createNewFile():在指定位置创建文件,如果该文件已经存在,则不创建,返回false。
和输出流不一样,输出流对象一建立创建文件。而且文件已经存在,会覆盖。
boolean mkdir():创建文件夹。
boolean mkdirs():创建多级文件夹。
2.删除
boolean delete():删除失败返回false。如果文件正在被使用,则删除不了返回falsel。
void deleteOnExit();在程序退出时删除指定文件。
3.判断
boolean exists() :文件是否存在.
isFile():
isDirectory();
isHidden();
isAbsolute();
4.获取信息
getName():
getPath():
getParent():
用递归的调用方式列出指定目录下的全部内容
import java.io.File; public class FileDemo { public static void main(String[] args) { File directory = new File("D:" + File.separator); print(directory); } public static void print(File file) { if(file != null) { if(file.isDirectory())//判断是否是目录 { File[] f = file.listFiles();//如果是目录,则列出全部的内容 if(f != null) { for(int i=0;i<f.length;i++) { print(f[i]); } } } } else { System.out.println(file);//如果不是目录,则直接打印路径信息 } } }
Properties类
Properties是Hashtable的子类。
也就是说它具备map集合的特点。而且它里面存储的键值对都是字符串。
特点:
1.可以持久化存储数据。
2.键值都是字符串。
3.一般用于配置文件。
常见操作:
load():将流中的数据加载进集合。
store():写入各个项后,刷新输出流。
list():将集合的键值数据列出到指定的目的地。
IO包中的其他流
打印流
该流提供了打印方法,可以将各种数据类型的数据都原样打印。
字节打印流:
PrintStream
构造函数可以接收的参数类型:
1,file对象。File
2,字符串路径。String
3,字节输出流。OutputStream
字符打印流:
PrintWriter
构造函数可以接收的参数类型:
1,file对象。File
2,字符串路径。String
3,字节输出流。OutputStream
4,字符输出流,Writer。
例:
import java.io.*; class PrintStr 4000 eamDemo { public static void main(String[] args) throws IOException { BufferedReader bufr = new BufferedReader(new InputStreamReader(System.in)); PrintWriter out = new PrintWriter(new FileWriter("a.txt"),true); String line = null; while((line=bufr.readLine())!=null) { if("over".equals(line)) break; out.println(line.toUpperCase()); } out.close(); bufr.close(); } }
序列流
import java.io.*; import java.util.*; class SplitFile { public static void main(String[] args) throws IOException { splitFile(); merge(); } public static void merge()throws IOException { ArrayList<FileInputStream> al = new ArrayList<FileInputStream>(); for(int x=1; x<=3; x++) { al.add(new FileInputStream("c:\\splitfiles\\"+x+".part")); } final Iterator<FileInputStream> it = al.iterator(); Enumeration<FileInputStream> en = new Enumeration<FileInputStream>() { public boolean hasMoreElements() { return it.hasNext(); } public FileInputStream nextElement() { return it.next(); } }; SequenceInputStream sis = new SequenceInputStream(en); FileOutputStream fos = new FileOutputStream("c:\\splitfiles\\0.bmp"); byte[] buf = new byte[1024]; int len = 0; while((len=sis.read(buf))!=-1) { fos.write(buf,0,len); } fos.close(); sis.close(); } public static void splitFile()throws IOException { FileInputStream fis = new FileInputStream("c:\\1.bmp"); FileOutputStream fos = null; byte[] buf = new byte[1024*1024]; int len = 0; int count = 1; while((len=fis.read(buf))!=-1) { fos = new FileOutputStream("c:\\splitfiles\\"+(count++)+".part"); fos.write(buf,0,len); fos.close(); } fis.close(); } }RandomAccessFile类
该类不算是IO体系中的子类,而是直接继承自Object。但是它是IO包中成员,因为它具备读和写功能。
内部封装了一个数组,而且通过指针对数组的元素进行操作。
可以通过getFilePointer获取指针位置,同时可以通过seek改变指针的位置。
其实完成读写的原理就是内部封装了字节输入流和输出流。
通过构造函数可以看出,该类只能操作文件。
而且操作文件还有模式:只读r,,读写rw等。
如果模式为只读 r。不会创建文件。会去读取一个已存在文件,如果该文件不存在,则会出现异常。
如果模式rw。操作的文件不存在,会自动创建。如果存则不会覆盖。
class RandomAccessFileDemo { public static void main(String[] args) throws IOException { writeFile() readFile(); } public static void readFile()throws IOException { RandomAccessFile raf = new RandomAccessFile("ran.txt","r"); //跳过指定的字节数 raf.skipBytes(8); byte[] buf = new byte[4]; raf.read(buf); String name = new String(buf); int age = raf.readInt(); System.out.println("name="+name); System.out.println("age="+age); raf.close(); } public static void writeFile()throws IOException { RandomAccessFile raf = new RandomAccessFile("ran.txt","rw"); raf.write("李四".getBytes()); raf.writeInt(97); raf.write("王五".getBytes()); raf.writeInt(99); raf.close(); } }管道流
主要作用是可以进行两个线程间的通信,分为管道输出流和管道输入流。
import java.io.*; class Read implements Runnable { private PipedInputStream in; Read(PipedInputStream in) { this.in = in; } public void run() { try { byte[] buf = new byte[1024]; System.out.println("读取前。。没有数据阻塞"); int len = in.read(buf); System.out.println("读到数据。。阻塞结束"); String s= new String(buf,0,len); System.out.println(s); in.close(); } catch (IOException e) { throw new RuntimeException("管道读取流失败"); } } } class Write implements Runnable { private PipedOutputStream out; Write(PipedOutputStream out) { this.out = out; } public void run() { try { System.out.println("开始写入数据,等待6秒后。"); Thread.sleep(6000); out.write("piped lai la".getBytes()); out.close(); } catch (Exception e) { throw new RuntimeException("管道输出流失败"); } } } class PipedStreamDemo { public static void main(String[] args) throws IOException { PipedInputStream in = new PipedInputStream(); PipedOutputStream out = new PipedOutputStream(); in.connect(out); Read r = new Read(in); Write w = new Write(out); new Thread(r).start(); new Thread(w).start(); } }
相关文章推荐
- 黑马程序员_JavaIO流(四)
- 黑马程序员_JavaIO流(一)
- 黑马程序员—JavaIO流
- 黑马程序员_JavaIO流(二)
- 黑马程序员_ javaIO流的一些总结
- 黑马程序员_JavaIO流(三)
- 黑马程序员——JavaIO流
- 黑马程序员---javaIO流
- 黑马程序员_javaIO流_2
- 黑马程序员-----javaIO流总结*
- 黑马程序员_java基础视频第19天_JavaIO流续1
- 黑马程序员_javaIO流(一)
- 黑马程序员_java基础视频第20天_JavaIO流续2
- 黑马程序员_javaIO流(二)
- 黑马程序员-动态代理
- 黑马程序员_基础加强_1
- 黑马程序员_<<基础加强--1.5新特性(泛型)(下)>>
- 黑马程序员_OC中的Foundation框架学习
- 黑马程序员----把Eclipse修改为黑色主题颜色方案
- 黑马程序员——GUI(1)基本窗体组件